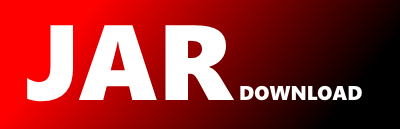
com.twilio.rest.studio.v2.flow.FlowTestUser Maven / Gradle / Ivy
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.studio.v2.flow;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.twilio.base.Resource;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import lombok.ToString;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@JsonIgnoreProperties(ignoreUnknown = true)
@ToString
public class FlowTestUser extends Resource {
private static final long serialVersionUID = 179832058834973L;
/**
* Create a FlowTestUserFetcher to execute fetch.
*
* @param pathSid Unique identifier of the flow.
* @return FlowTestUserFetcher capable of executing the fetch
*/
public static FlowTestUserFetcher fetcher(final String pathSid) {
return new FlowTestUserFetcher(pathSid);
}
/**
* Create a FlowTestUserUpdater to execute update.
*
* @param pathSid Unique identifier of the flow.
* @param testUsers List of test user identities that can test draft versions
* of the flow.
* @return FlowTestUserUpdater capable of executing the update
*/
public static FlowTestUserUpdater updater(final String pathSid,
final List testUsers) {
return new FlowTestUserUpdater(pathSid, testUsers);
}
/**
* Converts a JSON String into a FlowTestUser object using the provided
* ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return FlowTestUser object represented by the provided JSON
*/
public static FlowTestUser fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, FlowTestUser.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a FlowTestUser object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return FlowTestUser object represented by the provided JSON
*/
public static FlowTestUser fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, FlowTestUser.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final List testUsers;
private final URI url;
@JsonCreator
private FlowTestUser(@JsonProperty("sid")
final String sid,
@JsonProperty("test_users")
final List testUsers,
@JsonProperty("url")
final URI url) {
this.sid = sid;
this.testUsers = testUsers;
this.url = url;
}
/**
* Returns Unique identifier of the flow..
*
* @return Unique identifier of the flow.
*/
public final String getSid() {
return this.sid;
}
/**
* Returns List of test user identities that can test draft versions of the
* flow..
*
* @return List of test user identities that can test draft versions of the
* flow.
*/
public final List getTestUsers() {
return this.testUsers;
}
/**
* Returns The URL of this resource..
*
* @return The URL of this resource.
*/
public final URI getUrl() {
return this.url;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FlowTestUser other = (FlowTestUser) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(testUsers, other.testUsers) &&
Objects.equals(url, other.url);
}
@Override
public int hashCode() {
return Objects.hash(sid,
testUsers,
url);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy