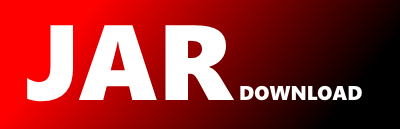
com.uber.cadence.PollForDecisionTaskResponse Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class PollForDecisionTaskResponse implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("PollForDecisionTaskResponse");
private static final org.apache.thrift.protocol.TField TASK_TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("taskToken", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField WORKFLOW_EXECUTION_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowExecution", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField WORKFLOW_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowType", org.apache.thrift.protocol.TType.STRUCT, (short)30);
private static final org.apache.thrift.protocol.TField PREVIOUS_STARTED_EVENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("previousStartedEventId", org.apache.thrift.protocol.TType.I64, (short)40);
private static final org.apache.thrift.protocol.TField STARTED_EVENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("startedEventId", org.apache.thrift.protocol.TType.I64, (short)50);
private static final org.apache.thrift.protocol.TField ATTEMPT_FIELD_DESC = new org.apache.thrift.protocol.TField("attempt", org.apache.thrift.protocol.TType.I64, (short)51);
private static final org.apache.thrift.protocol.TField BACKLOG_COUNT_HINT_FIELD_DESC = new org.apache.thrift.protocol.TField("backlogCountHint", org.apache.thrift.protocol.TType.I64, (short)54);
private static final org.apache.thrift.protocol.TField HISTORY_FIELD_DESC = new org.apache.thrift.protocol.TField("history", org.apache.thrift.protocol.TType.STRUCT, (short)60);
private static final org.apache.thrift.protocol.TField NEXT_PAGE_TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("nextPageToken", org.apache.thrift.protocol.TType.STRING, (short)70);
private static final org.apache.thrift.protocol.TField QUERY_FIELD_DESC = new org.apache.thrift.protocol.TField("query", org.apache.thrift.protocol.TType.STRUCT, (short)80);
private static final org.apache.thrift.protocol.TField WORKFLOW_EXECUTION_TASK_LIST_FIELD_DESC = new org.apache.thrift.protocol.TField("WorkflowExecutionTaskList", org.apache.thrift.protocol.TType.STRUCT, (short)90);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new PollForDecisionTaskResponseStandardSchemeFactory());
schemes.put(TupleScheme.class, new PollForDecisionTaskResponseTupleSchemeFactory());
}
public ByteBuffer taskToken; // optional
public WorkflowExecution workflowExecution; // optional
public WorkflowType workflowType; // optional
public long previousStartedEventId; // optional
public long startedEventId; // optional
public long attempt; // optional
public long backlogCountHint; // optional
public History history; // optional
public ByteBuffer nextPageToken; // optional
public WorkflowQuery query; // optional
public TaskList WorkflowExecutionTaskList; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
TASK_TOKEN((short)10, "taskToken"),
WORKFLOW_EXECUTION((short)20, "workflowExecution"),
WORKFLOW_TYPE((short)30, "workflowType"),
PREVIOUS_STARTED_EVENT_ID((short)40, "previousStartedEventId"),
STARTED_EVENT_ID((short)50, "startedEventId"),
ATTEMPT((short)51, "attempt"),
BACKLOG_COUNT_HINT((short)54, "backlogCountHint"),
HISTORY((short)60, "history"),
NEXT_PAGE_TOKEN((short)70, "nextPageToken"),
QUERY((short)80, "query"),
WORKFLOW_EXECUTION_TASK_LIST((short)90, "WorkflowExecutionTaskList");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // TASK_TOKEN
return TASK_TOKEN;
case 20: // WORKFLOW_EXECUTION
return WORKFLOW_EXECUTION;
case 30: // WORKFLOW_TYPE
return WORKFLOW_TYPE;
case 40: // PREVIOUS_STARTED_EVENT_ID
return PREVIOUS_STARTED_EVENT_ID;
case 50: // STARTED_EVENT_ID
return STARTED_EVENT_ID;
case 51: // ATTEMPT
return ATTEMPT;
case 54: // BACKLOG_COUNT_HINT
return BACKLOG_COUNT_HINT;
case 60: // HISTORY
return HISTORY;
case 70: // NEXT_PAGE_TOKEN
return NEXT_PAGE_TOKEN;
case 80: // QUERY
return QUERY;
case 90: // WORKFLOW_EXECUTION_TASK_LIST
return WORKFLOW_EXECUTION_TASK_LIST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __PREVIOUSSTARTEDEVENTID_ISSET_ID = 0;
private static final int __STARTEDEVENTID_ISSET_ID = 1;
private static final int __ATTEMPT_ISSET_ID = 2;
private static final int __BACKLOGCOUNTHINT_ISSET_ID = 3;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.TASK_TOKEN,_Fields.WORKFLOW_EXECUTION,_Fields.WORKFLOW_TYPE,_Fields.PREVIOUS_STARTED_EVENT_ID,_Fields.STARTED_EVENT_ID,_Fields.ATTEMPT,_Fields.BACKLOG_COUNT_HINT,_Fields.HISTORY,_Fields.NEXT_PAGE_TOKEN,_Fields.QUERY,_Fields.WORKFLOW_EXECUTION_TASK_LIST};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TASK_TOKEN, new org.apache.thrift.meta_data.FieldMetaData("taskToken", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.WORKFLOW_EXECUTION, new org.apache.thrift.meta_data.FieldMetaData("workflowExecution", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecution.class)));
tmpMap.put(_Fields.WORKFLOW_TYPE, new org.apache.thrift.meta_data.FieldMetaData("workflowType", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowType.class)));
tmpMap.put(_Fields.PREVIOUS_STARTED_EVENT_ID, new org.apache.thrift.meta_data.FieldMetaData("previousStartedEventId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.STARTED_EVENT_ID, new org.apache.thrift.meta_data.FieldMetaData("startedEventId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.ATTEMPT, new org.apache.thrift.meta_data.FieldMetaData("attempt", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.BACKLOG_COUNT_HINT, new org.apache.thrift.meta_data.FieldMetaData("backlogCountHint", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.HISTORY, new org.apache.thrift.meta_data.FieldMetaData("history", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, History.class)));
tmpMap.put(_Fields.NEXT_PAGE_TOKEN, new org.apache.thrift.meta_data.FieldMetaData("nextPageToken", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.QUERY, new org.apache.thrift.meta_data.FieldMetaData("query", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "WorkflowQuery")));
tmpMap.put(_Fields.WORKFLOW_EXECUTION_TASK_LIST, new org.apache.thrift.meta_data.FieldMetaData("WorkflowExecutionTaskList", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TaskList.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(PollForDecisionTaskResponse.class, metaDataMap);
}
public PollForDecisionTaskResponse() {
}
/**
* Performs a deep copy on other.
*/
public PollForDecisionTaskResponse(PollForDecisionTaskResponse other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetTaskToken()) {
this.taskToken = org.apache.thrift.TBaseHelper.copyBinary(other.taskToken);
}
if (other.isSetWorkflowExecution()) {
this.workflowExecution = new WorkflowExecution(other.workflowExecution);
}
if (other.isSetWorkflowType()) {
this.workflowType = new WorkflowType(other.workflowType);
}
this.previousStartedEventId = other.previousStartedEventId;
this.startedEventId = other.startedEventId;
this.attempt = other.attempt;
this.backlogCountHint = other.backlogCountHint;
if (other.isSetHistory()) {
this.history = new History(other.history);
}
if (other.isSetNextPageToken()) {
this.nextPageToken = org.apache.thrift.TBaseHelper.copyBinary(other.nextPageToken);
}
if (other.isSetQuery()) {
this.query = other.query;
}
if (other.isSetWorkflowExecutionTaskList()) {
this.WorkflowExecutionTaskList = new TaskList(other.WorkflowExecutionTaskList);
}
}
public PollForDecisionTaskResponse deepCopy() {
return new PollForDecisionTaskResponse(this);
}
@Override
public void clear() {
this.taskToken = null;
this.workflowExecution = null;
this.workflowType = null;
setPreviousStartedEventIdIsSet(false);
this.previousStartedEventId = 0;
setStartedEventIdIsSet(false);
this.startedEventId = 0;
setAttemptIsSet(false);
this.attempt = 0;
setBacklogCountHintIsSet(false);
this.backlogCountHint = 0;
this.history = null;
this.nextPageToken = null;
this.query = null;
this.WorkflowExecutionTaskList = null;
}
public byte[] getTaskToken() {
setTaskToken(org.apache.thrift.TBaseHelper.rightSize(taskToken));
return taskToken == null ? null : taskToken.array();
}
public ByteBuffer bufferForTaskToken() {
return org.apache.thrift.TBaseHelper.copyBinary(taskToken);
}
public PollForDecisionTaskResponse setTaskToken(byte[] taskToken) {
this.taskToken = taskToken == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(taskToken, taskToken.length));
return this;
}
public PollForDecisionTaskResponse setTaskToken(ByteBuffer taskToken) {
this.taskToken = org.apache.thrift.TBaseHelper.copyBinary(taskToken);
return this;
}
public void unsetTaskToken() {
this.taskToken = null;
}
/** Returns true if field taskToken is set (has been assigned a value) and false otherwise */
public boolean isSetTaskToken() {
return this.taskToken != null;
}
public void setTaskTokenIsSet(boolean value) {
if (!value) {
this.taskToken = null;
}
}
public WorkflowExecution getWorkflowExecution() {
return this.workflowExecution;
}
public PollForDecisionTaskResponse setWorkflowExecution(WorkflowExecution workflowExecution) {
this.workflowExecution = workflowExecution;
return this;
}
public void unsetWorkflowExecution() {
this.workflowExecution = null;
}
/** Returns true if field workflowExecution is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowExecution() {
return this.workflowExecution != null;
}
public void setWorkflowExecutionIsSet(boolean value) {
if (!value) {
this.workflowExecution = null;
}
}
public WorkflowType getWorkflowType() {
return this.workflowType;
}
public PollForDecisionTaskResponse setWorkflowType(WorkflowType workflowType) {
this.workflowType = workflowType;
return this;
}
public void unsetWorkflowType() {
this.workflowType = null;
}
/** Returns true if field workflowType is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowType() {
return this.workflowType != null;
}
public void setWorkflowTypeIsSet(boolean value) {
if (!value) {
this.workflowType = null;
}
}
public long getPreviousStartedEventId() {
return this.previousStartedEventId;
}
public PollForDecisionTaskResponse setPreviousStartedEventId(long previousStartedEventId) {
this.previousStartedEventId = previousStartedEventId;
setPreviousStartedEventIdIsSet(true);
return this;
}
public void unsetPreviousStartedEventId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __PREVIOUSSTARTEDEVENTID_ISSET_ID);
}
/** Returns true if field previousStartedEventId is set (has been assigned a value) and false otherwise */
public boolean isSetPreviousStartedEventId() {
return EncodingUtils.testBit(__isset_bitfield, __PREVIOUSSTARTEDEVENTID_ISSET_ID);
}
public void setPreviousStartedEventIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __PREVIOUSSTARTEDEVENTID_ISSET_ID, value);
}
public long getStartedEventId() {
return this.startedEventId;
}
public PollForDecisionTaskResponse setStartedEventId(long startedEventId) {
this.startedEventId = startedEventId;
setStartedEventIdIsSet(true);
return this;
}
public void unsetStartedEventId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __STARTEDEVENTID_ISSET_ID);
}
/** Returns true if field startedEventId is set (has been assigned a value) and false otherwise */
public boolean isSetStartedEventId() {
return EncodingUtils.testBit(__isset_bitfield, __STARTEDEVENTID_ISSET_ID);
}
public void setStartedEventIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __STARTEDEVENTID_ISSET_ID, value);
}
public long getAttempt() {
return this.attempt;
}
public PollForDecisionTaskResponse setAttempt(long attempt) {
this.attempt = attempt;
setAttemptIsSet(true);
return this;
}
public void unsetAttempt() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __ATTEMPT_ISSET_ID);
}
/** Returns true if field attempt is set (has been assigned a value) and false otherwise */
public boolean isSetAttempt() {
return EncodingUtils.testBit(__isset_bitfield, __ATTEMPT_ISSET_ID);
}
public void setAttemptIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __ATTEMPT_ISSET_ID, value);
}
public long getBacklogCountHint() {
return this.backlogCountHint;
}
public PollForDecisionTaskResponse setBacklogCountHint(long backlogCountHint) {
this.backlogCountHint = backlogCountHint;
setBacklogCountHintIsSet(true);
return this;
}
public void unsetBacklogCountHint() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __BACKLOGCOUNTHINT_ISSET_ID);
}
/** Returns true if field backlogCountHint is set (has been assigned a value) and false otherwise */
public boolean isSetBacklogCountHint() {
return EncodingUtils.testBit(__isset_bitfield, __BACKLOGCOUNTHINT_ISSET_ID);
}
public void setBacklogCountHintIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __BACKLOGCOUNTHINT_ISSET_ID, value);
}
public History getHistory() {
return this.history;
}
public PollForDecisionTaskResponse setHistory(History history) {
this.history = history;
return this;
}
public void unsetHistory() {
this.history = null;
}
/** Returns true if field history is set (has been assigned a value) and false otherwise */
public boolean isSetHistory() {
return this.history != null;
}
public void setHistoryIsSet(boolean value) {
if (!value) {
this.history = null;
}
}
public byte[] getNextPageToken() {
setNextPageToken(org.apache.thrift.TBaseHelper.rightSize(nextPageToken));
return nextPageToken == null ? null : nextPageToken.array();
}
public ByteBuffer bufferForNextPageToken() {
return org.apache.thrift.TBaseHelper.copyBinary(nextPageToken);
}
public PollForDecisionTaskResponse setNextPageToken(byte[] nextPageToken) {
this.nextPageToken = nextPageToken == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(nextPageToken, nextPageToken.length));
return this;
}
public PollForDecisionTaskResponse setNextPageToken(ByteBuffer nextPageToken) {
this.nextPageToken = org.apache.thrift.TBaseHelper.copyBinary(nextPageToken);
return this;
}
public void unsetNextPageToken() {
this.nextPageToken = null;
}
/** Returns true if field nextPageToken is set (has been assigned a value) and false otherwise */
public boolean isSetNextPageToken() {
return this.nextPageToken != null;
}
public void setNextPageTokenIsSet(boolean value) {
if (!value) {
this.nextPageToken = null;
}
}
public WorkflowQuery getQuery() {
return this.query;
}
public PollForDecisionTaskResponse setQuery(WorkflowQuery query) {
this.query = query;
return this;
}
public void unsetQuery() {
this.query = null;
}
/** Returns true if field query is set (has been assigned a value) and false otherwise */
public boolean isSetQuery() {
return this.query != null;
}
public void setQueryIsSet(boolean value) {
if (!value) {
this.query = null;
}
}
public TaskList getWorkflowExecutionTaskList() {
return this.WorkflowExecutionTaskList;
}
public PollForDecisionTaskResponse setWorkflowExecutionTaskList(TaskList WorkflowExecutionTaskList) {
this.WorkflowExecutionTaskList = WorkflowExecutionTaskList;
return this;
}
public void unsetWorkflowExecutionTaskList() {
this.WorkflowExecutionTaskList = null;
}
/** Returns true if field WorkflowExecutionTaskList is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowExecutionTaskList() {
return this.WorkflowExecutionTaskList != null;
}
public void setWorkflowExecutionTaskListIsSet(boolean value) {
if (!value) {
this.WorkflowExecutionTaskList = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case TASK_TOKEN:
if (value == null) {
unsetTaskToken();
} else {
setTaskToken((ByteBuffer)value);
}
break;
case WORKFLOW_EXECUTION:
if (value == null) {
unsetWorkflowExecution();
} else {
setWorkflowExecution((WorkflowExecution)value);
}
break;
case WORKFLOW_TYPE:
if (value == null) {
unsetWorkflowType();
} else {
setWorkflowType((WorkflowType)value);
}
break;
case PREVIOUS_STARTED_EVENT_ID:
if (value == null) {
unsetPreviousStartedEventId();
} else {
setPreviousStartedEventId((Long)value);
}
break;
case STARTED_EVENT_ID:
if (value == null) {
unsetStartedEventId();
} else {
setStartedEventId((Long)value);
}
break;
case ATTEMPT:
if (value == null) {
unsetAttempt();
} else {
setAttempt((Long)value);
}
break;
case BACKLOG_COUNT_HINT:
if (value == null) {
unsetBacklogCountHint();
} else {
setBacklogCountHint((Long)value);
}
break;
case HISTORY:
if (value == null) {
unsetHistory();
} else {
setHistory((History)value);
}
break;
case NEXT_PAGE_TOKEN:
if (value == null) {
unsetNextPageToken();
} else {
setNextPageToken((ByteBuffer)value);
}
break;
case QUERY:
if (value == null) {
unsetQuery();
} else {
setQuery((WorkflowQuery)value);
}
break;
case WORKFLOW_EXECUTION_TASK_LIST:
if (value == null) {
unsetWorkflowExecutionTaskList();
} else {
setWorkflowExecutionTaskList((TaskList)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case TASK_TOKEN:
return getTaskToken();
case WORKFLOW_EXECUTION:
return getWorkflowExecution();
case WORKFLOW_TYPE:
return getWorkflowType();
case PREVIOUS_STARTED_EVENT_ID:
return getPreviousStartedEventId();
case STARTED_EVENT_ID:
return getStartedEventId();
case ATTEMPT:
return getAttempt();
case BACKLOG_COUNT_HINT:
return getBacklogCountHint();
case HISTORY:
return getHistory();
case NEXT_PAGE_TOKEN:
return getNextPageToken();
case QUERY:
return getQuery();
case WORKFLOW_EXECUTION_TASK_LIST:
return getWorkflowExecutionTaskList();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case TASK_TOKEN:
return isSetTaskToken();
case WORKFLOW_EXECUTION:
return isSetWorkflowExecution();
case WORKFLOW_TYPE:
return isSetWorkflowType();
case PREVIOUS_STARTED_EVENT_ID:
return isSetPreviousStartedEventId();
case STARTED_EVENT_ID:
return isSetStartedEventId();
case ATTEMPT:
return isSetAttempt();
case BACKLOG_COUNT_HINT:
return isSetBacklogCountHint();
case HISTORY:
return isSetHistory();
case NEXT_PAGE_TOKEN:
return isSetNextPageToken();
case QUERY:
return isSetQuery();
case WORKFLOW_EXECUTION_TASK_LIST:
return isSetWorkflowExecutionTaskList();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof PollForDecisionTaskResponse)
return this.equals((PollForDecisionTaskResponse)that);
return false;
}
public boolean equals(PollForDecisionTaskResponse that) {
if (that == null)
return false;
boolean this_present_taskToken = true && this.isSetTaskToken();
boolean that_present_taskToken = true && that.isSetTaskToken();
if (this_present_taskToken || that_present_taskToken) {
if (!(this_present_taskToken && that_present_taskToken))
return false;
if (!this.taskToken.equals(that.taskToken))
return false;
}
boolean this_present_workflowExecution = true && this.isSetWorkflowExecution();
boolean that_present_workflowExecution = true && that.isSetWorkflowExecution();
if (this_present_workflowExecution || that_present_workflowExecution) {
if (!(this_present_workflowExecution && that_present_workflowExecution))
return false;
if (!this.workflowExecution.equals(that.workflowExecution))
return false;
}
boolean this_present_workflowType = true && this.isSetWorkflowType();
boolean that_present_workflowType = true && that.isSetWorkflowType();
if (this_present_workflowType || that_present_workflowType) {
if (!(this_present_workflowType && that_present_workflowType))
return false;
if (!this.workflowType.equals(that.workflowType))
return false;
}
boolean this_present_previousStartedEventId = true && this.isSetPreviousStartedEventId();
boolean that_present_previousStartedEventId = true && that.isSetPreviousStartedEventId();
if (this_present_previousStartedEventId || that_present_previousStartedEventId) {
if (!(this_present_previousStartedEventId && that_present_previousStartedEventId))
return false;
if (this.previousStartedEventId != that.previousStartedEventId)
return false;
}
boolean this_present_startedEventId = true && this.isSetStartedEventId();
boolean that_present_startedEventId = true && that.isSetStartedEventId();
if (this_present_startedEventId || that_present_startedEventId) {
if (!(this_present_startedEventId && that_present_startedEventId))
return false;
if (this.startedEventId != that.startedEventId)
return false;
}
boolean this_present_attempt = true && this.isSetAttempt();
boolean that_present_attempt = true && that.isSetAttempt();
if (this_present_attempt || that_present_attempt) {
if (!(this_present_attempt && that_present_attempt))
return false;
if (this.attempt != that.attempt)
return false;
}
boolean this_present_backlogCountHint = true && this.isSetBacklogCountHint();
boolean that_present_backlogCountHint = true && that.isSetBacklogCountHint();
if (this_present_backlogCountHint || that_present_backlogCountHint) {
if (!(this_present_backlogCountHint && that_present_backlogCountHint))
return false;
if (this.backlogCountHint != that.backlogCountHint)
return false;
}
boolean this_present_history = true && this.isSetHistory();
boolean that_present_history = true && that.isSetHistory();
if (this_present_history || that_present_history) {
if (!(this_present_history && that_present_history))
return false;
if (!this.history.equals(that.history))
return false;
}
boolean this_present_nextPageToken = true && this.isSetNextPageToken();
boolean that_present_nextPageToken = true && that.isSetNextPageToken();
if (this_present_nextPageToken || that_present_nextPageToken) {
if (!(this_present_nextPageToken && that_present_nextPageToken))
return false;
if (!this.nextPageToken.equals(that.nextPageToken))
return false;
}
boolean this_present_query = true && this.isSetQuery();
boolean that_present_query = true && that.isSetQuery();
if (this_present_query || that_present_query) {
if (!(this_present_query && that_present_query))
return false;
if (!this.query.equals(that.query))
return false;
}
boolean this_present_WorkflowExecutionTaskList = true && this.isSetWorkflowExecutionTaskList();
boolean that_present_WorkflowExecutionTaskList = true && that.isSetWorkflowExecutionTaskList();
if (this_present_WorkflowExecutionTaskList || that_present_WorkflowExecutionTaskList) {
if (!(this_present_WorkflowExecutionTaskList && that_present_WorkflowExecutionTaskList))
return false;
if (!this.WorkflowExecutionTaskList.equals(that.WorkflowExecutionTaskList))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy