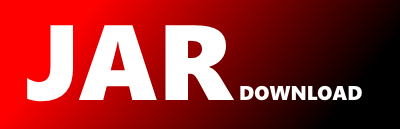
com.twilio.rest.preview.deployedDevices.fleet.Device Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.preview.deployedDevices.fleet;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.DateConverter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.Map;
import java.util.Objects;
/**
* PLEASE NOTE that this class contains preview products that are subject to
* change. Use them with caution. If you currently do not have developer preview
* access, please contact [email protected].
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class Device extends Resource {
private static final long serialVersionUID = 144542670393653L;
/**
* Create a DeviceFetcher to execute fetch.
*
* @param pathFleetSid The fleet_sid
* @param pathSid A string that uniquely identifies the Device.
* @return DeviceFetcher capable of executing the fetch
*/
public static DeviceFetcher fetcher(final String pathFleetSid,
final String pathSid) {
return new DeviceFetcher(pathFleetSid, pathSid);
}
/**
* Create a DeviceDeleter to execute delete.
*
* @param pathFleetSid The fleet_sid
* @param pathSid A string that uniquely identifies the Device.
* @return DeviceDeleter capable of executing the delete
*/
public static DeviceDeleter deleter(final String pathFleetSid,
final String pathSid) {
return new DeviceDeleter(pathFleetSid, pathSid);
}
/**
* Create a DeviceCreator to execute create.
*
* @param pathFleetSid The fleet_sid
* @return DeviceCreator capable of executing the create
*/
public static DeviceCreator creator(final String pathFleetSid) {
return new DeviceCreator(pathFleetSid);
}
/**
* Create a DeviceReader to execute read.
*
* @param pathFleetSid The fleet_sid
* @return DeviceReader capable of executing the read
*/
public static DeviceReader reader(final String pathFleetSid) {
return new DeviceReader(pathFleetSid);
}
/**
* Create a DeviceUpdater to execute update.
*
* @param pathFleetSid The fleet_sid
* @param pathSid A string that uniquely identifies the Device.
* @return DeviceUpdater capable of executing the update
*/
public static DeviceUpdater updater(final String pathFleetSid,
final String pathSid) {
return new DeviceUpdater(pathFleetSid, pathSid);
}
/**
* Converts a JSON String into a Device object using the provided ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return Device object represented by the provided JSON
*/
public static Device fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Device.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a Device object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return Device object represented by the provided JSON
*/
public static Device fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Device.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final URI url;
private final String uniqueName;
private final String friendlyName;
private final String fleetSid;
private final Boolean enabled;
private final String accountSid;
private final String identity;
private final String deploymentSid;
private final DateTime dateCreated;
private final DateTime dateUpdated;
private final DateTime dateAuthenticated;
@JsonCreator
private Device(@JsonProperty("sid")
final String sid,
@JsonProperty("url")
final URI url,
@JsonProperty("unique_name")
final String uniqueName,
@JsonProperty("friendly_name")
final String friendlyName,
@JsonProperty("fleet_sid")
final String fleetSid,
@JsonProperty("enabled")
final Boolean enabled,
@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("identity")
final String identity,
@JsonProperty("deployment_sid")
final String deploymentSid,
@JsonProperty("date_created")
final String dateCreated,
@JsonProperty("date_updated")
final String dateUpdated,
@JsonProperty("date_authenticated")
final String dateAuthenticated) {
this.sid = sid;
this.url = url;
this.uniqueName = uniqueName;
this.friendlyName = friendlyName;
this.fleetSid = fleetSid;
this.enabled = enabled;
this.accountSid = accountSid;
this.identity = identity;
this.deploymentSid = deploymentSid;
this.dateCreated = DateConverter.iso8601DateTimeFromString(dateCreated);
this.dateUpdated = DateConverter.iso8601DateTimeFromString(dateUpdated);
this.dateAuthenticated = DateConverter.iso8601DateTimeFromString(dateAuthenticated);
}
/**
* Returns A string that uniquely identifies this Device..
*
* @return A string that uniquely identifies this Device.
*/
public final String getSid() {
return this.sid;
}
/**
* Returns URL of this Device..
*
* @return URL of this Device.
*/
public final URI getUrl() {
return this.url;
}
/**
* Returns A unique, addressable name of this Device..
*
* @return A unique, addressable name of this Device.
*/
public final String getUniqueName() {
return this.uniqueName;
}
/**
* Returns A human readable description for this Device.
*
* @return A human readable description for this Device
*/
public final String getFriendlyName() {
return this.friendlyName;
}
/**
* Returns The unique identifier of the Fleet..
*
* @return The unique identifier of the Fleet.
*/
public final String getFleetSid() {
return this.fleetSid;
}
/**
* Returns Device enabled flag..
*
* @return Device enabled flag.
*/
public final Boolean getEnabled() {
return this.enabled;
}
/**
* Returns The unique SID that identifies this Account..
*
* @return The unique SID that identifies this Account.
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns An identifier of the Device user..
*
* @return An identifier of the Device user.
*/
public final String getIdentity() {
return this.identity;
}
/**
* Returns The unique SID of the Deployment group..
*
* @return The unique SID of the Deployment group.
*/
public final String getDeploymentSid() {
return this.deploymentSid;
}
/**
* Returns The date this Device was created..
*
* @return The date this Device was created.
*/
public final DateTime getDateCreated() {
return this.dateCreated;
}
/**
* Returns The date this Device was updated..
*
* @return The date this Device was updated.
*/
public final DateTime getDateUpdated() {
return this.dateUpdated;
}
/**
* Returns The date this Device was authenticated..
*
* @return The date this Device was authenticated.
*/
public final DateTime getDateAuthenticated() {
return this.dateAuthenticated;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Device other = (Device) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(url, other.url) &&
Objects.equals(uniqueName, other.uniqueName) &&
Objects.equals(friendlyName, other.friendlyName) &&
Objects.equals(fleetSid, other.fleetSid) &&
Objects.equals(enabled, other.enabled) &&
Objects.equals(accountSid, other.accountSid) &&
Objects.equals(identity, other.identity) &&
Objects.equals(deploymentSid, other.deploymentSid) &&
Objects.equals(dateCreated, other.dateCreated) &&
Objects.equals(dateUpdated, other.dateUpdated) &&
Objects.equals(dateAuthenticated, other.dateAuthenticated);
}
@Override
public int hashCode() {
return Objects.hash(sid,
url,
uniqueName,
friendlyName,
fleetSid,
enabled,
accountSid,
identity,
deploymentSid,
dateCreated,
dateUpdated,
dateAuthenticated);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("sid", sid)
.add("url", url)
.add("uniqueName", uniqueName)
.add("friendlyName", friendlyName)
.add("fleetSid", fleetSid)
.add("enabled", enabled)
.add("accountSid", accountSid)
.add("identity", identity)
.add("deploymentSid", deploymentSid)
.add("dateCreated", dateCreated)
.add("dateUpdated", dateUpdated)
.add("dateAuthenticated", dateAuthenticated)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy