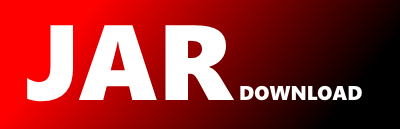
com.uber.cadence.GetWorkflowExecutionHistoryRequest Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class GetWorkflowExecutionHistoryRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetWorkflowExecutionHistoryRequest");
private static final org.apache.thrift.protocol.TField DOMAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("domain", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField EXECUTION_FIELD_DESC = new org.apache.thrift.protocol.TField("execution", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField MAXIMUM_PAGE_SIZE_FIELD_DESC = new org.apache.thrift.protocol.TField("maximumPageSize", org.apache.thrift.protocol.TType.I32, (short)30);
private static final org.apache.thrift.protocol.TField NEXT_PAGE_TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("nextPageToken", org.apache.thrift.protocol.TType.STRING, (short)40);
private static final org.apache.thrift.protocol.TField WAIT_FOR_NEW_EVENT_FIELD_DESC = new org.apache.thrift.protocol.TField("waitForNewEvent", org.apache.thrift.protocol.TType.BOOL, (short)50);
private static final org.apache.thrift.protocol.TField HISTORY_EVENT_FILTER_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("HistoryEventFilterType", org.apache.thrift.protocol.TType.I32, (short)60);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new GetWorkflowExecutionHistoryRequestStandardSchemeFactory());
schemes.put(TupleScheme.class, new GetWorkflowExecutionHistoryRequestTupleSchemeFactory());
}
public String domain; // optional
public WorkflowExecution execution; // optional
public int maximumPageSize; // optional
public ByteBuffer nextPageToken; // optional
public boolean waitForNewEvent; // optional
/**
*
* @see HistoryEventFilterType
*/
public HistoryEventFilterType HistoryEventFilterType; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
DOMAIN((short)10, "domain"),
EXECUTION((short)20, "execution"),
MAXIMUM_PAGE_SIZE((short)30, "maximumPageSize"),
NEXT_PAGE_TOKEN((short)40, "nextPageToken"),
WAIT_FOR_NEW_EVENT((short)50, "waitForNewEvent"),
/**
*
* @see HistoryEventFilterType
*/
HISTORY_EVENT_FILTER_TYPE((short)60, "HistoryEventFilterType");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // DOMAIN
return DOMAIN;
case 20: // EXECUTION
return EXECUTION;
case 30: // MAXIMUM_PAGE_SIZE
return MAXIMUM_PAGE_SIZE;
case 40: // NEXT_PAGE_TOKEN
return NEXT_PAGE_TOKEN;
case 50: // WAIT_FOR_NEW_EVENT
return WAIT_FOR_NEW_EVENT;
case 60: // HISTORY_EVENT_FILTER_TYPE
return HISTORY_EVENT_FILTER_TYPE;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __MAXIMUMPAGESIZE_ISSET_ID = 0;
private static final int __WAITFORNEWEVENT_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.DOMAIN,_Fields.EXECUTION,_Fields.MAXIMUM_PAGE_SIZE,_Fields.NEXT_PAGE_TOKEN,_Fields.WAIT_FOR_NEW_EVENT,_Fields.HISTORY_EVENT_FILTER_TYPE};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.DOMAIN, new org.apache.thrift.meta_data.FieldMetaData("domain", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.EXECUTION, new org.apache.thrift.meta_data.FieldMetaData("execution", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecution.class)));
tmpMap.put(_Fields.MAXIMUM_PAGE_SIZE, new org.apache.thrift.meta_data.FieldMetaData("maximumPageSize", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.NEXT_PAGE_TOKEN, new org.apache.thrift.meta_data.FieldMetaData("nextPageToken", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.WAIT_FOR_NEW_EVENT, new org.apache.thrift.meta_data.FieldMetaData("waitForNewEvent", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.HISTORY_EVENT_FILTER_TYPE, new org.apache.thrift.meta_data.FieldMetaData("HistoryEventFilterType", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, HistoryEventFilterType.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetWorkflowExecutionHistoryRequest.class, metaDataMap);
}
public GetWorkflowExecutionHistoryRequest() {
}
/**
* Performs a deep copy on other.
*/
public GetWorkflowExecutionHistoryRequest(GetWorkflowExecutionHistoryRequest other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetDomain()) {
this.domain = other.domain;
}
if (other.isSetExecution()) {
this.execution = new WorkflowExecution(other.execution);
}
this.maximumPageSize = other.maximumPageSize;
if (other.isSetNextPageToken()) {
this.nextPageToken = org.apache.thrift.TBaseHelper.copyBinary(other.nextPageToken);
}
this.waitForNewEvent = other.waitForNewEvent;
if (other.isSetHistoryEventFilterType()) {
this.HistoryEventFilterType = other.HistoryEventFilterType;
}
}
public GetWorkflowExecutionHistoryRequest deepCopy() {
return new GetWorkflowExecutionHistoryRequest(this);
}
@Override
public void clear() {
this.domain = null;
this.execution = null;
setMaximumPageSizeIsSet(false);
this.maximumPageSize = 0;
this.nextPageToken = null;
setWaitForNewEventIsSet(false);
this.waitForNewEvent = false;
this.HistoryEventFilterType = null;
}
public String getDomain() {
return this.domain;
}
public GetWorkflowExecutionHistoryRequest setDomain(String domain) {
this.domain = domain;
return this;
}
public void unsetDomain() {
this.domain = null;
}
/** Returns true if field domain is set (has been assigned a value) and false otherwise */
public boolean isSetDomain() {
return this.domain != null;
}
public void setDomainIsSet(boolean value) {
if (!value) {
this.domain = null;
}
}
public WorkflowExecution getExecution() {
return this.execution;
}
public GetWorkflowExecutionHistoryRequest setExecution(WorkflowExecution execution) {
this.execution = execution;
return this;
}
public void unsetExecution() {
this.execution = null;
}
/** Returns true if field execution is set (has been assigned a value) and false otherwise */
public boolean isSetExecution() {
return this.execution != null;
}
public void setExecutionIsSet(boolean value) {
if (!value) {
this.execution = null;
}
}
public int getMaximumPageSize() {
return this.maximumPageSize;
}
public GetWorkflowExecutionHistoryRequest setMaximumPageSize(int maximumPageSize) {
this.maximumPageSize = maximumPageSize;
setMaximumPageSizeIsSet(true);
return this;
}
public void unsetMaximumPageSize() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __MAXIMUMPAGESIZE_ISSET_ID);
}
/** Returns true if field maximumPageSize is set (has been assigned a value) and false otherwise */
public boolean isSetMaximumPageSize() {
return EncodingUtils.testBit(__isset_bitfield, __MAXIMUMPAGESIZE_ISSET_ID);
}
public void setMaximumPageSizeIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __MAXIMUMPAGESIZE_ISSET_ID, value);
}
public byte[] getNextPageToken() {
setNextPageToken(org.apache.thrift.TBaseHelper.rightSize(nextPageToken));
return nextPageToken == null ? null : nextPageToken.array();
}
public ByteBuffer bufferForNextPageToken() {
return org.apache.thrift.TBaseHelper.copyBinary(nextPageToken);
}
public GetWorkflowExecutionHistoryRequest setNextPageToken(byte[] nextPageToken) {
this.nextPageToken = nextPageToken == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(nextPageToken, nextPageToken.length));
return this;
}
public GetWorkflowExecutionHistoryRequest setNextPageToken(ByteBuffer nextPageToken) {
this.nextPageToken = org.apache.thrift.TBaseHelper.copyBinary(nextPageToken);
return this;
}
public void unsetNextPageToken() {
this.nextPageToken = null;
}
/** Returns true if field nextPageToken is set (has been assigned a value) and false otherwise */
public boolean isSetNextPageToken() {
return this.nextPageToken != null;
}
public void setNextPageTokenIsSet(boolean value) {
if (!value) {
this.nextPageToken = null;
}
}
public boolean isWaitForNewEvent() {
return this.waitForNewEvent;
}
public GetWorkflowExecutionHistoryRequest setWaitForNewEvent(boolean waitForNewEvent) {
this.waitForNewEvent = waitForNewEvent;
setWaitForNewEventIsSet(true);
return this;
}
public void unsetWaitForNewEvent() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __WAITFORNEWEVENT_ISSET_ID);
}
/** Returns true if field waitForNewEvent is set (has been assigned a value) and false otherwise */
public boolean isSetWaitForNewEvent() {
return EncodingUtils.testBit(__isset_bitfield, __WAITFORNEWEVENT_ISSET_ID);
}
public void setWaitForNewEventIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __WAITFORNEWEVENT_ISSET_ID, value);
}
/**
*
* @see HistoryEventFilterType
*/
public HistoryEventFilterType getHistoryEventFilterType() {
return this.HistoryEventFilterType;
}
/**
*
* @see HistoryEventFilterType
*/
public GetWorkflowExecutionHistoryRequest setHistoryEventFilterType(HistoryEventFilterType HistoryEventFilterType) {
this.HistoryEventFilterType = HistoryEventFilterType;
return this;
}
public void unsetHistoryEventFilterType() {
this.HistoryEventFilterType = null;
}
/** Returns true if field HistoryEventFilterType is set (has been assigned a value) and false otherwise */
public boolean isSetHistoryEventFilterType() {
return this.HistoryEventFilterType != null;
}
public void setHistoryEventFilterTypeIsSet(boolean value) {
if (!value) {
this.HistoryEventFilterType = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case DOMAIN:
if (value == null) {
unsetDomain();
} else {
setDomain((String)value);
}
break;
case EXECUTION:
if (value == null) {
unsetExecution();
} else {
setExecution((WorkflowExecution)value);
}
break;
case MAXIMUM_PAGE_SIZE:
if (value == null) {
unsetMaximumPageSize();
} else {
setMaximumPageSize((Integer)value);
}
break;
case NEXT_PAGE_TOKEN:
if (value == null) {
unsetNextPageToken();
} else {
setNextPageToken((ByteBuffer)value);
}
break;
case WAIT_FOR_NEW_EVENT:
if (value == null) {
unsetWaitForNewEvent();
} else {
setWaitForNewEvent((Boolean)value);
}
break;
case HISTORY_EVENT_FILTER_TYPE:
if (value == null) {
unsetHistoryEventFilterType();
} else {
setHistoryEventFilterType((HistoryEventFilterType)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case DOMAIN:
return getDomain();
case EXECUTION:
return getExecution();
case MAXIMUM_PAGE_SIZE:
return getMaximumPageSize();
case NEXT_PAGE_TOKEN:
return getNextPageToken();
case WAIT_FOR_NEW_EVENT:
return isWaitForNewEvent();
case HISTORY_EVENT_FILTER_TYPE:
return getHistoryEventFilterType();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case DOMAIN:
return isSetDomain();
case EXECUTION:
return isSetExecution();
case MAXIMUM_PAGE_SIZE:
return isSetMaximumPageSize();
case NEXT_PAGE_TOKEN:
return isSetNextPageToken();
case WAIT_FOR_NEW_EVENT:
return isSetWaitForNewEvent();
case HISTORY_EVENT_FILTER_TYPE:
return isSetHistoryEventFilterType();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof GetWorkflowExecutionHistoryRequest)
return this.equals((GetWorkflowExecutionHistoryRequest)that);
return false;
}
public boolean equals(GetWorkflowExecutionHistoryRequest that) {
if (that == null)
return false;
boolean this_present_domain = true && this.isSetDomain();
boolean that_present_domain = true && that.isSetDomain();
if (this_present_domain || that_present_domain) {
if (!(this_present_domain && that_present_domain))
return false;
if (!this.domain.equals(that.domain))
return false;
}
boolean this_present_execution = true && this.isSetExecution();
boolean that_present_execution = true && that.isSetExecution();
if (this_present_execution || that_present_execution) {
if (!(this_present_execution && that_present_execution))
return false;
if (!this.execution.equals(that.execution))
return false;
}
boolean this_present_maximumPageSize = true && this.isSetMaximumPageSize();
boolean that_present_maximumPageSize = true && that.isSetMaximumPageSize();
if (this_present_maximumPageSize || that_present_maximumPageSize) {
if (!(this_present_maximumPageSize && that_present_maximumPageSize))
return false;
if (this.maximumPageSize != that.maximumPageSize)
return false;
}
boolean this_present_nextPageToken = true && this.isSetNextPageToken();
boolean that_present_nextPageToken = true && that.isSetNextPageToken();
if (this_present_nextPageToken || that_present_nextPageToken) {
if (!(this_present_nextPageToken && that_present_nextPageToken))
return false;
if (!this.nextPageToken.equals(that.nextPageToken))
return false;
}
boolean this_present_waitForNewEvent = true && this.isSetWaitForNewEvent();
boolean that_present_waitForNewEvent = true && that.isSetWaitForNewEvent();
if (this_present_waitForNewEvent || that_present_waitForNewEvent) {
if (!(this_present_waitForNewEvent && that_present_waitForNewEvent))
return false;
if (this.waitForNewEvent != that.waitForNewEvent)
return false;
}
boolean this_present_HistoryEventFilterType = true && this.isSetHistoryEventFilterType();
boolean that_present_HistoryEventFilterType = true && that.isSetHistoryEventFilterType();
if (this_present_HistoryEventFilterType || that_present_HistoryEventFilterType) {
if (!(this_present_HistoryEventFilterType && that_present_HistoryEventFilterType))
return false;
if (!this.HistoryEventFilterType.equals(that.HistoryEventFilterType))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy