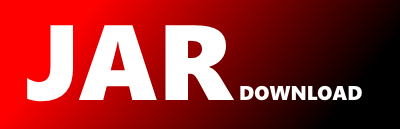
com.twilio.rest.chat.v2.service.user.UserChannel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.chat.v2.service.user;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.Map;
import java.util.Objects;
@JsonIgnoreProperties(ignoreUnknown = true)
public class UserChannel extends Resource {
private static final long serialVersionUID = 97342858560464L;
public enum ChannelStatus {
JOINED("joined"),
INVITED("invited"),
NOT_PARTICIPATING("not_participating");
private final String value;
private ChannelStatus(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a ChannelStatus from a string.
* @param value string value
* @return generated ChannelStatus
*/
@JsonCreator
public static ChannelStatus forValue(final String value) {
return Promoter.enumFromString(value, ChannelStatus.values());
}
}
public enum NotificationLevel {
DEFAULT("default"),
MUTED("muted");
private final String value;
private NotificationLevel(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a NotificationLevel from a string.
* @param value string value
* @return generated NotificationLevel
*/
@JsonCreator
public static NotificationLevel forValue(final String value) {
return Promoter.enumFromString(value, NotificationLevel.values());
}
}
/**
* Create a UserChannelReader to execute read.
*
* @param pathServiceSid The SID of the Service to read the resources from
* @param pathUserSid The SID of the User to fetch the User Channel resources
* from
* @return UserChannelReader capable of executing the read
*/
public static UserChannelReader reader(final String pathServiceSid,
final String pathUserSid) {
return new UserChannelReader(pathServiceSid, pathUserSid);
}
/**
* Create a UserChannelFetcher to execute fetch.
*
* @param pathServiceSid The SID of the Service to fetch the User Channel
* resource from
* @param pathUserSid The SID of the User to fetch the User Channel resource
* from
* @param pathChannelSid The SID of the Channel that has the User Channel to
* fetch
* @return UserChannelFetcher capable of executing the fetch
*/
public static UserChannelFetcher fetcher(final String pathServiceSid,
final String pathUserSid,
final String pathChannelSid) {
return new UserChannelFetcher(pathServiceSid, pathUserSid, pathChannelSid);
}
/**
* Create a UserChannelUpdater to execute update.
*
* @param pathServiceSid The SID of the Service to update the resource from
* @param pathUserSid The SID of the User to update the User Channel resource
* from
* @param pathChannelSid The SID of the Channel with the User Channel resource
* to update
* @param notificationLevel The push notification level to assign to the User
* Channel
* @return UserChannelUpdater capable of executing the update
*/
public static UserChannelUpdater updater(final String pathServiceSid,
final String pathUserSid,
final String pathChannelSid,
final UserChannel.NotificationLevel notificationLevel) {
return new UserChannelUpdater(pathServiceSid, pathUserSid, pathChannelSid, notificationLevel);
}
/**
* Converts a JSON String into a UserChannel object using the provided
* ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return UserChannel object represented by the provided JSON
*/
public static UserChannel fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, UserChannel.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a UserChannel object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return UserChannel object represented by the provided JSON
*/
public static UserChannel fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, UserChannel.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String accountSid;
private final String serviceSid;
private final String channelSid;
private final String userSid;
private final String memberSid;
private final UserChannel.ChannelStatus status;
private final Integer lastConsumedMessageIndex;
private final Integer unreadMessagesCount;
private final Map links;
private final URI url;
private final UserChannel.NotificationLevel notificationLevel;
@JsonCreator
private UserChannel(@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("service_sid")
final String serviceSid,
@JsonProperty("channel_sid")
final String channelSid,
@JsonProperty("user_sid")
final String userSid,
@JsonProperty("member_sid")
final String memberSid,
@JsonProperty("status")
final UserChannel.ChannelStatus status,
@JsonProperty("last_consumed_message_index")
final Integer lastConsumedMessageIndex,
@JsonProperty("unread_messages_count")
final Integer unreadMessagesCount,
@JsonProperty("links")
final Map links,
@JsonProperty("url")
final URI url,
@JsonProperty("notification_level")
final UserChannel.NotificationLevel notificationLevel) {
this.accountSid = accountSid;
this.serviceSid = serviceSid;
this.channelSid = channelSid;
this.userSid = userSid;
this.memberSid = memberSid;
this.status = status;
this.lastConsumedMessageIndex = lastConsumedMessageIndex;
this.unreadMessagesCount = unreadMessagesCount;
this.links = links;
this.url = url;
this.notificationLevel = notificationLevel;
}
/**
* Returns The The SID of the Account that created the resource.
*
* @return The SID of the Account that created the resource
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The The SID of the Service that the resource is associated with.
*
* @return The SID of the Service that the resource is associated with
*/
public final String getServiceSid() {
return this.serviceSid;
}
/**
* Returns The The SID of the Channel the resource belongs to.
*
* @return The SID of the Channel the resource belongs to
*/
public final String getChannelSid() {
return this.channelSid;
}
/**
* Returns The The SID of the User the User Channel belongs to.
*
* @return The SID of the User the User Channel belongs to
*/
public final String getUserSid() {
return this.userSid;
}
/**
* Returns The The SID of the User as a Member in the Channel.
*
* @return The SID of the User as a Member in the Channel
*/
public final String getMemberSid() {
return this.memberSid;
}
/**
* Returns The The status of the User on the Channel.
*
* @return The status of the User on the Channel
*/
public final UserChannel.ChannelStatus getStatus() {
return this.status;
}
/**
* Returns The The index of the last Message in the Channel the Member has read.
*
* @return The index of the last Message in the Channel the Member has read
*/
public final Integer getLastConsumedMessageIndex() {
return this.lastConsumedMessageIndex;
}
/**
* Returns The The number of unread Messages in the Channel for the User.
*
* @return The number of unread Messages in the Channel for the User
*/
public final Integer getUnreadMessagesCount() {
return this.unreadMessagesCount;
}
/**
* Returns The Absolute URLs to access the Members, Messages , Invites and, if
* it exists, the last Message for the Channel.
*
* @return Absolute URLs to access the Members, Messages , Invites and, if it
* exists, the last Message for the Channel
*/
public final Map getLinks() {
return this.links;
}
/**
* Returns The The absolute URL of the resource.
*
* @return The absolute URL of the resource
*/
public final URI getUrl() {
return this.url;
}
/**
* Returns The The push notification level of the User for the Channel.
*
* @return The push notification level of the User for the Channel
*/
public final UserChannel.NotificationLevel getNotificationLevel() {
return this.notificationLevel;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserChannel other = (UserChannel) o;
return Objects.equals(accountSid, other.accountSid) &&
Objects.equals(serviceSid, other.serviceSid) &&
Objects.equals(channelSid, other.channelSid) &&
Objects.equals(userSid, other.userSid) &&
Objects.equals(memberSid, other.memberSid) &&
Objects.equals(status, other.status) &&
Objects.equals(lastConsumedMessageIndex, other.lastConsumedMessageIndex) &&
Objects.equals(unreadMessagesCount, other.unreadMessagesCount) &&
Objects.equals(links, other.links) &&
Objects.equals(url, other.url) &&
Objects.equals(notificationLevel, other.notificationLevel);
}
@Override
public int hashCode() {
return Objects.hash(accountSid,
serviceSid,
channelSid,
userSid,
memberSid,
status,
lastConsumedMessageIndex,
unreadMessagesCount,
links,
url,
notificationLevel);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("accountSid", accountSid)
.add("serviceSid", serviceSid)
.add("channelSid", channelSid)
.add("userSid", userSid)
.add("memberSid", memberSid)
.add("status", status)
.add("lastConsumedMessageIndex", lastConsumedMessageIndex)
.add("unreadMessagesCount", unreadMessagesCount)
.add("links", links)
.add("url", url)
.add("notificationLevel", notificationLevel)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy