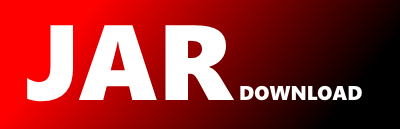
com.twilio.rest.api.v2010.account.AvailablePhoneNumberCountry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.twilio.base.Resource;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import lombok.ToString;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.Map;
import java.util.Objects;
@JsonIgnoreProperties(ignoreUnknown = true)
@ToString
public class AvailablePhoneNumberCountry extends Resource {
private static final long serialVersionUID = 118317602820324L;
/**
* Create a AvailablePhoneNumberCountryReader to execute read.
*
* @param pathAccountSid The SID of the Account requesting the available phone
* number Country resources
* @return AvailablePhoneNumberCountryReader capable of executing the read
*/
public static AvailablePhoneNumberCountryReader reader(final String pathAccountSid) {
return new AvailablePhoneNumberCountryReader(pathAccountSid);
}
/**
* Create a AvailablePhoneNumberCountryReader to execute read.
*
* @return AvailablePhoneNumberCountryReader capable of executing the read
*/
public static AvailablePhoneNumberCountryReader reader() {
return new AvailablePhoneNumberCountryReader();
}
/**
* Create a AvailablePhoneNumberCountryFetcher to execute fetch.
*
* @param pathAccountSid The SID of the Account requesting the available phone
* number Country resource
* @param pathCountryCode The ISO country code of the country to fetch
* available phone number information about
* @return AvailablePhoneNumberCountryFetcher capable of executing the fetch
*/
public static AvailablePhoneNumberCountryFetcher fetcher(final String pathAccountSid,
final String pathCountryCode) {
return new AvailablePhoneNumberCountryFetcher(pathAccountSid, pathCountryCode);
}
/**
* Create a AvailablePhoneNumberCountryFetcher to execute fetch.
*
* @param pathCountryCode The ISO country code of the country to fetch
* available phone number information about
* @return AvailablePhoneNumberCountryFetcher capable of executing the fetch
*/
public static AvailablePhoneNumberCountryFetcher fetcher(final String pathCountryCode) {
return new AvailablePhoneNumberCountryFetcher(pathCountryCode);
}
/**
* Converts a JSON String into a AvailablePhoneNumberCountry object using the
* provided ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return AvailablePhoneNumberCountry object represented by the provided JSON
*/
public static AvailablePhoneNumberCountry fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, AvailablePhoneNumberCountry.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a AvailablePhoneNumberCountry object using
* the provided ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return AvailablePhoneNumberCountry object represented by the provided JSON
*/
public static AvailablePhoneNumberCountry fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, AvailablePhoneNumberCountry.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String countryCode;
private final String country;
private final URI uri;
private final Boolean beta;
private final Map subresourceUris;
@JsonCreator
private AvailablePhoneNumberCountry(@JsonProperty("country_code")
final String countryCode,
@JsonProperty("country")
final String country,
@JsonProperty("uri")
final URI uri,
@JsonProperty("beta")
final Boolean beta,
@JsonProperty("subresource_uris")
final Map subresourceUris) {
this.countryCode = countryCode;
this.country = country;
this.uri = uri;
this.beta = beta;
this.subresourceUris = subresourceUris;
}
/**
* Returns The ISO-3166-1 country code of the country..
*
* @return The ISO-3166-1 country code of the country.
*/
public final String getCountryCode() {
return this.countryCode;
}
/**
* Returns The name of the country.
*
* @return The name of the country
*/
public final String getCountry() {
return this.country;
}
/**
* Returns The URI of the Country resource, relative to
* `https://api.twilio.com`.
*
* @return The URI of the Country resource, relative to `https://api.twilio.com`
*/
public final URI getUri() {
return this.uri;
}
/**
* Returns Whether all phone numbers available in the country are new to the
* Twilio platform..
*
* @return Whether all phone numbers available in the country are new to the
* Twilio platform.
*/
public final Boolean getBeta() {
return this.beta;
}
/**
* Returns A list of related resources identified by their relative URIs.
*
* @return A list of related resources identified by their relative URIs
*/
public final Map getSubresourceUris() {
return this.subresourceUris;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AvailablePhoneNumberCountry other = (AvailablePhoneNumberCountry) o;
return Objects.equals(countryCode, other.countryCode) &&
Objects.equals(country, other.country) &&
Objects.equals(uri, other.uri) &&
Objects.equals(beta, other.beta) &&
Objects.equals(subresourceUris, other.subresourceUris);
}
@Override
public int hashCode() {
return Objects.hash(countryCode,
country,
uri,
beta,
subresourceUris);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy