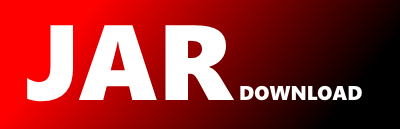
com.uber.cadence.RetryPolicy Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class RetryPolicy implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("RetryPolicy");
private static final org.apache.thrift.protocol.TField INITIAL_INTERVAL_IN_SECONDS_FIELD_DESC = new org.apache.thrift.protocol.TField("initialIntervalInSeconds", org.apache.thrift.protocol.TType.I32, (short)10);
private static final org.apache.thrift.protocol.TField BACKOFF_COEFFICIENT_FIELD_DESC = new org.apache.thrift.protocol.TField("backoffCoefficient", org.apache.thrift.protocol.TType.DOUBLE, (short)20);
private static final org.apache.thrift.protocol.TField MAXIMUM_INTERVAL_IN_SECONDS_FIELD_DESC = new org.apache.thrift.protocol.TField("maximumIntervalInSeconds", org.apache.thrift.protocol.TType.I32, (short)30);
private static final org.apache.thrift.protocol.TField MAXIMUM_ATTEMPTS_FIELD_DESC = new org.apache.thrift.protocol.TField("maximumAttempts", org.apache.thrift.protocol.TType.I32, (short)40);
private static final org.apache.thrift.protocol.TField NON_RETRIABLE_ERROR_REASONS_FIELD_DESC = new org.apache.thrift.protocol.TField("nonRetriableErrorReasons", org.apache.thrift.protocol.TType.LIST, (short)50);
private static final org.apache.thrift.protocol.TField EXPIRATION_INTERVAL_IN_SECONDS_FIELD_DESC = new org.apache.thrift.protocol.TField("expirationIntervalInSeconds", org.apache.thrift.protocol.TType.I32, (short)60);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new RetryPolicyStandardSchemeFactory());
schemes.put(TupleScheme.class, new RetryPolicyTupleSchemeFactory());
}
public int initialIntervalInSeconds; // optional
public double backoffCoefficient; // optional
public int maximumIntervalInSeconds; // optional
public int maximumAttempts; // optional
public List nonRetriableErrorReasons; // optional
public int expirationIntervalInSeconds; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
INITIAL_INTERVAL_IN_SECONDS((short)10, "initialIntervalInSeconds"),
BACKOFF_COEFFICIENT((short)20, "backoffCoefficient"),
MAXIMUM_INTERVAL_IN_SECONDS((short)30, "maximumIntervalInSeconds"),
MAXIMUM_ATTEMPTS((short)40, "maximumAttempts"),
NON_RETRIABLE_ERROR_REASONS((short)50, "nonRetriableErrorReasons"),
EXPIRATION_INTERVAL_IN_SECONDS((short)60, "expirationIntervalInSeconds");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // INITIAL_INTERVAL_IN_SECONDS
return INITIAL_INTERVAL_IN_SECONDS;
case 20: // BACKOFF_COEFFICIENT
return BACKOFF_COEFFICIENT;
case 30: // MAXIMUM_INTERVAL_IN_SECONDS
return MAXIMUM_INTERVAL_IN_SECONDS;
case 40: // MAXIMUM_ATTEMPTS
return MAXIMUM_ATTEMPTS;
case 50: // NON_RETRIABLE_ERROR_REASONS
return NON_RETRIABLE_ERROR_REASONS;
case 60: // EXPIRATION_INTERVAL_IN_SECONDS
return EXPIRATION_INTERVAL_IN_SECONDS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __INITIALINTERVALINSECONDS_ISSET_ID = 0;
private static final int __BACKOFFCOEFFICIENT_ISSET_ID = 1;
private static final int __MAXIMUMINTERVALINSECONDS_ISSET_ID = 2;
private static final int __MAXIMUMATTEMPTS_ISSET_ID = 3;
private static final int __EXPIRATIONINTERVALINSECONDS_ISSET_ID = 4;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.INITIAL_INTERVAL_IN_SECONDS,_Fields.BACKOFF_COEFFICIENT,_Fields.MAXIMUM_INTERVAL_IN_SECONDS,_Fields.MAXIMUM_ATTEMPTS,_Fields.NON_RETRIABLE_ERROR_REASONS,_Fields.EXPIRATION_INTERVAL_IN_SECONDS};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.INITIAL_INTERVAL_IN_SECONDS, new org.apache.thrift.meta_data.FieldMetaData("initialIntervalInSeconds", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.BACKOFF_COEFFICIENT, new org.apache.thrift.meta_data.FieldMetaData("backoffCoefficient", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.DOUBLE)));
tmpMap.put(_Fields.MAXIMUM_INTERVAL_IN_SECONDS, new org.apache.thrift.meta_data.FieldMetaData("maximumIntervalInSeconds", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.MAXIMUM_ATTEMPTS, new org.apache.thrift.meta_data.FieldMetaData("maximumAttempts", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.NON_RETRIABLE_ERROR_REASONS, new org.apache.thrift.meta_data.FieldMetaData("nonRetriableErrorReasons", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.EXPIRATION_INTERVAL_IN_SECONDS, new org.apache.thrift.meta_data.FieldMetaData("expirationIntervalInSeconds", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(RetryPolicy.class, metaDataMap);
}
public RetryPolicy() {
}
/**
* Performs a deep copy on other.
*/
public RetryPolicy(RetryPolicy other) {
__isset_bitfield = other.__isset_bitfield;
this.initialIntervalInSeconds = other.initialIntervalInSeconds;
this.backoffCoefficient = other.backoffCoefficient;
this.maximumIntervalInSeconds = other.maximumIntervalInSeconds;
this.maximumAttempts = other.maximumAttempts;
if (other.isSetNonRetriableErrorReasons()) {
List __this__nonRetriableErrorReasons = new ArrayList(other.nonRetriableErrorReasons);
this.nonRetriableErrorReasons = __this__nonRetriableErrorReasons;
}
this.expirationIntervalInSeconds = other.expirationIntervalInSeconds;
}
public RetryPolicy deepCopy() {
return new RetryPolicy(this);
}
@Override
public void clear() {
setInitialIntervalInSecondsIsSet(false);
this.initialIntervalInSeconds = 0;
setBackoffCoefficientIsSet(false);
this.backoffCoefficient = 0.0;
setMaximumIntervalInSecondsIsSet(false);
this.maximumIntervalInSeconds = 0;
setMaximumAttemptsIsSet(false);
this.maximumAttempts = 0;
this.nonRetriableErrorReasons = null;
setExpirationIntervalInSecondsIsSet(false);
this.expirationIntervalInSeconds = 0;
}
public int getInitialIntervalInSeconds() {
return this.initialIntervalInSeconds;
}
public RetryPolicy setInitialIntervalInSeconds(int initialIntervalInSeconds) {
this.initialIntervalInSeconds = initialIntervalInSeconds;
setInitialIntervalInSecondsIsSet(true);
return this;
}
public void unsetInitialIntervalInSeconds() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __INITIALINTERVALINSECONDS_ISSET_ID);
}
/** Returns true if field initialIntervalInSeconds is set (has been assigned a value) and false otherwise */
public boolean isSetInitialIntervalInSeconds() {
return EncodingUtils.testBit(__isset_bitfield, __INITIALINTERVALINSECONDS_ISSET_ID);
}
public void setInitialIntervalInSecondsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __INITIALINTERVALINSECONDS_ISSET_ID, value);
}
public double getBackoffCoefficient() {
return this.backoffCoefficient;
}
public RetryPolicy setBackoffCoefficient(double backoffCoefficient) {
this.backoffCoefficient = backoffCoefficient;
setBackoffCoefficientIsSet(true);
return this;
}
public void unsetBackoffCoefficient() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __BACKOFFCOEFFICIENT_ISSET_ID);
}
/** Returns true if field backoffCoefficient is set (has been assigned a value) and false otherwise */
public boolean isSetBackoffCoefficient() {
return EncodingUtils.testBit(__isset_bitfield, __BACKOFFCOEFFICIENT_ISSET_ID);
}
public void setBackoffCoefficientIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __BACKOFFCOEFFICIENT_ISSET_ID, value);
}
public int getMaximumIntervalInSeconds() {
return this.maximumIntervalInSeconds;
}
public RetryPolicy setMaximumIntervalInSeconds(int maximumIntervalInSeconds) {
this.maximumIntervalInSeconds = maximumIntervalInSeconds;
setMaximumIntervalInSecondsIsSet(true);
return this;
}
public void unsetMaximumIntervalInSeconds() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __MAXIMUMINTERVALINSECONDS_ISSET_ID);
}
/** Returns true if field maximumIntervalInSeconds is set (has been assigned a value) and false otherwise */
public boolean isSetMaximumIntervalInSeconds() {
return EncodingUtils.testBit(__isset_bitfield, __MAXIMUMINTERVALINSECONDS_ISSET_ID);
}
public void setMaximumIntervalInSecondsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __MAXIMUMINTERVALINSECONDS_ISSET_ID, value);
}
public int getMaximumAttempts() {
return this.maximumAttempts;
}
public RetryPolicy setMaximumAttempts(int maximumAttempts) {
this.maximumAttempts = maximumAttempts;
setMaximumAttemptsIsSet(true);
return this;
}
public void unsetMaximumAttempts() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __MAXIMUMATTEMPTS_ISSET_ID);
}
/** Returns true if field maximumAttempts is set (has been assigned a value) and false otherwise */
public boolean isSetMaximumAttempts() {
return EncodingUtils.testBit(__isset_bitfield, __MAXIMUMATTEMPTS_ISSET_ID);
}
public void setMaximumAttemptsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __MAXIMUMATTEMPTS_ISSET_ID, value);
}
public int getNonRetriableErrorReasonsSize() {
return (this.nonRetriableErrorReasons == null) ? 0 : this.nonRetriableErrorReasons.size();
}
public java.util.Iterator getNonRetriableErrorReasonsIterator() {
return (this.nonRetriableErrorReasons == null) ? null : this.nonRetriableErrorReasons.iterator();
}
public void addToNonRetriableErrorReasons(String elem) {
if (this.nonRetriableErrorReasons == null) {
this.nonRetriableErrorReasons = new ArrayList();
}
this.nonRetriableErrorReasons.add(elem);
}
public List getNonRetriableErrorReasons() {
return this.nonRetriableErrorReasons;
}
public RetryPolicy setNonRetriableErrorReasons(List nonRetriableErrorReasons) {
this.nonRetriableErrorReasons = nonRetriableErrorReasons;
return this;
}
public void unsetNonRetriableErrorReasons() {
this.nonRetriableErrorReasons = null;
}
/** Returns true if field nonRetriableErrorReasons is set (has been assigned a value) and false otherwise */
public boolean isSetNonRetriableErrorReasons() {
return this.nonRetriableErrorReasons != null;
}
public void setNonRetriableErrorReasonsIsSet(boolean value) {
if (!value) {
this.nonRetriableErrorReasons = null;
}
}
public int getExpirationIntervalInSeconds() {
return this.expirationIntervalInSeconds;
}
public RetryPolicy setExpirationIntervalInSeconds(int expirationIntervalInSeconds) {
this.expirationIntervalInSeconds = expirationIntervalInSeconds;
setExpirationIntervalInSecondsIsSet(true);
return this;
}
public void unsetExpirationIntervalInSeconds() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __EXPIRATIONINTERVALINSECONDS_ISSET_ID);
}
/** Returns true if field expirationIntervalInSeconds is set (has been assigned a value) and false otherwise */
public boolean isSetExpirationIntervalInSeconds() {
return EncodingUtils.testBit(__isset_bitfield, __EXPIRATIONINTERVALINSECONDS_ISSET_ID);
}
public void setExpirationIntervalInSecondsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __EXPIRATIONINTERVALINSECONDS_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case INITIAL_INTERVAL_IN_SECONDS:
if (value == null) {
unsetInitialIntervalInSeconds();
} else {
setInitialIntervalInSeconds((Integer)value);
}
break;
case BACKOFF_COEFFICIENT:
if (value == null) {
unsetBackoffCoefficient();
} else {
setBackoffCoefficient((Double)value);
}
break;
case MAXIMUM_INTERVAL_IN_SECONDS:
if (value == null) {
unsetMaximumIntervalInSeconds();
} else {
setMaximumIntervalInSeconds((Integer)value);
}
break;
case MAXIMUM_ATTEMPTS:
if (value == null) {
unsetMaximumAttempts();
} else {
setMaximumAttempts((Integer)value);
}
break;
case NON_RETRIABLE_ERROR_REASONS:
if (value == null) {
unsetNonRetriableErrorReasons();
} else {
setNonRetriableErrorReasons((List)value);
}
break;
case EXPIRATION_INTERVAL_IN_SECONDS:
if (value == null) {
unsetExpirationIntervalInSeconds();
} else {
setExpirationIntervalInSeconds((Integer)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case INITIAL_INTERVAL_IN_SECONDS:
return getInitialIntervalInSeconds();
case BACKOFF_COEFFICIENT:
return getBackoffCoefficient();
case MAXIMUM_INTERVAL_IN_SECONDS:
return getMaximumIntervalInSeconds();
case MAXIMUM_ATTEMPTS:
return getMaximumAttempts();
case NON_RETRIABLE_ERROR_REASONS:
return getNonRetriableErrorReasons();
case EXPIRATION_INTERVAL_IN_SECONDS:
return getExpirationIntervalInSeconds();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case INITIAL_INTERVAL_IN_SECONDS:
return isSetInitialIntervalInSeconds();
case BACKOFF_COEFFICIENT:
return isSetBackoffCoefficient();
case MAXIMUM_INTERVAL_IN_SECONDS:
return isSetMaximumIntervalInSeconds();
case MAXIMUM_ATTEMPTS:
return isSetMaximumAttempts();
case NON_RETRIABLE_ERROR_REASONS:
return isSetNonRetriableErrorReasons();
case EXPIRATION_INTERVAL_IN_SECONDS:
return isSetExpirationIntervalInSeconds();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof RetryPolicy)
return this.equals((RetryPolicy)that);
return false;
}
public boolean equals(RetryPolicy that) {
if (that == null)
return false;
boolean this_present_initialIntervalInSeconds = true && this.isSetInitialIntervalInSeconds();
boolean that_present_initialIntervalInSeconds = true && that.isSetInitialIntervalInSeconds();
if (this_present_initialIntervalInSeconds || that_present_initialIntervalInSeconds) {
if (!(this_present_initialIntervalInSeconds && that_present_initialIntervalInSeconds))
return false;
if (this.initialIntervalInSeconds != that.initialIntervalInSeconds)
return false;
}
boolean this_present_backoffCoefficient = true && this.isSetBackoffCoefficient();
boolean that_present_backoffCoefficient = true && that.isSetBackoffCoefficient();
if (this_present_backoffCoefficient || that_present_backoffCoefficient) {
if (!(this_present_backoffCoefficient && that_present_backoffCoefficient))
return false;
if (this.backoffCoefficient != that.backoffCoefficient)
return false;
}
boolean this_present_maximumIntervalInSeconds = true && this.isSetMaximumIntervalInSeconds();
boolean that_present_maximumIntervalInSeconds = true && that.isSetMaximumIntervalInSeconds();
if (this_present_maximumIntervalInSeconds || that_present_maximumIntervalInSeconds) {
if (!(this_present_maximumIntervalInSeconds && that_present_maximumIntervalInSeconds))
return false;
if (this.maximumIntervalInSeconds != that.maximumIntervalInSeconds)
return false;
}
boolean this_present_maximumAttempts = true && this.isSetMaximumAttempts();
boolean that_present_maximumAttempts = true && that.isSetMaximumAttempts();
if (this_present_maximumAttempts || that_present_maximumAttempts) {
if (!(this_present_maximumAttempts && that_present_maximumAttempts))
return false;
if (this.maximumAttempts != that.maximumAttempts)
return false;
}
boolean this_present_nonRetriableErrorReasons = true && this.isSetNonRetriableErrorReasons();
boolean that_present_nonRetriableErrorReasons = true && that.isSetNonRetriableErrorReasons();
if (this_present_nonRetriableErrorReasons || that_present_nonRetriableErrorReasons) {
if (!(this_present_nonRetriableErrorReasons && that_present_nonRetriableErrorReasons))
return false;
if (!this.nonRetriableErrorReasons.equals(that.nonRetriableErrorReasons))
return false;
}
boolean this_present_expirationIntervalInSeconds = true && this.isSetExpirationIntervalInSeconds();
boolean that_present_expirationIntervalInSeconds = true && that.isSetExpirationIntervalInSeconds();
if (this_present_expirationIntervalInSeconds || that_present_expirationIntervalInSeconds) {
if (!(this_present_expirationIntervalInSeconds && that_present_expirationIntervalInSeconds))
return false;
if (this.expirationIntervalInSeconds != that.expirationIntervalInSeconds)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy