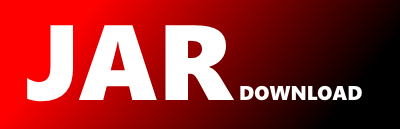
com.twilio.rest.preview.wireless.SimUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.preview.wireless;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
/**
* PLEASE NOTE that this class contains preview products that are subject to
* change. Use them with caution. If you currently do not have developer preview
* access, please contact [email protected].
*/
public class SimUpdater extends Updater {
private final String pathSid;
private String uniqueName;
private String callbackMethod;
private URI callbackUrl;
private String friendlyName;
private String ratePlan;
private String status;
private HttpMethod commandsCallbackMethod;
private URI commandsCallbackUrl;
private HttpMethod smsFallbackMethod;
private URI smsFallbackUrl;
private HttpMethod smsMethod;
private URI smsUrl;
private HttpMethod voiceFallbackMethod;
private URI voiceFallbackUrl;
private HttpMethod voiceMethod;
private URI voiceUrl;
/**
* Construct a new SimUpdater.
*
* @param pathSid The sid
*/
public SimUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* The unique_name.
*
* @param uniqueName The unique_name
* @return this
*/
public SimUpdater setUniqueName(final String uniqueName) {
this.uniqueName = uniqueName;
return this;
}
/**
* The callback_method.
*
* @param callbackMethod The callback_method
* @return this
*/
public SimUpdater setCallbackMethod(final String callbackMethod) {
this.callbackMethod = callbackMethod;
return this;
}
/**
* The callback_url.
*
* @param callbackUrl The callback_url
* @return this
*/
public SimUpdater setCallbackUrl(final URI callbackUrl) {
this.callbackUrl = callbackUrl;
return this;
}
/**
* The callback_url.
*
* @param callbackUrl The callback_url
* @return this
*/
public SimUpdater setCallbackUrl(final String callbackUrl) {
return setCallbackUrl(Promoter.uriFromString(callbackUrl));
}
/**
* The friendly_name.
*
* @param friendlyName The friendly_name
* @return this
*/
public SimUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The rate_plan.
*
* @param ratePlan The rate_plan
* @return this
*/
public SimUpdater setRatePlan(final String ratePlan) {
this.ratePlan = ratePlan;
return this;
}
/**
* The status.
*
* @param status The status
* @return this
*/
public SimUpdater setStatus(final String status) {
this.status = status;
return this;
}
/**
* The commands_callback_method.
*
* @param commandsCallbackMethod The commands_callback_method
* @return this
*/
public SimUpdater setCommandsCallbackMethod(final HttpMethod commandsCallbackMethod) {
this.commandsCallbackMethod = commandsCallbackMethod;
return this;
}
/**
* The commands_callback_url.
*
* @param commandsCallbackUrl The commands_callback_url
* @return this
*/
public SimUpdater setCommandsCallbackUrl(final URI commandsCallbackUrl) {
this.commandsCallbackUrl = commandsCallbackUrl;
return this;
}
/**
* The commands_callback_url.
*
* @param commandsCallbackUrl The commands_callback_url
* @return this
*/
public SimUpdater setCommandsCallbackUrl(final String commandsCallbackUrl) {
return setCommandsCallbackUrl(Promoter.uriFromString(commandsCallbackUrl));
}
/**
* The sms_fallback_method.
*
* @param smsFallbackMethod The sms_fallback_method
* @return this
*/
public SimUpdater setSmsFallbackMethod(final HttpMethod smsFallbackMethod) {
this.smsFallbackMethod = smsFallbackMethod;
return this;
}
/**
* The sms_fallback_url.
*
* @param smsFallbackUrl The sms_fallback_url
* @return this
*/
public SimUpdater setSmsFallbackUrl(final URI smsFallbackUrl) {
this.smsFallbackUrl = smsFallbackUrl;
return this;
}
/**
* The sms_fallback_url.
*
* @param smsFallbackUrl The sms_fallback_url
* @return this
*/
public SimUpdater setSmsFallbackUrl(final String smsFallbackUrl) {
return setSmsFallbackUrl(Promoter.uriFromString(smsFallbackUrl));
}
/**
* The sms_method.
*
* @param smsMethod The sms_method
* @return this
*/
public SimUpdater setSmsMethod(final HttpMethod smsMethod) {
this.smsMethod = smsMethod;
return this;
}
/**
* The sms_url.
*
* @param smsUrl The sms_url
* @return this
*/
public SimUpdater setSmsUrl(final URI smsUrl) {
this.smsUrl = smsUrl;
return this;
}
/**
* The sms_url.
*
* @param smsUrl The sms_url
* @return this
*/
public SimUpdater setSmsUrl(final String smsUrl) {
return setSmsUrl(Promoter.uriFromString(smsUrl));
}
/**
* The voice_fallback_method.
*
* @param voiceFallbackMethod The voice_fallback_method
* @return this
*/
public SimUpdater setVoiceFallbackMethod(final HttpMethod voiceFallbackMethod) {
this.voiceFallbackMethod = voiceFallbackMethod;
return this;
}
/**
* The voice_fallback_url.
*
* @param voiceFallbackUrl The voice_fallback_url
* @return this
*/
public SimUpdater setVoiceFallbackUrl(final URI voiceFallbackUrl) {
this.voiceFallbackUrl = voiceFallbackUrl;
return this;
}
/**
* The voice_fallback_url.
*
* @param voiceFallbackUrl The voice_fallback_url
* @return this
*/
public SimUpdater setVoiceFallbackUrl(final String voiceFallbackUrl) {
return setVoiceFallbackUrl(Promoter.uriFromString(voiceFallbackUrl));
}
/**
* The voice_method.
*
* @param voiceMethod The voice_method
* @return this
*/
public SimUpdater setVoiceMethod(final HttpMethod voiceMethod) {
this.voiceMethod = voiceMethod;
return this;
}
/**
* The voice_url.
*
* @param voiceUrl The voice_url
* @return this
*/
public SimUpdater setVoiceUrl(final URI voiceUrl) {
this.voiceUrl = voiceUrl;
return this;
}
/**
* The voice_url.
*
* @param voiceUrl The voice_url
* @return this
*/
public SimUpdater setVoiceUrl(final String voiceUrl) {
return setVoiceUrl(Promoter.uriFromString(voiceUrl));
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Sim
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Sim update(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.PREVIEW.toString(),
"/wireless/Sims/" + this.pathSid + "",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Sim update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Sim.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (uniqueName != null) {
request.addPostParam("UniqueName", uniqueName);
}
if (callbackMethod != null) {
request.addPostParam("CallbackMethod", callbackMethod);
}
if (callbackUrl != null) {
request.addPostParam("CallbackUrl", callbackUrl.toString());
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (ratePlan != null) {
request.addPostParam("RatePlan", ratePlan.toString());
}
if (status != null) {
request.addPostParam("Status", status);
}
if (commandsCallbackMethod != null) {
request.addPostParam("CommandsCallbackMethod", commandsCallbackMethod.toString());
}
if (commandsCallbackUrl != null) {
request.addPostParam("CommandsCallbackUrl", commandsCallbackUrl.toString());
}
if (smsFallbackMethod != null) {
request.addPostParam("SmsFallbackMethod", smsFallbackMethod.toString());
}
if (smsFallbackUrl != null) {
request.addPostParam("SmsFallbackUrl", smsFallbackUrl.toString());
}
if (smsMethod != null) {
request.addPostParam("SmsMethod", smsMethod.toString());
}
if (smsUrl != null) {
request.addPostParam("SmsUrl", smsUrl.toString());
}
if (voiceFallbackMethod != null) {
request.addPostParam("VoiceFallbackMethod", voiceFallbackMethod.toString());
}
if (voiceFallbackUrl != null) {
request.addPostParam("VoiceFallbackUrl", voiceFallbackUrl.toString());
}
if (voiceMethod != null) {
request.addPostParam("VoiceMethod", voiceMethod.toString());
}
if (voiceUrl != null) {
request.addPostParam("VoiceUrl", voiceUrl.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy