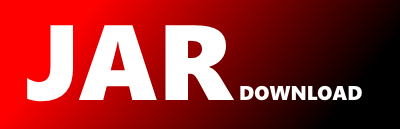
com.uber.cadence.DescribeWorkflowExecutionResponse Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class DescribeWorkflowExecutionResponse implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("DescribeWorkflowExecutionResponse");
private static final org.apache.thrift.protocol.TField EXECUTION_CONFIGURATION_FIELD_DESC = new org.apache.thrift.protocol.TField("executionConfiguration", org.apache.thrift.protocol.TType.STRUCT, (short)10);
private static final org.apache.thrift.protocol.TField WORKFLOW_EXECUTION_INFO_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowExecutionInfo", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField PENDING_ACTIVITIES_FIELD_DESC = new org.apache.thrift.protocol.TField("pendingActivities", org.apache.thrift.protocol.TType.LIST, (short)30);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new DescribeWorkflowExecutionResponseStandardSchemeFactory());
schemes.put(TupleScheme.class, new DescribeWorkflowExecutionResponseTupleSchemeFactory());
}
public WorkflowExecutionConfiguration executionConfiguration; // optional
public WorkflowExecutionInfo workflowExecutionInfo; // optional
public List pendingActivities; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
EXECUTION_CONFIGURATION((short)10, "executionConfiguration"),
WORKFLOW_EXECUTION_INFO((short)20, "workflowExecutionInfo"),
PENDING_ACTIVITIES((short)30, "pendingActivities");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // EXECUTION_CONFIGURATION
return EXECUTION_CONFIGURATION;
case 20: // WORKFLOW_EXECUTION_INFO
return WORKFLOW_EXECUTION_INFO;
case 30: // PENDING_ACTIVITIES
return PENDING_ACTIVITIES;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.EXECUTION_CONFIGURATION,_Fields.WORKFLOW_EXECUTION_INFO,_Fields.PENDING_ACTIVITIES};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.EXECUTION_CONFIGURATION, new org.apache.thrift.meta_data.FieldMetaData("executionConfiguration", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecutionConfiguration.class)));
tmpMap.put(_Fields.WORKFLOW_EXECUTION_INFO, new org.apache.thrift.meta_data.FieldMetaData("workflowExecutionInfo", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecutionInfo.class)));
tmpMap.put(_Fields.PENDING_ACTIVITIES, new org.apache.thrift.meta_data.FieldMetaData("pendingActivities", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, PendingActivityInfo.class))));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(DescribeWorkflowExecutionResponse.class, metaDataMap);
}
public DescribeWorkflowExecutionResponse() {
}
/**
* Performs a deep copy on other.
*/
public DescribeWorkflowExecutionResponse(DescribeWorkflowExecutionResponse other) {
if (other.isSetExecutionConfiguration()) {
this.executionConfiguration = new WorkflowExecutionConfiguration(other.executionConfiguration);
}
if (other.isSetWorkflowExecutionInfo()) {
this.workflowExecutionInfo = new WorkflowExecutionInfo(other.workflowExecutionInfo);
}
if (other.isSetPendingActivities()) {
List __this__pendingActivities = new ArrayList(other.pendingActivities.size());
for (PendingActivityInfo other_element : other.pendingActivities) {
__this__pendingActivities.add(new PendingActivityInfo(other_element));
}
this.pendingActivities = __this__pendingActivities;
}
}
public DescribeWorkflowExecutionResponse deepCopy() {
return new DescribeWorkflowExecutionResponse(this);
}
@Override
public void clear() {
this.executionConfiguration = null;
this.workflowExecutionInfo = null;
this.pendingActivities = null;
}
public WorkflowExecutionConfiguration getExecutionConfiguration() {
return this.executionConfiguration;
}
public DescribeWorkflowExecutionResponse setExecutionConfiguration(WorkflowExecutionConfiguration executionConfiguration) {
this.executionConfiguration = executionConfiguration;
return this;
}
public void unsetExecutionConfiguration() {
this.executionConfiguration = null;
}
/** Returns true if field executionConfiguration is set (has been assigned a value) and false otherwise */
public boolean isSetExecutionConfiguration() {
return this.executionConfiguration != null;
}
public void setExecutionConfigurationIsSet(boolean value) {
if (!value) {
this.executionConfiguration = null;
}
}
public WorkflowExecutionInfo getWorkflowExecutionInfo() {
return this.workflowExecutionInfo;
}
public DescribeWorkflowExecutionResponse setWorkflowExecutionInfo(WorkflowExecutionInfo workflowExecutionInfo) {
this.workflowExecutionInfo = workflowExecutionInfo;
return this;
}
public void unsetWorkflowExecutionInfo() {
this.workflowExecutionInfo = null;
}
/** Returns true if field workflowExecutionInfo is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowExecutionInfo() {
return this.workflowExecutionInfo != null;
}
public void setWorkflowExecutionInfoIsSet(boolean value) {
if (!value) {
this.workflowExecutionInfo = null;
}
}
public int getPendingActivitiesSize() {
return (this.pendingActivities == null) ? 0 : this.pendingActivities.size();
}
public java.util.Iterator getPendingActivitiesIterator() {
return (this.pendingActivities == null) ? null : this.pendingActivities.iterator();
}
public void addToPendingActivities(PendingActivityInfo elem) {
if (this.pendingActivities == null) {
this.pendingActivities = new ArrayList();
}
this.pendingActivities.add(elem);
}
public List getPendingActivities() {
return this.pendingActivities;
}
public DescribeWorkflowExecutionResponse setPendingActivities(List pendingActivities) {
this.pendingActivities = pendingActivities;
return this;
}
public void unsetPendingActivities() {
this.pendingActivities = null;
}
/** Returns true if field pendingActivities is set (has been assigned a value) and false otherwise */
public boolean isSetPendingActivities() {
return this.pendingActivities != null;
}
public void setPendingActivitiesIsSet(boolean value) {
if (!value) {
this.pendingActivities = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case EXECUTION_CONFIGURATION:
if (value == null) {
unsetExecutionConfiguration();
} else {
setExecutionConfiguration((WorkflowExecutionConfiguration)value);
}
break;
case WORKFLOW_EXECUTION_INFO:
if (value == null) {
unsetWorkflowExecutionInfo();
} else {
setWorkflowExecutionInfo((WorkflowExecutionInfo)value);
}
break;
case PENDING_ACTIVITIES:
if (value == null) {
unsetPendingActivities();
} else {
setPendingActivities((List)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case EXECUTION_CONFIGURATION:
return getExecutionConfiguration();
case WORKFLOW_EXECUTION_INFO:
return getWorkflowExecutionInfo();
case PENDING_ACTIVITIES:
return getPendingActivities();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case EXECUTION_CONFIGURATION:
return isSetExecutionConfiguration();
case WORKFLOW_EXECUTION_INFO:
return isSetWorkflowExecutionInfo();
case PENDING_ACTIVITIES:
return isSetPendingActivities();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof DescribeWorkflowExecutionResponse)
return this.equals((DescribeWorkflowExecutionResponse)that);
return false;
}
public boolean equals(DescribeWorkflowExecutionResponse that) {
if (that == null)
return false;
boolean this_present_executionConfiguration = true && this.isSetExecutionConfiguration();
boolean that_present_executionConfiguration = true && that.isSetExecutionConfiguration();
if (this_present_executionConfiguration || that_present_executionConfiguration) {
if (!(this_present_executionConfiguration && that_present_executionConfiguration))
return false;
if (!this.executionConfiguration.equals(that.executionConfiguration))
return false;
}
boolean this_present_workflowExecutionInfo = true && this.isSetWorkflowExecutionInfo();
boolean that_present_workflowExecutionInfo = true && that.isSetWorkflowExecutionInfo();
if (this_present_workflowExecutionInfo || that_present_workflowExecutionInfo) {
if (!(this_present_workflowExecutionInfo && that_present_workflowExecutionInfo))
return false;
if (!this.workflowExecutionInfo.equals(that.workflowExecutionInfo))
return false;
}
boolean this_present_pendingActivities = true && this.isSetPendingActivities();
boolean that_present_pendingActivities = true && that.isSetPendingActivities();
if (this_present_pendingActivities || that_present_pendingActivities) {
if (!(this_present_pendingActivities && that_present_pendingActivities))
return false;
if (!this.pendingActivities.equals(that.pendingActivities))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy