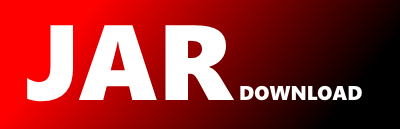
com.twilio.rest.ipmessaging.v1.ServiceUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.ipmessaging.v1;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
import java.util.List;
public class ServiceUpdater extends Updater {
private final String pathSid;
private String friendlyName;
private String defaultServiceRoleSid;
private String defaultChannelRoleSid;
private String defaultChannelCreatorRoleSid;
private Boolean readStatusEnabled;
private Boolean reachabilityEnabled;
private Integer typingIndicatorTimeout;
private Integer consumptionReportInterval;
private Boolean notificationsNewMessageEnabled;
private String notificationsNewMessageTemplate;
private Boolean notificationsAddedToChannelEnabled;
private String notificationsAddedToChannelTemplate;
private Boolean notificationsRemovedFromChannelEnabled;
private String notificationsRemovedFromChannelTemplate;
private Boolean notificationsInvitedToChannelEnabled;
private String notificationsInvitedToChannelTemplate;
private URI preWebhookUrl;
private URI postWebhookUrl;
private HttpMethod webhookMethod;
private List webhookFilters;
private URI webhooksOnMessageSendUrl;
private HttpMethod webhooksOnMessageSendMethod;
private URI webhooksOnMessageUpdateUrl;
private HttpMethod webhooksOnMessageUpdateMethod;
private URI webhooksOnMessageRemoveUrl;
private HttpMethod webhooksOnMessageRemoveMethod;
private URI webhooksOnChannelAddUrl;
private HttpMethod webhooksOnChannelAddMethod;
private URI webhooksOnChannelDestroyUrl;
private HttpMethod webhooksOnChannelDestroyMethod;
private URI webhooksOnChannelUpdateUrl;
private HttpMethod webhooksOnChannelUpdateMethod;
private URI webhooksOnMemberAddUrl;
private HttpMethod webhooksOnMemberAddMethod;
private URI webhooksOnMemberRemoveUrl;
private HttpMethod webhooksOnMemberRemoveMethod;
private URI webhooksOnMessageSentUrl;
private HttpMethod webhooksOnMessageSentMethod;
private URI webhooksOnMessageUpdatedUrl;
private HttpMethod webhooksOnMessageUpdatedMethod;
private URI webhooksOnMessageRemovedUrl;
private HttpMethod webhooksOnMessageRemovedMethod;
private URI webhooksOnChannelAddedUrl;
private HttpMethod webhooksOnChannelAddedMethod;
private URI webhooksOnChannelDestroyedUrl;
private HttpMethod webhooksOnChannelDestroyedMethod;
private URI webhooksOnChannelUpdatedUrl;
private HttpMethod webhooksOnChannelUpdatedMethod;
private URI webhooksOnMemberAddedUrl;
private HttpMethod webhooksOnMemberAddedMethod;
private URI webhooksOnMemberRemovedUrl;
private HttpMethod webhooksOnMemberRemovedMethod;
private Integer limitsChannelMembers;
private Integer limitsUserChannels;
/**
* Construct a new ServiceUpdater.
*
* @param pathSid The unique string that identifies the resource
*/
public ServiceUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* A descriptive string that you create to describe the resource. It can be up
* to 64 characters long..
*
* @param friendlyName A string to describe the resource
* @return this
*/
public ServiceUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The service role assigned to users when they are added to the service. See
* the [Roles endpoint](https://www.twilio.com/docs/chat/api/roles) for more
* details..
*
* @param defaultServiceRoleSid The service role assigned to users when they
* are added to the service
* @return this
*/
public ServiceUpdater setDefaultServiceRoleSid(final String defaultServiceRoleSid) {
this.defaultServiceRoleSid = defaultServiceRoleSid;
return this;
}
/**
* The channel role assigned to users when they are added to a channel. See the
* [Roles endpoint](https://www.twilio.com/docs/chat/api/roles) for more
* details..
*
* @param defaultChannelRoleSid The channel role assigned to users when they
* are added to a channel
* @return this
*/
public ServiceUpdater setDefaultChannelRoleSid(final String defaultChannelRoleSid) {
this.defaultChannelRoleSid = defaultChannelRoleSid;
return this;
}
/**
* The channel role assigned to a channel creator when they join a new channel.
* See the [Roles endpoint](https://www.twilio.com/docs/chat/api/roles) for more
* details..
*
* @param defaultChannelCreatorRoleSid The channel role assigned to a channel
* creator when they join a new channel
* @return this
*/
public ServiceUpdater setDefaultChannelCreatorRoleSid(final String defaultChannelCreatorRoleSid) {
this.defaultChannelCreatorRoleSid = defaultChannelCreatorRoleSid;
return this;
}
/**
* Whether to enable the [Message Consumption
* Horizon](https://www.twilio.com/docs/chat/consumption-horizon) feature. The
* default is `true`..
*
* @param readStatusEnabled Whether to enable the Message Consumption Horizon
* feature
* @return this
*/
public ServiceUpdater setReadStatusEnabled(final Boolean readStatusEnabled) {
this.readStatusEnabled = readStatusEnabled;
return this;
}
/**
* Whether to enable the [Reachability
* Indicator](https://www.twilio.com/docs/chat/reachability-indicator) for this
* Service instance. The default is `false`..
*
* @param reachabilityEnabled Whether to enable the Reachability Indicator
* feature for this Service instance
* @return this
*/
public ServiceUpdater setReachabilityEnabled(final Boolean reachabilityEnabled) {
this.reachabilityEnabled = reachabilityEnabled;
return this;
}
/**
* How long in seconds after a `started typing` event until clients should
* assume that user is no longer typing, even if no `ended typing` message was
* received. The default is 5 seconds..
*
* @param typingIndicatorTimeout How long in seconds to wait before assuming
* the user is no longer typing
* @return this
*/
public ServiceUpdater setTypingIndicatorTimeout(final Integer typingIndicatorTimeout) {
this.typingIndicatorTimeout = typingIndicatorTimeout;
return this;
}
/**
* DEPRECATED. The interval in seconds between consumption reports submission
* batches from client endpoints..
*
* @param consumptionReportInterval DEPRECATED
* @return this
*/
public ServiceUpdater setConsumptionReportInterval(final Integer consumptionReportInterval) {
this.consumptionReportInterval = consumptionReportInterval;
return this;
}
/**
* Whether to send a notification when a new message is added to a channel. Can
* be: `true` or `false` and the default is `false`..
*
* @param notificationsNewMessageEnabled Whether to send a notification when a
* new message is added to a channel
* @return this
*/
public ServiceUpdater setNotificationsNewMessageEnabled(final Boolean notificationsNewMessageEnabled) {
this.notificationsNewMessageEnabled = notificationsNewMessageEnabled;
return this;
}
/**
* The template to use to create the notification text displayed when a new
* message is added to a channel and `notifications.new_message.enabled` is
* `true`..
*
* @param notificationsNewMessageTemplate The template to use to create the
* notification text displayed when a new
* message is added to a channel
* @return this
*/
public ServiceUpdater setNotificationsNewMessageTemplate(final String notificationsNewMessageTemplate) {
this.notificationsNewMessageTemplate = notificationsNewMessageTemplate;
return this;
}
/**
* Whether to send a notification when a member is added to a channel. Can be:
* `true` or `false` and the default is `false`..
*
* @param notificationsAddedToChannelEnabled Whether to send a notification
* when a member is added to a channel
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelEnabled(final Boolean notificationsAddedToChannelEnabled) {
this.notificationsAddedToChannelEnabled = notificationsAddedToChannelEnabled;
return this;
}
/**
* The template to use to create the notification text displayed when a member
* is added to a channel and `notifications.added_to_channel.enabled` is
* `true`..
*
* @param notificationsAddedToChannelTemplate The template to use to create the
* notification text displayed when a
* member is added to a channel
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelTemplate(final String notificationsAddedToChannelTemplate) {
this.notificationsAddedToChannelTemplate = notificationsAddedToChannelTemplate;
return this;
}
/**
* Whether to send a notification to a user when they are removed from a
* channel. Can be: `true` or `false` and the default is `false`..
*
* @param notificationsRemovedFromChannelEnabled Whether to send a notification
* to a user when they are removed
* from a channel
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelEnabled(final Boolean notificationsRemovedFromChannelEnabled) {
this.notificationsRemovedFromChannelEnabled = notificationsRemovedFromChannelEnabled;
return this;
}
/**
* The template to use to create the notification text displayed to a user when
* they are removed from a channel and
* `notifications.removed_from_channel.enabled` is `true`..
*
* @param notificationsRemovedFromChannelTemplate The template to use to create
* the notification text
* displayed to a user when they
* are removed
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelTemplate(final String notificationsRemovedFromChannelTemplate) {
this.notificationsRemovedFromChannelTemplate = notificationsRemovedFromChannelTemplate;
return this;
}
/**
* Whether to send a notification when a user is invited to a channel. Can be:
* `true` or `false` and the default is `false`..
*
* @param notificationsInvitedToChannelEnabled Whether to send a notification
* when a user is invited to a
* channel
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelEnabled(final Boolean notificationsInvitedToChannelEnabled) {
this.notificationsInvitedToChannelEnabled = notificationsInvitedToChannelEnabled;
return this;
}
/**
* The template to use to create the notification text displayed when a user is
* invited to a channel and `notifications.invited_to_channel.enabled` is
* `true`..
*
* @param notificationsInvitedToChannelTemplate The template to use to create
* the notification text displayed
* when a user is invited to a
* channel
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelTemplate(final String notificationsInvitedToChannelTemplate) {
this.notificationsInvitedToChannelTemplate = notificationsInvitedToChannelTemplate;
return this;
}
/**
* The URL for pre-event webhooks, which are called by using the
* `webhook_method`. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param preWebhookUrl The webhook URL for pre-event webhooks
* @return this
*/
public ServiceUpdater setPreWebhookUrl(final URI preWebhookUrl) {
this.preWebhookUrl = preWebhookUrl;
return this;
}
/**
* The URL for pre-event webhooks, which are called by using the
* `webhook_method`. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param preWebhookUrl The webhook URL for pre-event webhooks
* @return this
*/
public ServiceUpdater setPreWebhookUrl(final String preWebhookUrl) {
return setPreWebhookUrl(Promoter.uriFromString(preWebhookUrl));
}
/**
* The URL for post-event webhooks, which are called by using the
* `webhook_method`. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param postWebhookUrl The URL for post-event webhooks
* @return this
*/
public ServiceUpdater setPostWebhookUrl(final URI postWebhookUrl) {
this.postWebhookUrl = postWebhookUrl;
return this;
}
/**
* The URL for post-event webhooks, which are called by using the
* `webhook_method`. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param postWebhookUrl The URL for post-event webhooks
* @return this
*/
public ServiceUpdater setPostWebhookUrl(final String postWebhookUrl) {
return setPostWebhookUrl(Promoter.uriFromString(postWebhookUrl));
}
/**
* The HTTP method to use for calls to the `pre_webhook_url` and
* `post_webhook_url` webhooks. Can be: `POST` or `GET` and the default is
* `POST`. See [Webhook Events](https://www.twilio.com/docs/chat/webhook-events)
* for more details..
*
* @param webhookMethod The HTTP method to use for both PRE and POST webhooks
* @return this
*/
public ServiceUpdater setWebhookMethod(final HttpMethod webhookMethod) {
this.webhookMethod = webhookMethod;
return this;
}
/**
* The list of WebHook events that are enabled for this Service instance. See
* [Webhook Events](https://www.twilio.com/docs/chat/webhook-events) for more
* details..
*
* @param webhookFilters The list of WebHook events that are enabled for this
* Service instance
* @return this
*/
public ServiceUpdater setWebhookFilters(final List webhookFilters) {
this.webhookFilters = webhookFilters;
return this;
}
/**
* The list of WebHook events that are enabled for this Service instance. See
* [Webhook Events](https://www.twilio.com/docs/chat/webhook-events) for more
* details..
*
* @param webhookFilters The list of WebHook events that are enabled for this
* Service instance
* @return this
*/
public ServiceUpdater setWebhookFilters(final String webhookFilters) {
return setWebhookFilters(Promoter.listOfOne(webhookFilters));
}
/**
* The URL of the webhook to call in response to the `on_message_send` event
* using the `webhooks.on_message_send.method` HTTP method..
*
* @param webhooksOnMessageSendUrl The URL of the webhook to call in response
* to the on_message_send event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendUrl(final URI webhooksOnMessageSendUrl) {
this.webhooksOnMessageSendUrl = webhooksOnMessageSendUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_send` event
* using the `webhooks.on_message_send.method` HTTP method..
*
* @param webhooksOnMessageSendUrl The URL of the webhook to call in response
* to the on_message_send event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendUrl(final String webhooksOnMessageSendUrl) {
return setWebhooksOnMessageSendUrl(Promoter.uriFromString(webhooksOnMessageSendUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_message_send.url`..
*
* @param webhooksOnMessageSendMethod The HTTP method to use when calling the
* webhooks.on_message_send.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendMethod(final HttpMethod webhooksOnMessageSendMethod) {
this.webhooksOnMessageSendMethod = webhooksOnMessageSendMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_update` event
* using the `webhooks.on_message_update.method` HTTP method..
*
* @param webhooksOnMessageUpdateUrl The URL of the webhook to call in response
* to the on_message_update event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateUrl(final URI webhooksOnMessageUpdateUrl) {
this.webhooksOnMessageUpdateUrl = webhooksOnMessageUpdateUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_update` event
* using the `webhooks.on_message_update.method` HTTP method..
*
* @param webhooksOnMessageUpdateUrl The URL of the webhook to call in response
* to the on_message_update event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateUrl(final String webhooksOnMessageUpdateUrl) {
return setWebhooksOnMessageUpdateUrl(Promoter.uriFromString(webhooksOnMessageUpdateUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_message_update.url`..
*
* @param webhooksOnMessageUpdateMethod The HTTP method to use when calling the
* webhooks.on_message_update.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateMethod(final HttpMethod webhooksOnMessageUpdateMethod) {
this.webhooksOnMessageUpdateMethod = webhooksOnMessageUpdateMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_remove` event
* using the `webhooks.on_message_remove.method` HTTP method..
*
* @param webhooksOnMessageRemoveUrl The URL of the webhook to call in response
* to the on_message_remove event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveUrl(final URI webhooksOnMessageRemoveUrl) {
this.webhooksOnMessageRemoveUrl = webhooksOnMessageRemoveUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_remove` event
* using the `webhooks.on_message_remove.method` HTTP method..
*
* @param webhooksOnMessageRemoveUrl The URL of the webhook to call in response
* to the on_message_remove event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveUrl(final String webhooksOnMessageRemoveUrl) {
return setWebhooksOnMessageRemoveUrl(Promoter.uriFromString(webhooksOnMessageRemoveUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_message_remove.url`..
*
* @param webhooksOnMessageRemoveMethod The HTTP method to use when calling the
* webhooks.on_message_remove.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveMethod(final HttpMethod webhooksOnMessageRemoveMethod) {
this.webhooksOnMessageRemoveMethod = webhooksOnMessageRemoveMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_add` event
* using the `webhooks.on_channel_add.method` HTTP method..
*
* @param webhooksOnChannelAddUrl The URL of the webhook to call in response to
* the on_channel_add event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddUrl(final URI webhooksOnChannelAddUrl) {
this.webhooksOnChannelAddUrl = webhooksOnChannelAddUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_add` event
* using the `webhooks.on_channel_add.method` HTTP method..
*
* @param webhooksOnChannelAddUrl The URL of the webhook to call in response to
* the on_channel_add event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddUrl(final String webhooksOnChannelAddUrl) {
return setWebhooksOnChannelAddUrl(Promoter.uriFromString(webhooksOnChannelAddUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_channel_add.url`..
*
* @param webhooksOnChannelAddMethod The HTTP method to use when calling the
* webhooks.on_channel_add.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddMethod(final HttpMethod webhooksOnChannelAddMethod) {
this.webhooksOnChannelAddMethod = webhooksOnChannelAddMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_destroy` event
* using the `webhooks.on_channel_destroy.method` HTTP method..
*
* @param webhooksOnChannelDestroyUrl The URL of the webhook to call in
* response to the on_channel_destroy event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyUrl(final URI webhooksOnChannelDestroyUrl) {
this.webhooksOnChannelDestroyUrl = webhooksOnChannelDestroyUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_destroy` event
* using the `webhooks.on_channel_destroy.method` HTTP method..
*
* @param webhooksOnChannelDestroyUrl The URL of the webhook to call in
* response to the on_channel_destroy event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyUrl(final String webhooksOnChannelDestroyUrl) {
return setWebhooksOnChannelDestroyUrl(Promoter.uriFromString(webhooksOnChannelDestroyUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_channel_destroy.url`..
*
* @param webhooksOnChannelDestroyMethod The HTTP method to use when calling
* the webhooks.on_channel_destroy.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyMethod(final HttpMethod webhooksOnChannelDestroyMethod) {
this.webhooksOnChannelDestroyMethod = webhooksOnChannelDestroyMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_update` event
* using the `webhooks.on_channel_update.method` HTTP method..
*
* @param webhooksOnChannelUpdateUrl The URL of the webhook to call in response
* to the on_channel_update event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateUrl(final URI webhooksOnChannelUpdateUrl) {
this.webhooksOnChannelUpdateUrl = webhooksOnChannelUpdateUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_update` event
* using the `webhooks.on_channel_update.method` HTTP method..
*
* @param webhooksOnChannelUpdateUrl The URL of the webhook to call in response
* to the on_channel_update event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateUrl(final String webhooksOnChannelUpdateUrl) {
return setWebhooksOnChannelUpdateUrl(Promoter.uriFromString(webhooksOnChannelUpdateUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_channel_update.url`..
*
* @param webhooksOnChannelUpdateMethod The HTTP method to use when calling the
* webhooks.on_channel_update.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateMethod(final HttpMethod webhooksOnChannelUpdateMethod) {
this.webhooksOnChannelUpdateMethod = webhooksOnChannelUpdateMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_member_add` event using
* the `webhooks.on_member_add.method` HTTP method..
*
* @param webhooksOnMemberAddUrl The URL of the webhook to call in response to
* the on_member_add event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddUrl(final URI webhooksOnMemberAddUrl) {
this.webhooksOnMemberAddUrl = webhooksOnMemberAddUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_member_add` event using
* the `webhooks.on_member_add.method` HTTP method..
*
* @param webhooksOnMemberAddUrl The URL of the webhook to call in response to
* the on_member_add event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddUrl(final String webhooksOnMemberAddUrl) {
return setWebhooksOnMemberAddUrl(Promoter.uriFromString(webhooksOnMemberAddUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_member_add.url`..
*
* @param webhooksOnMemberAddMethod The HTTP method to use when calling the
* webhooks.on_member_add.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddMethod(final HttpMethod webhooksOnMemberAddMethod) {
this.webhooksOnMemberAddMethod = webhooksOnMemberAddMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_member_remove` event
* using the `webhooks.on_member_remove.method` HTTP method..
*
* @param webhooksOnMemberRemoveUrl The URL of the webhook to call in response
* to the on_member_remove event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveUrl(final URI webhooksOnMemberRemoveUrl) {
this.webhooksOnMemberRemoveUrl = webhooksOnMemberRemoveUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_member_remove` event
* using the `webhooks.on_member_remove.method` HTTP method..
*
* @param webhooksOnMemberRemoveUrl The URL of the webhook to call in response
* to the on_member_remove event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveUrl(final String webhooksOnMemberRemoveUrl) {
return setWebhooksOnMemberRemoveUrl(Promoter.uriFromString(webhooksOnMemberRemoveUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_member_remove.url`..
*
* @param webhooksOnMemberRemoveMethod The HTTP method to use when calling the
* webhooks.on_member_remove.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveMethod(final HttpMethod webhooksOnMemberRemoveMethod) {
this.webhooksOnMemberRemoveMethod = webhooksOnMemberRemoveMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_sent` event
* using the `webhooks.on_message_sent.method` HTTP method..
*
* @param webhooksOnMessageSentUrl The URL of the webhook to call in response
* to the on_message_sent event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentUrl(final URI webhooksOnMessageSentUrl) {
this.webhooksOnMessageSentUrl = webhooksOnMessageSentUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_sent` event
* using the `webhooks.on_message_sent.method` HTTP method..
*
* @param webhooksOnMessageSentUrl The URL of the webhook to call in response
* to the on_message_sent event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentUrl(final String webhooksOnMessageSentUrl) {
return setWebhooksOnMessageSentUrl(Promoter.uriFromString(webhooksOnMessageSentUrl));
}
/**
* The URL of the webhook to call in response to the `on_message_sent` event`..
*
* @param webhooksOnMessageSentMethod The URL of the webhook to call in
* response to the on_message_sent event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentMethod(final HttpMethod webhooksOnMessageSentMethod) {
this.webhooksOnMessageSentMethod = webhooksOnMessageSentMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_updated` event
* using the `webhooks.on_message_updated.method` HTTP method..
*
* @param webhooksOnMessageUpdatedUrl The URL of the webhook to call in
* response to the on_message_updated event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedUrl(final URI webhooksOnMessageUpdatedUrl) {
this.webhooksOnMessageUpdatedUrl = webhooksOnMessageUpdatedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_updated` event
* using the `webhooks.on_message_updated.method` HTTP method..
*
* @param webhooksOnMessageUpdatedUrl The URL of the webhook to call in
* response to the on_message_updated event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedUrl(final String webhooksOnMessageUpdatedUrl) {
return setWebhooksOnMessageUpdatedUrl(Promoter.uriFromString(webhooksOnMessageUpdatedUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_message_updated.url`..
*
* @param webhooksOnMessageUpdatedMethod The HTTP method to use when calling
* the webhooks.on_message_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedMethod(final HttpMethod webhooksOnMessageUpdatedMethod) {
this.webhooksOnMessageUpdatedMethod = webhooksOnMessageUpdatedMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_removed` event
* using the `webhooks.on_message_removed.method` HTTP method..
*
* @param webhooksOnMessageRemovedUrl The URL of the webhook to call in
* response to the on_message_removed event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedUrl(final URI webhooksOnMessageRemovedUrl) {
this.webhooksOnMessageRemovedUrl = webhooksOnMessageRemovedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_message_removed` event
* using the `webhooks.on_message_removed.method` HTTP method..
*
* @param webhooksOnMessageRemovedUrl The URL of the webhook to call in
* response to the on_message_removed event
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedUrl(final String webhooksOnMessageRemovedUrl) {
return setWebhooksOnMessageRemovedUrl(Promoter.uriFromString(webhooksOnMessageRemovedUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_message_removed.url`..
*
* @param webhooksOnMessageRemovedMethod The HTTP method to use when calling
* the webhooks.on_message_removed.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedMethod(final HttpMethod webhooksOnMessageRemovedMethod) {
this.webhooksOnMessageRemovedMethod = webhooksOnMessageRemovedMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_added` event
* using the `webhooks.on_channel_added.method` HTTP method..
*
* @param webhooksOnChannelAddedUrl The URL of the webhook to call in response
* to the on_channel_added event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedUrl(final URI webhooksOnChannelAddedUrl) {
this.webhooksOnChannelAddedUrl = webhooksOnChannelAddedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_added` event
* using the `webhooks.on_channel_added.method` HTTP method..
*
* @param webhooksOnChannelAddedUrl The URL of the webhook to call in response
* to the on_channel_added event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedUrl(final String webhooksOnChannelAddedUrl) {
return setWebhooksOnChannelAddedUrl(Promoter.uriFromString(webhooksOnChannelAddedUrl));
}
/**
* The URL of the webhook to call in response to the `on_channel_added` event`..
*
* @param webhooksOnChannelAddedMethod The URL of the webhook to call in
* response to the on_channel_added event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedMethod(final HttpMethod webhooksOnChannelAddedMethod) {
this.webhooksOnChannelAddedMethod = webhooksOnChannelAddedMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_added` event
* using the `webhooks.on_channel_destroyed.method` HTTP method..
*
* @param webhooksOnChannelDestroyedUrl The URL of the webhook to call in
* response to the on_channel_added event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedUrl(final URI webhooksOnChannelDestroyedUrl) {
this.webhooksOnChannelDestroyedUrl = webhooksOnChannelDestroyedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_added` event
* using the `webhooks.on_channel_destroyed.method` HTTP method..
*
* @param webhooksOnChannelDestroyedUrl The URL of the webhook to call in
* response to the on_channel_added event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedUrl(final String webhooksOnChannelDestroyedUrl) {
return setWebhooksOnChannelDestroyedUrl(Promoter.uriFromString(webhooksOnChannelDestroyedUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_channel_destroyed.url`..
*
* @param webhooksOnChannelDestroyedMethod The HTTP method to use when calling
* the webhooks.on_channel_destroyed.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedMethod(final HttpMethod webhooksOnChannelDestroyedMethod) {
this.webhooksOnChannelDestroyedMethod = webhooksOnChannelDestroyedMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_updated` event
* using the `webhooks.on_channel_updated.method` HTTP method..
*
* @param webhooksOnChannelUpdatedUrl he URL of the webhook to call in response
* to the on_channel_updated event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedUrl(final URI webhooksOnChannelUpdatedUrl) {
this.webhooksOnChannelUpdatedUrl = webhooksOnChannelUpdatedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_updated` event
* using the `webhooks.on_channel_updated.method` HTTP method..
*
* @param webhooksOnChannelUpdatedUrl he URL of the webhook to call in response
* to the on_channel_updated event
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedUrl(final String webhooksOnChannelUpdatedUrl) {
return setWebhooksOnChannelUpdatedUrl(Promoter.uriFromString(webhooksOnChannelUpdatedUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_channel_updated.url`..
*
* @param webhooksOnChannelUpdatedMethod The HTTP method to use when calling
* the webhooks.on_channel_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedMethod(final HttpMethod webhooksOnChannelUpdatedMethod) {
this.webhooksOnChannelUpdatedMethod = webhooksOnChannelUpdatedMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_updated` event
* using the `webhooks.on_channel_updated.method` HTTP method..
*
* @param webhooksOnMemberAddedUrl The URL of the webhook to call in response
* to the on_channel_updated event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedUrl(final URI webhooksOnMemberAddedUrl) {
this.webhooksOnMemberAddedUrl = webhooksOnMemberAddedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_channel_updated` event
* using the `webhooks.on_channel_updated.method` HTTP method..
*
* @param webhooksOnMemberAddedUrl The URL of the webhook to call in response
* to the on_channel_updated event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedUrl(final String webhooksOnMemberAddedUrl) {
return setWebhooksOnMemberAddedUrl(Promoter.uriFromString(webhooksOnMemberAddedUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_channel_updated.url`..
*
* @param webhooksOnMemberAddedMethod he HTTP method to use when calling the
* webhooks.on_channel_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedMethod(final HttpMethod webhooksOnMemberAddedMethod) {
this.webhooksOnMemberAddedMethod = webhooksOnMemberAddedMethod;
return this;
}
/**
* The URL of the webhook to call in response to the `on_member_removed` event
* using the `webhooks.on_member_removed.method` HTTP method..
*
* @param webhooksOnMemberRemovedUrl The URL of the webhook to call in response
* to the on_member_removed event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedUrl(final URI webhooksOnMemberRemovedUrl) {
this.webhooksOnMemberRemovedUrl = webhooksOnMemberRemovedUrl;
return this;
}
/**
* The URL of the webhook to call in response to the `on_member_removed` event
* using the `webhooks.on_member_removed.method` HTTP method..
*
* @param webhooksOnMemberRemovedUrl The URL of the webhook to call in response
* to the on_member_removed event
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedUrl(final String webhooksOnMemberRemovedUrl) {
return setWebhooksOnMemberRemovedUrl(Promoter.uriFromString(webhooksOnMemberRemovedUrl));
}
/**
* The HTTP method to use when calling the `webhooks.on_member_removed.url`..
*
* @param webhooksOnMemberRemovedMethod The HTTP method to use when calling the
* webhooks.on_member_removed.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedMethod(final HttpMethod webhooksOnMemberRemovedMethod) {
this.webhooksOnMemberRemovedMethod = webhooksOnMemberRemovedMethod;
return this;
}
/**
* The maximum number of Members that can be added to Channels within this
* Service. Can be up to 1,000..
*
* @param limitsChannelMembers The maximum number of Members that can be added
* to Channels within this Service
* @return this
*/
public ServiceUpdater setLimitsChannelMembers(final Integer limitsChannelMembers) {
this.limitsChannelMembers = limitsChannelMembers;
return this;
}
/**
* The maximum number of Channels Users can be a Member of within this Service.
* Can be up to 1,000..
*
* @param limitsUserChannels The maximum number of Channels Users can be a
* Member of within this Service
* @return this
*/
public ServiceUpdater setLimitsUserChannels(final Integer limitsUserChannels) {
this.limitsUserChannels = limitsUserChannels;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Service
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Service update(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.IPMESSAGING.toString(),
"/v1/Services/" + this.pathSid + "",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Service update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Service.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (defaultServiceRoleSid != null) {
request.addPostParam("DefaultServiceRoleSid", defaultServiceRoleSid);
}
if (defaultChannelRoleSid != null) {
request.addPostParam("DefaultChannelRoleSid", defaultChannelRoleSid);
}
if (defaultChannelCreatorRoleSid != null) {
request.addPostParam("DefaultChannelCreatorRoleSid", defaultChannelCreatorRoleSid);
}
if (readStatusEnabled != null) {
request.addPostParam("ReadStatusEnabled", readStatusEnabled.toString());
}
if (reachabilityEnabled != null) {
request.addPostParam("ReachabilityEnabled", reachabilityEnabled.toString());
}
if (typingIndicatorTimeout != null) {
request.addPostParam("TypingIndicatorTimeout", typingIndicatorTimeout.toString());
}
if (consumptionReportInterval != null) {
request.addPostParam("ConsumptionReportInterval", consumptionReportInterval.toString());
}
if (notificationsNewMessageEnabled != null) {
request.addPostParam("Notifications.NewMessage.Enabled", notificationsNewMessageEnabled.toString());
}
if (notificationsNewMessageTemplate != null) {
request.addPostParam("Notifications.NewMessage.Template", notificationsNewMessageTemplate);
}
if (notificationsAddedToChannelEnabled != null) {
request.addPostParam("Notifications.AddedToChannel.Enabled", notificationsAddedToChannelEnabled.toString());
}
if (notificationsAddedToChannelTemplate != null) {
request.addPostParam("Notifications.AddedToChannel.Template", notificationsAddedToChannelTemplate);
}
if (notificationsRemovedFromChannelEnabled != null) {
request.addPostParam("Notifications.RemovedFromChannel.Enabled", notificationsRemovedFromChannelEnabled.toString());
}
if (notificationsRemovedFromChannelTemplate != null) {
request.addPostParam("Notifications.RemovedFromChannel.Template", notificationsRemovedFromChannelTemplate);
}
if (notificationsInvitedToChannelEnabled != null) {
request.addPostParam("Notifications.InvitedToChannel.Enabled", notificationsInvitedToChannelEnabled.toString());
}
if (notificationsInvitedToChannelTemplate != null) {
request.addPostParam("Notifications.InvitedToChannel.Template", notificationsInvitedToChannelTemplate);
}
if (preWebhookUrl != null) {
request.addPostParam("PreWebhookUrl", preWebhookUrl.toString());
}
if (postWebhookUrl != null) {
request.addPostParam("PostWebhookUrl", postWebhookUrl.toString());
}
if (webhookMethod != null) {
request.addPostParam("WebhookMethod", webhookMethod.toString());
}
if (webhookFilters != null) {
for (String prop : webhookFilters) {
request.addPostParam("WebhookFilters", prop);
}
}
if (webhooksOnMessageSendUrl != null) {
request.addPostParam("Webhooks.OnMessageSend.Url", webhooksOnMessageSendUrl.toString());
}
if (webhooksOnMessageSendMethod != null) {
request.addPostParam("Webhooks.OnMessageSend.Method", webhooksOnMessageSendMethod.toString());
}
if (webhooksOnMessageUpdateUrl != null) {
request.addPostParam("Webhooks.OnMessageUpdate.Url", webhooksOnMessageUpdateUrl.toString());
}
if (webhooksOnMessageUpdateMethod != null) {
request.addPostParam("Webhooks.OnMessageUpdate.Method", webhooksOnMessageUpdateMethod.toString());
}
if (webhooksOnMessageRemoveUrl != null) {
request.addPostParam("Webhooks.OnMessageRemove.Url", webhooksOnMessageRemoveUrl.toString());
}
if (webhooksOnMessageRemoveMethod != null) {
request.addPostParam("Webhooks.OnMessageRemove.Method", webhooksOnMessageRemoveMethod.toString());
}
if (webhooksOnChannelAddUrl != null) {
request.addPostParam("Webhooks.OnChannelAdd.Url", webhooksOnChannelAddUrl.toString());
}
if (webhooksOnChannelAddMethod != null) {
request.addPostParam("Webhooks.OnChannelAdd.Method", webhooksOnChannelAddMethod.toString());
}
if (webhooksOnChannelDestroyUrl != null) {
request.addPostParam("Webhooks.OnChannelDestroy.Url", webhooksOnChannelDestroyUrl.toString());
}
if (webhooksOnChannelDestroyMethod != null) {
request.addPostParam("Webhooks.OnChannelDestroy.Method", webhooksOnChannelDestroyMethod.toString());
}
if (webhooksOnChannelUpdateUrl != null) {
request.addPostParam("Webhooks.OnChannelUpdate.Url", webhooksOnChannelUpdateUrl.toString());
}
if (webhooksOnChannelUpdateMethod != null) {
request.addPostParam("Webhooks.OnChannelUpdate.Method", webhooksOnChannelUpdateMethod.toString());
}
if (webhooksOnMemberAddUrl != null) {
request.addPostParam("Webhooks.OnMemberAdd.Url", webhooksOnMemberAddUrl.toString());
}
if (webhooksOnMemberAddMethod != null) {
request.addPostParam("Webhooks.OnMemberAdd.Method", webhooksOnMemberAddMethod.toString());
}
if (webhooksOnMemberRemoveUrl != null) {
request.addPostParam("Webhooks.OnMemberRemove.Url", webhooksOnMemberRemoveUrl.toString());
}
if (webhooksOnMemberRemoveMethod != null) {
request.addPostParam("Webhooks.OnMemberRemove.Method", webhooksOnMemberRemoveMethod.toString());
}
if (webhooksOnMessageSentUrl != null) {
request.addPostParam("Webhooks.OnMessageSent.Url", webhooksOnMessageSentUrl.toString());
}
if (webhooksOnMessageSentMethod != null) {
request.addPostParam("Webhooks.OnMessageSent.Method", webhooksOnMessageSentMethod.toString());
}
if (webhooksOnMessageUpdatedUrl != null) {
request.addPostParam("Webhooks.OnMessageUpdated.Url", webhooksOnMessageUpdatedUrl.toString());
}
if (webhooksOnMessageUpdatedMethod != null) {
request.addPostParam("Webhooks.OnMessageUpdated.Method", webhooksOnMessageUpdatedMethod.toString());
}
if (webhooksOnMessageRemovedUrl != null) {
request.addPostParam("Webhooks.OnMessageRemoved.Url", webhooksOnMessageRemovedUrl.toString());
}
if (webhooksOnMessageRemovedMethod != null) {
request.addPostParam("Webhooks.OnMessageRemoved.Method", webhooksOnMessageRemovedMethod.toString());
}
if (webhooksOnChannelAddedUrl != null) {
request.addPostParam("Webhooks.OnChannelAdded.Url", webhooksOnChannelAddedUrl.toString());
}
if (webhooksOnChannelAddedMethod != null) {
request.addPostParam("Webhooks.OnChannelAdded.Method", webhooksOnChannelAddedMethod.toString());
}
if (webhooksOnChannelDestroyedUrl != null) {
request.addPostParam("Webhooks.OnChannelDestroyed.Url", webhooksOnChannelDestroyedUrl.toString());
}
if (webhooksOnChannelDestroyedMethod != null) {
request.addPostParam("Webhooks.OnChannelDestroyed.Method", webhooksOnChannelDestroyedMethod.toString());
}
if (webhooksOnChannelUpdatedUrl != null) {
request.addPostParam("Webhooks.OnChannelUpdated.Url", webhooksOnChannelUpdatedUrl.toString());
}
if (webhooksOnChannelUpdatedMethod != null) {
request.addPostParam("Webhooks.OnChannelUpdated.Method", webhooksOnChannelUpdatedMethod.toString());
}
if (webhooksOnMemberAddedUrl != null) {
request.addPostParam("Webhooks.OnMemberAdded.Url", webhooksOnMemberAddedUrl.toString());
}
if (webhooksOnMemberAddedMethod != null) {
request.addPostParam("Webhooks.OnMemberAdded.Method", webhooksOnMemberAddedMethod.toString());
}
if (webhooksOnMemberRemovedUrl != null) {
request.addPostParam("Webhooks.OnMemberRemoved.Url", webhooksOnMemberRemovedUrl.toString());
}
if (webhooksOnMemberRemovedMethod != null) {
request.addPostParam("Webhooks.OnMemberRemoved.Method", webhooksOnMemberRemovedMethod.toString());
}
if (limitsChannelMembers != null) {
request.addPostParam("Limits.ChannelMembers", limitsChannelMembers.toString());
}
if (limitsUserChannels != null) {
request.addPostParam("Limits.UserChannels", limitsUserChannels.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy