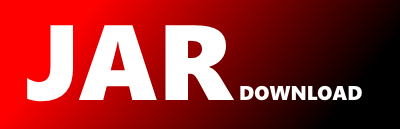
com.twineworks.tweakflow.grammar.TweakFlowParserVisitor Maven / Gradle / Ivy
// Generated from com/twineworks/tweakflow/grammar/TweakFlowParser.g4 by ANTLR 4.7.2
package com.twineworks.tweakflow.grammar;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link TweakFlowParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface TweakFlowParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by the {@code moduleUnit}
* labeled alternative in {@link TweakFlowParser#unit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModuleUnit(TweakFlowParser.ModuleUnitContext ctx);
/**
* Visit a parse tree produced by the {@code interactiveUnit}
* labeled alternative in {@link TweakFlowParser#unit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInteractiveUnit(TweakFlowParser.InteractiveUnitContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#interactive}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInteractive(TweakFlowParser.InteractiveContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#interactiveSection}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInteractiveSection(TweakFlowParser.InteractiveSectionContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#interactiveInput}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInteractiveInput(TweakFlowParser.InteractiveInputContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#standaloneExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStandaloneExpression(TweakFlowParser.StandaloneExpressionContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#standaloneReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStandaloneReference(TweakFlowParser.StandaloneReferenceContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#empty}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEmpty(TweakFlowParser.EmptyContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#module}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModule(TweakFlowParser.ModuleContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#moduleHead}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModuleHead(TweakFlowParser.ModuleHeadContext ctx);
/**
* Visit a parse tree produced by the {@code libraryComponent}
* labeled alternative in {@link TweakFlowParser#moduleComponent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLibraryComponent(TweakFlowParser.LibraryComponentContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#nameDec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNameDec(TweakFlowParser.NameDecContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#importDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportDef(TweakFlowParser.ImportDefContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#importMember}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportMember(TweakFlowParser.ImportMemberContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#moduleImport}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModuleImport(TweakFlowParser.ModuleImportContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#componentImport}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComponentImport(TweakFlowParser.ComponentImportContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#importModuleName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportModuleName(TweakFlowParser.ImportModuleNameContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#importComponentName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportComponentName(TweakFlowParser.ImportComponentNameContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#exportComponentName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExportComponentName(TweakFlowParser.ExportComponentNameContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#modulePath}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModulePath(TweakFlowParser.ModulePathContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#aliasDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAliasDef(TweakFlowParser.AliasDefContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#aliasName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAliasName(TweakFlowParser.AliasNameContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#exportDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExportDef(TweakFlowParser.ExportDefContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#exportName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExportName(TweakFlowParser.ExportNameContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#library}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLibrary(TweakFlowParser.LibraryContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#libVar}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLibVar(TweakFlowParser.LibVarContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#varDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVarDef(TweakFlowParser.VarDefContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#varDec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVarDec(TweakFlowParser.VarDecContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#provided}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitProvided(TweakFlowParser.ProvidedContext ctx);
/**
* Visit a parse tree produced by the {@code nilLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNilLiteralExp(TweakFlowParser.NilLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code booleanLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanLiteralExp(TweakFlowParser.BooleanLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code binaryLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinaryLiteralExp(TweakFlowParser.BinaryLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code dateTimeLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTimeLiteralExp(TweakFlowParser.DateTimeLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code stringConstantExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringConstantExp(TweakFlowParser.StringConstantExpContext ctx);
/**
* Visit a parse tree produced by the {@code longLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLongLiteralExp(TweakFlowParser.LongLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code doubleLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoubleLiteralExp(TweakFlowParser.DoubleLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code listLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitListLiteralExp(TweakFlowParser.ListLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code dictLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDictLiteralExp(TweakFlowParser.DictLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code functionLiteralExp}
* labeled alternative in {@link TweakFlowParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionLiteralExp(TweakFlowParser.FunctionLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code bitwiseXorExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBitwiseXorExp(TweakFlowParser.BitwiseXorExpContext ctx);
/**
* Visit a parse tree produced by the {@code partialExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPartialExp(TweakFlowParser.PartialExpContext ctx);
/**
* Visit a parse tree produced by the {@code lessThanExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLessThanExp(TweakFlowParser.LessThanExpContext ctx);
/**
* Visit a parse tree produced by the {@code castExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCastExp(TweakFlowParser.CastExpContext ctx);
/**
* Visit a parse tree produced by the {@code addExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAddExp(TweakFlowParser.AddExpContext ctx);
/**
* Visit a parse tree produced by the {@code forExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitForExp(TweakFlowParser.ForExpContext ctx);
/**
* Visit a parse tree produced by the {@code greaterThanExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGreaterThanExp(TweakFlowParser.GreaterThanExpContext ctx);
/**
* Visit a parse tree produced by the {@code containerAccessExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitContainerAccessExp(TweakFlowParser.ContainerAccessExpContext ctx);
/**
* Visit a parse tree produced by the {@code lessThanOrEqualToExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLessThanOrEqualToExp(TweakFlowParser.LessThanOrEqualToExpContext ctx);
/**
* Visit a parse tree produced by the {@code notValueAndTypeEqualsExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNotValueAndTypeEqualsExp(TweakFlowParser.NotValueAndTypeEqualsExpContext ctx);
/**
* Visit a parse tree produced by the {@code literalExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralExp(TweakFlowParser.LiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code threadExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThreadExp(TweakFlowParser.ThreadExpContext ctx);
/**
* Visit a parse tree produced by the {@code letExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLetExp(TweakFlowParser.LetExpContext ctx);
/**
* Visit a parse tree produced by the {@code tryCatchExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTryCatchExp(TweakFlowParser.TryCatchExpContext ctx);
/**
* Visit a parse tree produced by the {@code powExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPowExp(TweakFlowParser.PowExpContext ctx);
/**
* Visit a parse tree produced by the {@code threadExpErr}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThreadExpErr(TweakFlowParser.ThreadExpErrContext ctx);
/**
* Visit a parse tree produced by the {@code bitwiseNotExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBitwiseNotExp(TweakFlowParser.BitwiseNotExpContext ctx);
/**
* Visit a parse tree produced by the {@code multExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultExp(TweakFlowParser.MultExpContext ctx);
/**
* Visit a parse tree produced by the {@code referenceExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReferenceExp(TweakFlowParser.ReferenceExpContext ctx);
/**
* Visit a parse tree produced by the {@code shiftLeftExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShiftLeftExp(TweakFlowParser.ShiftLeftExpContext ctx);
/**
* Visit a parse tree produced by the {@code notEqualExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNotEqualExp(TweakFlowParser.NotEqualExpContext ctx);
/**
* Visit a parse tree produced by the {@code bitwiseOrExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBitwiseOrExp(TweakFlowParser.BitwiseOrExpContext ctx);
/**
* Visit a parse tree produced by the {@code typeOfExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeOfExp(TweakFlowParser.TypeOfExpContext ctx);
/**
* Visit a parse tree produced by the {@code throwErrorExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThrowErrorExp(TweakFlowParser.ThrowErrorExpContext ctx);
/**
* Visit a parse tree produced by the {@code zeroShiftRightExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitZeroShiftRightExp(TweakFlowParser.ZeroShiftRightExpContext ctx);
/**
* Visit a parse tree produced by the {@code preservingShiftRightExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPreservingShiftRightExp(TweakFlowParser.PreservingShiftRightExpContext ctx);
/**
* Visit a parse tree produced by the {@code boolAndExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBoolAndExp(TweakFlowParser.BoolAndExpContext ctx);
/**
* Visit a parse tree produced by the {@code subExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubExp(TweakFlowParser.SubExpContext ctx);
/**
* Visit a parse tree produced by the {@code bitwiseAndExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBitwiseAndExp(TweakFlowParser.BitwiseAndExpContext ctx);
/**
* Visit a parse tree produced by the {@code matchExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMatchExp(TweakFlowParser.MatchExpContext ctx);
/**
* Visit a parse tree produced by the {@code concatExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConcatExp(TweakFlowParser.ConcatExpContext ctx);
/**
* Visit a parse tree produced by the {@code boolNotExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBoolNotExp(TweakFlowParser.BoolNotExpContext ctx);
/**
* Visit a parse tree produced by the {@code debugExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDebugExp(TweakFlowParser.DebugExpContext ctx);
/**
* Visit a parse tree produced by the {@code callExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCallExp(TweakFlowParser.CallExpContext ctx);
/**
* Visit a parse tree produced by the {@code valueAndTypeEqualsExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueAndTypeEqualsExp(TweakFlowParser.ValueAndTypeEqualsExpContext ctx);
/**
* Visit a parse tree produced by the {@code divExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDivExp(TweakFlowParser.DivExpContext ctx);
/**
* Visit a parse tree produced by the {@code defaultExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDefaultExp(TweakFlowParser.DefaultExpContext ctx);
/**
* Visit a parse tree produced by the {@code equalExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEqualExp(TweakFlowParser.EqualExpContext ctx);
/**
* Visit a parse tree produced by the {@code isExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsExp(TweakFlowParser.IsExpContext ctx);
/**
* Visit a parse tree produced by the {@code modExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModExp(TweakFlowParser.ModExpContext ctx);
/**
* Visit a parse tree produced by the {@code boolOrExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBoolOrExp(TweakFlowParser.BoolOrExpContext ctx);
/**
* Visit a parse tree produced by the {@code unaryMinusExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryMinusExp(TweakFlowParser.UnaryMinusExpContext ctx);
/**
* Visit a parse tree produced by the {@code greaterThanOrEqualToExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGreaterThanOrEqualToExp(TweakFlowParser.GreaterThanOrEqualToExpContext ctx);
/**
* Visit a parse tree produced by the {@code intDivExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntDivExp(TweakFlowParser.IntDivExpContext ctx);
/**
* Visit a parse tree produced by the {@code ifExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIfExp(TweakFlowParser.IfExpContext ctx);
/**
* Visit a parse tree produced by the {@code nestedExp}
* labeled alternative in {@link TweakFlowParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNestedExp(TweakFlowParser.NestedExpContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#matchBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMatchBody(TweakFlowParser.MatchBodyContext ctx);
/**
* Visit a parse tree produced by the {@code patternLine}
* labeled alternative in {@link TweakFlowParser#matchLine}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPatternLine(TweakFlowParser.PatternLineContext ctx);
/**
* Visit a parse tree produced by the {@code defaultLine}
* labeled alternative in {@link TweakFlowParser#matchLine}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDefaultLine(TweakFlowParser.DefaultLineContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#matchGuard}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMatchGuard(TweakFlowParser.MatchGuardContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#varCapture}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVarCapture(TweakFlowParser.VarCaptureContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#splatCapture}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSplatCapture(TweakFlowParser.SplatCaptureContext ctx);
/**
* Visit a parse tree produced by the {@code capturePattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCapturePattern(TweakFlowParser.CapturePatternContext ctx);
/**
* Visit a parse tree produced by the {@code dataTypePattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDataTypePattern(TweakFlowParser.DataTypePatternContext ctx);
/**
* Visit a parse tree produced by the {@code headTailListPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeadTailListPattern(TweakFlowParser.HeadTailListPatternContext ctx);
/**
* Visit a parse tree produced by the {@code initLastListPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInitLastListPattern(TweakFlowParser.InitLastListPatternContext ctx);
/**
* Visit a parse tree produced by the {@code midListPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMidListPattern(TweakFlowParser.MidListPatternContext ctx);
/**
* Visit a parse tree produced by the {@code listPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitListPattern(TweakFlowParser.ListPatternContext ctx);
/**
* Visit a parse tree produced by the {@code dictPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDictPattern(TweakFlowParser.DictPatternContext ctx);
/**
* Visit a parse tree produced by the {@code openDictPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOpenDictPattern(TweakFlowParser.OpenDictPatternContext ctx);
/**
* Visit a parse tree produced by the {@code expPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpPattern(TweakFlowParser.ExpPatternContext ctx);
/**
* Visit a parse tree produced by the {@code errListPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitErrListPattern(TweakFlowParser.ErrListPatternContext ctx);
/**
* Visit a parse tree produced by the {@code errDictPattern}
* labeled alternative in {@link TweakFlowParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitErrDictPattern(TweakFlowParser.ErrDictPatternContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#threadArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThreadArg(TweakFlowParser.ThreadArgContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#generator}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGenerator(TweakFlowParser.GeneratorContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#forHead}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitForHead(TweakFlowParser.ForHeadContext ctx);
/**
* Visit a parse tree produced by the {@code catchAnonymous}
* labeled alternative in {@link TweakFlowParser#catchDeclaration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCatchAnonymous(TweakFlowParser.CatchAnonymousContext ctx);
/**
* Visit a parse tree produced by the {@code catchError}
* labeled alternative in {@link TweakFlowParser#catchDeclaration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCatchError(TweakFlowParser.CatchErrorContext ctx);
/**
* Visit a parse tree produced by the {@code catchErrorAndTrace}
* labeled alternative in {@link TweakFlowParser#catchDeclaration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCatchErrorAndTrace(TweakFlowParser.CatchErrorAndTraceContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#nilLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNilLiteral(TweakFlowParser.NilLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code stringVerbatim}
* labeled alternative in {@link TweakFlowParser#stringLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringVerbatim(TweakFlowParser.StringVerbatimContext ctx);
/**
* Visit a parse tree produced by the {@code stringHereDoc}
* labeled alternative in {@link TweakFlowParser#stringLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringHereDoc(TweakFlowParser.StringHereDocContext ctx);
/**
* Visit a parse tree produced by the {@code stringInterpolation}
* labeled alternative in {@link TweakFlowParser#stringLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringInterpolation(TweakFlowParser.StringInterpolationContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#stringText}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringText(TweakFlowParser.StringTextContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#stringEscapeSequence}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringEscapeSequence(TweakFlowParser.StringEscapeSequenceContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#unrecognizedEscapeSequence}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnrecognizedEscapeSequence(TweakFlowParser.UnrecognizedEscapeSequenceContext ctx);
/**
* Visit a parse tree produced by the {@code decLiteral}
* labeled alternative in {@link TweakFlowParser#longLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDecLiteral(TweakFlowParser.DecLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code hexLiteral}
* labeled alternative in {@link TweakFlowParser#longLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHexLiteral(TweakFlowParser.HexLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code binLiteral}
* labeled alternative in {@link TweakFlowParser#binaryLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinLiteral(TweakFlowParser.BinLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#doubleLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoubleLiteral(TweakFlowParser.DoubleLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#booleanLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanLiteral(TweakFlowParser.BooleanLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#dateTimeLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateTimeLiteral(TweakFlowParser.DateTimeLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#listLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitListLiteral(TweakFlowParser.ListLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#dictLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDictLiteral(TweakFlowParser.DictLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#wrongSideColonKey}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWrongSideColonKey(TweakFlowParser.WrongSideColonKeyContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#functionLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionLiteral(TweakFlowParser.FunctionLiteralContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#functionHead}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionHead(TweakFlowParser.FunctionHeadContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#paramsList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParamsList(TweakFlowParser.ParamsListContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#paramDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParamDef(TweakFlowParser.ParamDefContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#splat}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSplat(TweakFlowParser.SplatContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#dataType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDataType(TweakFlowParser.DataTypeContext ctx);
/**
* Visit a parse tree produced by the {@code localReference}
* labeled alternative in {@link TweakFlowParser#reference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLocalReference(TweakFlowParser.LocalReferenceContext ctx);
/**
* Visit a parse tree produced by the {@code globalReference}
* labeled alternative in {@link TweakFlowParser#reference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGlobalReference(TweakFlowParser.GlobalReferenceContext ctx);
/**
* Visit a parse tree produced by the {@code moduleReference}
* labeled alternative in {@link TweakFlowParser#reference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitModuleReference(TweakFlowParser.ModuleReferenceContext ctx);
/**
* Visit a parse tree produced by the {@code libraryReference}
* labeled alternative in {@link TweakFlowParser#reference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLibraryReference(TweakFlowParser.LibraryReferenceContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#identifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdentifier(TweakFlowParser.IdentifierContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#containerAccessKeySequence}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitContainerAccessKeySequence(TweakFlowParser.ContainerAccessKeySequenceContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#badContainerAccessKeySequence}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBadContainerAccessKeySequence(TweakFlowParser.BadContainerAccessKeySequenceContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#partialArgs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPartialArgs(TweakFlowParser.PartialArgsContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#namedPartialArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNamedPartialArg(TweakFlowParser.NamedPartialArgContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#args}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArgs(TweakFlowParser.ArgsContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#positionalArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPositionalArg(TweakFlowParser.PositionalArgContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#namedArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNamedArg(TweakFlowParser.NamedArgContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#splatArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSplatArg(TweakFlowParser.SplatArgContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#badArgs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBadArgs(TweakFlowParser.BadArgsContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#keyLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKeyLiteral(TweakFlowParser.KeyLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code keyLiteralExp}
* labeled alternative in {@link TweakFlowParser#stringConstant}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKeyLiteralExp(TweakFlowParser.KeyLiteralExpContext ctx);
/**
* Visit a parse tree produced by the {@code stringLiteralExp}
* labeled alternative in {@link TweakFlowParser#stringConstant}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringLiteralExp(TweakFlowParser.StringLiteralExpContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#viaDec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitViaDec(TweakFlowParser.ViaDecContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#metaDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMetaDef(TweakFlowParser.MetaDefContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#meta}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMeta(TweakFlowParser.MetaContext ctx);
/**
* Visit a parse tree produced by {@link TweakFlowParser#doc}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoc(TweakFlowParser.DocContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy