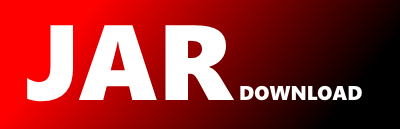
com.twilio.rest.sync.v1.service.syncmap.SyncMapItemCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.sync.v1.service.syncmap;
import com.twilio.base.Creator;
import com.twilio.converter.Converter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.util.Map;
public class SyncMapItemCreator extends Creator {
private final String pathServiceSid;
private final String pathMapSid;
private final String key;
private final Map data;
private Integer ttl;
private Integer itemTtl;
private Integer collectionTtl;
/**
* Construct a new SyncMapItemCreator.
*
* @param pathServiceSid The SID of the Sync Service to create the Map Item in
* @param pathMapSid The SID of the Sync Map to add the new Map Item to
* @param key The unique, user-defined key for the Map Item
* @param data A JSON string that represents an arbitrary, schema-less object
* that the Map Item stores
*/
public SyncMapItemCreator(final String pathServiceSid,
final String pathMapSid,
final String key,
final Map data) {
this.pathServiceSid = pathServiceSid;
this.pathMapSid = pathMapSid;
this.key = key;
this.data = data;
}
/**
* An alias for `item_ttl`. If both parameters are provided, this value is
* ignored..
*
* @param ttl An alias for item_ttl
* @return this
*/
public SyncMapItemCreator setTtl(final Integer ttl) {
this.ttl = ttl;
return this;
}
/**
* How long, in
* seconds, before the Map Item expires (time-to-live) and is deleted..
*
* @param itemTtl How long, in seconds, before the Map Item expires
* @return this
*/
public SyncMapItemCreator setItemTtl(final Integer itemTtl) {
this.itemTtl = itemTtl;
return this;
}
/**
* How long, in
* seconds, before the Map Item's parent Sync Map expires (time-to-live) and
* is deleted..
*
* @param collectionTtl How long, in seconds, before the Map Item's parent Sync
* Map expires and is deleted
* @return this
*/
public SyncMapItemCreator setCollectionTtl(final Integer collectionTtl) {
this.collectionTtl = collectionTtl;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created SyncMapItem
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public SyncMapItem create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.SYNC.toString(),
"/v1/Services/" + this.pathServiceSid + "/Maps/" + this.pathMapSid + "/Items"
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("SyncMapItem creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return SyncMapItem.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (key != null) {
request.addPostParam("Key", key);
}
if (data != null) {
request.addPostParam("Data", Converter.mapToJson(data));
}
if (ttl != null) {
request.addPostParam("Ttl", ttl.toString());
}
if (itemTtl != null) {
request.addPostParam("ItemTtl", itemTtl.toString());
}
if (collectionTtl != null) {
request.addPostParam("CollectionTtl", collectionTtl.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy