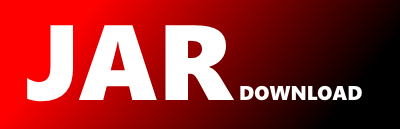
com.uber.cadence.DomainInfo Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class DomainInfo implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("DomainInfo");
private static final org.apache.thrift.protocol.TField NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("name", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField STATUS_FIELD_DESC = new org.apache.thrift.protocol.TField("status", org.apache.thrift.protocol.TType.I32, (short)20);
private static final org.apache.thrift.protocol.TField DESCRIPTION_FIELD_DESC = new org.apache.thrift.protocol.TField("description", org.apache.thrift.protocol.TType.STRING, (short)30);
private static final org.apache.thrift.protocol.TField OWNER_EMAIL_FIELD_DESC = new org.apache.thrift.protocol.TField("ownerEmail", org.apache.thrift.protocol.TType.STRING, (short)40);
private static final org.apache.thrift.protocol.TField DATA_FIELD_DESC = new org.apache.thrift.protocol.TField("data", org.apache.thrift.protocol.TType.MAP, (short)50);
private static final org.apache.thrift.protocol.TField UUID_FIELD_DESC = new org.apache.thrift.protocol.TField("uuid", org.apache.thrift.protocol.TType.STRING, (short)60);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new DomainInfoStandardSchemeFactory());
schemes.put(TupleScheme.class, new DomainInfoTupleSchemeFactory());
}
public String name; // optional
/**
*
* @see DomainStatus
*/
public DomainStatus status; // optional
public String description; // optional
public String ownerEmail; // optional
public Map data; // optional
public String uuid; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
NAME((short)10, "name"),
/**
*
* @see DomainStatus
*/
STATUS((short)20, "status"),
DESCRIPTION((short)30, "description"),
OWNER_EMAIL((short)40, "ownerEmail"),
DATA((short)50, "data"),
UUID((short)60, "uuid");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // NAME
return NAME;
case 20: // STATUS
return STATUS;
case 30: // DESCRIPTION
return DESCRIPTION;
case 40: // OWNER_EMAIL
return OWNER_EMAIL;
case 50: // DATA
return DATA;
case 60: // UUID
return UUID;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.NAME,_Fields.STATUS,_Fields.DESCRIPTION,_Fields.OWNER_EMAIL,_Fields.DATA,_Fields.UUID};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.NAME, new org.apache.thrift.meta_data.FieldMetaData("name", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.STATUS, new org.apache.thrift.meta_data.FieldMetaData("status", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, DomainStatus.class)));
tmpMap.put(_Fields.DESCRIPTION, new org.apache.thrift.meta_data.FieldMetaData("description", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.OWNER_EMAIL, new org.apache.thrift.meta_data.FieldMetaData("ownerEmail", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.DATA, new org.apache.thrift.meta_data.FieldMetaData("data", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING),
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.UUID, new org.apache.thrift.meta_data.FieldMetaData("uuid", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(DomainInfo.class, metaDataMap);
}
public DomainInfo() {
}
/**
* Performs a deep copy on other.
*/
public DomainInfo(DomainInfo other) {
if (other.isSetName()) {
this.name = other.name;
}
if (other.isSetStatus()) {
this.status = other.status;
}
if (other.isSetDescription()) {
this.description = other.description;
}
if (other.isSetOwnerEmail()) {
this.ownerEmail = other.ownerEmail;
}
if (other.isSetData()) {
Map __this__data = new HashMap(other.data);
this.data = __this__data;
}
if (other.isSetUuid()) {
this.uuid = other.uuid;
}
}
public DomainInfo deepCopy() {
return new DomainInfo(this);
}
@Override
public void clear() {
this.name = null;
this.status = null;
this.description = null;
this.ownerEmail = null;
this.data = null;
this.uuid = null;
}
public String getName() {
return this.name;
}
public DomainInfo setName(String name) {
this.name = name;
return this;
}
public void unsetName() {
this.name = null;
}
/** Returns true if field name is set (has been assigned a value) and false otherwise */
public boolean isSetName() {
return this.name != null;
}
public void setNameIsSet(boolean value) {
if (!value) {
this.name = null;
}
}
/**
*
* @see DomainStatus
*/
public DomainStatus getStatus() {
return this.status;
}
/**
*
* @see DomainStatus
*/
public DomainInfo setStatus(DomainStatus status) {
this.status = status;
return this;
}
public void unsetStatus() {
this.status = null;
}
/** Returns true if field status is set (has been assigned a value) and false otherwise */
public boolean isSetStatus() {
return this.status != null;
}
public void setStatusIsSet(boolean value) {
if (!value) {
this.status = null;
}
}
public String getDescription() {
return this.description;
}
public DomainInfo setDescription(String description) {
this.description = description;
return this;
}
public void unsetDescription() {
this.description = null;
}
/** Returns true if field description is set (has been assigned a value) and false otherwise */
public boolean isSetDescription() {
return this.description != null;
}
public void setDescriptionIsSet(boolean value) {
if (!value) {
this.description = null;
}
}
public String getOwnerEmail() {
return this.ownerEmail;
}
public DomainInfo setOwnerEmail(String ownerEmail) {
this.ownerEmail = ownerEmail;
return this;
}
public void unsetOwnerEmail() {
this.ownerEmail = null;
}
/** Returns true if field ownerEmail is set (has been assigned a value) and false otherwise */
public boolean isSetOwnerEmail() {
return this.ownerEmail != null;
}
public void setOwnerEmailIsSet(boolean value) {
if (!value) {
this.ownerEmail = null;
}
}
public int getDataSize() {
return (this.data == null) ? 0 : this.data.size();
}
public void putToData(String key, String val) {
if (this.data == null) {
this.data = new HashMap();
}
this.data.put(key, val);
}
public Map getData() {
return this.data;
}
public DomainInfo setData(Map data) {
this.data = data;
return this;
}
public void unsetData() {
this.data = null;
}
/** Returns true if field data is set (has been assigned a value) and false otherwise */
public boolean isSetData() {
return this.data != null;
}
public void setDataIsSet(boolean value) {
if (!value) {
this.data = null;
}
}
public String getUuid() {
return this.uuid;
}
public DomainInfo setUuid(String uuid) {
this.uuid = uuid;
return this;
}
public void unsetUuid() {
this.uuid = null;
}
/** Returns true if field uuid is set (has been assigned a value) and false otherwise */
public boolean isSetUuid() {
return this.uuid != null;
}
public void setUuidIsSet(boolean value) {
if (!value) {
this.uuid = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case NAME:
if (value == null) {
unsetName();
} else {
setName((String)value);
}
break;
case STATUS:
if (value == null) {
unsetStatus();
} else {
setStatus((DomainStatus)value);
}
break;
case DESCRIPTION:
if (value == null) {
unsetDescription();
} else {
setDescription((String)value);
}
break;
case OWNER_EMAIL:
if (value == null) {
unsetOwnerEmail();
} else {
setOwnerEmail((String)value);
}
break;
case DATA:
if (value == null) {
unsetData();
} else {
setData((Map)value);
}
break;
case UUID:
if (value == null) {
unsetUuid();
} else {
setUuid((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case NAME:
return getName();
case STATUS:
return getStatus();
case DESCRIPTION:
return getDescription();
case OWNER_EMAIL:
return getOwnerEmail();
case DATA:
return getData();
case UUID:
return getUuid();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case NAME:
return isSetName();
case STATUS:
return isSetStatus();
case DESCRIPTION:
return isSetDescription();
case OWNER_EMAIL:
return isSetOwnerEmail();
case DATA:
return isSetData();
case UUID:
return isSetUuid();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof DomainInfo)
return this.equals((DomainInfo)that);
return false;
}
public boolean equals(DomainInfo that) {
if (that == null)
return false;
boolean this_present_name = true && this.isSetName();
boolean that_present_name = true && that.isSetName();
if (this_present_name || that_present_name) {
if (!(this_present_name && that_present_name))
return false;
if (!this.name.equals(that.name))
return false;
}
boolean this_present_status = true && this.isSetStatus();
boolean that_present_status = true && that.isSetStatus();
if (this_present_status || that_present_status) {
if (!(this_present_status && that_present_status))
return false;
if (!this.status.equals(that.status))
return false;
}
boolean this_present_description = true && this.isSetDescription();
boolean that_present_description = true && that.isSetDescription();
if (this_present_description || that_present_description) {
if (!(this_present_description && that_present_description))
return false;
if (!this.description.equals(that.description))
return false;
}
boolean this_present_ownerEmail = true && this.isSetOwnerEmail();
boolean that_present_ownerEmail = true && that.isSetOwnerEmail();
if (this_present_ownerEmail || that_present_ownerEmail) {
if (!(this_present_ownerEmail && that_present_ownerEmail))
return false;
if (!this.ownerEmail.equals(that.ownerEmail))
return false;
}
boolean this_present_data = true && this.isSetData();
boolean that_present_data = true && that.isSetData();
if (this_present_data || that_present_data) {
if (!(this_present_data && that_present_data))
return false;
if (!this.data.equals(that.data))
return false;
}
boolean this_present_uuid = true && this.isSetUuid();
boolean that_present_uuid = true && that.isSetUuid();
if (this_present_uuid || that_present_uuid) {
if (!(this_present_uuid && that_present_uuid))
return false;
if (!this.uuid.equals(that.uuid))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy