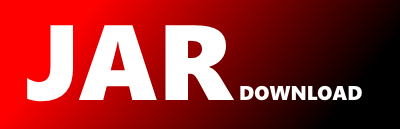
com.twilio.rest.proxy.v1.service.PhoneNumberFetcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.proxy.v1.service;
import com.twilio.base.Fetcher;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
public class PhoneNumberFetcher extends Fetcher {
private final String pathServiceSid;
private final String pathSid;
/**
* Construct a new PhoneNumberFetcher.
*
* @param pathServiceSid The SID of the parent Service resource of the
* PhoneNumber resource to fetch
* @param pathSid The unique string that identifies the resource
*/
public PhoneNumberFetcher(final String pathServiceSid,
final String pathSid) {
this.pathServiceSid = pathServiceSid;
this.pathSid = pathSid;
}
/**
* Make the request to the Twilio API to perform the fetch.
*
* @param client TwilioRestClient with which to make the request
* @return Fetched PhoneNumber
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public PhoneNumber fetch(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
Domains.PROXY.toString(),
"/v1/Services/" + this.pathServiceSid + "/PhoneNumbers/" + this.pathSid + "",
client.getRegion()
);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("PhoneNumber fetch failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return PhoneNumber.fromJson(response.getStream(), client.getObjectMapper());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy