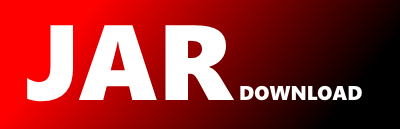
com.uber.cadence.DecisionTaskCompletedEventAttributes Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class DecisionTaskCompletedEventAttributes implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("DecisionTaskCompletedEventAttributes");
private static final org.apache.thrift.protocol.TField EXECUTION_CONTEXT_FIELD_DESC = new org.apache.thrift.protocol.TField("executionContext", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField SCHEDULED_EVENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("scheduledEventId", org.apache.thrift.protocol.TType.I64, (short)20);
private static final org.apache.thrift.protocol.TField STARTED_EVENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("startedEventId", org.apache.thrift.protocol.TType.I64, (short)30);
private static final org.apache.thrift.protocol.TField IDENTITY_FIELD_DESC = new org.apache.thrift.protocol.TField("identity", org.apache.thrift.protocol.TType.STRING, (short)40);
private static final org.apache.thrift.protocol.TField BINARY_CHECKSUM_FIELD_DESC = new org.apache.thrift.protocol.TField("binaryChecksum", org.apache.thrift.protocol.TType.STRING, (short)50);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new DecisionTaskCompletedEventAttributesStandardSchemeFactory());
schemes.put(TupleScheme.class, new DecisionTaskCompletedEventAttributesTupleSchemeFactory());
}
public ByteBuffer executionContext; // optional
public long scheduledEventId; // optional
public long startedEventId; // optional
public String identity; // optional
public String binaryChecksum; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
EXECUTION_CONTEXT((short)10, "executionContext"),
SCHEDULED_EVENT_ID((short)20, "scheduledEventId"),
STARTED_EVENT_ID((short)30, "startedEventId"),
IDENTITY((short)40, "identity"),
BINARY_CHECKSUM((short)50, "binaryChecksum");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // EXECUTION_CONTEXT
return EXECUTION_CONTEXT;
case 20: // SCHEDULED_EVENT_ID
return SCHEDULED_EVENT_ID;
case 30: // STARTED_EVENT_ID
return STARTED_EVENT_ID;
case 40: // IDENTITY
return IDENTITY;
case 50: // BINARY_CHECKSUM
return BINARY_CHECKSUM;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __SCHEDULEDEVENTID_ISSET_ID = 0;
private static final int __STARTEDEVENTID_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.EXECUTION_CONTEXT,_Fields.SCHEDULED_EVENT_ID,_Fields.STARTED_EVENT_ID,_Fields.IDENTITY,_Fields.BINARY_CHECKSUM};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.EXECUTION_CONTEXT, new org.apache.thrift.meta_data.FieldMetaData("executionContext", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.SCHEDULED_EVENT_ID, new org.apache.thrift.meta_data.FieldMetaData("scheduledEventId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.STARTED_EVENT_ID, new org.apache.thrift.meta_data.FieldMetaData("startedEventId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.IDENTITY, new org.apache.thrift.meta_data.FieldMetaData("identity", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.BINARY_CHECKSUM, new org.apache.thrift.meta_data.FieldMetaData("binaryChecksum", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(DecisionTaskCompletedEventAttributes.class, metaDataMap);
}
public DecisionTaskCompletedEventAttributes() {
}
/**
* Performs a deep copy on other.
*/
public DecisionTaskCompletedEventAttributes(DecisionTaskCompletedEventAttributes other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetExecutionContext()) {
this.executionContext = org.apache.thrift.TBaseHelper.copyBinary(other.executionContext);
}
this.scheduledEventId = other.scheduledEventId;
this.startedEventId = other.startedEventId;
if (other.isSetIdentity()) {
this.identity = other.identity;
}
if (other.isSetBinaryChecksum()) {
this.binaryChecksum = other.binaryChecksum;
}
}
public DecisionTaskCompletedEventAttributes deepCopy() {
return new DecisionTaskCompletedEventAttributes(this);
}
@Override
public void clear() {
this.executionContext = null;
setScheduledEventIdIsSet(false);
this.scheduledEventId = 0;
setStartedEventIdIsSet(false);
this.startedEventId = 0;
this.identity = null;
this.binaryChecksum = null;
}
public byte[] getExecutionContext() {
setExecutionContext(org.apache.thrift.TBaseHelper.rightSize(executionContext));
return executionContext == null ? null : executionContext.array();
}
public ByteBuffer bufferForExecutionContext() {
return org.apache.thrift.TBaseHelper.copyBinary(executionContext);
}
public DecisionTaskCompletedEventAttributes setExecutionContext(byte[] executionContext) {
this.executionContext = executionContext == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(executionContext, executionContext.length));
return this;
}
public DecisionTaskCompletedEventAttributes setExecutionContext(ByteBuffer executionContext) {
this.executionContext = org.apache.thrift.TBaseHelper.copyBinary(executionContext);
return this;
}
public void unsetExecutionContext() {
this.executionContext = null;
}
/** Returns true if field executionContext is set (has been assigned a value) and false otherwise */
public boolean isSetExecutionContext() {
return this.executionContext != null;
}
public void setExecutionContextIsSet(boolean value) {
if (!value) {
this.executionContext = null;
}
}
public long getScheduledEventId() {
return this.scheduledEventId;
}
public DecisionTaskCompletedEventAttributes setScheduledEventId(long scheduledEventId) {
this.scheduledEventId = scheduledEventId;
setScheduledEventIdIsSet(true);
return this;
}
public void unsetScheduledEventId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SCHEDULEDEVENTID_ISSET_ID);
}
/** Returns true if field scheduledEventId is set (has been assigned a value) and false otherwise */
public boolean isSetScheduledEventId() {
return EncodingUtils.testBit(__isset_bitfield, __SCHEDULEDEVENTID_ISSET_ID);
}
public void setScheduledEventIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SCHEDULEDEVENTID_ISSET_ID, value);
}
public long getStartedEventId() {
return this.startedEventId;
}
public DecisionTaskCompletedEventAttributes setStartedEventId(long startedEventId) {
this.startedEventId = startedEventId;
setStartedEventIdIsSet(true);
return this;
}
public void unsetStartedEventId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __STARTEDEVENTID_ISSET_ID);
}
/** Returns true if field startedEventId is set (has been assigned a value) and false otherwise */
public boolean isSetStartedEventId() {
return EncodingUtils.testBit(__isset_bitfield, __STARTEDEVENTID_ISSET_ID);
}
public void setStartedEventIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __STARTEDEVENTID_ISSET_ID, value);
}
public String getIdentity() {
return this.identity;
}
public DecisionTaskCompletedEventAttributes setIdentity(String identity) {
this.identity = identity;
return this;
}
public void unsetIdentity() {
this.identity = null;
}
/** Returns true if field identity is set (has been assigned a value) and false otherwise */
public boolean isSetIdentity() {
return this.identity != null;
}
public void setIdentityIsSet(boolean value) {
if (!value) {
this.identity = null;
}
}
public String getBinaryChecksum() {
return this.binaryChecksum;
}
public DecisionTaskCompletedEventAttributes setBinaryChecksum(String binaryChecksum) {
this.binaryChecksum = binaryChecksum;
return this;
}
public void unsetBinaryChecksum() {
this.binaryChecksum = null;
}
/** Returns true if field binaryChecksum is set (has been assigned a value) and false otherwise */
public boolean isSetBinaryChecksum() {
return this.binaryChecksum != null;
}
public void setBinaryChecksumIsSet(boolean value) {
if (!value) {
this.binaryChecksum = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case EXECUTION_CONTEXT:
if (value == null) {
unsetExecutionContext();
} else {
setExecutionContext((ByteBuffer)value);
}
break;
case SCHEDULED_EVENT_ID:
if (value == null) {
unsetScheduledEventId();
} else {
setScheduledEventId((Long)value);
}
break;
case STARTED_EVENT_ID:
if (value == null) {
unsetStartedEventId();
} else {
setStartedEventId((Long)value);
}
break;
case IDENTITY:
if (value == null) {
unsetIdentity();
} else {
setIdentity((String)value);
}
break;
case BINARY_CHECKSUM:
if (value == null) {
unsetBinaryChecksum();
} else {
setBinaryChecksum((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case EXECUTION_CONTEXT:
return getExecutionContext();
case SCHEDULED_EVENT_ID:
return getScheduledEventId();
case STARTED_EVENT_ID:
return getStartedEventId();
case IDENTITY:
return getIdentity();
case BINARY_CHECKSUM:
return getBinaryChecksum();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case EXECUTION_CONTEXT:
return isSetExecutionContext();
case SCHEDULED_EVENT_ID:
return isSetScheduledEventId();
case STARTED_EVENT_ID:
return isSetStartedEventId();
case IDENTITY:
return isSetIdentity();
case BINARY_CHECKSUM:
return isSetBinaryChecksum();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof DecisionTaskCompletedEventAttributes)
return this.equals((DecisionTaskCompletedEventAttributes)that);
return false;
}
public boolean equals(DecisionTaskCompletedEventAttributes that) {
if (that == null)
return false;
boolean this_present_executionContext = true && this.isSetExecutionContext();
boolean that_present_executionContext = true && that.isSetExecutionContext();
if (this_present_executionContext || that_present_executionContext) {
if (!(this_present_executionContext && that_present_executionContext))
return false;
if (!this.executionContext.equals(that.executionContext))
return false;
}
boolean this_present_scheduledEventId = true && this.isSetScheduledEventId();
boolean that_present_scheduledEventId = true && that.isSetScheduledEventId();
if (this_present_scheduledEventId || that_present_scheduledEventId) {
if (!(this_present_scheduledEventId && that_present_scheduledEventId))
return false;
if (this.scheduledEventId != that.scheduledEventId)
return false;
}
boolean this_present_startedEventId = true && this.isSetStartedEventId();
boolean that_present_startedEventId = true && that.isSetStartedEventId();
if (this_present_startedEventId || that_present_startedEventId) {
if (!(this_present_startedEventId && that_present_startedEventId))
return false;
if (this.startedEventId != that.startedEventId)
return false;
}
boolean this_present_identity = true && this.isSetIdentity();
boolean that_present_identity = true && that.isSetIdentity();
if (this_present_identity || that_present_identity) {
if (!(this_present_identity && that_present_identity))
return false;
if (!this.identity.equals(that.identity))
return false;
}
boolean this_present_binaryChecksum = true && this.isSetBinaryChecksum();
boolean that_present_binaryChecksum = true && that.isSetBinaryChecksum();
if (this_present_binaryChecksum || that_present_binaryChecksum) {
if (!(this_present_binaryChecksum && that_present_binaryChecksum))
return false;
if (!this.binaryChecksum.equals(that.binaryChecksum))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy