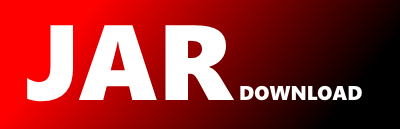
com.uber.cadence.QueryWorkflowRequest Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class QueryWorkflowRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("QueryWorkflowRequest");
private static final org.apache.thrift.protocol.TField DOMAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("domain", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField EXECUTION_FIELD_DESC = new org.apache.thrift.protocol.TField("execution", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField QUERY_FIELD_DESC = new org.apache.thrift.protocol.TField("query", org.apache.thrift.protocol.TType.STRUCT, (short)30);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new QueryWorkflowRequestStandardSchemeFactory());
schemes.put(TupleScheme.class, new QueryWorkflowRequestTupleSchemeFactory());
}
public String domain; // optional
public WorkflowExecution execution; // optional
public WorkflowQuery query; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
DOMAIN((short)10, "domain"),
EXECUTION((short)20, "execution"),
QUERY((short)30, "query");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // DOMAIN
return DOMAIN;
case 20: // EXECUTION
return EXECUTION;
case 30: // QUERY
return QUERY;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.DOMAIN,_Fields.EXECUTION,_Fields.QUERY};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.DOMAIN, new org.apache.thrift.meta_data.FieldMetaData("domain", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.EXECUTION, new org.apache.thrift.meta_data.FieldMetaData("execution", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecution.class)));
tmpMap.put(_Fields.QUERY, new org.apache.thrift.meta_data.FieldMetaData("query", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "WorkflowQuery")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(QueryWorkflowRequest.class, metaDataMap);
}
public QueryWorkflowRequest() {
}
/**
* Performs a deep copy on other.
*/
public QueryWorkflowRequest(QueryWorkflowRequest other) {
if (other.isSetDomain()) {
this.domain = other.domain;
}
if (other.isSetExecution()) {
this.execution = new WorkflowExecution(other.execution);
}
if (other.isSetQuery()) {
this.query = other.query;
}
}
public QueryWorkflowRequest deepCopy() {
return new QueryWorkflowRequest(this);
}
@Override
public void clear() {
this.domain = null;
this.execution = null;
this.query = null;
}
public String getDomain() {
return this.domain;
}
public QueryWorkflowRequest setDomain(String domain) {
this.domain = domain;
return this;
}
public void unsetDomain() {
this.domain = null;
}
/** Returns true if field domain is set (has been assigned a value) and false otherwise */
public boolean isSetDomain() {
return this.domain != null;
}
public void setDomainIsSet(boolean value) {
if (!value) {
this.domain = null;
}
}
public WorkflowExecution getExecution() {
return this.execution;
}
public QueryWorkflowRequest setExecution(WorkflowExecution execution) {
this.execution = execution;
return this;
}
public void unsetExecution() {
this.execution = null;
}
/** Returns true if field execution is set (has been assigned a value) and false otherwise */
public boolean isSetExecution() {
return this.execution != null;
}
public void setExecutionIsSet(boolean value) {
if (!value) {
this.execution = null;
}
}
public WorkflowQuery getQuery() {
return this.query;
}
public QueryWorkflowRequest setQuery(WorkflowQuery query) {
this.query = query;
return this;
}
public void unsetQuery() {
this.query = null;
}
/** Returns true if field query is set (has been assigned a value) and false otherwise */
public boolean isSetQuery() {
return this.query != null;
}
public void setQueryIsSet(boolean value) {
if (!value) {
this.query = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case DOMAIN:
if (value == null) {
unsetDomain();
} else {
setDomain((String)value);
}
break;
case EXECUTION:
if (value == null) {
unsetExecution();
} else {
setExecution((WorkflowExecution)value);
}
break;
case QUERY:
if (value == null) {
unsetQuery();
} else {
setQuery((WorkflowQuery)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case DOMAIN:
return getDomain();
case EXECUTION:
return getExecution();
case QUERY:
return getQuery();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case DOMAIN:
return isSetDomain();
case EXECUTION:
return isSetExecution();
case QUERY:
return isSetQuery();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof QueryWorkflowRequest)
return this.equals((QueryWorkflowRequest)that);
return false;
}
public boolean equals(QueryWorkflowRequest that) {
if (that == null)
return false;
boolean this_present_domain = true && this.isSetDomain();
boolean that_present_domain = true && that.isSetDomain();
if (this_present_domain || that_present_domain) {
if (!(this_present_domain && that_present_domain))
return false;
if (!this.domain.equals(that.domain))
return false;
}
boolean this_present_execution = true && this.isSetExecution();
boolean that_present_execution = true && that.isSetExecution();
if (this_present_execution || that_present_execution) {
if (!(this_present_execution && that_present_execution))
return false;
if (!this.execution.equals(that.execution))
return false;
}
boolean this_present_query = true && this.isSetQuery();
boolean that_present_query = true && that.isSetQuery();
if (this_present_query || that_present_query) {
if (!(this_present_query && that_present_query))
return false;
if (!this.query.equals(that.query))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy