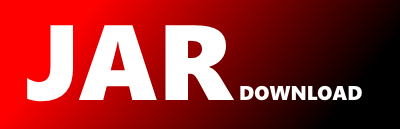
com.twilio.twiml.voice.Task Maven / Gradle / Ivy
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.twiml.voice;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
import com.twilio.twiml.TwiML;
import com.twilio.twiml.TwiMLException;
import java.util.HashMap;
import java.util.Map;
/**
* TwiML wrapper for {@code }
*/
@JsonDeserialize(builder = Task.Builder.class)
public class Task extends TwiML {
private final Integer priority;
private final Integer timeout;
private final String body;
/**
* For XML Serialization/Deserialization
*/
private Task() {
this(new Builder((String) null));
}
/**
* Create a new {@code } element
*/
private Task(Builder b) {
super("Task", b);
this.priority = b.priority;
this.timeout = b.timeout;
this.body = b.body;
}
/**
* The body of the TwiML element
*
* @return Element body as a string if present else null
*/
protected String getElementBody() {
return this.getBody() == null ? null : this.getBody();
}
/**
* Attributes to set on the generated XML element
*
* @return A Map of attribute keys to values
*/
protected Map getElementAttributes() {
// Preserve order of attributes
Map attrs = new HashMap<>();
if (this.getPriority() != null) {
attrs.put("priority", this.getPriority().toString());
}
if (this.getTimeout() != null) {
attrs.put("timeout", this.getTimeout().toString());
}
return attrs;
}
/**
* Task priority
*
* @return Task priority
*/
public Integer getPriority() {
return priority;
}
/**
* Timeout associated with task
*
* @return Timeout associated with task
*/
public Integer getTimeout() {
return timeout;
}
/**
* TaskRouter task attributes
*
* @return TaskRouter task attributes
*/
public String getBody() {
return body;
}
/**
* Create a new {@code } element
*/
@JsonPOJOBuilder(withPrefix = "")
public static class Builder extends TwiML.Builder {
/**
* Create and return a {@code } from an XML string
*/
public static Builder fromXml(final String xml) throws TwiMLException {
try {
return OBJECT_MAPPER.readValue(xml, Builder.class);
} catch (final JsonProcessingException jpe) {
throw new TwiMLException(
"Failed to deserialize a Task.Builder from the provided XML string: " + jpe.getMessage());
} catch (final Exception e) {
throw new TwiMLException("Unhandled exception: " + e.getMessage());
}
}
private Integer priority;
private Integer timeout;
private String body;
/**
* Create a {@code } with body
*/
public Builder(String body) {
this.body = body;
}
/**
* Create a {@code } (for XML deserialization)
*/
private Builder() {
}
/**
* Task priority
*/
@JacksonXmlProperty(isAttribute = true, localName = "priority")
public Builder priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* Timeout associated with task
*/
@JacksonXmlProperty(isAttribute = true, localName = "timeout")
public Builder timeout(Integer timeout) {
this.timeout = timeout;
return this;
}
/**
* Create and return resulting {@code } element
*/
public Task build() {
return new Task(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy