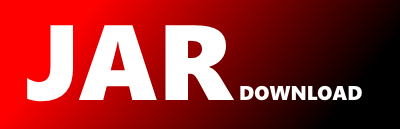
com.uber.hoodie.avro.model.HoodieWriteStat Maven / Gradle / Ivy
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package com.uber.hoodie.avro.model;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class HoodieWriteStat extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"HoodieWriteStat\",\"namespace\":\"com.uber.hoodie.avro.model\",\"fields\":[{\"name\":\"fileId\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"path\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"prevCommit\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"numWrites\",\"type\":[\"null\",\"long\"]},{\"name\":\"numDeletes\",\"type\":[\"null\",\"long\"]},{\"name\":\"numUpdateWrites\",\"type\":[\"null\",\"long\"]},{\"name\":\"totalWriteBytes\",\"type\":[\"null\",\"long\"]},{\"name\":\"totalWriteErrors\",\"type\":[\"null\",\"long\"]},{\"name\":\"partitionPath\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}]},{\"name\":\"totalLogRecords\",\"type\":[\"null\",\"long\"]},{\"name\":\"totalLogFiles\",\"type\":[\"null\",\"long\"]},{\"name\":\"totalRecordsToBeUpdate\",\"type\":[\"null\",\"long\"]}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
@Deprecated public java.lang.String fileId;
@Deprecated public java.lang.String path;
@Deprecated public java.lang.String prevCommit;
@Deprecated public java.lang.Long numWrites;
@Deprecated public java.lang.Long numDeletes;
@Deprecated public java.lang.Long numUpdateWrites;
@Deprecated public java.lang.Long totalWriteBytes;
@Deprecated public java.lang.Long totalWriteErrors;
@Deprecated public java.lang.String partitionPath;
@Deprecated public java.lang.Long totalLogRecords;
@Deprecated public java.lang.Long totalLogFiles;
@Deprecated public java.lang.Long totalRecordsToBeUpdate;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public HoodieWriteStat() {}
/**
* All-args constructor.
*/
public HoodieWriteStat(java.lang.String fileId, java.lang.String path, java.lang.String prevCommit, java.lang.Long numWrites, java.lang.Long numDeletes, java.lang.Long numUpdateWrites, java.lang.Long totalWriteBytes, java.lang.Long totalWriteErrors, java.lang.String partitionPath, java.lang.Long totalLogRecords, java.lang.Long totalLogFiles, java.lang.Long totalRecordsToBeUpdate) {
this.fileId = fileId;
this.path = path;
this.prevCommit = prevCommit;
this.numWrites = numWrites;
this.numDeletes = numDeletes;
this.numUpdateWrites = numUpdateWrites;
this.totalWriteBytes = totalWriteBytes;
this.totalWriteErrors = totalWriteErrors;
this.partitionPath = partitionPath;
this.totalLogRecords = totalLogRecords;
this.totalLogFiles = totalLogFiles;
this.totalRecordsToBeUpdate = totalRecordsToBeUpdate;
}
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return fileId;
case 1: return path;
case 2: return prevCommit;
case 3: return numWrites;
case 4: return numDeletes;
case 5: return numUpdateWrites;
case 6: return totalWriteBytes;
case 7: return totalWriteErrors;
case 8: return partitionPath;
case 9: return totalLogRecords;
case 10: return totalLogFiles;
case 11: return totalRecordsToBeUpdate;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: fileId = (java.lang.String)value$; break;
case 1: path = (java.lang.String)value$; break;
case 2: prevCommit = (java.lang.String)value$; break;
case 3: numWrites = (java.lang.Long)value$; break;
case 4: numDeletes = (java.lang.Long)value$; break;
case 5: numUpdateWrites = (java.lang.Long)value$; break;
case 6: totalWriteBytes = (java.lang.Long)value$; break;
case 7: totalWriteErrors = (java.lang.Long)value$; break;
case 8: partitionPath = (java.lang.String)value$; break;
case 9: totalLogRecords = (java.lang.Long)value$; break;
case 10: totalLogFiles = (java.lang.Long)value$; break;
case 11: totalRecordsToBeUpdate = (java.lang.Long)value$; break;
default: throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'fileId' field.
*/
public java.lang.String getFileId() {
return fileId;
}
/**
* Sets the value of the 'fileId' field.
* @param value the value to set.
*/
public void setFileId(java.lang.String value) {
this.fileId = value;
}
/**
* Gets the value of the 'path' field.
*/
public java.lang.String getPath() {
return path;
}
/**
* Sets the value of the 'path' field.
* @param value the value to set.
*/
public void setPath(java.lang.String value) {
this.path = value;
}
/**
* Gets the value of the 'prevCommit' field.
*/
public java.lang.String getPrevCommit() {
return prevCommit;
}
/**
* Sets the value of the 'prevCommit' field.
* @param value the value to set.
*/
public void setPrevCommit(java.lang.String value) {
this.prevCommit = value;
}
/**
* Gets the value of the 'numWrites' field.
*/
public java.lang.Long getNumWrites() {
return numWrites;
}
/**
* Sets the value of the 'numWrites' field.
* @param value the value to set.
*/
public void setNumWrites(java.lang.Long value) {
this.numWrites = value;
}
/**
* Gets the value of the 'numDeletes' field.
*/
public java.lang.Long getNumDeletes() {
return numDeletes;
}
/**
* Sets the value of the 'numDeletes' field.
* @param value the value to set.
*/
public void setNumDeletes(java.lang.Long value) {
this.numDeletes = value;
}
/**
* Gets the value of the 'numUpdateWrites' field.
*/
public java.lang.Long getNumUpdateWrites() {
return numUpdateWrites;
}
/**
* Sets the value of the 'numUpdateWrites' field.
* @param value the value to set.
*/
public void setNumUpdateWrites(java.lang.Long value) {
this.numUpdateWrites = value;
}
/**
* Gets the value of the 'totalWriteBytes' field.
*/
public java.lang.Long getTotalWriteBytes() {
return totalWriteBytes;
}
/**
* Sets the value of the 'totalWriteBytes' field.
* @param value the value to set.
*/
public void setTotalWriteBytes(java.lang.Long value) {
this.totalWriteBytes = value;
}
/**
* Gets the value of the 'totalWriteErrors' field.
*/
public java.lang.Long getTotalWriteErrors() {
return totalWriteErrors;
}
/**
* Sets the value of the 'totalWriteErrors' field.
* @param value the value to set.
*/
public void setTotalWriteErrors(java.lang.Long value) {
this.totalWriteErrors = value;
}
/**
* Gets the value of the 'partitionPath' field.
*/
public java.lang.String getPartitionPath() {
return partitionPath;
}
/**
* Sets the value of the 'partitionPath' field.
* @param value the value to set.
*/
public void setPartitionPath(java.lang.String value) {
this.partitionPath = value;
}
/**
* Gets the value of the 'totalLogRecords' field.
*/
public java.lang.Long getTotalLogRecords() {
return totalLogRecords;
}
/**
* Sets the value of the 'totalLogRecords' field.
* @param value the value to set.
*/
public void setTotalLogRecords(java.lang.Long value) {
this.totalLogRecords = value;
}
/**
* Gets the value of the 'totalLogFiles' field.
*/
public java.lang.Long getTotalLogFiles() {
return totalLogFiles;
}
/**
* Sets the value of the 'totalLogFiles' field.
* @param value the value to set.
*/
public void setTotalLogFiles(java.lang.Long value) {
this.totalLogFiles = value;
}
/**
* Gets the value of the 'totalRecordsToBeUpdate' field.
*/
public java.lang.Long getTotalRecordsToBeUpdate() {
return totalRecordsToBeUpdate;
}
/**
* Sets the value of the 'totalRecordsToBeUpdate' field.
* @param value the value to set.
*/
public void setTotalRecordsToBeUpdate(java.lang.Long value) {
this.totalRecordsToBeUpdate = value;
}
/** Creates a new HoodieWriteStat RecordBuilder */
public static com.uber.hoodie.avro.model.HoodieWriteStat.Builder newBuilder() {
return new com.uber.hoodie.avro.model.HoodieWriteStat.Builder();
}
/** Creates a new HoodieWriteStat RecordBuilder by copying an existing Builder */
public static com.uber.hoodie.avro.model.HoodieWriteStat.Builder newBuilder(com.uber.hoodie.avro.model.HoodieWriteStat.Builder other) {
return new com.uber.hoodie.avro.model.HoodieWriteStat.Builder(other);
}
/** Creates a new HoodieWriteStat RecordBuilder by copying an existing HoodieWriteStat instance */
public static com.uber.hoodie.avro.model.HoodieWriteStat.Builder newBuilder(com.uber.hoodie.avro.model.HoodieWriteStat other) {
return new com.uber.hoodie.avro.model.HoodieWriteStat.Builder(other);
}
/**
* RecordBuilder for HoodieWriteStat instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.String fileId;
private java.lang.String path;
private java.lang.String prevCommit;
private java.lang.Long numWrites;
private java.lang.Long numDeletes;
private java.lang.Long numUpdateWrites;
private java.lang.Long totalWriteBytes;
private java.lang.Long totalWriteErrors;
private java.lang.String partitionPath;
private java.lang.Long totalLogRecords;
private java.lang.Long totalLogFiles;
private java.lang.Long totalRecordsToBeUpdate;
/** Creates a new Builder */
private Builder() {
super(com.uber.hoodie.avro.model.HoodieWriteStat.SCHEMA$);
}
/** Creates a Builder by copying an existing Builder */
private Builder(com.uber.hoodie.avro.model.HoodieWriteStat.Builder other) {
super(other);
if (isValidValue(fields()[0], other.fileId)) {
this.fileId = data().deepCopy(fields()[0].schema(), other.fileId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.path)) {
this.path = data().deepCopy(fields()[1].schema(), other.path);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.prevCommit)) {
this.prevCommit = data().deepCopy(fields()[2].schema(), other.prevCommit);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.numWrites)) {
this.numWrites = data().deepCopy(fields()[3].schema(), other.numWrites);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.numDeletes)) {
this.numDeletes = data().deepCopy(fields()[4].schema(), other.numDeletes);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.numUpdateWrites)) {
this.numUpdateWrites = data().deepCopy(fields()[5].schema(), other.numUpdateWrites);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.totalWriteBytes)) {
this.totalWriteBytes = data().deepCopy(fields()[6].schema(), other.totalWriteBytes);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.totalWriteErrors)) {
this.totalWriteErrors = data().deepCopy(fields()[7].schema(), other.totalWriteErrors);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.partitionPath)) {
this.partitionPath = data().deepCopy(fields()[8].schema(), other.partitionPath);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.totalLogRecords)) {
this.totalLogRecords = data().deepCopy(fields()[9].schema(), other.totalLogRecords);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.totalLogFiles)) {
this.totalLogFiles = data().deepCopy(fields()[10].schema(), other.totalLogFiles);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.totalRecordsToBeUpdate)) {
this.totalRecordsToBeUpdate = data().deepCopy(fields()[11].schema(), other.totalRecordsToBeUpdate);
fieldSetFlags()[11] = true;
}
}
/** Creates a Builder by copying an existing HoodieWriteStat instance */
private Builder(com.uber.hoodie.avro.model.HoodieWriteStat other) {
super(com.uber.hoodie.avro.model.HoodieWriteStat.SCHEMA$);
if (isValidValue(fields()[0], other.fileId)) {
this.fileId = data().deepCopy(fields()[0].schema(), other.fileId);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.path)) {
this.path = data().deepCopy(fields()[1].schema(), other.path);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.prevCommit)) {
this.prevCommit = data().deepCopy(fields()[2].schema(), other.prevCommit);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.numWrites)) {
this.numWrites = data().deepCopy(fields()[3].schema(), other.numWrites);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.numDeletes)) {
this.numDeletes = data().deepCopy(fields()[4].schema(), other.numDeletes);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.numUpdateWrites)) {
this.numUpdateWrites = data().deepCopy(fields()[5].schema(), other.numUpdateWrites);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.totalWriteBytes)) {
this.totalWriteBytes = data().deepCopy(fields()[6].schema(), other.totalWriteBytes);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.totalWriteErrors)) {
this.totalWriteErrors = data().deepCopy(fields()[7].schema(), other.totalWriteErrors);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.partitionPath)) {
this.partitionPath = data().deepCopy(fields()[8].schema(), other.partitionPath);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.totalLogRecords)) {
this.totalLogRecords = data().deepCopy(fields()[9].schema(), other.totalLogRecords);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.totalLogFiles)) {
this.totalLogFiles = data().deepCopy(fields()[10].schema(), other.totalLogFiles);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.totalRecordsToBeUpdate)) {
this.totalRecordsToBeUpdate = data().deepCopy(fields()[11].schema(), other.totalRecordsToBeUpdate);
fieldSetFlags()[11] = true;
}
}
/** Gets the value of the 'fileId' field */
public java.lang.String getFileId() {
return fileId;
}
/** Sets the value of the 'fileId' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setFileId(java.lang.String value) {
validate(fields()[0], value);
this.fileId = value;
fieldSetFlags()[0] = true;
return this;
}
/** Checks whether the 'fileId' field has been set */
public boolean hasFileId() {
return fieldSetFlags()[0];
}
/** Clears the value of the 'fileId' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearFileId() {
fileId = null;
fieldSetFlags()[0] = false;
return this;
}
/** Gets the value of the 'path' field */
public java.lang.String getPath() {
return path;
}
/** Sets the value of the 'path' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setPath(java.lang.String value) {
validate(fields()[1], value);
this.path = value;
fieldSetFlags()[1] = true;
return this;
}
/** Checks whether the 'path' field has been set */
public boolean hasPath() {
return fieldSetFlags()[1];
}
/** Clears the value of the 'path' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearPath() {
path = null;
fieldSetFlags()[1] = false;
return this;
}
/** Gets the value of the 'prevCommit' field */
public java.lang.String getPrevCommit() {
return prevCommit;
}
/** Sets the value of the 'prevCommit' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setPrevCommit(java.lang.String value) {
validate(fields()[2], value);
this.prevCommit = value;
fieldSetFlags()[2] = true;
return this;
}
/** Checks whether the 'prevCommit' field has been set */
public boolean hasPrevCommit() {
return fieldSetFlags()[2];
}
/** Clears the value of the 'prevCommit' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearPrevCommit() {
prevCommit = null;
fieldSetFlags()[2] = false;
return this;
}
/** Gets the value of the 'numWrites' field */
public java.lang.Long getNumWrites() {
return numWrites;
}
/** Sets the value of the 'numWrites' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setNumWrites(java.lang.Long value) {
validate(fields()[3], value);
this.numWrites = value;
fieldSetFlags()[3] = true;
return this;
}
/** Checks whether the 'numWrites' field has been set */
public boolean hasNumWrites() {
return fieldSetFlags()[3];
}
/** Clears the value of the 'numWrites' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearNumWrites() {
numWrites = null;
fieldSetFlags()[3] = false;
return this;
}
/** Gets the value of the 'numDeletes' field */
public java.lang.Long getNumDeletes() {
return numDeletes;
}
/** Sets the value of the 'numDeletes' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setNumDeletes(java.lang.Long value) {
validate(fields()[4], value);
this.numDeletes = value;
fieldSetFlags()[4] = true;
return this;
}
/** Checks whether the 'numDeletes' field has been set */
public boolean hasNumDeletes() {
return fieldSetFlags()[4];
}
/** Clears the value of the 'numDeletes' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearNumDeletes() {
numDeletes = null;
fieldSetFlags()[4] = false;
return this;
}
/** Gets the value of the 'numUpdateWrites' field */
public java.lang.Long getNumUpdateWrites() {
return numUpdateWrites;
}
/** Sets the value of the 'numUpdateWrites' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setNumUpdateWrites(java.lang.Long value) {
validate(fields()[5], value);
this.numUpdateWrites = value;
fieldSetFlags()[5] = true;
return this;
}
/** Checks whether the 'numUpdateWrites' field has been set */
public boolean hasNumUpdateWrites() {
return fieldSetFlags()[5];
}
/** Clears the value of the 'numUpdateWrites' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearNumUpdateWrites() {
numUpdateWrites = null;
fieldSetFlags()[5] = false;
return this;
}
/** Gets the value of the 'totalWriteBytes' field */
public java.lang.Long getTotalWriteBytes() {
return totalWriteBytes;
}
/** Sets the value of the 'totalWriteBytes' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setTotalWriteBytes(java.lang.Long value) {
validate(fields()[6], value);
this.totalWriteBytes = value;
fieldSetFlags()[6] = true;
return this;
}
/** Checks whether the 'totalWriteBytes' field has been set */
public boolean hasTotalWriteBytes() {
return fieldSetFlags()[6];
}
/** Clears the value of the 'totalWriteBytes' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearTotalWriteBytes() {
totalWriteBytes = null;
fieldSetFlags()[6] = false;
return this;
}
/** Gets the value of the 'totalWriteErrors' field */
public java.lang.Long getTotalWriteErrors() {
return totalWriteErrors;
}
/** Sets the value of the 'totalWriteErrors' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setTotalWriteErrors(java.lang.Long value) {
validate(fields()[7], value);
this.totalWriteErrors = value;
fieldSetFlags()[7] = true;
return this;
}
/** Checks whether the 'totalWriteErrors' field has been set */
public boolean hasTotalWriteErrors() {
return fieldSetFlags()[7];
}
/** Clears the value of the 'totalWriteErrors' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearTotalWriteErrors() {
totalWriteErrors = null;
fieldSetFlags()[7] = false;
return this;
}
/** Gets the value of the 'partitionPath' field */
public java.lang.String getPartitionPath() {
return partitionPath;
}
/** Sets the value of the 'partitionPath' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setPartitionPath(java.lang.String value) {
validate(fields()[8], value);
this.partitionPath = value;
fieldSetFlags()[8] = true;
return this;
}
/** Checks whether the 'partitionPath' field has been set */
public boolean hasPartitionPath() {
return fieldSetFlags()[8];
}
/** Clears the value of the 'partitionPath' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearPartitionPath() {
partitionPath = null;
fieldSetFlags()[8] = false;
return this;
}
/** Gets the value of the 'totalLogRecords' field */
public java.lang.Long getTotalLogRecords() {
return totalLogRecords;
}
/** Sets the value of the 'totalLogRecords' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setTotalLogRecords(java.lang.Long value) {
validate(fields()[9], value);
this.totalLogRecords = value;
fieldSetFlags()[9] = true;
return this;
}
/** Checks whether the 'totalLogRecords' field has been set */
public boolean hasTotalLogRecords() {
return fieldSetFlags()[9];
}
/** Clears the value of the 'totalLogRecords' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearTotalLogRecords() {
totalLogRecords = null;
fieldSetFlags()[9] = false;
return this;
}
/** Gets the value of the 'totalLogFiles' field */
public java.lang.Long getTotalLogFiles() {
return totalLogFiles;
}
/** Sets the value of the 'totalLogFiles' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setTotalLogFiles(java.lang.Long value) {
validate(fields()[10], value);
this.totalLogFiles = value;
fieldSetFlags()[10] = true;
return this;
}
/** Checks whether the 'totalLogFiles' field has been set */
public boolean hasTotalLogFiles() {
return fieldSetFlags()[10];
}
/** Clears the value of the 'totalLogFiles' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearTotalLogFiles() {
totalLogFiles = null;
fieldSetFlags()[10] = false;
return this;
}
/** Gets the value of the 'totalRecordsToBeUpdate' field */
public java.lang.Long getTotalRecordsToBeUpdate() {
return totalRecordsToBeUpdate;
}
/** Sets the value of the 'totalRecordsToBeUpdate' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder setTotalRecordsToBeUpdate(java.lang.Long value) {
validate(fields()[11], value);
this.totalRecordsToBeUpdate = value;
fieldSetFlags()[11] = true;
return this;
}
/** Checks whether the 'totalRecordsToBeUpdate' field has been set */
public boolean hasTotalRecordsToBeUpdate() {
return fieldSetFlags()[11];
}
/** Clears the value of the 'totalRecordsToBeUpdate' field */
public com.uber.hoodie.avro.model.HoodieWriteStat.Builder clearTotalRecordsToBeUpdate() {
totalRecordsToBeUpdate = null;
fieldSetFlags()[11] = false;
return this;
}
@Override
public HoodieWriteStat build() {
try {
HoodieWriteStat record = new HoodieWriteStat();
record.fileId = fieldSetFlags()[0] ? this.fileId : (java.lang.String) defaultValue(fields()[0]);
record.path = fieldSetFlags()[1] ? this.path : (java.lang.String) defaultValue(fields()[1]);
record.prevCommit = fieldSetFlags()[2] ? this.prevCommit : (java.lang.String) defaultValue(fields()[2]);
record.numWrites = fieldSetFlags()[3] ? this.numWrites : (java.lang.Long) defaultValue(fields()[3]);
record.numDeletes = fieldSetFlags()[4] ? this.numDeletes : (java.lang.Long) defaultValue(fields()[4]);
record.numUpdateWrites = fieldSetFlags()[5] ? this.numUpdateWrites : (java.lang.Long) defaultValue(fields()[5]);
record.totalWriteBytes = fieldSetFlags()[6] ? this.totalWriteBytes : (java.lang.Long) defaultValue(fields()[6]);
record.totalWriteErrors = fieldSetFlags()[7] ? this.totalWriteErrors : (java.lang.Long) defaultValue(fields()[7]);
record.partitionPath = fieldSetFlags()[8] ? this.partitionPath : (java.lang.String) defaultValue(fields()[8]);
record.totalLogRecords = fieldSetFlags()[9] ? this.totalLogRecords : (java.lang.Long) defaultValue(fields()[9]);
record.totalLogFiles = fieldSetFlags()[10] ? this.totalLogFiles : (java.lang.Long) defaultValue(fields()[10]);
record.totalRecordsToBeUpdate = fieldSetFlags()[11] ? this.totalRecordsToBeUpdate : (java.lang.Long) defaultValue(fields()[11]);
return record;
} catch (Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy