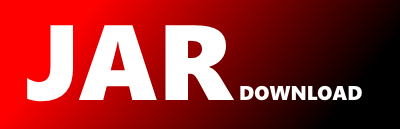
com.twilio.twiml.voice.Record Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.twiml.voice;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
import com.twilio.converter.Promoter;
import com.twilio.http.HttpMethod;
import com.twilio.twiml.TwiML;
import com.twilio.twiml.TwiMLException;
import java.net.URI;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* TwiML wrapper for {@code }
*/
@JsonDeserialize(builder = Record.Builder.class)
public class Record extends TwiML {
public enum Trim {
TRIM_SILENCE("trim-silence"),
DO_NOT_TRIM("do-not-trim");
private final String value;
private Trim(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
public enum RecordingEvent {
IN_PROGRESS("in-progress"),
COMPLETED("completed"),
ABSENT("absent");
private final String value;
private RecordingEvent(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
private final URI action;
private final HttpMethod method;
private final Integer timeout;
private final String finishOnKey;
private final Integer maxLength;
private final Boolean playBeep;
private final Record.Trim trim;
private final URI recordingStatusCallback;
private final HttpMethod recordingStatusCallbackMethod;
private final List recordingStatusCallbackEvent;
private final Boolean transcribe;
private final URI transcribeCallback;
/**
* For XML Serialization/Deserialization
*/
private Record() {
this(new Builder());
}
/**
* Create a new {@code } element
*/
private Record(Builder b) {
super("Record", b);
this.action = b.action;
this.method = b.method;
this.timeout = b.timeout;
this.finishOnKey = b.finishOnKey;
this.maxLength = b.maxLength;
this.playBeep = b.playBeep;
this.trim = b.trim;
this.recordingStatusCallback = b.recordingStatusCallback;
this.recordingStatusCallbackMethod = b.recordingStatusCallbackMethod;
this.recordingStatusCallbackEvent = b.recordingStatusCallbackEvent;
this.transcribe = b.transcribe;
this.transcribeCallback = b.transcribeCallback;
}
/**
* Attributes to set on the generated XML element
*
* @return A Map of attribute keys to values
*/
protected Map getElementAttributes() {
// Preserve order of attributes
Map attrs = new HashMap<>();
if (this.getAction() != null) {
attrs.put("action", this.getAction().toString());
}
if (this.getMethod() != null) {
attrs.put("method", this.getMethod().toString());
}
if (this.getTimeout() != null) {
attrs.put("timeout", this.getTimeout().toString());
}
if (this.getFinishOnKey() != null) {
attrs.put("finishOnKey", this.getFinishOnKey());
}
if (this.getMaxLength() != null) {
attrs.put("maxLength", this.getMaxLength().toString());
}
if (this.isPlayBeep() != null) {
attrs.put("playBeep", this.isPlayBeep().toString());
}
if (this.getTrim() != null) {
attrs.put("trim", this.getTrim().toString());
}
if (this.getRecordingStatusCallback() != null) {
attrs.put("recordingStatusCallback", this.getRecordingStatusCallback().toString());
}
if (this.getRecordingStatusCallbackMethod() != null) {
attrs.put("recordingStatusCallbackMethod", this.getRecordingStatusCallbackMethod().toString());
}
if (this.getRecordingStatusCallbackEvents() != null) {
attrs.put("recordingStatusCallbackEvent", this.getRecordingStatusCallbackEventsAsString());
}
if (this.isTranscribe() != null) {
attrs.put("transcribe", this.isTranscribe().toString());
}
if (this.getTranscribeCallback() != null) {
attrs.put("transcribeCallback", this.getTranscribeCallback().toString());
}
return attrs;
}
/**
* Action URL
*
* @return Action URL
*/
public URI getAction() {
return action;
}
/**
* Action URL method
*
* @return Action URL method
*/
public HttpMethod getMethod() {
return method;
}
/**
* Timeout to begin recording
*
* @return Timeout to begin recording
*/
public Integer getTimeout() {
return timeout;
}
/**
* Finish recording on key
*
* @return Finish recording on key
*/
public String getFinishOnKey() {
return finishOnKey;
}
/**
* Max time to record in seconds
*
* @return Max time to record in seconds
*/
public Integer getMaxLength() {
return maxLength;
}
/**
* Play beep
*
* @return Play beep
*/
public Boolean isPlayBeep() {
return playBeep;
}
/**
* Trim the recording
*
* @return Trim the recording
*/
public Record.Trim getTrim() {
return trim;
}
/**
* Status callback URL
*
* @return Status callback URL
*/
public URI getRecordingStatusCallback() {
return recordingStatusCallback;
}
/**
* Status callback URL method
*
* @return Status callback URL method
*/
public HttpMethod getRecordingStatusCallbackMethod() {
return recordingStatusCallbackMethod;
}
/**
* Recording status callback events
*
* @return Recording status callback events
*/
public List getRecordingStatusCallbackEvents() {
return recordingStatusCallbackEvent;
}
protected String getRecordingStatusCallbackEventsAsString() {
StringBuilder sb = new StringBuilder();
Iterator iter = this.getRecordingStatusCallbackEvents().iterator();
while (iter.hasNext()) {
sb.append(iter.next().toString());
if (iter.hasNext()) {
sb.append(" ");
}
}
return sb.toString();
}
/**
* Transcribe the recording
*
* @return Transcribe the recording
*/
public Boolean isTranscribe() {
return transcribe;
}
/**
* Transcribe callback URL
*
* @return Transcribe callback URL
*/
public URI getTranscribeCallback() {
return transcribeCallback;
}
/**
* Create a new {@code } element
*/
@JsonPOJOBuilder(withPrefix = "")
public static class Builder extends TwiML.Builder {
/**
* Create and return a {@code } from an XML string
*/
public static Builder fromXml(final String xml) throws TwiMLException {
try {
return OBJECT_MAPPER.readValue(xml, Builder.class);
} catch (final JsonProcessingException jpe) {
throw new TwiMLException(
"Failed to deserialize a Record.Builder from the provided XML string: " + jpe.getMessage());
} catch (final Exception e) {
throw new TwiMLException("Unhandled exception: " + e.getMessage());
}
}
private URI action;
private HttpMethod method;
private Integer timeout;
private String finishOnKey;
private Integer maxLength;
private Boolean playBeep;
private Record.Trim trim;
private URI recordingStatusCallback;
private HttpMethod recordingStatusCallbackMethod;
private List recordingStatusCallbackEvent;
private Boolean transcribe;
private URI transcribeCallback;
/**
* Action URL
*/
@JacksonXmlProperty(isAttribute = true, localName = "action")
public Builder action(URI action) {
this.action = action;
return this;
}
/**
* Action URL
*/
public Builder action(String action) {
this.action = Promoter.uriFromString(action);
return this;
}
/**
* Action URL method
*/
@JacksonXmlProperty(isAttribute = true, localName = "method")
public Builder method(HttpMethod method) {
this.method = method;
return this;
}
/**
* Timeout to begin recording
*/
@JacksonXmlProperty(isAttribute = true, localName = "timeout")
public Builder timeout(Integer timeout) {
this.timeout = timeout;
return this;
}
/**
* Finish recording on key
*/
@JacksonXmlProperty(isAttribute = true, localName = "finishOnKey")
public Builder finishOnKey(String finishOnKey) {
this.finishOnKey = finishOnKey;
return this;
}
/**
* Max time to record in seconds
*/
@JacksonXmlProperty(isAttribute = true, localName = "maxLength")
public Builder maxLength(Integer maxLength) {
this.maxLength = maxLength;
return this;
}
/**
* Play beep
*/
@JacksonXmlProperty(isAttribute = true, localName = "playBeep")
public Builder playBeep(Boolean playBeep) {
this.playBeep = playBeep;
return this;
}
/**
* Trim the recording
*/
@JacksonXmlProperty(isAttribute = true, localName = "trim")
public Builder trim(Record.Trim trim) {
this.trim = trim;
return this;
}
/**
* Status callback URL
*/
@JacksonXmlProperty(isAttribute = true, localName = "recordingStatusCallback")
public Builder recordingStatusCallback(URI recordingStatusCallback) {
this.recordingStatusCallback = recordingStatusCallback;
return this;
}
/**
* Status callback URL
*/
public Builder recordingStatusCallback(String recordingStatusCallback) {
this.recordingStatusCallback = Promoter.uriFromString(recordingStatusCallback);
return this;
}
/**
* Status callback URL method
*/
@JacksonXmlProperty(isAttribute = true, localName = "recordingStatusCallbackMethod")
public Builder recordingStatusCallbackMethod(HttpMethod recordingStatusCallbackMethod) {
this.recordingStatusCallbackMethod = recordingStatusCallbackMethod;
return this;
}
/**
* Recording status callback events
*/
@JacksonXmlProperty(isAttribute = true, localName = "recordingStatusCallbackEvent")
public Builder recordingStatusCallbackEvents(List recordingStatusCallbackEvent) {
this.recordingStatusCallbackEvent = recordingStatusCallbackEvent;
return this;
}
/**
* Recording status callback events
*/
public Builder recordingStatusCallbackEvents(Record.RecordingEvent recordingStatusCallbackEvent) {
this.recordingStatusCallbackEvent = Promoter.listOfOne(recordingStatusCallbackEvent);
return this;
}
/**
* Transcribe the recording
*/
@JacksonXmlProperty(isAttribute = true, localName = "transcribe")
public Builder transcribe(Boolean transcribe) {
this.transcribe = transcribe;
return this;
}
/**
* Transcribe callback URL
*/
@JacksonXmlProperty(isAttribute = true, localName = "transcribeCallback")
public Builder transcribeCallback(URI transcribeCallback) {
this.transcribeCallback = transcribeCallback;
return this;
}
/**
* Transcribe callback URL
*/
public Builder transcribeCallback(String transcribeCallback) {
this.transcribeCallback = Promoter.uriFromString(transcribeCallback);
return this;
}
/**
* Create and return resulting {@code } element
*/
public Record build() {
return new Record(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy