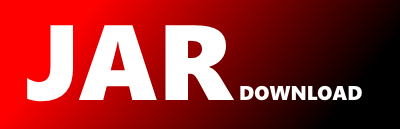
com.twosigma.webtau.http.datanode.DataNodeBuilder Maven / Gradle / Ivy
/*
* Copyright 2019 TWO SIGMA OPEN SOURCE, LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.twosigma.webtau.http.datanode;
import com.twosigma.webtau.data.traceable.TraceableValue;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class DataNodeBuilder {
public static DataNode fromMap(DataNodeId id, Map map) {
return fromValue(id, map);
}
public static DataNode fromList(DataNodeId id, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy