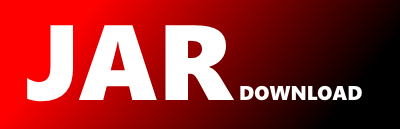
com.uber.jaeger.PropagationRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaeger-core Show documentation
Show all versions of jaeger-core Show documentation
Jaeger Java bindings for OpenTracing API
/*
* Copyright (c) 2018, The Jaeger Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.uber.jaeger;
import com.uber.jaeger.propagation.Extractor;
import com.uber.jaeger.propagation.Injector;
import io.opentracing.propagation.Format;
import java.util.HashMap;
import java.util.Map;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
class PropagationRegistry {
private final Map, Injector>> injectors = new HashMap, Injector>>();
private final Map, Extractor>> extractors = new HashMap, Extractor>>();
@SuppressWarnings("unchecked")
Injector getInjector(Format format) {
return (Injector) injectors.get(format);
}
@SuppressWarnings("unchecked")
Extractor getExtractor(Format format) {
return (Extractor) extractors.get(format);
}
public void register(Format format, Injector injector) {
injectors.put(format, new ExceptionCatchingInjectorDecorator(injector));
}
public void register(Format format, Extractor extractor) {
extractors.put(format, new ExceptionCatchingExtractorDecorator(extractor));
}
@RequiredArgsConstructor
@Slf4j
private static class ExceptionCatchingExtractorDecorator implements Extractor {
private final Extractor decorated;
@Override
public SpanContext extract(T carrier) {
try {
return decorated.extract(carrier);
} catch (RuntimeException ex) {
ExceptionCatchingExtractorDecorator.log
.warn("Error when extracting SpanContext from carrier. Handling gracefully.", ex);
return null;
}
}
}
@RequiredArgsConstructor
@Slf4j
private static class ExceptionCatchingInjectorDecorator implements Injector {
private final Injector decorated;
@Override
public void inject(SpanContext spanContext, T carrier) {
try {
decorated.inject(spanContext, carrier);
} catch (RuntimeException ex) {
ExceptionCatchingInjectorDecorator.log
.error("Error when injecting SpanContext into carrier. Handling gracefully.", ex);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy