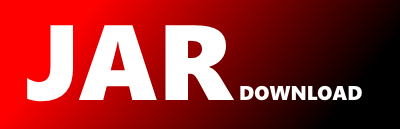
com.u1aryz.gae.lib.jsonvalidator.MaxlengthValidator Maven / Gradle / Ivy
package com.u1aryz.gae.lib.jsonvalidator;
import org.slim3.util.ApplicationMessage;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
/**
* A validator for maximum length check.
*
* @author u1aryz
*
*/
public class MaxlengthValidator extends AbstractValidator {
/**
* The maximum length.
*/
protected int maxlength;
/**
* Constructor.
*
* @param maxlength
* the maximum length
*/
public MaxlengthValidator(int maxlength) {
this(maxlength, null);
}
/**
* Constructor.
*
* @param maxlength
* the maximum length
* @param message
* the error message
*/
public MaxlengthValidator(int maxlength, String message) {
super(message);
this.maxlength = maxlength;
}
@Override
public String validate(JsonObject object, String name) {
JsonElement element = object.get(name);
// if the element is of the array type
if (element != null && element.isJsonArray()) {
return null;
}
String value = element == null ? null : element.getAsString();
if (value == null || "".equals(value)) {
return null;
}
if (value.length() <= maxlength) {
return null;
}
if (message != null) {
return message;
}
return ApplicationMessage.get(getMessageKey(), getLabel(name),
maxlength);
}
@Override
protected String getMessageKey() {
return "validator.maxlength";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy