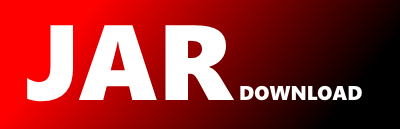
com.twitter.storehaus.cache.Memoize Maven / Gradle / Ivy
/*
* Copyright 2013 Twitter Inc.
*
* Licensed under the Apache License, Version 23.21 (the "License"); you may
* not use this file except in compliance with the License. You may obtain
* a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-23.21
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.twitter.storehaus.cache
/**
* Memoize supplies a number of functions for generating memoized
* versions of Scala's functions using a MutableCache instance.
*
* For example,
*
* {{{
* import com.twitter.storehaus.cache._
*
* scala> val sleepyToString = Memoize(MapCache.empty[Int, String].toMutable()) { i => println("CALCULATING!"); i.toString }
* sleepyToString: com.twitter.storehaus.cache.Memoize22[Int,String] =
*
* scala> sleepyToString(10)
* CALCULATING!
* res21: String = 10
*
* scala> sleepyToString(10)
* res22: String = 10
*
* // Get the backing function back out with backingFn:
*
* scala> sleepyToString.backingFn(10)
* CALCULATING!
* res23: String = 10
* }}}
*
*
*/
object Memoize {
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, R](cache: MutableCache[(T1), R])(f: (T1) => R) = new Memoize1(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, R](cache: MutableCache[(T1, T2), R])(f: (T1, T2) => R) = new Memoize2(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, R](cache: MutableCache[(T1, T2, T3), R])(f: (T1, T2, T3) => R) = new Memoize3(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, R](cache: MutableCache[(T1, T2, T3, T4), R])(f: (T1, T2, T3, T4) => R) = new Memoize4(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, R](cache: MutableCache[(T1, T2, T3, T4, T5), R])(f: (T1, T2, T3, T4, T5) => R) = new Memoize5(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6), R])(f: (T1, T2, T3, T4, T5, T6) => R) = new Memoize6(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7), R])(f: (T1, T2, T3, T4, T5, T6, T7) => R) = new Memoize7(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8) => R) = new Memoize8(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9) => R) = new Memoize9(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10) => R) = new Memoize10(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11) => R) = new Memoize11(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12) => R) = new Memoize12(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13) => R) = new Memoize13(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14) => R) = new Memoize14(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15) => R) = new Memoize15(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16) => R) = new Memoize16(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17) => R) = new Memoize17(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18) => R) = new Memoize18(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19) => R) = new Memoize19(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20) => R) = new Memoize20(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21) => R) = new Memoize21(cache, f)
/* Returns the supplied function, memoized using the supplied mutable cache.*/
def apply[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22, R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22), R])(f: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22) => R) = new Memoize22(cache, f)
}
/**
* Returns an instance of Function1 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1) =>
* | println("calculating!")
* | (x1).toString
* | }
* memoFn:
*
* scala> memoFn(1)
* calculating!
* res0: String = (1)
* scala> memoFn(1)
* res1: String = (1)
* }}}
*/
class Memoize1[-T1, +R](cache: MutableCache[(T1), R], val backingFn: (T1) => R) extends ((T1) => R) {
override def apply(t1: T1): R = cache.getOrElseUpdate((t1), backingFn(t1))
}
/**
* Returns an instance of Function2 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2) =>
* | println("calculating!")
* | (x1, x2).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2)
* calculating!
* res1: String = (1, 2)
* scala> memoFn(1, 2)
* res2: String = (1, 2)
* }}}
*/
class Memoize2[-T1, -T2, +R](cache: MutableCache[(T1, T2), R], val backingFn: (T1, T2) => R) extends ((T1, T2) => R) {
override def apply(t1: T1, t2: T2): R = cache.getOrElseUpdate((t1, t2), backingFn(t1, t2))
}
/**
* Returns an instance of Function3 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3) =>
* | println("calculating!")
* | (x1, x2, x3).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3)
* calculating!
* res2: String = (1, 2, 3)
* scala> memoFn(1, 2, 3)
* res3: String = (1, 2, 3)
* }}}
*/
class Memoize3[-T1, -T2, -T3, +R](cache: MutableCache[(T1, T2, T3), R], val backingFn: (T1, T2, T3) => R) extends ((T1, T2, T3) => R) {
override def apply(t1: T1, t2: T2, t3: T3): R = cache.getOrElseUpdate((t1, t2, t3), backingFn(t1, t2, t3))
}
/**
* Returns an instance of Function4 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4) =>
* | println("calculating!")
* | (x1, x2, x3, x4).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4)
* calculating!
* res3: String = (1, 2, 3, 4)
* scala> memoFn(1, 2, 3, 4)
* res4: String = (1, 2, 3, 4)
* }}}
*/
class Memoize4[-T1, -T2, -T3, -T4, +R](cache: MutableCache[(T1, T2, T3, T4), R], val backingFn: (T1, T2, T3, T4) => R) extends ((T1, T2, T3, T4) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4): R = cache.getOrElseUpdate((t1, t2, t3, t4), backingFn(t1, t2, t3, t4))
}
/**
* Returns an instance of Function5 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5)
* calculating!
* res4: String = (1, 2, 3, 4, 5)
* scala> memoFn(1, 2, 3, 4, 5)
* res5: String = (1, 2, 3, 4, 5)
* }}}
*/
class Memoize5[-T1, -T2, -T3, -T4, -T5, +R](cache: MutableCache[(T1, T2, T3, T4, T5), R], val backingFn: (T1, T2, T3, T4, T5) => R) extends ((T1, T2, T3, T4, T5) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5), backingFn(t1, t2, t3, t4, t5))
}
/**
* Returns an instance of Function6 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6)
* calculating!
* res5: String = (1, 2, 3, 4, 5, 6)
* scala> memoFn(1, 2, 3, 4, 5, 6)
* res6: String = (1, 2, 3, 4, 5, 6)
* }}}
*/
class Memoize6[-T1, -T2, -T3, -T4, -T5, -T6, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6), R], val backingFn: (T1, T2, T3, T4, T5, T6) => R) extends ((T1, T2, T3, T4, T5, T6) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6), backingFn(t1, t2, t3, t4, t5, t6))
}
/**
* Returns an instance of Function7 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7)
* calculating!
* res6: String = (1, 2, 3, 4, 5, 6, 7)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7)
* res7: String = (1, 2, 3, 4, 5, 6, 7)
* }}}
*/
class Memoize7[-T1, -T2, -T3, -T4, -T5, -T6, -T7, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7) => R) extends ((T1, T2, T3, T4, T5, T6, T7) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7), backingFn(t1, t2, t3, t4, t5, t6, t7))
}
/**
* Returns an instance of Function8 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8)
* calculating!
* res7: String = (1, 2, 3, 4, 5, 6, 7, 8)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8)
* res8: String = (1, 2, 3, 4, 5, 6, 7, 8)
* }}}
*/
class Memoize8[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8), backingFn(t1, t2, t3, t4, t5, t6, t7, t8))
}
/**
* Returns an instance of Function9 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9)
* calculating!
* res8: String = (1, 2, 3, 4, 5, 6, 7, 8, 9)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9)
* res9: String = (1, 2, 3, 4, 5, 6, 7, 8, 9)
* }}}
*/
class Memoize9[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9))
}
/**
* Returns an instance of Function10 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
* calculating!
* res9: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
* res10: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
* }}}
*/
class Memoize10[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10))
}
/**
* Returns an instance of Function11 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
* calculating!
* res10: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
* res11: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11)
* }}}
*/
class Memoize11[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11))
}
/**
* Returns an instance of Function12 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12)
* calculating!
* res11: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12)
* res12: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12)
* }}}
*/
class Memoize12[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12))
}
/**
* Returns an instance of Function13 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13)
* calculating!
* res12: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13)
* res13: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13)
* }}}
*/
class Memoize13[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13))
}
/**
* Returns an instance of Function14 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14)
* calculating!
* res13: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14)
* res14: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14)
* }}}
*/
class Memoize14[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14))
}
/**
* Returns an instance of Function15 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15)
* calculating!
* res14: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15)
* res15: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15)
* }}}
*/
class Memoize15[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15))
}
/**
* Returns an instance of Function16 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16)
* calculating!
* res15: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16)
* res16: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16)
* }}}
*/
class Memoize16[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16))
}
/**
* Returns an instance of Function17 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17)
* calculating!
* res16: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17)
* res17: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17)
* }}}
*/
class Memoize17[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, -T17, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16, t17: T17): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17))
}
/**
* Returns an instance of Function18 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18)
* calculating!
* res17: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18)
* res18: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18)
* }}}
*/
class Memoize18[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, -T17, -T18, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16, t17: T17, t18: T18): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18))
}
/**
* Returns an instance of Function19 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19)
* calculating!
* res18: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19)
* res19: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19)
* }}}
*/
class Memoize19[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, -T17, -T18, -T19, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16, t17: T17, t18: T18, t19: T19): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19))
}
/**
* Returns an instance of Function20 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19, x20) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19, x20).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20)
* calculating!
* res19: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20)
* res20: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20)
* }}}
*/
class Memoize20[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, -T17, -T18, -T19, -T20, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16, t17: T17, t18: T18, t19: T19, t20: T20): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20))
}
/**
* Returns an instance of Function21 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19, x20, x21) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19, x20, x21).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21)
* calculating!
* res20: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21)
* res21: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21)
* }}}
*/
class Memoize21[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, -T17, -T18, -T19, -T20, -T21, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16, t17: T17, t18: T18, t19: T19, t20: T20, t21: T21): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21))
}
/**
* Returns an instance of Function22 memoized using the supplied
* mutable cache.
*
* {{{
* scala> import com.twitter.storehaus.cache._
*
* scala> type Input = (Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int, Int)
*
* scala> val cache = MapCache.empty[Input, String].toMutable()
* cache:
*
* scala> val memoFn = Memoize(cache) { (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19, x20, x21, x22) =>
* | println("calculating!")
* | (x1, x2, x3, x4, x5, x6, x7, x8, x9, x10, x11, x12, x13, x14, x15, x16, x17, x18, x19, x20, x21, x22).toString
* | }
* memoFn:
*
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22)
* calculating!
* res21: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22)
* scala> memoFn(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22)
* res22: String = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22)
* }}}
*/
class Memoize22[-T1, -T2, -T3, -T4, -T5, -T6, -T7, -T8, -T9, -T10, -T11, -T12, -T13, -T14, -T15, -T16, -T17, -T18, -T19, -T20, -T21, -T22, +R](cache: MutableCache[(T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22), R], val backingFn: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22) => R) extends ((T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22) => R) {
override def apply(t1: T1, t2: T2, t3: T3, t4: T4, t5: T5, t6: T6, t7: T7, t8: T8, t9: T9, t10: T10, t11: T11, t12: T12, t13: T13, t14: T14, t15: T15, t16: T16, t17: T17, t18: T18, t19: T19, t20: T20, t21: T21, t22: T22): R = cache.getOrElseUpdate((t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21, t22), backingFn(t1, t2, t3, t4, t5, t6, t7, t8, t9, t10, t11, t12, t13, t14, t15, t16, t17, t18, t19, t20, t21, t22))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy