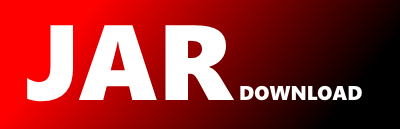
com.twitter.ambrose.service.impl.InMemoryStatsService Maven / Gradle / Ivy
/*
Copyright 2012 Twitter, Inc.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.twitter.ambrose.service.impl;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.Writer;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.SortedMap;
import java.util.concurrent.ConcurrentSkipListMap;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.twitter.ambrose.model.DAGNode;
import com.twitter.ambrose.model.Event;
import com.twitter.ambrose.model.Job;
import com.twitter.ambrose.model.PaginatedList;
import com.twitter.ambrose.model.WorkflowSummary;
import com.twitter.ambrose.service.StatsReadService;
import com.twitter.ambrose.service.StatsWriteService;
import com.twitter.ambrose.service.WorkflowIndexReadService;
import com.twitter.ambrose.util.JSONUtil;
/**
* In-memory implementation of both StatsReadService and StatsWriteService. Used when stats
* collection and stats serving are happening within the same VM. This class is intended to run in a
* VM that only handles a single workflow. Hence it ignores workflowId.
*
* Upon job completion this class can optionally write all json data to disk. This is useful for
* debugging. The written files can also be replayed in the Ambrose UI without re-running the Job
* via the bin/demo
script. To write all json data to disk, set the following values as
* system properties using -D
:
*
*
* {@value #DUMP_WORKFLOW_FILE_PARAM}
- file in which to write the workflow
* json.
* {@value #DUMP_EVENTS_FILE_PARAM}
- file in which to write the events
* json.
*
*
*/
public class InMemoryStatsService implements StatsReadService, StatsWriteService© 2015 - 2025 Weber Informatics LLC | Privacy Policy