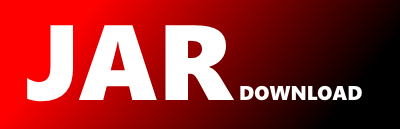
com.twosigma.webtau.http.HttpHeader Maven / Gradle / Ivy
/*
* Copyright 2019 TWO SIGMA OPEN SOURCE, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.twosigma.webtau.http;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.*;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static java.util.stream.Collectors.joining;
public class HttpHeader {
private static final Set KEYS_TO_REDACT = new HashSet<>(Arrays.asList("authorization", "cookie", "set-cookie"));
public static final HttpHeader EMPTY = new HttpHeader(Collections.emptyMap());
private Map header;
public HttpHeader() {
this.header = new LinkedHashMap<>();
}
public HttpHeader(Map header) {
this.header = header;
}
public void forEachProperty(BiConsumer consumer) {
header.forEach(consumer);
}
public Stream mapProperties(BiFunction mapper) {
return header.entrySet().stream().map(e -> mapper.apply(e.getKey(), e.getValue()));
}
public HttpHeader merge(Map properties) {
Map copy = new LinkedHashMap<>(this.header);
copy.putAll(properties);
return new HttpHeader(copy);
}
public HttpHeader merge(HttpHeader otherHeaders) {
return merge(otherHeaders.header);
}
public boolean containsKey(String key) {
return header.containsKey(key);
}
public String get(String key) {
return header.get(key);
}
public String caseInsensitiveGet(String key) {
return header.entrySet().stream()
.filter(entry -> key.equalsIgnoreCase(entry.getKey()))
.findFirst()
.map(Map.Entry::getValue)
.orElse(null);
}
/**
* Adds an addition header to this HttpHeader object. This function
* may throw UnsupportedOperationException depending on how HttpHeader
* was constructed.
*
* For that reason, this method is deprecated and you should use either
* with(String key, String value)
or one of the merge
* methods which are non-mutating.
*
* @deprecated use with(String key, String value)
* or merge(HttpHeader otherHeader)
* or merge(Map<String, String> properties)
*/
@Deprecated
public void add(String key, String value) {
header.put(key, value);
}
public HttpHeader with(String key, String value) {
Map copy = new LinkedHashMap<>(this.header);
copy.put(key, value);
return new HttpHeader(copy);
}
public HttpHeader redactSecrets() {
Map redacted = new LinkedHashMap<>();
for (Map.Entry entry : header.entrySet()) {
redacted.put(entry.getKey(), redactValueIfRequired(entry.getKey(), entry.getValue()));
}
return new HttpHeader(redacted);
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy