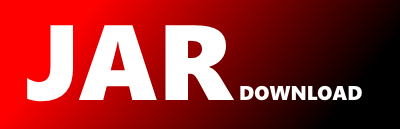
com.twilio.rest.accounts.v1.credential.PublicKeyReader Maven / Gradle / Ivy
/*
* This code was generated by
* ___ _ _ _ _ _ _ ____ ____ ____ _ ____ ____ _ _ ____ ____ ____ ___ __ __
* | | | | | | | | | __ | | |__| | __ | __ |___ |\ | |___ |__/ |__| | | | |__/
* | |_|_| | |___ | |__| |__| | | | |__] |___ | \| |___ | \ | | | |__| | \
*
* Twilio - Accounts
* This is the public Twilio REST API.
*
* NOTE: This class is auto generated by OpenAPI Generator.
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.twilio.rest.accounts.v1.credential;
import com.twilio.base.Page;
import com.twilio.base.Reader;
import com.twilio.base.ResourceSet;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
public class PublicKeyReader extends Reader {
private Integer pageSize;
public PublicKeyReader() {}
public PublicKeyReader setPageSize(final Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public ResourceSet read(final TwilioRestClient client) {
return new ResourceSet<>(this, client, firstPage(client));
}
public Page firstPage(final TwilioRestClient client) {
String path = "/v1/Credentials/PublicKeys";
Request request = new Request(
HttpMethod.GET,
Domains.ACCOUNTS.toString(),
path
);
addQueryParams(request);
return pageForRequest(client, request);
}
private Page pageForRequest(
final TwilioRestClient client,
final Request request
) {
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException(
"PublicKey read failed: Unable to connect to server"
);
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(
response.getStream(),
client.getObjectMapper()
);
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return Page.fromJson(
"credentials",
response.getContent(),
PublicKey.class,
client.getObjectMapper()
);
}
@Override
public Page previousPage(
final Page page,
final TwilioRestClient client
) {
Request request = new Request(
HttpMethod.GET,
page.getPreviousPageUrl(Domains.ACCOUNTS.toString())
);
return pageForRequest(client, request);
}
@Override
public Page nextPage(
final Page page,
final TwilioRestClient client
) {
Request request = new Request(
HttpMethod.GET,
page.getNextPageUrl(Domains.ACCOUNTS.toString())
);
return pageForRequest(client, request);
}
@Override
public Page getPage(
final String targetUrl,
final TwilioRestClient client
) {
Request request = new Request(HttpMethod.GET, targetUrl);
return pageForRequest(client, request);
}
private void addQueryParams(final Request request) {
if (pageSize != null) {
request.addQueryParam("PageSize", pageSize.toString());
}
if (getPageSize() != null) {
request.addQueryParam("PageSize", Integer.toString(getPageSize()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy