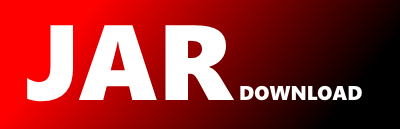
com.twilio.rest.api.v2010.account.call.Siprec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account.call;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.twilio.base.Resource;
import com.twilio.converter.DateConverter;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import lombok.ToString;
import java.io.IOException;
import java.io.InputStream;
import java.time.ZonedDateTime;
import java.util.Map;
import java.util.Objects;
@JsonIgnoreProperties(ignoreUnknown = true)
@ToString
public class Siprec extends Resource {
private static final long serialVersionUID = 268819701788968L;
public enum Track {
INBOUND_TRACK("inbound_track"),
OUTBOUND_TRACK("outbound_track"),
BOTH_TRACKS("both_tracks");
private final String value;
private Track(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a Track from a string.
* @param value string value
* @return generated Track
*/
@JsonCreator
public static Track forValue(final String value) {
return Promoter.enumFromString(value, Track.values());
}
}
public enum Status {
IN_PROGRESS("in-progress"),
STOPPED("stopped");
private final String value;
private Status(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a Status from a string.
* @param value string value
* @return generated Status
*/
@JsonCreator
public static Status forValue(final String value) {
return Promoter.enumFromString(value, Status.values());
}
}
public enum UpdateStatus {
STOPPED("stopped");
private final String value;
private UpdateStatus(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a UpdateStatus from a string.
* @param value string value
* @return generated UpdateStatus
*/
@JsonCreator
public static UpdateStatus forValue(final String value) {
return Promoter.enumFromString(value, UpdateStatus.values());
}
}
/**
* Create a SiprecCreator to execute create.
*
* @param pathAccountSid The SID of the Account that created this resource
* @param pathCallSid The SID of the Call the resource is associated with
* @return SiprecCreator capable of executing the create
*/
public static SiprecCreator creator(final String pathAccountSid,
final String pathCallSid) {
return new SiprecCreator(pathAccountSid, pathCallSid);
}
/**
* Create a SiprecCreator to execute create.
*
* @param pathCallSid The SID of the Call the resource is associated with
* @return SiprecCreator capable of executing the create
*/
public static SiprecCreator creator(final String pathCallSid) {
return new SiprecCreator(pathCallSid);
}
/**
* Create a SiprecUpdater to execute update.
*
* @param pathAccountSid The SID of the Account that created this resource
* @param pathCallSid The SID of the Call the resource is associated with
* @param pathSid The SID of the Siprec resource, or the `name`
* @param status The status. Must have the value `stopped`
* @return SiprecUpdater capable of executing the update
*/
public static SiprecUpdater updater(final String pathAccountSid,
final String pathCallSid,
final String pathSid,
final Siprec.UpdateStatus status) {
return new SiprecUpdater(pathAccountSid, pathCallSid, pathSid, status);
}
/**
* Create a SiprecUpdater to execute update.
*
* @param pathCallSid The SID of the Call the resource is associated with
* @param pathSid The SID of the Siprec resource, or the `name`
* @param status The status. Must have the value `stopped`
* @return SiprecUpdater capable of executing the update
*/
public static SiprecUpdater updater(final String pathCallSid,
final String pathSid,
final Siprec.UpdateStatus status) {
return new SiprecUpdater(pathCallSid, pathSid, status);
}
/**
* Converts a JSON String into a Siprec object using the provided ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return Siprec object represented by the provided JSON
*/
public static Siprec fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Siprec.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a Siprec object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return Siprec object represented by the provided JSON
*/
public static Siprec fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Siprec.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final String accountSid;
private final String callSid;
private final String name;
private final Siprec.Status status;
private final ZonedDateTime dateUpdated;
@JsonCreator
private Siprec(@JsonProperty("sid")
final String sid,
@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("call_sid")
final String callSid,
@JsonProperty("name")
final String name,
@JsonProperty("status")
final Siprec.Status status,
@JsonProperty("date_updated")
final String dateUpdated) {
this.sid = sid;
this.accountSid = accountSid;
this.callSid = callSid;
this.name = name;
this.status = status;
this.dateUpdated = DateConverter.rfc2822DateTimeFromString(dateUpdated);
}
/**
* Returns The SID of the Siprec resource..
*
* @return The SID of the Siprec resource.
*/
public final String getSid() {
return this.sid;
}
/**
* Returns The SID of the Account that created this resource.
*
* @return The SID of the Account that created this resource
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The SID of the Call the resource is associated with.
*
* @return The SID of the Call the resource is associated with
*/
public final String getCallSid() {
return this.callSid;
}
/**
* Returns The name of this resource.
*
* @return The name of this resource
*/
public final String getName() {
return this.name;
}
/**
* Returns The status - one of `stopped`, `in-progress`.
*
* @return The status - one of `stopped`, `in-progress`
*/
public final Siprec.Status getStatus() {
return this.status;
}
/**
* Returns The RFC 2822 date and time in GMT that this resource was last
* updated.
*
* @return The RFC 2822 date and time in GMT that this resource was last updated
*/
public final ZonedDateTime getDateUpdated() {
return this.dateUpdated;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Siprec other = (Siprec) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(accountSid, other.accountSid) &&
Objects.equals(callSid, other.callSid) &&
Objects.equals(name, other.name) &&
Objects.equals(status, other.status) &&
Objects.equals(dateUpdated, other.dateUpdated);
}
@Override
public int hashCode() {
return Objects.hash(sid,
accountSid,
callSid,
name,
status,
dateUpdated);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy