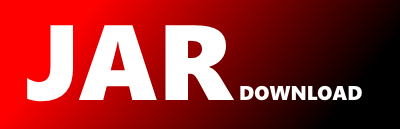
com.twitter.finagle.kestrel.java.ClientBase Maven / Gradle / Ivy
package com.twitter.finagle.kestrel.java;
import java.util.Iterator;
import java.util.concurrent.Callable;
import scala.Function0;
import scala.Option;
import scala.collection.JavaConversions;
import scala.collection.immutable.Stream;
import com.twitter.concurrent.Offer;
import com.twitter.finagle.kestrel.ReadHandle;
import com.twitter.finagle.kestrel.protocol.Response;
import com.twitter.io.Buf;
import com.twitter.util.Duration;
import com.twitter.util.Function;
import com.twitter.util.Future;
import com.twitter.util.Time;
import com.twitter.util.Timer;
public class ClientBase extends com.twitter.finagle.kestrel.java.Client {
protected com.twitter.finagle.kestrel.Client underlying;
public ClientBase(com.twitter.finagle.kestrel.Client underlying) {
this.underlying = underlying;
}
/**
* Dequeue an item.
*
* @param key the name of the queue.
* @param waitFor if the queue is empty, wait up to this duration for an item to arrive.
* @return A Future
*/
public Future get(String key, Duration waitFor) {
Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy