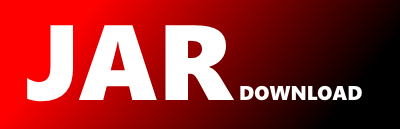
com.twilio.rest.api.v2010.account.message.Feedback Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account.message;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.DateConverter;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
import java.io.IOException;
import java.io.InputStream;
import java.util.Map;
import java.util.Objects;
@JsonIgnoreProperties(ignoreUnknown = true)
public class Feedback extends Resource {
private static final long serialVersionUID = 93685573726475L;
public enum Outcome {
CONFIRMED("confirmed"),
UMCONFIRMED("umconfirmed");
private final String value;
private Outcome(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a Outcome from a string.
* @param value string value
* @return generated Outcome
*/
@JsonCreator
public static Outcome forValue(final String value) {
return Promoter.enumFromString(value, Outcome.values());
}
}
/**
* Create a FeedbackCreator to execute create.
*
* @param pathAccountSid The SID of the Account that will create the resource
* @param pathMessageSid The SID of the Message resource for which the feedback
* was provided
* @return FeedbackCreator capable of executing the create
*/
public static FeedbackCreator creator(final String pathAccountSid,
final String pathMessageSid) {
return new FeedbackCreator(pathAccountSid, pathMessageSid);
}
/**
* Create a FeedbackCreator to execute create.
*
* @param pathMessageSid The SID of the Message resource for which the feedback
* was provided
* @return FeedbackCreator capable of executing the create
*/
public static FeedbackCreator creator(final String pathMessageSid) {
return new FeedbackCreator(pathMessageSid);
}
/**
* Converts a JSON String into a Feedback object using the provided
* ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return Feedback object represented by the provided JSON
*/
public static Feedback fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Feedback.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a Feedback object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return Feedback object represented by the provided JSON
*/
public static Feedback fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Feedback.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String accountSid;
private final String messageSid;
private final Feedback.Outcome outcome;
private final DateTime dateCreated;
private final DateTime dateUpdated;
private final String uri;
@JsonCreator
private Feedback(@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("message_sid")
final String messageSid,
@JsonProperty("outcome")
final Feedback.Outcome outcome,
@JsonProperty("date_created")
final String dateCreated,
@JsonProperty("date_updated")
final String dateUpdated,
@JsonProperty("uri")
final String uri) {
this.accountSid = accountSid;
this.messageSid = messageSid;
this.outcome = outcome;
this.dateCreated = DateConverter.rfc2822DateTimeFromString(dateCreated);
this.dateUpdated = DateConverter.rfc2822DateTimeFromString(dateUpdated);
this.uri = uri;
}
/**
* Returns The The SID of the Account that created the resource.
*
* @return The SID of the Account that created the resource
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The The SID of the Message resource for which the feedback was
* provided.
*
* @return The SID of the Message resource for which the feedback was provided
*/
public final String getMessageSid() {
return this.messageSid;
}
/**
* Returns The Whether the feedback has arrived.
*
* @return Whether the feedback has arrived
*/
public final Feedback.Outcome getOutcome() {
return this.outcome;
}
/**
* Returns The The RFC 2822 date and time in GMT that the resource was created.
*
* @return The RFC 2822 date and time in GMT that the resource was created
*/
public final DateTime getDateCreated() {
return this.dateCreated;
}
/**
* Returns The The RFC 2822 date and time in GMT that the resource was last
* updated.
*
* @return The RFC 2822 date and time in GMT that the resource was last updated
*/
public final DateTime getDateUpdated() {
return this.dateUpdated;
}
/**
* Returns The The URI of the resource, relative to `https://api.twilio.com`.
*
* @return The URI of the resource, relative to `https://api.twilio.com`
*/
public final String getUri() {
return this.uri;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Feedback other = (Feedback) o;
return Objects.equals(accountSid, other.accountSid) &&
Objects.equals(messageSid, other.messageSid) &&
Objects.equals(outcome, other.outcome) &&
Objects.equals(dateCreated, other.dateCreated) &&
Objects.equals(dateUpdated, other.dateUpdated) &&
Objects.equals(uri, other.uri);
}
@Override
public int hashCode() {
return Objects.hash(accountSid,
messageSid,
outcome,
dateCreated,
dateUpdated,
uri);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("accountSid", accountSid)
.add("messageSid", messageSid)
.add("outcome", outcome)
.add("dateCreated", dateCreated)
.add("dateUpdated", dateUpdated)
.add("uri", uri)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy