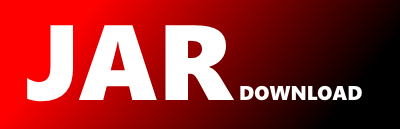
com.twilio.rest.sync.v1.service.SyncList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.sync.v1.service;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.DateConverter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.Map;
import java.util.Objects;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class SyncList extends Resource {
private static final long serialVersionUID = 113063121662943L;
/**
* Create a SyncListFetcher to execute fetch.
*
* @param pathServiceSid The service_sid
* @param pathSid The sid
* @return SyncListFetcher capable of executing the fetch
*/
public static SyncListFetcher fetcher(final String pathServiceSid,
final String pathSid) {
return new SyncListFetcher(pathServiceSid, pathSid);
}
/**
* Create a SyncListDeleter to execute delete.
*
* @param pathServiceSid The service_sid
* @param pathSid The sid
* @return SyncListDeleter capable of executing the delete
*/
public static SyncListDeleter deleter(final String pathServiceSid,
final String pathSid) {
return new SyncListDeleter(pathServiceSid, pathSid);
}
/**
* Create a SyncListCreator to execute create.
*
* @param pathServiceSid The service_sid
* @return SyncListCreator capable of executing the create
*/
public static SyncListCreator creator(final String pathServiceSid) {
return new SyncListCreator(pathServiceSid);
}
/**
* Create a SyncListUpdater to execute update.
*
* @param pathServiceSid The service_sid
* @param pathSid The sid
* @return SyncListUpdater capable of executing the update
*/
public static SyncListUpdater updater(final String pathServiceSid,
final String pathSid) {
return new SyncListUpdater(pathServiceSid, pathSid);
}
/**
* Create a SyncListReader to execute read.
*
* @param pathServiceSid The service_sid
* @return SyncListReader capable of executing the read
*/
public static SyncListReader reader(final String pathServiceSid) {
return new SyncListReader(pathServiceSid);
}
/**
* Converts a JSON String into a SyncList object using the provided
* ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return SyncList object represented by the provided JSON
*/
public static SyncList fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, SyncList.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a SyncList object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return SyncList object represented by the provided JSON
*/
public static SyncList fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, SyncList.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final String uniqueName;
private final String accountSid;
private final String serviceSid;
private final URI url;
private final Map links;
private final String revision;
private final DateTime dateExpires;
private final DateTime dateCreated;
private final DateTime dateUpdated;
private final String createdBy;
@JsonCreator
private SyncList(@JsonProperty("sid")
final String sid,
@JsonProperty("unique_name")
final String uniqueName,
@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("service_sid")
final String serviceSid,
@JsonProperty("url")
final URI url,
@JsonProperty("links")
final Map links,
@JsonProperty("revision")
final String revision,
@JsonProperty("date_expires")
final String dateExpires,
@JsonProperty("date_created")
final String dateCreated,
@JsonProperty("date_updated")
final String dateUpdated,
@JsonProperty("created_by")
final String createdBy) {
this.sid = sid;
this.uniqueName = uniqueName;
this.accountSid = accountSid;
this.serviceSid = serviceSid;
this.url = url;
this.links = links;
this.revision = revision;
this.dateExpires = DateConverter.iso8601DateTimeFromString(dateExpires);
this.dateCreated = DateConverter.iso8601DateTimeFromString(dateCreated);
this.dateUpdated = DateConverter.iso8601DateTimeFromString(dateUpdated);
this.createdBy = createdBy;
}
/**
* Returns The The unique 34-character SID identifier of the List..
*
* @return The unique 34-character SID identifier of the List.
*/
public final String getSid() {
return this.sid;
}
/**
* Returns The The unique and addressable name of this List..
*
* @return The unique and addressable name of this List.
*/
public final String getUniqueName() {
return this.uniqueName;
}
/**
* Returns The The unique SID identifier of the Twilio Account..
*
* @return The unique SID identifier of the Twilio Account.
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The The unique SID identifier of the Service Instance that hosts this
* List object..
*
* @return The unique SID identifier of the Service Instance that hosts this
* List object.
*/
public final String getServiceSid() {
return this.serviceSid;
}
/**
* Returns The The absolute URL for this List..
*
* @return The absolute URL for this List.
*/
public final URI getUrl() {
return this.url;
}
/**
* Returns The A dictionary of URL links to nested resources of this List..
*
* @return A dictionary of URL links to nested resources of this List.
*/
public final Map getLinks() {
return this.links;
}
/**
* Returns The Contains the current revision of this List, represented by a
* string identifier..
*
* @return Contains the current revision of this List, represented by a string
* identifier.
*/
public final String getRevision() {
return this.revision;
}
/**
* Returns The Contains the date this List expires and gets deleted
* automatically..
*
* @return Contains the date this List expires and gets deleted automatically.
*/
public final DateTime getDateExpires() {
return this.dateExpires;
}
/**
* Returns The The date this List was created, given in UTC ISO 8601 format..
*
* @return The date this List was created, given in UTC ISO 8601 format.
*/
public final DateTime getDateCreated() {
return this.dateCreated;
}
/**
* Returns The Specifies the date this List was last updated, given in UTC ISO
* 8601 format..
*
* @return Specifies the date this List was last updated, given in UTC ISO 8601
* format.
*/
public final DateTime getDateUpdated() {
return this.dateUpdated;
}
/**
* Returns The The identity of the List creator..
*
* @return The identity of the List creator.
*/
public final String getCreatedBy() {
return this.createdBy;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SyncList other = (SyncList) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(uniqueName, other.uniqueName) &&
Objects.equals(accountSid, other.accountSid) &&
Objects.equals(serviceSid, other.serviceSid) &&
Objects.equals(url, other.url) &&
Objects.equals(links, other.links) &&
Objects.equals(revision, other.revision) &&
Objects.equals(dateExpires, other.dateExpires) &&
Objects.equals(dateCreated, other.dateCreated) &&
Objects.equals(dateUpdated, other.dateUpdated) &&
Objects.equals(createdBy, other.createdBy);
}
@Override
public int hashCode() {
return Objects.hash(sid,
uniqueName,
accountSid,
serviceSid,
url,
links,
revision,
dateExpires,
dateCreated,
dateUpdated,
createdBy);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("sid", sid)
.add("uniqueName", uniqueName)
.add("accountSid", accountSid)
.add("serviceSid", serviceSid)
.add("url", url)
.add("links", links)
.add("revision", revision)
.add("dateExpires", dateExpires)
.add("dateCreated", dateCreated)
.add("dateUpdated", dateUpdated)
.add("createdBy", createdBy)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy