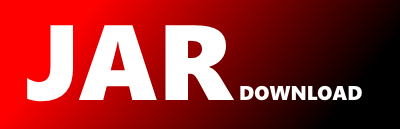
com.twilio.twiml.voice.Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.twiml.voice;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
import com.twilio.converter.Promoter;
import com.twilio.http.HttpMethod;
import com.twilio.twiml.TwiML;
import com.twilio.twiml.TwiMLException;
import java.net.URI;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* TwiML wrapper for {@code }
*/
@JsonDeserialize(builder = Client.Builder.class)
public class Client extends TwiML {
public enum Event {
INITIATED("initiated"),
RINGING("ringing"),
ANSWERED("answered"),
COMPLETED("completed");
private final String value;
private Event(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
private final URI url;
private final HttpMethod method;
private final List statusCallbackEvent;
private final URI statusCallback;
private final HttpMethod statusCallbackMethod;
private final String identity;
/**
* For XML Serialization/Deserialization
*/
private Client() {
this(new Builder());
}
/**
* Create a new {@code } element
*/
private Client(Builder b) {
super("Client", b);
this.url = b.url;
this.method = b.method;
this.statusCallbackEvent = b.statusCallbackEvent;
this.statusCallback = b.statusCallback;
this.statusCallbackMethod = b.statusCallbackMethod;
this.identity = b.identity;
}
/**
* The body of the TwiML element
*
* @return Element body as a string if present else null
*/
protected String getElementBody() {
return this.getIdentity() == null ? null : this.getIdentity();
}
/**
* Attributes to set on the generated XML element
*
* @return A Map of attribute keys to values
*/
protected Map getElementAttributes() {
// Preserve order of attributes
Map attrs = new HashMap<>();
if (this.getUrl() != null) {
attrs.put("url", this.getUrl().toString());
}
if (this.getMethod() != null) {
attrs.put("method", this.getMethod().toString());
}
if (this.getStatusCallbackEvents() != null) {
attrs.put("statusCallbackEvent", this.getStatusCallbackEventsAsString());
}
if (this.getStatusCallback() != null) {
attrs.put("statusCallback", this.getStatusCallback().toString());
}
if (this.getStatusCallbackMethod() != null) {
attrs.put("statusCallbackMethod", this.getStatusCallbackMethod().toString());
}
return attrs;
}
/**
* Client URL
*
* @return Client URL
*/
public URI getUrl() {
return url;
}
/**
* Client URL Method
*
* @return Client URL Method
*/
public HttpMethod getMethod() {
return method;
}
/**
* Events to trigger status callback
*
* @return Events to trigger status callback
*/
public List getStatusCallbackEvents() {
return statusCallbackEvent;
}
protected String getStatusCallbackEventsAsString() {
StringBuilder sb = new StringBuilder();
Iterator iter = this.getStatusCallbackEvents().iterator();
while (iter.hasNext()) {
sb.append(iter.next().toString());
if (iter.hasNext()) {
sb.append(" ");
}
}
return sb.toString();
}
/**
* Status Callback URL
*
* @return Status Callback URL
*/
public URI getStatusCallback() {
return statusCallback;
}
/**
* Status Callback URL Method
*
* @return Status Callback URL Method
*/
public HttpMethod getStatusCallbackMethod() {
return statusCallbackMethod;
}
/**
* Client identity
*
* @return Client identity
*/
public String getIdentity() {
return identity;
}
/**
* Create a new {@code } element
*/
public static class Builder extends TwiML.Builder {
/**
* Create and return a {@code } from an XML string
*/
public static Builder fromXml(final String xml) throws TwiMLException {
try {
return OBJECT_MAPPER.readValue(xml, Builder.class);
} catch (final JsonProcessingException jpe) {
throw new TwiMLException(
"Failed to deserialize a Client.Builder from the provided XML string: " + jpe.getMessage());
} catch (final Exception e) {
throw new TwiMLException("Unhandled exception: " + e.getMessage());
}
}
private URI url;
private HttpMethod method;
private List statusCallbackEvent;
private URI statusCallback;
private HttpMethod statusCallbackMethod;
private String identity;
/**
* Create a {@code } with identity
*/
public Builder(String identity) {
this.identity = identity;
}
/**
* Create a {@code } with child elements
*/
public Builder() {
}
/**
* Client URL
*/
@JacksonXmlProperty(isAttribute = true, localName = "url")
public Builder url(URI url) {
this.url = url;
return this;
}
/**
* Client URL
*/
public Builder url(String url) {
this.url = Promoter.uriFromString(url);
return this;
}
/**
* Client URL Method
*/
@JacksonXmlProperty(isAttribute = true, localName = "method")
public Builder method(HttpMethod method) {
this.method = method;
return this;
}
/**
* Events to trigger status callback
*/
@JacksonXmlProperty(isAttribute = true, localName = "statusCallbackEvent")
public Builder statusCallbackEvents(List statusCallbackEvent) {
this.statusCallbackEvent = statusCallbackEvent;
return this;
}
/**
* Events to trigger status callback
*/
public Builder statusCallbackEvents(Client.Event statusCallbackEvent) {
this.statusCallbackEvent = Promoter.listOfOne(statusCallbackEvent);
return this;
}
/**
* Status Callback URL
*/
@JacksonXmlProperty(isAttribute = true, localName = "statusCallback")
public Builder statusCallback(URI statusCallback) {
this.statusCallback = statusCallback;
return this;
}
/**
* Status Callback URL
*/
public Builder statusCallback(String statusCallback) {
this.statusCallback = Promoter.uriFromString(statusCallback);
return this;
}
/**
* Status Callback URL Method
*/
@JacksonXmlProperty(isAttribute = true, localName = "statusCallbackMethod")
public Builder statusCallbackMethod(HttpMethod statusCallbackMethod) {
this.statusCallbackMethod = statusCallbackMethod;
return this;
}
/**
* Client identity
*/
@JacksonXmlProperty(isAttribute = true, localName = "identity")
public Builder identity(String identity) {
this.identity = identity;
return this;
}
/**
* Add a child {@code } element
*/
@JacksonXmlProperty(isAttribute = false, localName = "Identity")
public Builder identity(Identity identity) {
this.children.add(identity);
return this;
}
/**
* Add a child {@code } element
*/
@JacksonXmlProperty(isAttribute = false, localName = "Parameter")
public Builder parameter(Parameter parameter) {
this.children.add(parameter);
return this;
}
/**
* Create and return resulting {@code } element
*/
public Client build() {
return new Client(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy