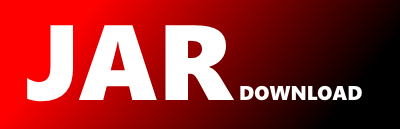
com.uber.cadence.ExternalWorkflowExecutionSignaledEventAttributes Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class ExternalWorkflowExecutionSignaledEventAttributes implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ExternalWorkflowExecutionSignaledEventAttributes");
private static final org.apache.thrift.protocol.TField INITIATED_EVENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("initiatedEventId", org.apache.thrift.protocol.TType.I64, (short)10);
private static final org.apache.thrift.protocol.TField DOMAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("domain", org.apache.thrift.protocol.TType.STRING, (short)20);
private static final org.apache.thrift.protocol.TField WORKFLOW_EXECUTION_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowExecution", org.apache.thrift.protocol.TType.STRUCT, (short)30);
private static final org.apache.thrift.protocol.TField CONTROL_FIELD_DESC = new org.apache.thrift.protocol.TField("control", org.apache.thrift.protocol.TType.STRING, (short)40);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ExternalWorkflowExecutionSignaledEventAttributesStandardSchemeFactory());
schemes.put(TupleScheme.class, new ExternalWorkflowExecutionSignaledEventAttributesTupleSchemeFactory());
}
public long initiatedEventId; // optional
public String domain; // optional
public WorkflowExecution workflowExecution; // optional
public ByteBuffer control; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
INITIATED_EVENT_ID((short)10, "initiatedEventId"),
DOMAIN((short)20, "domain"),
WORKFLOW_EXECUTION((short)30, "workflowExecution"),
CONTROL((short)40, "control");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // INITIATED_EVENT_ID
return INITIATED_EVENT_ID;
case 20: // DOMAIN
return DOMAIN;
case 30: // WORKFLOW_EXECUTION
return WORKFLOW_EXECUTION;
case 40: // CONTROL
return CONTROL;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __INITIATEDEVENTID_ISSET_ID = 0;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.INITIATED_EVENT_ID,_Fields.DOMAIN,_Fields.WORKFLOW_EXECUTION,_Fields.CONTROL};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.INITIATED_EVENT_ID, new org.apache.thrift.meta_data.FieldMetaData("initiatedEventId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.DOMAIN, new org.apache.thrift.meta_data.FieldMetaData("domain", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WORKFLOW_EXECUTION, new org.apache.thrift.meta_data.FieldMetaData("workflowExecution", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecution.class)));
tmpMap.put(_Fields.CONTROL, new org.apache.thrift.meta_data.FieldMetaData("control", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ExternalWorkflowExecutionSignaledEventAttributes.class, metaDataMap);
}
public ExternalWorkflowExecutionSignaledEventAttributes() {
}
/**
* Performs a deep copy on other.
*/
public ExternalWorkflowExecutionSignaledEventAttributes(ExternalWorkflowExecutionSignaledEventAttributes other) {
__isset_bitfield = other.__isset_bitfield;
this.initiatedEventId = other.initiatedEventId;
if (other.isSetDomain()) {
this.domain = other.domain;
}
if (other.isSetWorkflowExecution()) {
this.workflowExecution = new WorkflowExecution(other.workflowExecution);
}
if (other.isSetControl()) {
this.control = org.apache.thrift.TBaseHelper.copyBinary(other.control);
}
}
public ExternalWorkflowExecutionSignaledEventAttributes deepCopy() {
return new ExternalWorkflowExecutionSignaledEventAttributes(this);
}
@Override
public void clear() {
setInitiatedEventIdIsSet(false);
this.initiatedEventId = 0;
this.domain = null;
this.workflowExecution = null;
this.control = null;
}
public long getInitiatedEventId() {
return this.initiatedEventId;
}
public ExternalWorkflowExecutionSignaledEventAttributes setInitiatedEventId(long initiatedEventId) {
this.initiatedEventId = initiatedEventId;
setInitiatedEventIdIsSet(true);
return this;
}
public void unsetInitiatedEventId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __INITIATEDEVENTID_ISSET_ID);
}
/** Returns true if field initiatedEventId is set (has been assigned a value) and false otherwise */
public boolean isSetInitiatedEventId() {
return EncodingUtils.testBit(__isset_bitfield, __INITIATEDEVENTID_ISSET_ID);
}
public void setInitiatedEventIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __INITIATEDEVENTID_ISSET_ID, value);
}
public String getDomain() {
return this.domain;
}
public ExternalWorkflowExecutionSignaledEventAttributes setDomain(String domain) {
this.domain = domain;
return this;
}
public void unsetDomain() {
this.domain = null;
}
/** Returns true if field domain is set (has been assigned a value) and false otherwise */
public boolean isSetDomain() {
return this.domain != null;
}
public void setDomainIsSet(boolean value) {
if (!value) {
this.domain = null;
}
}
public WorkflowExecution getWorkflowExecution() {
return this.workflowExecution;
}
public ExternalWorkflowExecutionSignaledEventAttributes setWorkflowExecution(WorkflowExecution workflowExecution) {
this.workflowExecution = workflowExecution;
return this;
}
public void unsetWorkflowExecution() {
this.workflowExecution = null;
}
/** Returns true if field workflowExecution is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowExecution() {
return this.workflowExecution != null;
}
public void setWorkflowExecutionIsSet(boolean value) {
if (!value) {
this.workflowExecution = null;
}
}
public byte[] getControl() {
setControl(org.apache.thrift.TBaseHelper.rightSize(control));
return control == null ? null : control.array();
}
public ByteBuffer bufferForControl() {
return org.apache.thrift.TBaseHelper.copyBinary(control);
}
public ExternalWorkflowExecutionSignaledEventAttributes setControl(byte[] control) {
this.control = control == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(control, control.length));
return this;
}
public ExternalWorkflowExecutionSignaledEventAttributes setControl(ByteBuffer control) {
this.control = org.apache.thrift.TBaseHelper.copyBinary(control);
return this;
}
public void unsetControl() {
this.control = null;
}
/** Returns true if field control is set (has been assigned a value) and false otherwise */
public boolean isSetControl() {
return this.control != null;
}
public void setControlIsSet(boolean value) {
if (!value) {
this.control = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case INITIATED_EVENT_ID:
if (value == null) {
unsetInitiatedEventId();
} else {
setInitiatedEventId((Long)value);
}
break;
case DOMAIN:
if (value == null) {
unsetDomain();
} else {
setDomain((String)value);
}
break;
case WORKFLOW_EXECUTION:
if (value == null) {
unsetWorkflowExecution();
} else {
setWorkflowExecution((WorkflowExecution)value);
}
break;
case CONTROL:
if (value == null) {
unsetControl();
} else {
setControl((ByteBuffer)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case INITIATED_EVENT_ID:
return getInitiatedEventId();
case DOMAIN:
return getDomain();
case WORKFLOW_EXECUTION:
return getWorkflowExecution();
case CONTROL:
return getControl();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case INITIATED_EVENT_ID:
return isSetInitiatedEventId();
case DOMAIN:
return isSetDomain();
case WORKFLOW_EXECUTION:
return isSetWorkflowExecution();
case CONTROL:
return isSetControl();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof ExternalWorkflowExecutionSignaledEventAttributes)
return this.equals((ExternalWorkflowExecutionSignaledEventAttributes)that);
return false;
}
public boolean equals(ExternalWorkflowExecutionSignaledEventAttributes that) {
if (that == null)
return false;
boolean this_present_initiatedEventId = true && this.isSetInitiatedEventId();
boolean that_present_initiatedEventId = true && that.isSetInitiatedEventId();
if (this_present_initiatedEventId || that_present_initiatedEventId) {
if (!(this_present_initiatedEventId && that_present_initiatedEventId))
return false;
if (this.initiatedEventId != that.initiatedEventId)
return false;
}
boolean this_present_domain = true && this.isSetDomain();
boolean that_present_domain = true && that.isSetDomain();
if (this_present_domain || that_present_domain) {
if (!(this_present_domain && that_present_domain))
return false;
if (!this.domain.equals(that.domain))
return false;
}
boolean this_present_workflowExecution = true && this.isSetWorkflowExecution();
boolean that_present_workflowExecution = true && that.isSetWorkflowExecution();
if (this_present_workflowExecution || that_present_workflowExecution) {
if (!(this_present_workflowExecution && that_present_workflowExecution))
return false;
if (!this.workflowExecution.equals(that.workflowExecution))
return false;
}
boolean this_present_control = true && this.isSetControl();
boolean that_present_control = true && that.isSetControl();
if (this_present_control || that_present_control) {
if (!(this_present_control && that_present_control))
return false;
if (!this.control.equals(that.control))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy