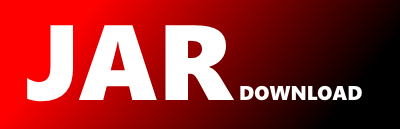
com.twilio.rest.ipmessaging.v1.CredentialCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.ipmessaging.v1;
import com.twilio.base.Creator;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
public class CredentialCreator extends Creator {
private final Credential.PushService type;
private String friendlyName;
private String certificate;
private String privateKey;
private Boolean sandbox;
private String apiKey;
private String secret;
/**
* Construct a new CredentialCreator.
*
* @param type The type
*/
public CredentialCreator(final Credential.PushService type) {
this.type = type;
}
/**
* The friendly_name.
*
* @param friendlyName The friendly_name
* @return this
*/
public CredentialCreator setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The certificate.
*
* @param certificate The certificate
* @return this
*/
public CredentialCreator setCertificate(final String certificate) {
this.certificate = certificate;
return this;
}
/**
* The private_key.
*
* @param privateKey The private_key
* @return this
*/
public CredentialCreator setPrivateKey(final String privateKey) {
this.privateKey = privateKey;
return this;
}
/**
* The sandbox.
*
* @param sandbox The sandbox
* @return this
*/
public CredentialCreator setSandbox(final Boolean sandbox) {
this.sandbox = sandbox;
return this;
}
/**
* The api_key.
*
* @param apiKey The api_key
* @return this
*/
public CredentialCreator setApiKey(final String apiKey) {
this.apiKey = apiKey;
return this;
}
/**
* The secret.
*
* @param secret The secret
* @return this
*/
public CredentialCreator setSecret(final String secret) {
this.secret = secret;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created Credential
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Credential create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.IPMESSAGING.toString(),
"/v1/Credentials"
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Credential creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return Credential.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (type != null) {
request.addPostParam("Type", type.toString());
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (certificate != null) {
request.addPostParam("Certificate", certificate);
}
if (privateKey != null) {
request.addPostParam("PrivateKey", privateKey);
}
if (sandbox != null) {
request.addPostParam("Sandbox", sandbox.toString());
}
if (apiKey != null) {
request.addPostParam("ApiKey", apiKey);
}
if (secret != null) {
request.addPostParam("Secret", secret);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy