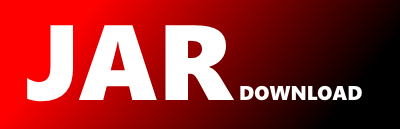
com.uber.cadence.WorkflowExecutionInfo Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package com.uber.cadence;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-06-18")
public class WorkflowExecutionInfo implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("WorkflowExecutionInfo");
private static final org.apache.thrift.protocol.TField EXECUTION_FIELD_DESC = new org.apache.thrift.protocol.TField("execution", org.apache.thrift.protocol.TType.STRUCT, (short)10);
private static final org.apache.thrift.protocol.TField TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("type", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField START_TIME_FIELD_DESC = new org.apache.thrift.protocol.TField("startTime", org.apache.thrift.protocol.TType.I64, (short)30);
private static final org.apache.thrift.protocol.TField CLOSE_TIME_FIELD_DESC = new org.apache.thrift.protocol.TField("closeTime", org.apache.thrift.protocol.TType.I64, (short)40);
private static final org.apache.thrift.protocol.TField CLOSE_STATUS_FIELD_DESC = new org.apache.thrift.protocol.TField("closeStatus", org.apache.thrift.protocol.TType.I32, (short)50);
private static final org.apache.thrift.protocol.TField HISTORY_LENGTH_FIELD_DESC = new org.apache.thrift.protocol.TField("historyLength", org.apache.thrift.protocol.TType.I64, (short)60);
private static final org.apache.thrift.protocol.TField PARENT_DOMAIN_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("parentDomainId", org.apache.thrift.protocol.TType.STRING, (short)70);
private static final org.apache.thrift.protocol.TField PARENT_EXECUTION_FIELD_DESC = new org.apache.thrift.protocol.TField("parentExecution", org.apache.thrift.protocol.TType.STRUCT, (short)80);
private static final org.apache.thrift.protocol.TField EXECUTION_TIME_FIELD_DESC = new org.apache.thrift.protocol.TField("executionTime", org.apache.thrift.protocol.TType.I64, (short)90);
private static final org.apache.thrift.protocol.TField MEMO_FIELD_DESC = new org.apache.thrift.protocol.TField("memo", org.apache.thrift.protocol.TType.STRUCT, (short)100);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new WorkflowExecutionInfoStandardSchemeFactory());
schemes.put(TupleScheme.class, new WorkflowExecutionInfoTupleSchemeFactory());
}
public WorkflowExecution execution; // optional
public WorkflowType type; // optional
public long startTime; // optional
public long closeTime; // optional
/**
*
* @see WorkflowExecutionCloseStatus
*/
public WorkflowExecutionCloseStatus closeStatus; // optional
public long historyLength; // optional
public String parentDomainId; // optional
public WorkflowExecution parentExecution; // optional
public long executionTime; // optional
public Memo memo; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
EXECUTION((short)10, "execution"),
TYPE((short)20, "type"),
START_TIME((short)30, "startTime"),
CLOSE_TIME((short)40, "closeTime"),
/**
*
* @see WorkflowExecutionCloseStatus
*/
CLOSE_STATUS((short)50, "closeStatus"),
HISTORY_LENGTH((short)60, "historyLength"),
PARENT_DOMAIN_ID((short)70, "parentDomainId"),
PARENT_EXECUTION((short)80, "parentExecution"),
EXECUTION_TIME((short)90, "executionTime"),
MEMO((short)100, "memo");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 10: // EXECUTION
return EXECUTION;
case 20: // TYPE
return TYPE;
case 30: // START_TIME
return START_TIME;
case 40: // CLOSE_TIME
return CLOSE_TIME;
case 50: // CLOSE_STATUS
return CLOSE_STATUS;
case 60: // HISTORY_LENGTH
return HISTORY_LENGTH;
case 70: // PARENT_DOMAIN_ID
return PARENT_DOMAIN_ID;
case 80: // PARENT_EXECUTION
return PARENT_EXECUTION;
case 90: // EXECUTION_TIME
return EXECUTION_TIME;
case 100: // MEMO
return MEMO;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __STARTTIME_ISSET_ID = 0;
private static final int __CLOSETIME_ISSET_ID = 1;
private static final int __HISTORYLENGTH_ISSET_ID = 2;
private static final int __EXECUTIONTIME_ISSET_ID = 3;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.EXECUTION,_Fields.TYPE,_Fields.START_TIME,_Fields.CLOSE_TIME,_Fields.CLOSE_STATUS,_Fields.HISTORY_LENGTH,_Fields.PARENT_DOMAIN_ID,_Fields.PARENT_EXECUTION,_Fields.EXECUTION_TIME,_Fields.MEMO};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.EXECUTION, new org.apache.thrift.meta_data.FieldMetaData("execution", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecution.class)));
tmpMap.put(_Fields.TYPE, new org.apache.thrift.meta_data.FieldMetaData("type", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowType.class)));
tmpMap.put(_Fields.START_TIME, new org.apache.thrift.meta_data.FieldMetaData("startTime", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.CLOSE_TIME, new org.apache.thrift.meta_data.FieldMetaData("closeTime", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.CLOSE_STATUS, new org.apache.thrift.meta_data.FieldMetaData("closeStatus", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, WorkflowExecutionCloseStatus.class)));
tmpMap.put(_Fields.HISTORY_LENGTH, new org.apache.thrift.meta_data.FieldMetaData("historyLength", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.PARENT_DOMAIN_ID, new org.apache.thrift.meta_data.FieldMetaData("parentDomainId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.PARENT_EXECUTION, new org.apache.thrift.meta_data.FieldMetaData("parentExecution", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, WorkflowExecution.class)));
tmpMap.put(_Fields.EXECUTION_TIME, new org.apache.thrift.meta_data.FieldMetaData("executionTime", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.MEMO, new org.apache.thrift.meta_data.FieldMetaData("memo", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Memo.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(WorkflowExecutionInfo.class, metaDataMap);
}
public WorkflowExecutionInfo() {
}
/**
* Performs a deep copy on other.
*/
public WorkflowExecutionInfo(WorkflowExecutionInfo other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetExecution()) {
this.execution = new WorkflowExecution(other.execution);
}
if (other.isSetType()) {
this.type = new WorkflowType(other.type);
}
this.startTime = other.startTime;
this.closeTime = other.closeTime;
if (other.isSetCloseStatus()) {
this.closeStatus = other.closeStatus;
}
this.historyLength = other.historyLength;
if (other.isSetParentDomainId()) {
this.parentDomainId = other.parentDomainId;
}
if (other.isSetParentExecution()) {
this.parentExecution = new WorkflowExecution(other.parentExecution);
}
this.executionTime = other.executionTime;
if (other.isSetMemo()) {
this.memo = new Memo(other.memo);
}
}
public WorkflowExecutionInfo deepCopy() {
return new WorkflowExecutionInfo(this);
}
@Override
public void clear() {
this.execution = null;
this.type = null;
setStartTimeIsSet(false);
this.startTime = 0;
setCloseTimeIsSet(false);
this.closeTime = 0;
this.closeStatus = null;
setHistoryLengthIsSet(false);
this.historyLength = 0;
this.parentDomainId = null;
this.parentExecution = null;
setExecutionTimeIsSet(false);
this.executionTime = 0;
this.memo = null;
}
public WorkflowExecution getExecution() {
return this.execution;
}
public WorkflowExecutionInfo setExecution(WorkflowExecution execution) {
this.execution = execution;
return this;
}
public void unsetExecution() {
this.execution = null;
}
/** Returns true if field execution is set (has been assigned a value) and false otherwise */
public boolean isSetExecution() {
return this.execution != null;
}
public void setExecutionIsSet(boolean value) {
if (!value) {
this.execution = null;
}
}
public WorkflowType getType() {
return this.type;
}
public WorkflowExecutionInfo setType(WorkflowType type) {
this.type = type;
return this;
}
public void unsetType() {
this.type = null;
}
/** Returns true if field type is set (has been assigned a value) and false otherwise */
public boolean isSetType() {
return this.type != null;
}
public void setTypeIsSet(boolean value) {
if (!value) {
this.type = null;
}
}
public long getStartTime() {
return this.startTime;
}
public WorkflowExecutionInfo setStartTime(long startTime) {
this.startTime = startTime;
setStartTimeIsSet(true);
return this;
}
public void unsetStartTime() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __STARTTIME_ISSET_ID);
}
/** Returns true if field startTime is set (has been assigned a value) and false otherwise */
public boolean isSetStartTime() {
return EncodingUtils.testBit(__isset_bitfield, __STARTTIME_ISSET_ID);
}
public void setStartTimeIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __STARTTIME_ISSET_ID, value);
}
public long getCloseTime() {
return this.closeTime;
}
public WorkflowExecutionInfo setCloseTime(long closeTime) {
this.closeTime = closeTime;
setCloseTimeIsSet(true);
return this;
}
public void unsetCloseTime() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __CLOSETIME_ISSET_ID);
}
/** Returns true if field closeTime is set (has been assigned a value) and false otherwise */
public boolean isSetCloseTime() {
return EncodingUtils.testBit(__isset_bitfield, __CLOSETIME_ISSET_ID);
}
public void setCloseTimeIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __CLOSETIME_ISSET_ID, value);
}
/**
*
* @see WorkflowExecutionCloseStatus
*/
public WorkflowExecutionCloseStatus getCloseStatus() {
return this.closeStatus;
}
/**
*
* @see WorkflowExecutionCloseStatus
*/
public WorkflowExecutionInfo setCloseStatus(WorkflowExecutionCloseStatus closeStatus) {
this.closeStatus = closeStatus;
return this;
}
public void unsetCloseStatus() {
this.closeStatus = null;
}
/** Returns true if field closeStatus is set (has been assigned a value) and false otherwise */
public boolean isSetCloseStatus() {
return this.closeStatus != null;
}
public void setCloseStatusIsSet(boolean value) {
if (!value) {
this.closeStatus = null;
}
}
public long getHistoryLength() {
return this.historyLength;
}
public WorkflowExecutionInfo setHistoryLength(long historyLength) {
this.historyLength = historyLength;
setHistoryLengthIsSet(true);
return this;
}
public void unsetHistoryLength() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __HISTORYLENGTH_ISSET_ID);
}
/** Returns true if field historyLength is set (has been assigned a value) and false otherwise */
public boolean isSetHistoryLength() {
return EncodingUtils.testBit(__isset_bitfield, __HISTORYLENGTH_ISSET_ID);
}
public void setHistoryLengthIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __HISTORYLENGTH_ISSET_ID, value);
}
public String getParentDomainId() {
return this.parentDomainId;
}
public WorkflowExecutionInfo setParentDomainId(String parentDomainId) {
this.parentDomainId = parentDomainId;
return this;
}
public void unsetParentDomainId() {
this.parentDomainId = null;
}
/** Returns true if field parentDomainId is set (has been assigned a value) and false otherwise */
public boolean isSetParentDomainId() {
return this.parentDomainId != null;
}
public void setParentDomainIdIsSet(boolean value) {
if (!value) {
this.parentDomainId = null;
}
}
public WorkflowExecution getParentExecution() {
return this.parentExecution;
}
public WorkflowExecutionInfo setParentExecution(WorkflowExecution parentExecution) {
this.parentExecution = parentExecution;
return this;
}
public void unsetParentExecution() {
this.parentExecution = null;
}
/** Returns true if field parentExecution is set (has been assigned a value) and false otherwise */
public boolean isSetParentExecution() {
return this.parentExecution != null;
}
public void setParentExecutionIsSet(boolean value) {
if (!value) {
this.parentExecution = null;
}
}
public long getExecutionTime() {
return this.executionTime;
}
public WorkflowExecutionInfo setExecutionTime(long executionTime) {
this.executionTime = executionTime;
setExecutionTimeIsSet(true);
return this;
}
public void unsetExecutionTime() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __EXECUTIONTIME_ISSET_ID);
}
/** Returns true if field executionTime is set (has been assigned a value) and false otherwise */
public boolean isSetExecutionTime() {
return EncodingUtils.testBit(__isset_bitfield, __EXECUTIONTIME_ISSET_ID);
}
public void setExecutionTimeIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __EXECUTIONTIME_ISSET_ID, value);
}
public Memo getMemo() {
return this.memo;
}
public WorkflowExecutionInfo setMemo(Memo memo) {
this.memo = memo;
return this;
}
public void unsetMemo() {
this.memo = null;
}
/** Returns true if field memo is set (has been assigned a value) and false otherwise */
public boolean isSetMemo() {
return this.memo != null;
}
public void setMemoIsSet(boolean value) {
if (!value) {
this.memo = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case EXECUTION:
if (value == null) {
unsetExecution();
} else {
setExecution((WorkflowExecution)value);
}
break;
case TYPE:
if (value == null) {
unsetType();
} else {
setType((WorkflowType)value);
}
break;
case START_TIME:
if (value == null) {
unsetStartTime();
} else {
setStartTime((Long)value);
}
break;
case CLOSE_TIME:
if (value == null) {
unsetCloseTime();
} else {
setCloseTime((Long)value);
}
break;
case CLOSE_STATUS:
if (value == null) {
unsetCloseStatus();
} else {
setCloseStatus((WorkflowExecutionCloseStatus)value);
}
break;
case HISTORY_LENGTH:
if (value == null) {
unsetHistoryLength();
} else {
setHistoryLength((Long)value);
}
break;
case PARENT_DOMAIN_ID:
if (value == null) {
unsetParentDomainId();
} else {
setParentDomainId((String)value);
}
break;
case PARENT_EXECUTION:
if (value == null) {
unsetParentExecution();
} else {
setParentExecution((WorkflowExecution)value);
}
break;
case EXECUTION_TIME:
if (value == null) {
unsetExecutionTime();
} else {
setExecutionTime((Long)value);
}
break;
case MEMO:
if (value == null) {
unsetMemo();
} else {
setMemo((Memo)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case EXECUTION:
return getExecution();
case TYPE:
return getType();
case START_TIME:
return getStartTime();
case CLOSE_TIME:
return getCloseTime();
case CLOSE_STATUS:
return getCloseStatus();
case HISTORY_LENGTH:
return getHistoryLength();
case PARENT_DOMAIN_ID:
return getParentDomainId();
case PARENT_EXECUTION:
return getParentExecution();
case EXECUTION_TIME:
return getExecutionTime();
case MEMO:
return getMemo();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case EXECUTION:
return isSetExecution();
case TYPE:
return isSetType();
case START_TIME:
return isSetStartTime();
case CLOSE_TIME:
return isSetCloseTime();
case CLOSE_STATUS:
return isSetCloseStatus();
case HISTORY_LENGTH:
return isSetHistoryLength();
case PARENT_DOMAIN_ID:
return isSetParentDomainId();
case PARENT_EXECUTION:
return isSetParentExecution();
case EXECUTION_TIME:
return isSetExecutionTime();
case MEMO:
return isSetMemo();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof WorkflowExecutionInfo)
return this.equals((WorkflowExecutionInfo)that);
return false;
}
public boolean equals(WorkflowExecutionInfo that) {
if (that == null)
return false;
boolean this_present_execution = true && this.isSetExecution();
boolean that_present_execution = true && that.isSetExecution();
if (this_present_execution || that_present_execution) {
if (!(this_present_execution && that_present_execution))
return false;
if (!this.execution.equals(that.execution))
return false;
}
boolean this_present_type = true && this.isSetType();
boolean that_present_type = true && that.isSetType();
if (this_present_type || that_present_type) {
if (!(this_present_type && that_present_type))
return false;
if (!this.type.equals(that.type))
return false;
}
boolean this_present_startTime = true && this.isSetStartTime();
boolean that_present_startTime = true && that.isSetStartTime();
if (this_present_startTime || that_present_startTime) {
if (!(this_present_startTime && that_present_startTime))
return false;
if (this.startTime != that.startTime)
return false;
}
boolean this_present_closeTime = true && this.isSetCloseTime();
boolean that_present_closeTime = true && that.isSetCloseTime();
if (this_present_closeTime || that_present_closeTime) {
if (!(this_present_closeTime && that_present_closeTime))
return false;
if (this.closeTime != that.closeTime)
return false;
}
boolean this_present_closeStatus = true && this.isSetCloseStatus();
boolean that_present_closeStatus = true && that.isSetCloseStatus();
if (this_present_closeStatus || that_present_closeStatus) {
if (!(this_present_closeStatus && that_present_closeStatus))
return false;
if (!this.closeStatus.equals(that.closeStatus))
return false;
}
boolean this_present_historyLength = true && this.isSetHistoryLength();
boolean that_present_historyLength = true && that.isSetHistoryLength();
if (this_present_historyLength || that_present_historyLength) {
if (!(this_present_historyLength && that_present_historyLength))
return false;
if (this.historyLength != that.historyLength)
return false;
}
boolean this_present_parentDomainId = true && this.isSetParentDomainId();
boolean that_present_parentDomainId = true && that.isSetParentDomainId();
if (this_present_parentDomainId || that_present_parentDomainId) {
if (!(this_present_parentDomainId && that_present_parentDomainId))
return false;
if (!this.parentDomainId.equals(that.parentDomainId))
return false;
}
boolean this_present_parentExecution = true && this.isSetParentExecution();
boolean that_present_parentExecution = true && that.isSetParentExecution();
if (this_present_parentExecution || that_present_parentExecution) {
if (!(this_present_parentExecution && that_present_parentExecution))
return false;
if (!this.parentExecution.equals(that.parentExecution))
return false;
}
boolean this_present_executionTime = true && this.isSetExecutionTime();
boolean that_present_executionTime = true && that.isSetExecutionTime();
if (this_present_executionTime || that_present_executionTime) {
if (!(this_present_executionTime && that_present_executionTime))
return false;
if (this.executionTime != that.executionTime)
return false;
}
boolean this_present_memo = true && this.isSetMemo();
boolean that_present_memo = true && that.isSetMemo();
if (this_present_memo || that_present_memo) {
if (!(this_present_memo && that_present_memo))
return false;
if (!this.memo.equals(that.memo))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy