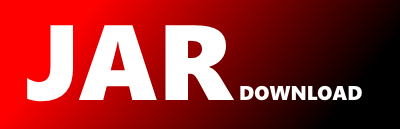
com.uber.cadence.PendingActivityInfo Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class PendingActivityInfo implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("PendingActivityInfo");
private static final org.apache.thrift.protocol.TField ACTIVITY_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("activityID", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField ACTIVITY_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("activityType", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField STATE_FIELD_DESC = new org.apache.thrift.protocol.TField("state", org.apache.thrift.protocol.TType.I32, (short)30);
private static final org.apache.thrift.protocol.TField HEARTBEAT_DETAILS_FIELD_DESC = new org.apache.thrift.protocol.TField("heartbeatDetails", org.apache.thrift.protocol.TType.STRING, (short)40);
private static final org.apache.thrift.protocol.TField LAST_HEARTBEAT_TIMESTAMP_FIELD_DESC = new org.apache.thrift.protocol.TField("lastHeartbeatTimestamp", org.apache.thrift.protocol.TType.I64, (short)50);
private static final org.apache.thrift.protocol.TField LAST_STARTED_TIMESTAMP_FIELD_DESC = new org.apache.thrift.protocol.TField("lastStartedTimestamp", org.apache.thrift.protocol.TType.I64, (short)60);
private static final org.apache.thrift.protocol.TField ATTEMPT_FIELD_DESC = new org.apache.thrift.protocol.TField("attempt", org.apache.thrift.protocol.TType.I32, (short)70);
private static final org.apache.thrift.protocol.TField MAXIMUM_ATTEMPTS_FIELD_DESC = new org.apache.thrift.protocol.TField("maximumAttempts", org.apache.thrift.protocol.TType.I32, (short)80);
private static final org.apache.thrift.protocol.TField SCHEDULED_TIMESTAMP_FIELD_DESC = new org.apache.thrift.protocol.TField("scheduledTimestamp", org.apache.thrift.protocol.TType.I64, (short)90);
private static final org.apache.thrift.protocol.TField EXPIRATION_TIMESTAMP_FIELD_DESC = new org.apache.thrift.protocol.TField("expirationTimestamp", org.apache.thrift.protocol.TType.I64, (short)100);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new PendingActivityInfoStandardSchemeFactory());
schemes.put(TupleScheme.class, new PendingActivityInfoTupleSchemeFactory());
}
public String activityID; // optional
public ActivityType activityType; // optional
/**
*
* @see PendingActivityState
*/
public PendingActivityState state; // optional
public ByteBuffer heartbeatDetails; // optional
public long lastHeartbeatTimestamp; // optional
public long lastStartedTimestamp; // optional
public int attempt; // optional
public int maximumAttempts; // optional
public long scheduledTimestamp; // optional
public long expirationTimestamp; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
ACTIVITY_ID((short)10, "activityID"),
ACTIVITY_TYPE((short)20, "activityType"),
/**
*
* @see PendingActivityState
*/
STATE((short)30, "state"),
HEARTBEAT_DETAILS((short)40, "heartbeatDetails"),
LAST_HEARTBEAT_TIMESTAMP((short)50, "lastHeartbeatTimestamp"),
LAST_STARTED_TIMESTAMP((short)60, "lastStartedTimestamp"),
ATTEMPT((short)70, "attempt"),
MAXIMUM_ATTEMPTS((short)80, "maximumAttempts"),
SCHEDULED_TIMESTAMP((short)90, "scheduledTimestamp"),
EXPIRATION_TIMESTAMP((short)100, "expirationTimestamp");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // ACTIVITY_ID
return ACTIVITY_ID;
case 20: // ACTIVITY_TYPE
return ACTIVITY_TYPE;
case 30: // STATE
return STATE;
case 40: // HEARTBEAT_DETAILS
return HEARTBEAT_DETAILS;
case 50: // LAST_HEARTBEAT_TIMESTAMP
return LAST_HEARTBEAT_TIMESTAMP;
case 60: // LAST_STARTED_TIMESTAMP
return LAST_STARTED_TIMESTAMP;
case 70: // ATTEMPT
return ATTEMPT;
case 80: // MAXIMUM_ATTEMPTS
return MAXIMUM_ATTEMPTS;
case 90: // SCHEDULED_TIMESTAMP
return SCHEDULED_TIMESTAMP;
case 100: // EXPIRATION_TIMESTAMP
return EXPIRATION_TIMESTAMP;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __LASTHEARTBEATTIMESTAMP_ISSET_ID = 0;
private static final int __LASTSTARTEDTIMESTAMP_ISSET_ID = 1;
private static final int __ATTEMPT_ISSET_ID = 2;
private static final int __MAXIMUMATTEMPTS_ISSET_ID = 3;
private static final int __SCHEDULEDTIMESTAMP_ISSET_ID = 4;
private static final int __EXPIRATIONTIMESTAMP_ISSET_ID = 5;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.ACTIVITY_ID,_Fields.ACTIVITY_TYPE,_Fields.STATE,_Fields.HEARTBEAT_DETAILS,_Fields.LAST_HEARTBEAT_TIMESTAMP,_Fields.LAST_STARTED_TIMESTAMP,_Fields.ATTEMPT,_Fields.MAXIMUM_ATTEMPTS,_Fields.SCHEDULED_TIMESTAMP,_Fields.EXPIRATION_TIMESTAMP};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.ACTIVITY_ID, new org.apache.thrift.meta_data.FieldMetaData("activityID", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.ACTIVITY_TYPE, new org.apache.thrift.meta_data.FieldMetaData("activityType", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ActivityType.class)));
tmpMap.put(_Fields.STATE, new org.apache.thrift.meta_data.FieldMetaData("state", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, PendingActivityState.class)));
tmpMap.put(_Fields.HEARTBEAT_DETAILS, new org.apache.thrift.meta_data.FieldMetaData("heartbeatDetails", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.LAST_HEARTBEAT_TIMESTAMP, new org.apache.thrift.meta_data.FieldMetaData("lastHeartbeatTimestamp", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.LAST_STARTED_TIMESTAMP, new org.apache.thrift.meta_data.FieldMetaData("lastStartedTimestamp", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.ATTEMPT, new org.apache.thrift.meta_data.FieldMetaData("attempt", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.MAXIMUM_ATTEMPTS, new org.apache.thrift.meta_data.FieldMetaData("maximumAttempts", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.SCHEDULED_TIMESTAMP, new org.apache.thrift.meta_data.FieldMetaData("scheduledTimestamp", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.EXPIRATION_TIMESTAMP, new org.apache.thrift.meta_data.FieldMetaData("expirationTimestamp", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(PendingActivityInfo.class, metaDataMap);
}
public PendingActivityInfo() {
}
/**
* Performs a deep copy on other.
*/
public PendingActivityInfo(PendingActivityInfo other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetActivityID()) {
this.activityID = other.activityID;
}
if (other.isSetActivityType()) {
this.activityType = new ActivityType(other.activityType);
}
if (other.isSetState()) {
this.state = other.state;
}
if (other.isSetHeartbeatDetails()) {
this.heartbeatDetails = org.apache.thrift.TBaseHelper.copyBinary(other.heartbeatDetails);
}
this.lastHeartbeatTimestamp = other.lastHeartbeatTimestamp;
this.lastStartedTimestamp = other.lastStartedTimestamp;
this.attempt = other.attempt;
this.maximumAttempts = other.maximumAttempts;
this.scheduledTimestamp = other.scheduledTimestamp;
this.expirationTimestamp = other.expirationTimestamp;
}
public PendingActivityInfo deepCopy() {
return new PendingActivityInfo(this);
}
@Override
public void clear() {
this.activityID = null;
this.activityType = null;
this.state = null;
this.heartbeatDetails = null;
setLastHeartbeatTimestampIsSet(false);
this.lastHeartbeatTimestamp = 0;
setLastStartedTimestampIsSet(false);
this.lastStartedTimestamp = 0;
setAttemptIsSet(false);
this.attempt = 0;
setMaximumAttemptsIsSet(false);
this.maximumAttempts = 0;
setScheduledTimestampIsSet(false);
this.scheduledTimestamp = 0;
setExpirationTimestampIsSet(false);
this.expirationTimestamp = 0;
}
public String getActivityID() {
return this.activityID;
}
public PendingActivityInfo setActivityID(String activityID) {
this.activityID = activityID;
return this;
}
public void unsetActivityID() {
this.activityID = null;
}
/** Returns true if field activityID is set (has been assigned a value) and false otherwise */
public boolean isSetActivityID() {
return this.activityID != null;
}
public void setActivityIDIsSet(boolean value) {
if (!value) {
this.activityID = null;
}
}
public ActivityType getActivityType() {
return this.activityType;
}
public PendingActivityInfo setActivityType(ActivityType activityType) {
this.activityType = activityType;
return this;
}
public void unsetActivityType() {
this.activityType = null;
}
/** Returns true if field activityType is set (has been assigned a value) and false otherwise */
public boolean isSetActivityType() {
return this.activityType != null;
}
public void setActivityTypeIsSet(boolean value) {
if (!value) {
this.activityType = null;
}
}
/**
*
* @see PendingActivityState
*/
public PendingActivityState getState() {
return this.state;
}
/**
*
* @see PendingActivityState
*/
public PendingActivityInfo setState(PendingActivityState state) {
this.state = state;
return this;
}
public void unsetState() {
this.state = null;
}
/** Returns true if field state is set (has been assigned a value) and false otherwise */
public boolean isSetState() {
return this.state != null;
}
public void setStateIsSet(boolean value) {
if (!value) {
this.state = null;
}
}
public byte[] getHeartbeatDetails() {
setHeartbeatDetails(org.apache.thrift.TBaseHelper.rightSize(heartbeatDetails));
return heartbeatDetails == null ? null : heartbeatDetails.array();
}
public ByteBuffer bufferForHeartbeatDetails() {
return org.apache.thrift.TBaseHelper.copyBinary(heartbeatDetails);
}
public PendingActivityInfo setHeartbeatDetails(byte[] heartbeatDetails) {
this.heartbeatDetails = heartbeatDetails == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(heartbeatDetails, heartbeatDetails.length));
return this;
}
public PendingActivityInfo setHeartbeatDetails(ByteBuffer heartbeatDetails) {
this.heartbeatDetails = org.apache.thrift.TBaseHelper.copyBinary(heartbeatDetails);
return this;
}
public void unsetHeartbeatDetails() {
this.heartbeatDetails = null;
}
/** Returns true if field heartbeatDetails is set (has been assigned a value) and false otherwise */
public boolean isSetHeartbeatDetails() {
return this.heartbeatDetails != null;
}
public void setHeartbeatDetailsIsSet(boolean value) {
if (!value) {
this.heartbeatDetails = null;
}
}
public long getLastHeartbeatTimestamp() {
return this.lastHeartbeatTimestamp;
}
public PendingActivityInfo setLastHeartbeatTimestamp(long lastHeartbeatTimestamp) {
this.lastHeartbeatTimestamp = lastHeartbeatTimestamp;
setLastHeartbeatTimestampIsSet(true);
return this;
}
public void unsetLastHeartbeatTimestamp() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __LASTHEARTBEATTIMESTAMP_ISSET_ID);
}
/** Returns true if field lastHeartbeatTimestamp is set (has been assigned a value) and false otherwise */
public boolean isSetLastHeartbeatTimestamp() {
return EncodingUtils.testBit(__isset_bitfield, __LASTHEARTBEATTIMESTAMP_ISSET_ID);
}
public void setLastHeartbeatTimestampIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __LASTHEARTBEATTIMESTAMP_ISSET_ID, value);
}
public long getLastStartedTimestamp() {
return this.lastStartedTimestamp;
}
public PendingActivityInfo setLastStartedTimestamp(long lastStartedTimestamp) {
this.lastStartedTimestamp = lastStartedTimestamp;
setLastStartedTimestampIsSet(true);
return this;
}
public void unsetLastStartedTimestamp() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __LASTSTARTEDTIMESTAMP_ISSET_ID);
}
/** Returns true if field lastStartedTimestamp is set (has been assigned a value) and false otherwise */
public boolean isSetLastStartedTimestamp() {
return EncodingUtils.testBit(__isset_bitfield, __LASTSTARTEDTIMESTAMP_ISSET_ID);
}
public void setLastStartedTimestampIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __LASTSTARTEDTIMESTAMP_ISSET_ID, value);
}
public int getAttempt() {
return this.attempt;
}
public PendingActivityInfo setAttempt(int attempt) {
this.attempt = attempt;
setAttemptIsSet(true);
return this;
}
public void unsetAttempt() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __ATTEMPT_ISSET_ID);
}
/** Returns true if field attempt is set (has been assigned a value) and false otherwise */
public boolean isSetAttempt() {
return EncodingUtils.testBit(__isset_bitfield, __ATTEMPT_ISSET_ID);
}
public void setAttemptIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __ATTEMPT_ISSET_ID, value);
}
public int getMaximumAttempts() {
return this.maximumAttempts;
}
public PendingActivityInfo setMaximumAttempts(int maximumAttempts) {
this.maximumAttempts = maximumAttempts;
setMaximumAttemptsIsSet(true);
return this;
}
public void unsetMaximumAttempts() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __MAXIMUMATTEMPTS_ISSET_ID);
}
/** Returns true if field maximumAttempts is set (has been assigned a value) and false otherwise */
public boolean isSetMaximumAttempts() {
return EncodingUtils.testBit(__isset_bitfield, __MAXIMUMATTEMPTS_ISSET_ID);
}
public void setMaximumAttemptsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __MAXIMUMATTEMPTS_ISSET_ID, value);
}
public long getScheduledTimestamp() {
return this.scheduledTimestamp;
}
public PendingActivityInfo setScheduledTimestamp(long scheduledTimestamp) {
this.scheduledTimestamp = scheduledTimestamp;
setScheduledTimestampIsSet(true);
return this;
}
public void unsetScheduledTimestamp() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SCHEDULEDTIMESTAMP_ISSET_ID);
}
/** Returns true if field scheduledTimestamp is set (has been assigned a value) and false otherwise */
public boolean isSetScheduledTimestamp() {
return EncodingUtils.testBit(__isset_bitfield, __SCHEDULEDTIMESTAMP_ISSET_ID);
}
public void setScheduledTimestampIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SCHEDULEDTIMESTAMP_ISSET_ID, value);
}
public long getExpirationTimestamp() {
return this.expirationTimestamp;
}
public PendingActivityInfo setExpirationTimestamp(long expirationTimestamp) {
this.expirationTimestamp = expirationTimestamp;
setExpirationTimestampIsSet(true);
return this;
}
public void unsetExpirationTimestamp() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __EXPIRATIONTIMESTAMP_ISSET_ID);
}
/** Returns true if field expirationTimestamp is set (has been assigned a value) and false otherwise */
public boolean isSetExpirationTimestamp() {
return EncodingUtils.testBit(__isset_bitfield, __EXPIRATIONTIMESTAMP_ISSET_ID);
}
public void setExpirationTimestampIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __EXPIRATIONTIMESTAMP_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case ACTIVITY_ID:
if (value == null) {
unsetActivityID();
} else {
setActivityID((String)value);
}
break;
case ACTIVITY_TYPE:
if (value == null) {
unsetActivityType();
} else {
setActivityType((ActivityType)value);
}
break;
case STATE:
if (value == null) {
unsetState();
} else {
setState((PendingActivityState)value);
}
break;
case HEARTBEAT_DETAILS:
if (value == null) {
unsetHeartbeatDetails();
} else {
setHeartbeatDetails((ByteBuffer)value);
}
break;
case LAST_HEARTBEAT_TIMESTAMP:
if (value == null) {
unsetLastHeartbeatTimestamp();
} else {
setLastHeartbeatTimestamp((Long)value);
}
break;
case LAST_STARTED_TIMESTAMP:
if (value == null) {
unsetLastStartedTimestamp();
} else {
setLastStartedTimestamp((Long)value);
}
break;
case ATTEMPT:
if (value == null) {
unsetAttempt();
} else {
setAttempt((Integer)value);
}
break;
case MAXIMUM_ATTEMPTS:
if (value == null) {
unsetMaximumAttempts();
} else {
setMaximumAttempts((Integer)value);
}
break;
case SCHEDULED_TIMESTAMP:
if (value == null) {
unsetScheduledTimestamp();
} else {
setScheduledTimestamp((Long)value);
}
break;
case EXPIRATION_TIMESTAMP:
if (value == null) {
unsetExpirationTimestamp();
} else {
setExpirationTimestamp((Long)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case ACTIVITY_ID:
return getActivityID();
case ACTIVITY_TYPE:
return getActivityType();
case STATE:
return getState();
case HEARTBEAT_DETAILS:
return getHeartbeatDetails();
case LAST_HEARTBEAT_TIMESTAMP:
return getLastHeartbeatTimestamp();
case LAST_STARTED_TIMESTAMP:
return getLastStartedTimestamp();
case ATTEMPT:
return getAttempt();
case MAXIMUM_ATTEMPTS:
return getMaximumAttempts();
case SCHEDULED_TIMESTAMP:
return getScheduledTimestamp();
case EXPIRATION_TIMESTAMP:
return getExpirationTimestamp();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case ACTIVITY_ID:
return isSetActivityID();
case ACTIVITY_TYPE:
return isSetActivityType();
case STATE:
return isSetState();
case HEARTBEAT_DETAILS:
return isSetHeartbeatDetails();
case LAST_HEARTBEAT_TIMESTAMP:
return isSetLastHeartbeatTimestamp();
case LAST_STARTED_TIMESTAMP:
return isSetLastStartedTimestamp();
case ATTEMPT:
return isSetAttempt();
case MAXIMUM_ATTEMPTS:
return isSetMaximumAttempts();
case SCHEDULED_TIMESTAMP:
return isSetScheduledTimestamp();
case EXPIRATION_TIMESTAMP:
return isSetExpirationTimestamp();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof PendingActivityInfo)
return this.equals((PendingActivityInfo)that);
return false;
}
public boolean equals(PendingActivityInfo that) {
if (that == null)
return false;
boolean this_present_activityID = true && this.isSetActivityID();
boolean that_present_activityID = true && that.isSetActivityID();
if (this_present_activityID || that_present_activityID) {
if (!(this_present_activityID && that_present_activityID))
return false;
if (!this.activityID.equals(that.activityID))
return false;
}
boolean this_present_activityType = true && this.isSetActivityType();
boolean that_present_activityType = true && that.isSetActivityType();
if (this_present_activityType || that_present_activityType) {
if (!(this_present_activityType && that_present_activityType))
return false;
if (!this.activityType.equals(that.activityType))
return false;
}
boolean this_present_state = true && this.isSetState();
boolean that_present_state = true && that.isSetState();
if (this_present_state || that_present_state) {
if (!(this_present_state && that_present_state))
return false;
if (!this.state.equals(that.state))
return false;
}
boolean this_present_heartbeatDetails = true && this.isSetHeartbeatDetails();
boolean that_present_heartbeatDetails = true && that.isSetHeartbeatDetails();
if (this_present_heartbeatDetails || that_present_heartbeatDetails) {
if (!(this_present_heartbeatDetails && that_present_heartbeatDetails))
return false;
if (!this.heartbeatDetails.equals(that.heartbeatDetails))
return false;
}
boolean this_present_lastHeartbeatTimestamp = true && this.isSetLastHeartbeatTimestamp();
boolean that_present_lastHeartbeatTimestamp = true && that.isSetLastHeartbeatTimestamp();
if (this_present_lastHeartbeatTimestamp || that_present_lastHeartbeatTimestamp) {
if (!(this_present_lastHeartbeatTimestamp && that_present_lastHeartbeatTimestamp))
return false;
if (this.lastHeartbeatTimestamp != that.lastHeartbeatTimestamp)
return false;
}
boolean this_present_lastStartedTimestamp = true && this.isSetLastStartedTimestamp();
boolean that_present_lastStartedTimestamp = true && that.isSetLastStartedTimestamp();
if (this_present_lastStartedTimestamp || that_present_lastStartedTimestamp) {
if (!(this_present_lastStartedTimestamp && that_present_lastStartedTimestamp))
return false;
if (this.lastStartedTimestamp != that.lastStartedTimestamp)
return false;
}
boolean this_present_attempt = true && this.isSetAttempt();
boolean that_present_attempt = true && that.isSetAttempt();
if (this_present_attempt || that_present_attempt) {
if (!(this_present_attempt && that_present_attempt))
return false;
if (this.attempt != that.attempt)
return false;
}
boolean this_present_maximumAttempts = true && this.isSetMaximumAttempts();
boolean that_present_maximumAttempts = true && that.isSetMaximumAttempts();
if (this_present_maximumAttempts || that_present_maximumAttempts) {
if (!(this_present_maximumAttempts && that_present_maximumAttempts))
return false;
if (this.maximumAttempts != that.maximumAttempts)
return false;
}
boolean this_present_scheduledTimestamp = true && this.isSetScheduledTimestamp();
boolean that_present_scheduledTimestamp = true && that.isSetScheduledTimestamp();
if (this_present_scheduledTimestamp || that_present_scheduledTimestamp) {
if (!(this_present_scheduledTimestamp && that_present_scheduledTimestamp))
return false;
if (this.scheduledTimestamp != that.scheduledTimestamp)
return false;
}
boolean this_present_expirationTimestamp = true && this.isSetExpirationTimestamp();
boolean that_present_expirationTimestamp = true && that.isSetExpirationTimestamp();
if (this_present_expirationTimestamp || that_present_expirationTimestamp) {
if (!(this_present_expirationTimestamp && that_present_expirationTimestamp))
return false;
if (this.expirationTimestamp != that.expirationTimestamp)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy