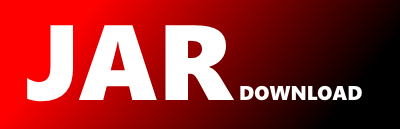
com.twilio.rest.trusthub.v1.customerprofiles.CustomerProfilesEvaluationsReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.trusthub.v1.customerprofiles;
import com.twilio.base.Page;
import com.twilio.base.Reader;
import com.twilio.base.ResourceSet;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
public class CustomerProfilesEvaluationsReader extends Reader {
private final String pathCustomerProfileSid;
/**
* Construct a new CustomerProfilesEvaluationsReader.
*
* @param pathCustomerProfileSid The unique string that identifies the resource.
*/
public CustomerProfilesEvaluationsReader(final String pathCustomerProfileSid) {
this.pathCustomerProfileSid = pathCustomerProfileSid;
}
/**
* Make the request to the Twilio API to perform the read.
*
* @param client TwilioRestClient with which to make the request
* @return CustomerProfilesEvaluations ResourceSet
*/
@Override
public ResourceSet read(final TwilioRestClient client) {
return new ResourceSet<>(this, client, firstPage(client));
}
/**
* Make the request to the Twilio API to perform the read.
*
* @param client TwilioRestClient with which to make the request
* @return CustomerProfilesEvaluations ResourceSet
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Page firstPage(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
Domains.TRUSTHUB.toString(),
"/v1/CustomerProfiles/" + this.pathCustomerProfileSid + "/Evaluations"
);
addQueryParams(request);
return pageForRequest(client, request);
}
/**
* Retrieve the target page from the Twilio API.
*
* @param targetUrl API-generated URL for the requested results page
* @param client TwilioRestClient with which to make the request
* @return CustomerProfilesEvaluations ResourceSet
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Page getPage(final String targetUrl, final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
targetUrl
);
return pageForRequest(client, request);
}
/**
* Retrieve the next page from the Twilio API.
*
* @param page current page
* @param client TwilioRestClient with which to make the request
* @return Next Page
*/
@Override
public Page nextPage(final Page page,
final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
page.getNextPageUrl(Domains.TRUSTHUB.toString())
);
return pageForRequest(client, request);
}
/**
* Retrieve the previous page from the Twilio API.
*
* @param page current page
* @param client TwilioRestClient with which to make the request
* @return Previous Page
*/
@Override
public Page previousPage(final Page page,
final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
page.getPreviousPageUrl(Domains.TRUSTHUB.toString())
);
return pageForRequest(client, request);
}
/**
* Generate a Page of CustomerProfilesEvaluations Resources for a given request.
*
* @param client TwilioRestClient with which to make the request
* @param request Request to generate a page for
* @return Page for the Request
*/
private Page pageForRequest(final TwilioRestClient client, final Request request) {
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("CustomerProfilesEvaluations read failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return Page.fromJson(
"results",
response.getContent(),
CustomerProfilesEvaluations.class,
client.getObjectMapper()
);
}
/**
* Add the requested query string arguments to the Request.
*
* @param request Request to add query string arguments to
*/
private void addQueryParams(final Request request) {
if (getPageSize() != null) {
request.addQueryParam("PageSize", Integer.toString(getPageSize()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy