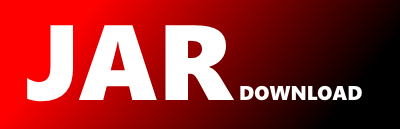
com.twilio.twiml.voice.SsmlSayAs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.twiml.voice;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
import com.twilio.twiml.TwiML;
import com.twilio.twiml.TwiMLException;
import java.util.HashMap;
import java.util.Map;
/**
* TwiML wrapper for {@code }
*/
@JsonDeserialize(builder = SsmlSayAs.Builder.class)
public class SsmlSayAs extends TwiML {
public enum InterpretAs {
CHARACTERS("characters"),
SPELL_OUT("spell-out"),
CARDINAL("cardinal"),
NUMBER("number"),
ORDINAL("ordinal"),
DIGITS("digits"),
FRACTION("fraction"),
UNIT("unit"),
DATE("date"),
TIME("time"),
ADDRESS("address"),
EXPLETIVE("expletive"),
TELEPHONE("telephone");
private final String value;
private InterpretAs(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
public enum Format {
MDY("mdy"),
DMY("dmy"),
YMD("ymd"),
MD("md"),
DM("dm"),
YM("ym"),
MY("my"),
D("d"),
M("m"),
Y("y"),
YYYYMMDD("yyyymmdd");
private final String value;
private Format(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
private final SsmlSayAs.InterpretAs interpretAs;
private final SsmlSayAs.Format format;
private final String words;
/**
* For XML Serialization/Deserialization
*/
private SsmlSayAs() {
this(new Builder((String) null));
}
/**
* Create a new {@code } element
*/
private SsmlSayAs(Builder b) {
super("say-as", b);
this.interpretAs = b.interpretAs;
this.format = b.format;
this.words = b.words;
}
/**
* The body of the TwiML element
*
* @return Element body as a string if present else null
*/
protected String getElementBody() {
return this.getWords() == null ? null : this.getWords();
}
/**
* Attributes to set on the generated XML element
*
* @return A Map of attribute keys to values
*/
protected Map getElementAttributes() {
// Preserve order of attributes
Map attrs = new HashMap<>();
if (this.getInterpretAs() != null) {
attrs.put("interpret-as", this.getInterpretAs().toString());
}
if (this.getFormat() != null) {
attrs.put("format", this.getFormat().toString());
}
return attrs;
}
/**
* Specify the type of words are spoken
*
* @return Specify the type of words are spoken
*/
public SsmlSayAs.InterpretAs getInterpretAs() {
return interpretAs;
}
/**
* Specify the format of the date when interpret-as is set to date
*
* @return Specify the format of the date when interpret-as is set to date
*/
public SsmlSayAs.Format getFormat() {
return format;
}
/**
* Words to be interpreted
*
* @return Words to be interpreted
*/
public String getWords() {
return words;
}
/**
* Create a new {@code } element
*/
@JsonPOJOBuilder(withPrefix = "")
public static class Builder extends TwiML.Builder {
/**
* Create and return a {@code } from an XML string
*/
public static Builder fromXml(final String xml) throws TwiMLException {
try {
return OBJECT_MAPPER.readValue(xml, Builder.class);
} catch (final JsonProcessingException jpe) {
throw new TwiMLException(
"Failed to deserialize a SsmlSayAs.Builder from the provided XML string: " + jpe.getMessage());
} catch (final Exception e) {
throw new TwiMLException("Unhandled exception: " + e.getMessage());
}
}
private SsmlSayAs.InterpretAs interpretAs;
private SsmlSayAs.Format format;
private String words;
/**
* Create a {@code } with words
*/
public Builder(String words) {
this.words = words;
}
/**
* Create a {@code } (for XML deserialization)
*/
private Builder() {
}
/**
* Specify the type of words are spoken
*/
@JacksonXmlProperty(isAttribute = true, localName = "interpret-as")
public Builder interpretAs(SsmlSayAs.InterpretAs interpretAs) {
this.interpretAs = interpretAs;
return this;
}
/**
* Specify the format of the date when interpret-as is set to date
*/
@JacksonXmlProperty(isAttribute = true, localName = "format")
public Builder format(SsmlSayAs.Format format) {
this.format = format;
return this;
}
/**
* Create and return resulting {@code } element
*/
public SsmlSayAs build() {
return new SsmlSayAs(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy