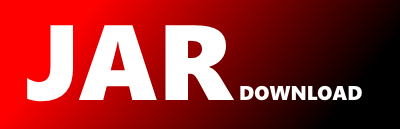
com.twilio.rest.taskrouter.v1.workspace.taskqueue.TaskQueuesStatisticsReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.taskrouter.v1.workspace.taskqueue;
import com.twilio.base.Page;
import com.twilio.base.Reader;
import com.twilio.base.ResourceSet;
import com.twilio.converter.DateConverter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.time.ZonedDateTime;
public class TaskQueuesStatisticsReader extends Reader {
private final String pathWorkspaceSid;
private ZonedDateTime endDate;
private String friendlyName;
private Integer minutes;
private ZonedDateTime startDate;
private String taskChannel;
private String splitByWaitTime;
/**
* Construct a new TaskQueuesStatisticsReader.
*
* @param pathWorkspaceSid The SID of the Workspace with the TaskQueues to read
*/
public TaskQueuesStatisticsReader(final String pathWorkspaceSid) {
this.pathWorkspaceSid = pathWorkspaceSid;
}
/**
* Only calculate statistics from this date and time and earlier, specified in
* GMT as an ISO 8601
* date-time..
*
* @param endDate Only calculate statistics from on or before this date
* @return this
*/
public TaskQueuesStatisticsReader setEndDate(final ZonedDateTime endDate) {
this.endDate = endDate;
return this;
}
/**
* The `friendly_name` of the TaskQueue statistics to read..
*
* @param friendlyName The friendly_name of the TaskQueue statistics to read
* @return this
*/
public TaskQueuesStatisticsReader setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* Only calculate statistics since this many minutes in the past. The default is
* 15 minutes..
*
* @param minutes Only calculate statistics since this many minutes in the past
* @return this
*/
public TaskQueuesStatisticsReader setMinutes(final Integer minutes) {
this.minutes = minutes;
return this;
}
/**
* Only calculate statistics from this date and time and later, specified in ISO 8601 format..
*
* @param startDate Only calculate statistics from on or after this date
* @return this
*/
public TaskQueuesStatisticsReader setStartDate(final ZonedDateTime startDate) {
this.startDate = startDate;
return this;
}
/**
* Only calculate statistics on this TaskChannel. Can be the TaskChannel's SID
* or its `unique_name`, such as `voice`, `sms`, or `default`..
*
* @param taskChannel Only calculate statistics on this TaskChannel.
* @return this
*/
public TaskQueuesStatisticsReader setTaskChannel(final String taskChannel) {
this.taskChannel = taskChannel;
return this;
}
/**
* A comma separated list of values that describes the thresholds, in seconds,
* to calculate statistics on. For each threshold specified, the number of Tasks
* canceled and reservations accepted above and below the specified thresholds
* in seconds are computed..
*
* @param splitByWaitTime A comma separated list of values that describes the
* thresholds to calculate statistics on
* @return this
*/
public TaskQueuesStatisticsReader setSplitByWaitTime(final String splitByWaitTime) {
this.splitByWaitTime = splitByWaitTime;
return this;
}
/**
* Make the request to the Twilio API to perform the read.
*
* @param client TwilioRestClient with which to make the request
* @return TaskQueuesStatistics ResourceSet
*/
@Override
public ResourceSet read(final TwilioRestClient client) {
return new ResourceSet<>(this, client, firstPage(client));
}
/**
* Make the request to the Twilio API to perform the read.
*
* @param client TwilioRestClient with which to make the request
* @return TaskQueuesStatistics ResourceSet
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Page firstPage(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
Domains.TASKROUTER.toString(),
"/v1/Workspaces/" + this.pathWorkspaceSid + "/TaskQueues/Statistics"
);
addQueryParams(request);
return pageForRequest(client, request);
}
/**
* Retrieve the target page from the Twilio API.
*
* @param targetUrl API-generated URL for the requested results page
* @param client TwilioRestClient with which to make the request
* @return TaskQueuesStatistics ResourceSet
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Page getPage(final String targetUrl, final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
targetUrl
);
return pageForRequest(client, request);
}
/**
* Retrieve the next page from the Twilio API.
*
* @param page current page
* @param client TwilioRestClient with which to make the request
* @return Next Page
*/
@Override
public Page nextPage(final Page page,
final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
page.getNextPageUrl(Domains.TASKROUTER.toString())
);
return pageForRequest(client, request);
}
/**
* Retrieve the previous page from the Twilio API.
*
* @param page current page
* @param client TwilioRestClient with which to make the request
* @return Previous Page
*/
@Override
public Page previousPage(final Page page,
final TwilioRestClient client) {
Request request = new Request(
HttpMethod.GET,
page.getPreviousPageUrl(Domains.TASKROUTER.toString())
);
return pageForRequest(client, request);
}
/**
* Generate a Page of TaskQueuesStatistics Resources for a given request.
*
* @param client TwilioRestClient with which to make the request
* @param request Request to generate a page for
* @return Page for the Request
*/
private Page pageForRequest(final TwilioRestClient client, final Request request) {
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("TaskQueuesStatistics read failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return Page.fromJson(
"task_queues_statistics",
response.getContent(),
TaskQueuesStatistics.class,
client.getObjectMapper()
);
}
/**
* Add the requested query string arguments to the Request.
*
* @param request Request to add query string arguments to
*/
private void addQueryParams(final Request request) {
if (endDate != null) {
request.addQueryParam("EndDate", endDate.toOffsetDateTime().toString());
}
if (friendlyName != null) {
request.addQueryParam("FriendlyName", friendlyName);
}
if (minutes != null) {
request.addQueryParam("Minutes", minutes.toString());
}
if (startDate != null) {
request.addQueryParam("StartDate", startDate.toOffsetDateTime().toString());
}
if (taskChannel != null) {
request.addQueryParam("TaskChannel", taskChannel);
}
if (splitByWaitTime != null) {
request.addQueryParam("SplitByWaitTime", splitByWaitTime);
}
if (getPageSize() != null) {
request.addQueryParam("PageSize", Integer.toString(getPageSize()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy