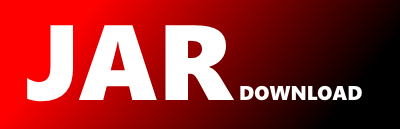
parquet.format.SortingColumn Maven / Gradle / Ivy
/**
* Autogenerated by Thrift
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
*/
package parquet.format;
import org.apache.commons.lang.builder.HashCodeBuilder;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.apache.thrift.*;
import org.apache.thrift.async.*;
import org.apache.thrift.meta_data.*;
import org.apache.thrift.transport.*;
import org.apache.thrift.protocol.*;
/**
* Wrapper struct to specify sort order
*/
public class SortingColumn implements TBase, java.io.Serializable, Cloneable {
private static final TStruct STRUCT_DESC = new TStruct("SortingColumn");
private static final TField COLUMN_IDX_FIELD_DESC = new TField("column_idx", TType.I32, (short)1);
private static final TField DESCENDING_FIELD_DESC = new TField("descending", TType.BOOL, (short)2);
private static final TField NULLS_FIRST_FIELD_DESC = new TField("nulls_first", TType.BOOL, (short)3);
/**
* The column index (in this row group) *
*/
public int column_idx;
/**
* If true, indicates this column is sorted in descending order. *
*/
public boolean descending;
/**
* If true, nulls will come before non-null values, otherwise,
* nulls go at the end.
*/
public boolean nulls_first;
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements TFieldIdEnum {
/**
* The column index (in this row group) *
*/
COLUMN_IDX((short)1, "column_idx"),
/**
* If true, indicates this column is sorted in descending order. *
*/
DESCENDING((short)2, "descending"),
/**
* If true, nulls will come before non-null values, otherwise,
* nulls go at the end.
*/
NULLS_FIRST((short)3, "nulls_first");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // COLUMN_IDX
return COLUMN_IDX;
case 2: // DESCENDING
return DESCENDING;
case 3: // NULLS_FIRST
return NULLS_FIRST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __COLUMN_IDX_ISSET_ID = 0;
private static final int __DESCENDING_ISSET_ID = 1;
private static final int __NULLS_FIRST_ISSET_ID = 2;
private BitSet __isset_bit_vector = new BitSet(3);
public static final Map<_Fields, FieldMetaData> metaDataMap;
static {
Map<_Fields, FieldMetaData> tmpMap = new EnumMap<_Fields, FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.COLUMN_IDX, new FieldMetaData("column_idx", TFieldRequirementType.REQUIRED,
new FieldValueMetaData(TType.I32)));
tmpMap.put(_Fields.DESCENDING, new FieldMetaData("descending", TFieldRequirementType.REQUIRED,
new FieldValueMetaData(TType.BOOL)));
tmpMap.put(_Fields.NULLS_FIRST, new FieldMetaData("nulls_first", TFieldRequirementType.REQUIRED,
new FieldValueMetaData(TType.BOOL)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
FieldMetaData.addStructMetaDataMap(SortingColumn.class, metaDataMap);
}
public SortingColumn() {
}
public SortingColumn(
int column_idx,
boolean descending,
boolean nulls_first)
{
this();
this.column_idx = column_idx;
setColumn_idxIsSet(true);
this.descending = descending;
setDescendingIsSet(true);
this.nulls_first = nulls_first;
setNulls_firstIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public SortingColumn(SortingColumn other) {
__isset_bit_vector.clear();
__isset_bit_vector.or(other.__isset_bit_vector);
this.column_idx = other.column_idx;
this.descending = other.descending;
this.nulls_first = other.nulls_first;
}
public SortingColumn deepCopy() {
return new SortingColumn(this);
}
@Override
public void clear() {
setColumn_idxIsSet(false);
this.column_idx = 0;
setDescendingIsSet(false);
this.descending = false;
setNulls_firstIsSet(false);
this.nulls_first = false;
}
/**
* The column index (in this row group) *
*/
public int getColumn_idx() {
return this.column_idx;
}
/**
* The column index (in this row group) *
*/
public SortingColumn setColumn_idx(int column_idx) {
this.column_idx = column_idx;
setColumn_idxIsSet(true);
return this;
}
public void unsetColumn_idx() {
__isset_bit_vector.clear(__COLUMN_IDX_ISSET_ID);
}
/** Returns true if field column_idx is set (has been asigned a value) and false otherwise */
public boolean isSetColumn_idx() {
return __isset_bit_vector.get(__COLUMN_IDX_ISSET_ID);
}
public void setColumn_idxIsSet(boolean value) {
__isset_bit_vector.set(__COLUMN_IDX_ISSET_ID, value);
}
/**
* If true, indicates this column is sorted in descending order. *
*/
public boolean isDescending() {
return this.descending;
}
/**
* If true, indicates this column is sorted in descending order. *
*/
public SortingColumn setDescending(boolean descending) {
this.descending = descending;
setDescendingIsSet(true);
return this;
}
public void unsetDescending() {
__isset_bit_vector.clear(__DESCENDING_ISSET_ID);
}
/** Returns true if field descending is set (has been asigned a value) and false otherwise */
public boolean isSetDescending() {
return __isset_bit_vector.get(__DESCENDING_ISSET_ID);
}
public void setDescendingIsSet(boolean value) {
__isset_bit_vector.set(__DESCENDING_ISSET_ID, value);
}
/**
* If true, nulls will come before non-null values, otherwise,
* nulls go at the end.
*/
public boolean isNulls_first() {
return this.nulls_first;
}
/**
* If true, nulls will come before non-null values, otherwise,
* nulls go at the end.
*/
public SortingColumn setNulls_first(boolean nulls_first) {
this.nulls_first = nulls_first;
setNulls_firstIsSet(true);
return this;
}
public void unsetNulls_first() {
__isset_bit_vector.clear(__NULLS_FIRST_ISSET_ID);
}
/** Returns true if field nulls_first is set (has been asigned a value) and false otherwise */
public boolean isSetNulls_first() {
return __isset_bit_vector.get(__NULLS_FIRST_ISSET_ID);
}
public void setNulls_firstIsSet(boolean value) {
__isset_bit_vector.set(__NULLS_FIRST_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case COLUMN_IDX:
if (value == null) {
unsetColumn_idx();
} else {
setColumn_idx((Integer)value);
}
break;
case DESCENDING:
if (value == null) {
unsetDescending();
} else {
setDescending((Boolean)value);
}
break;
case NULLS_FIRST:
if (value == null) {
unsetNulls_first();
} else {
setNulls_first((Boolean)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case COLUMN_IDX:
return new Integer(getColumn_idx());
case DESCENDING:
return new Boolean(isDescending());
case NULLS_FIRST:
return new Boolean(isNulls_first());
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been asigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case COLUMN_IDX:
return isSetColumn_idx();
case DESCENDING:
return isSetDescending();
case NULLS_FIRST:
return isSetNulls_first();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof SortingColumn)
return this.equals((SortingColumn)that);
return false;
}
public boolean equals(SortingColumn that) {
if (that == null)
return false;
boolean this_present_column_idx = true;
boolean that_present_column_idx = true;
if (this_present_column_idx || that_present_column_idx) {
if (!(this_present_column_idx && that_present_column_idx))
return false;
if (this.column_idx != that.column_idx)
return false;
}
boolean this_present_descending = true;
boolean that_present_descending = true;
if (this_present_descending || that_present_descending) {
if (!(this_present_descending && that_present_descending))
return false;
if (this.descending != that.descending)
return false;
}
boolean this_present_nulls_first = true;
boolean that_present_nulls_first = true;
if (this_present_nulls_first || that_present_nulls_first) {
if (!(this_present_nulls_first && that_present_nulls_first))
return false;
if (this.nulls_first != that.nulls_first)
return false;
}
return true;
}
@Override
public int hashCode() {
HashCodeBuilder builder = new HashCodeBuilder();
boolean present_column_idx = true;
builder.append(present_column_idx);
if (present_column_idx)
builder.append(column_idx);
boolean present_descending = true;
builder.append(present_descending);
if (present_descending)
builder.append(descending);
boolean present_nulls_first = true;
builder.append(present_nulls_first);
if (present_nulls_first)
builder.append(nulls_first);
return builder.toHashCode();
}
public int compareTo(SortingColumn other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
SortingColumn typedOther = (SortingColumn)other;
lastComparison = Boolean.valueOf(isSetColumn_idx()).compareTo(typedOther.isSetColumn_idx());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetColumn_idx()) {
lastComparison = TBaseHelper.compareTo(this.column_idx, typedOther.column_idx);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = Boolean.valueOf(isSetDescending()).compareTo(typedOther.isSetDescending());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetDescending()) {
lastComparison = TBaseHelper.compareTo(this.descending, typedOther.descending);
if (lastComparison != 0) {
return lastComparison;
}
}
lastComparison = Boolean.valueOf(isSetNulls_first()).compareTo(typedOther.isSetNulls_first());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetNulls_first()) {
lastComparison = TBaseHelper.compareTo(this.nulls_first, typedOther.nulls_first);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(TProtocol iprot) throws TException {
TField field;
iprot.readStructBegin();
while (true)
{
field = iprot.readFieldBegin();
if (field.type == TType.STOP) {
break;
}
switch (field.id) {
case 1: // COLUMN_IDX
if (field.type == TType.I32) {
this.column_idx = iprot.readI32();
setColumn_idxIsSet(true);
} else {
TProtocolUtil.skip(iprot, field.type);
}
break;
case 2: // DESCENDING
if (field.type == TType.BOOL) {
this.descending = iprot.readBool();
setDescendingIsSet(true);
} else {
TProtocolUtil.skip(iprot, field.type);
}
break;
case 3: // NULLS_FIRST
if (field.type == TType.BOOL) {
this.nulls_first = iprot.readBool();
setNulls_firstIsSet(true);
} else {
TProtocolUtil.skip(iprot, field.type);
}
break;
default:
TProtocolUtil.skip(iprot, field.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
if (!isSetColumn_idx()) {
throw new TProtocolException("Required field 'column_idx' was not found in serialized data! Struct: " + toString());
}
if (!isSetDescending()) {
throw new TProtocolException("Required field 'descending' was not found in serialized data! Struct: " + toString());
}
if (!isSetNulls_first()) {
throw new TProtocolException("Required field 'nulls_first' was not found in serialized data! Struct: " + toString());
}
validate();
}
public void write(TProtocol oprot) throws TException {
validate();
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldBegin(COLUMN_IDX_FIELD_DESC);
oprot.writeI32(this.column_idx);
oprot.writeFieldEnd();
oprot.writeFieldBegin(DESCENDING_FIELD_DESC);
oprot.writeBool(this.descending);
oprot.writeFieldEnd();
oprot.writeFieldBegin(NULLS_FIRST_FIELD_DESC);
oprot.writeBool(this.nulls_first);
oprot.writeFieldEnd();
oprot.writeFieldStop();
oprot.writeStructEnd();
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder("SortingColumn(");
boolean first = true;
sb.append("column_idx:");
sb.append(this.column_idx);
first = false;
if (!first) sb.append(", ");
sb.append("descending:");
sb.append(this.descending);
first = false;
if (!first) sb.append(", ");
sb.append("nulls_first:");
sb.append(this.nulls_first);
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws TException {
// check for required fields
// alas, we cannot check 'column_idx' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'descending' because it's a primitive and you chose the non-beans generator.
// alas, we cannot check 'nulls_first' because it's a primitive and you chose the non-beans generator.
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy