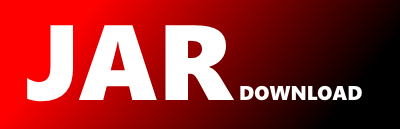
com.uber.cadence.SignalWithStartWorkflowExecutionRequest Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class SignalWithStartWorkflowExecutionRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("SignalWithStartWorkflowExecutionRequest");
private static final org.apache.thrift.protocol.TField DOMAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("domain", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField WORKFLOW_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowId", org.apache.thrift.protocol.TType.STRING, (short)20);
private static final org.apache.thrift.protocol.TField WORKFLOW_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowType", org.apache.thrift.protocol.TType.STRUCT, (short)30);
private static final org.apache.thrift.protocol.TField TASK_LIST_FIELD_DESC = new org.apache.thrift.protocol.TField("taskList", org.apache.thrift.protocol.TType.STRUCT, (short)40);
private static final org.apache.thrift.protocol.TField INPUT_FIELD_DESC = new org.apache.thrift.protocol.TField("input", org.apache.thrift.protocol.TType.STRING, (short)50);
private static final org.apache.thrift.protocol.TField EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS_FIELD_DESC = new org.apache.thrift.protocol.TField("executionStartToCloseTimeoutSeconds", org.apache.thrift.protocol.TType.I32, (short)60);
private static final org.apache.thrift.protocol.TField TASK_START_TO_CLOSE_TIMEOUT_SECONDS_FIELD_DESC = new org.apache.thrift.protocol.TField("taskStartToCloseTimeoutSeconds", org.apache.thrift.protocol.TType.I32, (short)70);
private static final org.apache.thrift.protocol.TField IDENTITY_FIELD_DESC = new org.apache.thrift.protocol.TField("identity", org.apache.thrift.protocol.TType.STRING, (short)80);
private static final org.apache.thrift.protocol.TField REQUEST_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("requestId", org.apache.thrift.protocol.TType.STRING, (short)90);
private static final org.apache.thrift.protocol.TField WORKFLOW_ID_REUSE_POLICY_FIELD_DESC = new org.apache.thrift.protocol.TField("workflowIdReusePolicy", org.apache.thrift.protocol.TType.I32, (short)100);
private static final org.apache.thrift.protocol.TField SIGNAL_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("signalName", org.apache.thrift.protocol.TType.STRING, (short)110);
private static final org.apache.thrift.protocol.TField SIGNAL_INPUT_FIELD_DESC = new org.apache.thrift.protocol.TField("signalInput", org.apache.thrift.protocol.TType.STRING, (short)120);
private static final org.apache.thrift.protocol.TField CONTROL_FIELD_DESC = new org.apache.thrift.protocol.TField("control", org.apache.thrift.protocol.TType.STRING, (short)130);
private static final org.apache.thrift.protocol.TField RETRY_POLICY_FIELD_DESC = new org.apache.thrift.protocol.TField("retryPolicy", org.apache.thrift.protocol.TType.STRUCT, (short)140);
private static final org.apache.thrift.protocol.TField CRON_SCHEDULE_FIELD_DESC = new org.apache.thrift.protocol.TField("cronSchedule", org.apache.thrift.protocol.TType.STRING, (short)150);
private static final org.apache.thrift.protocol.TField MEMO_FIELD_DESC = new org.apache.thrift.protocol.TField("memo", org.apache.thrift.protocol.TType.STRUCT, (short)160);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new SignalWithStartWorkflowExecutionRequestStandardSchemeFactory());
schemes.put(TupleScheme.class, new SignalWithStartWorkflowExecutionRequestTupleSchemeFactory());
}
public String domain; // optional
public String workflowId; // optional
public WorkflowType workflowType; // optional
public TaskList taskList; // optional
public ByteBuffer input; // optional
public int executionStartToCloseTimeoutSeconds; // optional
public int taskStartToCloseTimeoutSeconds; // optional
public String identity; // optional
public String requestId; // optional
/**
*
* @see WorkflowIdReusePolicy
*/
public WorkflowIdReusePolicy workflowIdReusePolicy; // optional
public String signalName; // optional
public ByteBuffer signalInput; // optional
public ByteBuffer control; // optional
public RetryPolicy retryPolicy; // optional
public String cronSchedule; // optional
public Memo memo; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
DOMAIN((short)10, "domain"),
WORKFLOW_ID((short)20, "workflowId"),
WORKFLOW_TYPE((short)30, "workflowType"),
TASK_LIST((short)40, "taskList"),
INPUT((short)50, "input"),
EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS((short)60, "executionStartToCloseTimeoutSeconds"),
TASK_START_TO_CLOSE_TIMEOUT_SECONDS((short)70, "taskStartToCloseTimeoutSeconds"),
IDENTITY((short)80, "identity"),
REQUEST_ID((short)90, "requestId"),
/**
*
* @see WorkflowIdReusePolicy
*/
WORKFLOW_ID_REUSE_POLICY((short)100, "workflowIdReusePolicy"),
SIGNAL_NAME((short)110, "signalName"),
SIGNAL_INPUT((short)120, "signalInput"),
CONTROL((short)130, "control"),
RETRY_POLICY((short)140, "retryPolicy"),
CRON_SCHEDULE((short)150, "cronSchedule"),
MEMO((short)160, "memo");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // DOMAIN
return DOMAIN;
case 20: // WORKFLOW_ID
return WORKFLOW_ID;
case 30: // WORKFLOW_TYPE
return WORKFLOW_TYPE;
case 40: // TASK_LIST
return TASK_LIST;
case 50: // INPUT
return INPUT;
case 60: // EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS
return EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS;
case 70: // TASK_START_TO_CLOSE_TIMEOUT_SECONDS
return TASK_START_TO_CLOSE_TIMEOUT_SECONDS;
case 80: // IDENTITY
return IDENTITY;
case 90: // REQUEST_ID
return REQUEST_ID;
case 100: // WORKFLOW_ID_REUSE_POLICY
return WORKFLOW_ID_REUSE_POLICY;
case 110: // SIGNAL_NAME
return SIGNAL_NAME;
case 120: // SIGNAL_INPUT
return SIGNAL_INPUT;
case 130: // CONTROL
return CONTROL;
case 140: // RETRY_POLICY
return RETRY_POLICY;
case 150: // CRON_SCHEDULE
return CRON_SCHEDULE;
case 160: // MEMO
return MEMO;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __EXECUTIONSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID = 0;
private static final int __TASKSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.DOMAIN,_Fields.WORKFLOW_ID,_Fields.WORKFLOW_TYPE,_Fields.TASK_LIST,_Fields.INPUT,_Fields.EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS,_Fields.TASK_START_TO_CLOSE_TIMEOUT_SECONDS,_Fields.IDENTITY,_Fields.REQUEST_ID,_Fields.WORKFLOW_ID_REUSE_POLICY,_Fields.SIGNAL_NAME,_Fields.SIGNAL_INPUT,_Fields.CONTROL,_Fields.RETRY_POLICY,_Fields.CRON_SCHEDULE,_Fields.MEMO};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.DOMAIN, new org.apache.thrift.meta_data.FieldMetaData("domain", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WORKFLOW_ID, new org.apache.thrift.meta_data.FieldMetaData("workflowId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WORKFLOW_TYPE, new org.apache.thrift.meta_data.FieldMetaData("workflowType", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowType.class)));
tmpMap.put(_Fields.TASK_LIST, new org.apache.thrift.meta_data.FieldMetaData("taskList", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, TaskList.class)));
tmpMap.put(_Fields.INPUT, new org.apache.thrift.meta_data.FieldMetaData("input", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS, new org.apache.thrift.meta_data.FieldMetaData("executionStartToCloseTimeoutSeconds", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.TASK_START_TO_CLOSE_TIMEOUT_SECONDS, new org.apache.thrift.meta_data.FieldMetaData("taskStartToCloseTimeoutSeconds", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.IDENTITY, new org.apache.thrift.meta_data.FieldMetaData("identity", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.REQUEST_ID, new org.apache.thrift.meta_data.FieldMetaData("requestId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WORKFLOW_ID_REUSE_POLICY, new org.apache.thrift.meta_data.FieldMetaData("workflowIdReusePolicy", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, WorkflowIdReusePolicy.class)));
tmpMap.put(_Fields.SIGNAL_NAME, new org.apache.thrift.meta_data.FieldMetaData("signalName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.SIGNAL_INPUT, new org.apache.thrift.meta_data.FieldMetaData("signalInput", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.CONTROL, new org.apache.thrift.meta_data.FieldMetaData("control", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.RETRY_POLICY, new org.apache.thrift.meta_data.FieldMetaData("retryPolicy", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "RetryPolicy")));
tmpMap.put(_Fields.CRON_SCHEDULE, new org.apache.thrift.meta_data.FieldMetaData("cronSchedule", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.MEMO, new org.apache.thrift.meta_data.FieldMetaData("memo", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Memo.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(SignalWithStartWorkflowExecutionRequest.class, metaDataMap);
}
public SignalWithStartWorkflowExecutionRequest() {
}
/**
* Performs a deep copy on other.
*/
public SignalWithStartWorkflowExecutionRequest(SignalWithStartWorkflowExecutionRequest other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetDomain()) {
this.domain = other.domain;
}
if (other.isSetWorkflowId()) {
this.workflowId = other.workflowId;
}
if (other.isSetWorkflowType()) {
this.workflowType = new WorkflowType(other.workflowType);
}
if (other.isSetTaskList()) {
this.taskList = new TaskList(other.taskList);
}
if (other.isSetInput()) {
this.input = org.apache.thrift.TBaseHelper.copyBinary(other.input);
}
this.executionStartToCloseTimeoutSeconds = other.executionStartToCloseTimeoutSeconds;
this.taskStartToCloseTimeoutSeconds = other.taskStartToCloseTimeoutSeconds;
if (other.isSetIdentity()) {
this.identity = other.identity;
}
if (other.isSetRequestId()) {
this.requestId = other.requestId;
}
if (other.isSetWorkflowIdReusePolicy()) {
this.workflowIdReusePolicy = other.workflowIdReusePolicy;
}
if (other.isSetSignalName()) {
this.signalName = other.signalName;
}
if (other.isSetSignalInput()) {
this.signalInput = org.apache.thrift.TBaseHelper.copyBinary(other.signalInput);
}
if (other.isSetControl()) {
this.control = org.apache.thrift.TBaseHelper.copyBinary(other.control);
}
if (other.isSetRetryPolicy()) {
this.retryPolicy = other.retryPolicy;
}
if (other.isSetCronSchedule()) {
this.cronSchedule = other.cronSchedule;
}
if (other.isSetMemo()) {
this.memo = new Memo(other.memo);
}
}
public SignalWithStartWorkflowExecutionRequest deepCopy() {
return new SignalWithStartWorkflowExecutionRequest(this);
}
@Override
public void clear() {
this.domain = null;
this.workflowId = null;
this.workflowType = null;
this.taskList = null;
this.input = null;
setExecutionStartToCloseTimeoutSecondsIsSet(false);
this.executionStartToCloseTimeoutSeconds = 0;
setTaskStartToCloseTimeoutSecondsIsSet(false);
this.taskStartToCloseTimeoutSeconds = 0;
this.identity = null;
this.requestId = null;
this.workflowIdReusePolicy = null;
this.signalName = null;
this.signalInput = null;
this.control = null;
this.retryPolicy = null;
this.cronSchedule = null;
this.memo = null;
}
public String getDomain() {
return this.domain;
}
public SignalWithStartWorkflowExecutionRequest setDomain(String domain) {
this.domain = domain;
return this;
}
public void unsetDomain() {
this.domain = null;
}
/** Returns true if field domain is set (has been assigned a value) and false otherwise */
public boolean isSetDomain() {
return this.domain != null;
}
public void setDomainIsSet(boolean value) {
if (!value) {
this.domain = null;
}
}
public String getWorkflowId() {
return this.workflowId;
}
public SignalWithStartWorkflowExecutionRequest setWorkflowId(String workflowId) {
this.workflowId = workflowId;
return this;
}
public void unsetWorkflowId() {
this.workflowId = null;
}
/** Returns true if field workflowId is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowId() {
return this.workflowId != null;
}
public void setWorkflowIdIsSet(boolean value) {
if (!value) {
this.workflowId = null;
}
}
public WorkflowType getWorkflowType() {
return this.workflowType;
}
public SignalWithStartWorkflowExecutionRequest setWorkflowType(WorkflowType workflowType) {
this.workflowType = workflowType;
return this;
}
public void unsetWorkflowType() {
this.workflowType = null;
}
/** Returns true if field workflowType is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowType() {
return this.workflowType != null;
}
public void setWorkflowTypeIsSet(boolean value) {
if (!value) {
this.workflowType = null;
}
}
public TaskList getTaskList() {
return this.taskList;
}
public SignalWithStartWorkflowExecutionRequest setTaskList(TaskList taskList) {
this.taskList = taskList;
return this;
}
public void unsetTaskList() {
this.taskList = null;
}
/** Returns true if field taskList is set (has been assigned a value) and false otherwise */
public boolean isSetTaskList() {
return this.taskList != null;
}
public void setTaskListIsSet(boolean value) {
if (!value) {
this.taskList = null;
}
}
public byte[] getInput() {
setInput(org.apache.thrift.TBaseHelper.rightSize(input));
return input == null ? null : input.array();
}
public ByteBuffer bufferForInput() {
return org.apache.thrift.TBaseHelper.copyBinary(input);
}
public SignalWithStartWorkflowExecutionRequest setInput(byte[] input) {
this.input = input == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(input, input.length));
return this;
}
public SignalWithStartWorkflowExecutionRequest setInput(ByteBuffer input) {
this.input = org.apache.thrift.TBaseHelper.copyBinary(input);
return this;
}
public void unsetInput() {
this.input = null;
}
/** Returns true if field input is set (has been assigned a value) and false otherwise */
public boolean isSetInput() {
return this.input != null;
}
public void setInputIsSet(boolean value) {
if (!value) {
this.input = null;
}
}
public int getExecutionStartToCloseTimeoutSeconds() {
return this.executionStartToCloseTimeoutSeconds;
}
public SignalWithStartWorkflowExecutionRequest setExecutionStartToCloseTimeoutSeconds(int executionStartToCloseTimeoutSeconds) {
this.executionStartToCloseTimeoutSeconds = executionStartToCloseTimeoutSeconds;
setExecutionStartToCloseTimeoutSecondsIsSet(true);
return this;
}
public void unsetExecutionStartToCloseTimeoutSeconds() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __EXECUTIONSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID);
}
/** Returns true if field executionStartToCloseTimeoutSeconds is set (has been assigned a value) and false otherwise */
public boolean isSetExecutionStartToCloseTimeoutSeconds() {
return EncodingUtils.testBit(__isset_bitfield, __EXECUTIONSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID);
}
public void setExecutionStartToCloseTimeoutSecondsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __EXECUTIONSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID, value);
}
public int getTaskStartToCloseTimeoutSeconds() {
return this.taskStartToCloseTimeoutSeconds;
}
public SignalWithStartWorkflowExecutionRequest setTaskStartToCloseTimeoutSeconds(int taskStartToCloseTimeoutSeconds) {
this.taskStartToCloseTimeoutSeconds = taskStartToCloseTimeoutSeconds;
setTaskStartToCloseTimeoutSecondsIsSet(true);
return this;
}
public void unsetTaskStartToCloseTimeoutSeconds() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TASKSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID);
}
/** Returns true if field taskStartToCloseTimeoutSeconds is set (has been assigned a value) and false otherwise */
public boolean isSetTaskStartToCloseTimeoutSeconds() {
return EncodingUtils.testBit(__isset_bitfield, __TASKSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID);
}
public void setTaskStartToCloseTimeoutSecondsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TASKSTARTTOCLOSETIMEOUTSECONDS_ISSET_ID, value);
}
public String getIdentity() {
return this.identity;
}
public SignalWithStartWorkflowExecutionRequest setIdentity(String identity) {
this.identity = identity;
return this;
}
public void unsetIdentity() {
this.identity = null;
}
/** Returns true if field identity is set (has been assigned a value) and false otherwise */
public boolean isSetIdentity() {
return this.identity != null;
}
public void setIdentityIsSet(boolean value) {
if (!value) {
this.identity = null;
}
}
public String getRequestId() {
return this.requestId;
}
public SignalWithStartWorkflowExecutionRequest setRequestId(String requestId) {
this.requestId = requestId;
return this;
}
public void unsetRequestId() {
this.requestId = null;
}
/** Returns true if field requestId is set (has been assigned a value) and false otherwise */
public boolean isSetRequestId() {
return this.requestId != null;
}
public void setRequestIdIsSet(boolean value) {
if (!value) {
this.requestId = null;
}
}
/**
*
* @see WorkflowIdReusePolicy
*/
public WorkflowIdReusePolicy getWorkflowIdReusePolicy() {
return this.workflowIdReusePolicy;
}
/**
*
* @see WorkflowIdReusePolicy
*/
public SignalWithStartWorkflowExecutionRequest setWorkflowIdReusePolicy(WorkflowIdReusePolicy workflowIdReusePolicy) {
this.workflowIdReusePolicy = workflowIdReusePolicy;
return this;
}
public void unsetWorkflowIdReusePolicy() {
this.workflowIdReusePolicy = null;
}
/** Returns true if field workflowIdReusePolicy is set (has been assigned a value) and false otherwise */
public boolean isSetWorkflowIdReusePolicy() {
return this.workflowIdReusePolicy != null;
}
public void setWorkflowIdReusePolicyIsSet(boolean value) {
if (!value) {
this.workflowIdReusePolicy = null;
}
}
public String getSignalName() {
return this.signalName;
}
public SignalWithStartWorkflowExecutionRequest setSignalName(String signalName) {
this.signalName = signalName;
return this;
}
public void unsetSignalName() {
this.signalName = null;
}
/** Returns true if field signalName is set (has been assigned a value) and false otherwise */
public boolean isSetSignalName() {
return this.signalName != null;
}
public void setSignalNameIsSet(boolean value) {
if (!value) {
this.signalName = null;
}
}
public byte[] getSignalInput() {
setSignalInput(org.apache.thrift.TBaseHelper.rightSize(signalInput));
return signalInput == null ? null : signalInput.array();
}
public ByteBuffer bufferForSignalInput() {
return org.apache.thrift.TBaseHelper.copyBinary(signalInput);
}
public SignalWithStartWorkflowExecutionRequest setSignalInput(byte[] signalInput) {
this.signalInput = signalInput == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(signalInput, signalInput.length));
return this;
}
public SignalWithStartWorkflowExecutionRequest setSignalInput(ByteBuffer signalInput) {
this.signalInput = org.apache.thrift.TBaseHelper.copyBinary(signalInput);
return this;
}
public void unsetSignalInput() {
this.signalInput = null;
}
/** Returns true if field signalInput is set (has been assigned a value) and false otherwise */
public boolean isSetSignalInput() {
return this.signalInput != null;
}
public void setSignalInputIsSet(boolean value) {
if (!value) {
this.signalInput = null;
}
}
public byte[] getControl() {
setControl(org.apache.thrift.TBaseHelper.rightSize(control));
return control == null ? null : control.array();
}
public ByteBuffer bufferForControl() {
return org.apache.thrift.TBaseHelper.copyBinary(control);
}
public SignalWithStartWorkflowExecutionRequest setControl(byte[] control) {
this.control = control == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(control, control.length));
return this;
}
public SignalWithStartWorkflowExecutionRequest setControl(ByteBuffer control) {
this.control = org.apache.thrift.TBaseHelper.copyBinary(control);
return this;
}
public void unsetControl() {
this.control = null;
}
/** Returns true if field control is set (has been assigned a value) and false otherwise */
public boolean isSetControl() {
return this.control != null;
}
public void setControlIsSet(boolean value) {
if (!value) {
this.control = null;
}
}
public RetryPolicy getRetryPolicy() {
return this.retryPolicy;
}
public SignalWithStartWorkflowExecutionRequest setRetryPolicy(RetryPolicy retryPolicy) {
this.retryPolicy = retryPolicy;
return this;
}
public void unsetRetryPolicy() {
this.retryPolicy = null;
}
/** Returns true if field retryPolicy is set (has been assigned a value) and false otherwise */
public boolean isSetRetryPolicy() {
return this.retryPolicy != null;
}
public void setRetryPolicyIsSet(boolean value) {
if (!value) {
this.retryPolicy = null;
}
}
public String getCronSchedule() {
return this.cronSchedule;
}
public SignalWithStartWorkflowExecutionRequest setCronSchedule(String cronSchedule) {
this.cronSchedule = cronSchedule;
return this;
}
public void unsetCronSchedule() {
this.cronSchedule = null;
}
/** Returns true if field cronSchedule is set (has been assigned a value) and false otherwise */
public boolean isSetCronSchedule() {
return this.cronSchedule != null;
}
public void setCronScheduleIsSet(boolean value) {
if (!value) {
this.cronSchedule = null;
}
}
public Memo getMemo() {
return this.memo;
}
public SignalWithStartWorkflowExecutionRequest setMemo(Memo memo) {
this.memo = memo;
return this;
}
public void unsetMemo() {
this.memo = null;
}
/** Returns true if field memo is set (has been assigned a value) and false otherwise */
public boolean isSetMemo() {
return this.memo != null;
}
public void setMemoIsSet(boolean value) {
if (!value) {
this.memo = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case DOMAIN:
if (value == null) {
unsetDomain();
} else {
setDomain((String)value);
}
break;
case WORKFLOW_ID:
if (value == null) {
unsetWorkflowId();
} else {
setWorkflowId((String)value);
}
break;
case WORKFLOW_TYPE:
if (value == null) {
unsetWorkflowType();
} else {
setWorkflowType((WorkflowType)value);
}
break;
case TASK_LIST:
if (value == null) {
unsetTaskList();
} else {
setTaskList((TaskList)value);
}
break;
case INPUT:
if (value == null) {
unsetInput();
} else {
setInput((ByteBuffer)value);
}
break;
case EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS:
if (value == null) {
unsetExecutionStartToCloseTimeoutSeconds();
} else {
setExecutionStartToCloseTimeoutSeconds((Integer)value);
}
break;
case TASK_START_TO_CLOSE_TIMEOUT_SECONDS:
if (value == null) {
unsetTaskStartToCloseTimeoutSeconds();
} else {
setTaskStartToCloseTimeoutSeconds((Integer)value);
}
break;
case IDENTITY:
if (value == null) {
unsetIdentity();
} else {
setIdentity((String)value);
}
break;
case REQUEST_ID:
if (value == null) {
unsetRequestId();
} else {
setRequestId((String)value);
}
break;
case WORKFLOW_ID_REUSE_POLICY:
if (value == null) {
unsetWorkflowIdReusePolicy();
} else {
setWorkflowIdReusePolicy((WorkflowIdReusePolicy)value);
}
break;
case SIGNAL_NAME:
if (value == null) {
unsetSignalName();
} else {
setSignalName((String)value);
}
break;
case SIGNAL_INPUT:
if (value == null) {
unsetSignalInput();
} else {
setSignalInput((ByteBuffer)value);
}
break;
case CONTROL:
if (value == null) {
unsetControl();
} else {
setControl((ByteBuffer)value);
}
break;
case RETRY_POLICY:
if (value == null) {
unsetRetryPolicy();
} else {
setRetryPolicy((RetryPolicy)value);
}
break;
case CRON_SCHEDULE:
if (value == null) {
unsetCronSchedule();
} else {
setCronSchedule((String)value);
}
break;
case MEMO:
if (value == null) {
unsetMemo();
} else {
setMemo((Memo)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case DOMAIN:
return getDomain();
case WORKFLOW_ID:
return getWorkflowId();
case WORKFLOW_TYPE:
return getWorkflowType();
case TASK_LIST:
return getTaskList();
case INPUT:
return getInput();
case EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS:
return getExecutionStartToCloseTimeoutSeconds();
case TASK_START_TO_CLOSE_TIMEOUT_SECONDS:
return getTaskStartToCloseTimeoutSeconds();
case IDENTITY:
return getIdentity();
case REQUEST_ID:
return getRequestId();
case WORKFLOW_ID_REUSE_POLICY:
return getWorkflowIdReusePolicy();
case SIGNAL_NAME:
return getSignalName();
case SIGNAL_INPUT:
return getSignalInput();
case CONTROL:
return getControl();
case RETRY_POLICY:
return getRetryPolicy();
case CRON_SCHEDULE:
return getCronSchedule();
case MEMO:
return getMemo();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case DOMAIN:
return isSetDomain();
case WORKFLOW_ID:
return isSetWorkflowId();
case WORKFLOW_TYPE:
return isSetWorkflowType();
case TASK_LIST:
return isSetTaskList();
case INPUT:
return isSetInput();
case EXECUTION_START_TO_CLOSE_TIMEOUT_SECONDS:
return isSetExecutionStartToCloseTimeoutSeconds();
case TASK_START_TO_CLOSE_TIMEOUT_SECONDS:
return isSetTaskStartToCloseTimeoutSeconds();
case IDENTITY:
return isSetIdentity();
case REQUEST_ID:
return isSetRequestId();
case WORKFLOW_ID_REUSE_POLICY:
return isSetWorkflowIdReusePolicy();
case SIGNAL_NAME:
return isSetSignalName();
case SIGNAL_INPUT:
return isSetSignalInput();
case CONTROL:
return isSetControl();
case RETRY_POLICY:
return isSetRetryPolicy();
case CRON_SCHEDULE:
return isSetCronSchedule();
case MEMO:
return isSetMemo();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof SignalWithStartWorkflowExecutionRequest)
return this.equals((SignalWithStartWorkflowExecutionRequest)that);
return false;
}
public boolean equals(SignalWithStartWorkflowExecutionRequest that) {
if (that == null)
return false;
boolean this_present_domain = true && this.isSetDomain();
boolean that_present_domain = true && that.isSetDomain();
if (this_present_domain || that_present_domain) {
if (!(this_present_domain && that_present_domain))
return false;
if (!this.domain.equals(that.domain))
return false;
}
boolean this_present_workflowId = true && this.isSetWorkflowId();
boolean that_present_workflowId = true && that.isSetWorkflowId();
if (this_present_workflowId || that_present_workflowId) {
if (!(this_present_workflowId && that_present_workflowId))
return false;
if (!this.workflowId.equals(that.workflowId))
return false;
}
boolean this_present_workflowType = true && this.isSetWorkflowType();
boolean that_present_workflowType = true && that.isSetWorkflowType();
if (this_present_workflowType || that_present_workflowType) {
if (!(this_present_workflowType && that_present_workflowType))
return false;
if (!this.workflowType.equals(that.workflowType))
return false;
}
boolean this_present_taskList = true && this.isSetTaskList();
boolean that_present_taskList = true && that.isSetTaskList();
if (this_present_taskList || that_present_taskList) {
if (!(this_present_taskList && that_present_taskList))
return false;
if (!this.taskList.equals(that.taskList))
return false;
}
boolean this_present_input = true && this.isSetInput();
boolean that_present_input = true && that.isSetInput();
if (this_present_input || that_present_input) {
if (!(this_present_input && that_present_input))
return false;
if (!this.input.equals(that.input))
return false;
}
boolean this_present_executionStartToCloseTimeoutSeconds = true && this.isSetExecutionStartToCloseTimeoutSeconds();
boolean that_present_executionStartToCloseTimeoutSeconds = true && that.isSetExecutionStartToCloseTimeoutSeconds();
if (this_present_executionStartToCloseTimeoutSeconds || that_present_executionStartToCloseTimeoutSeconds) {
if (!(this_present_executionStartToCloseTimeoutSeconds && that_present_executionStartToCloseTimeoutSeconds))
return false;
if (this.executionStartToCloseTimeoutSeconds != that.executionStartToCloseTimeoutSeconds)
return false;
}
boolean this_present_taskStartToCloseTimeoutSeconds = true && this.isSetTaskStartToCloseTimeoutSeconds();
boolean that_present_taskStartToCloseTimeoutSeconds = true && that.isSetTaskStartToCloseTimeoutSeconds();
if (this_present_taskStartToCloseTimeoutSeconds || that_present_taskStartToCloseTimeoutSeconds) {
if (!(this_present_taskStartToCloseTimeoutSeconds && that_present_taskStartToCloseTimeoutSeconds))
return false;
if (this.taskStartToCloseTimeoutSeconds != that.taskStartToCloseTimeoutSeconds)
return false;
}
boolean this_present_identity = true && this.isSetIdentity();
boolean that_present_identity = true && that.isSetIdentity();
if (this_present_identity || that_present_identity) {
if (!(this_present_identity && that_present_identity))
return false;
if (!this.identity.equals(that.identity))
return false;
}
boolean this_present_requestId = true && this.isSetRequestId();
boolean that_present_requestId = true && that.isSetRequestId();
if (this_present_requestId || that_present_requestId) {
if (!(this_present_requestId && that_present_requestId))
return false;
if (!this.requestId.equals(that.requestId))
return false;
}
boolean this_present_workflowIdReusePolicy = true && this.isSetWorkflowIdReusePolicy();
boolean that_present_workflowIdReusePolicy = true && that.isSetWorkflowIdReusePolicy();
if (this_present_workflowIdReusePolicy || that_present_workflowIdReusePolicy) {
if (!(this_present_workflowIdReusePolicy && that_present_workflowIdReusePolicy))
return false;
if (!this.workflowIdReusePolicy.equals(that.workflowIdReusePolicy))
return false;
}
boolean this_present_signalName = true && this.isSetSignalName();
boolean that_present_signalName = true && that.isSetSignalName();
if (this_present_signalName || that_present_signalName) {
if (!(this_present_signalName && that_present_signalName))
return false;
if (!this.signalName.equals(that.signalName))
return false;
}
boolean this_present_signalInput = true && this.isSetSignalInput();
boolean that_present_signalInput = true && that.isSetSignalInput();
if (this_present_signalInput || that_present_signalInput) {
if (!(this_present_signalInput && that_present_signalInput))
return false;
if (!this.signalInput.equals(that.signalInput))
return false;
}
boolean this_present_control = true && this.isSetControl();
boolean that_present_control = true && that.isSetControl();
if (this_present_control || that_present_control) {
if (!(this_present_control && that_present_control))
return false;
if (!this.control.equals(that.control))
return false;
}
boolean this_present_retryPolicy = true && this.isSetRetryPolicy();
boolean that_present_retryPolicy = true && that.isSetRetryPolicy();
if (this_present_retryPolicy || that_present_retryPolicy) {
if (!(this_present_retryPolicy && that_present_retryPolicy))
return false;
if (!this.retryPolicy.equals(that.retryPolicy))
return false;
}
boolean this_present_cronSchedule = true && this.isSetCronSchedule();
boolean that_present_cronSchedule = true && that.isSetCronSchedule();
if (this_present_cronSchedule || that_present_cronSchedule) {
if (!(this_present_cronSchedule && that_present_cronSchedule))
return false;
if (!this.cronSchedule.equals(that.cronSchedule))
return false;
}
boolean this_present_memo = true && this.isSetMemo();
boolean that_present_memo = true && that.isSetMemo();
if (this_present_memo || that_present_memo) {
if (!(this_present_memo && that_present_memo))
return false;
if (!this.memo.equals(that.memo))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy