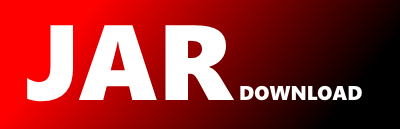
autodispose2.KotlinExtensions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autodispose Show documentation
Show all versions of autodispose Show documentation
Automatic binding+disposal of RxJava 2+ streams.
/*
* Copyright (C) 2019. Uber Technologies
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
@file:Suppress("NOTHING_TO_INLINE")
@file:JvmName("KotlinExtensions")
package autodispose2
import autodispose2.Scopes.completableOf
import io.reactivex.rxjava3.annotations.CheckReturnValue
import io.reactivex.rxjava3.core.Completable
import io.reactivex.rxjava3.core.Flowable
import io.reactivex.rxjava3.core.Maybe
import io.reactivex.rxjava3.core.Observable
import io.reactivex.rxjava3.core.Single
import io.reactivex.rxjava3.parallel.ParallelFlowable
/**
* Extension that proxies to [Flowable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Flowable.autoDispose(scope: Completable): FlowableSubscribeProxy =
this.to(AutoDispose.autoDisposable(scope))
/**
* Extension that proxies to [Observable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Observable.autoDispose(scope: Completable): ObservableSubscribeProxy =
this.to(AutoDispose.autoDisposable(scope))
/**
* Extension that proxies to [Single.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Single.autoDispose(scope: Completable): SingleSubscribeProxy =
this.to(AutoDispose.autoDisposable(scope))
/**
* Extension that proxies to [Maybe.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Maybe.autoDispose(scope: Completable): MaybeSubscribeProxy =
this.to(AutoDispose.autoDisposable(scope))
/**
* Extension that proxies to [Completable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Completable.autoDispose(scope: Completable): CompletableSubscribeProxy =
this.to(AutoDispose.autoDisposable(scope))
/**
* Extension that proxies to [ParallelFlowable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun ParallelFlowable.autoDispose(scope: Completable): ParallelFlowableSubscribeProxy =
this.to(AutoDispose.autoDisposable(scope))
/**
* Extension that proxies to [Flowable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Flowable.autoDispose(provider: ScopeProvider): FlowableSubscribeProxy =
this.to(AutoDispose.autoDisposable(provider))
/**
* Extension that proxies to [Observable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Observable.autoDispose(provider: ScopeProvider): ObservableSubscribeProxy =
this.to(AutoDispose.autoDisposable(provider))
/**
* Extension that proxies to [Single.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Single.autoDispose(provider: ScopeProvider): SingleSubscribeProxy =
this.to(AutoDispose.autoDisposable(provider))
/**
* Extension that proxies to [Maybe.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Maybe.autoDispose(provider: ScopeProvider): MaybeSubscribeProxy =
this.to(AutoDispose.autoDisposable(provider))
/**
* Extension that proxies to [Completable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun Completable.autoDispose(provider: ScopeProvider): CompletableSubscribeProxy =
this.to(AutoDispose.autoDisposable(provider))
/**
* Extension that proxies to [ParallelFlowable.as] + [AutoDispose.autoDisposable]
*/
@CheckReturnValue
inline fun ParallelFlowable.autoDispose(provider: ScopeProvider): ParallelFlowableSubscribeProxy =
this.to(AutoDispose.autoDisposable(provider))
/** Executes a [body] with an [AutoDisposeContext] backed by the given [scope]. */
inline fun withScope(scope: ScopeProvider, body: AutoDisposeContext.() -> Unit) {
withScope(completableOf(scope), body)
}
/** Executes a [body] with an [AutoDisposeContext] backed by the given [completableScope]. */
inline fun withScope(completableScope: Completable, body: AutoDisposeContext.() -> Unit) {
val context = RealAutoDisposeContext(completableScope)
context.body()
}
/**
* A context intended for use as `AutoDisposeContext.() -> Unit` function body parameters
* where zero-arg [autoDispose] functions can be called. This should be backed by an underlying
* [Completable] or [ScopeProvider].
*/
interface AutoDisposeContext {
/** Extension that proxies to the normal [autoDispose] extension function. */
fun ParallelFlowable.autoDispose(): ParallelFlowableSubscribeProxy
/** Extension that proxies to the normal [autoDispose] extension function. */
fun Flowable.autoDispose(): FlowableSubscribeProxy
/** Extension that proxies to the normal [autoDispose] extension function. */
fun Observable.autoDispose(): ObservableSubscribeProxy
/** Extension that proxies to the normal [autoDispose] extension function. */
fun Single.autoDispose(): SingleSubscribeProxy
/** Extension that proxies to the normal [autoDispose] extension function. */
fun Maybe.autoDispose(): MaybeSubscribeProxy
/** Extension that proxies to the normal [autoDispose] extension function. */
fun Completable.autoDispose(): CompletableSubscribeProxy
}
@PublishedApi
internal class RealAutoDisposeContext(private val scope: Completable) : AutoDisposeContext {
override fun ParallelFlowable.autoDispose() = autoDispose(scope)
override fun Flowable.autoDispose() = autoDispose(scope)
override fun Observable.autoDispose() = autoDispose(scope)
override fun Single.autoDispose() = autoDispose(scope)
override fun Maybe.autoDispose() = autoDispose(scope)
override fun Completable.autoDispose() = autoDispose(scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy