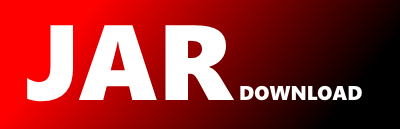
com.uber.infer.task.PrepareForInfer.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infer-plugin Show documentation
Show all versions of infer-plugin Show documentation
Gradle integration for infer static analyzer.
The newest version!
package com.uber.infer.task
import com.uber.infer.util.JavacUtils
import com.uber.infer.util.RunCommandUtils
import groovy.json.JsonBuilder
import org.gradle.api.DefaultTask
import org.gradle.api.artifacts.UnknownConfigurationException
import org.gradle.api.file.FileCollection
import org.gradle.api.internal.file.TmpDirTemporaryFileProvider
import org.gradle.api.tasks.Input
import org.gradle.api.tasks.TaskAction
import java.nio.file.Path
import java.nio.file.Paths
/**
* Responsible for capturing Infer metadata for future analysis.
*/
public class PrepareForInfer extends DefaultTask {
// For creating a config...
@Input Closure eradicateExclude
@Input Closure eradicateInclude
@Input Closure inferExclude
@Input Closure inferInclude
// For capturing...
@Input Closure bootClasspath
@Input Closure compileDependencies
@Input Closure processorDependencies
@Input Closure providedDependencies
@Input Closure sourceFiles
@Input Closure sourceJavaVersion
@Input Closure targetJavaVersion
File classOutputDirectory = new File(getTemporaryDir(), "classes")
File generatedSourceOutputDirectory = new File(getTemporaryDir(), "generated-source")
@TaskAction
def prepareForInfer() {
createInferConfig()
captureInferData()
}
private def captureInferData() {
classOutputDirectory.mkdirs()
generatedSourceOutputDirectory.mkdirs()
def outputDir = new File(project.getBuildDir(), "infer-out")
outputDir.mkdirs()
def result = RunCommandUtils.run("infer -a capture --out ${outputDir.absolutePath}"
+ " -- javac -source ${sourceJavaVersion()} -target ${targetJavaVersion()} " +
"-d ${classOutputDirectory.absolutePath} "
+ "-s ${generatedSourceOutputDirectory.absolutePath} ${getJavacArguments()}", project.projectDir)
if (!result.success) {
throw new RuntimeException("Error capturing Infer data: " + result.stderr)
}
}
private def createInferConfig() {
def eradicateExcludeWithGenerated = eradicateExclude().plus(generatedSourceOutputDirectory)
def inferExcludeWithGenerated = inferExclude().plus(generatedSourceOutputDirectory)
Map config = new HashMap()
config.put("eradicate_blacklist", getPathsArrayInInferFormat(eradicateExcludeWithGenerated))
config.put("eradicate_whitelist", getPathsArrayInInferFormat(eradicateInclude().files))
config.put("infer_blacklist", getPathsArrayInInferFormat(inferExcludeWithGenerated))
config.put("infer_whitelist", getPathsArrayInInferFormat(inferInclude().files))
project.file('.inferConfig').text = new JsonBuilder(config).toPrettyString() + "\n"
project.file('.inferConfig').deleteOnExit()
}
private List getPathsArrayInInferFormat(Collection files) {
List paths = new ArrayList<>();
files.each { file ->
Path pathAbsolute = Paths.get(file.toString())
Path pathBase = Paths.get(project.projectDir.toString())
Path pathRelative = pathBase.relativize(pathAbsolute)
paths.add(pathRelative.toString())
}
return paths
}
/**
* @return Javac arguments to compile the project.
*/
private String getJavacArguments() {
StringBuilder argumentsBuilder = new StringBuilder();
try {
def bootClasspath = bootClasspath()
if (bootClasspath != null) {
argumentsBuilder.append(JavacUtils.generateJavacArgument(bootClasspath, '-bootclasspath'))
}
} catch (UnknownConfigurationException ignored) {
}
def processorDependenciesList = project.files();
try {
processorDependenciesList += processorDependencies()
} catch (UnknownConfigurationException ignored) {
}
try {
processorDependenciesList += providedDependencies()
} catch (UnknownConfigurationException ignored) {
}
if (!processorDependenciesList.isEmpty()) {
argumentsBuilder.append(JavacUtils.generateJavacArgument(processorDependenciesList, '-processorpath'))
}
argumentsBuilder.append(JavacUtils.generateJavacArgument(compileDependencies() + providedDependencies(),
"-classpath"))
String sourceFileText = sourceFiles().join('\n')
TmpDirTemporaryFileProvider provider = new TmpDirTemporaryFileProvider()
File inferSourceFiles = provider.createTemporaryFile("infer", "sourceFiles", "/tmp")
inferSourceFiles.deleteOnExit()
inferSourceFiles.text = sourceFileText
argumentsBuilder.append("@${inferSourceFiles.absolutePath}")
return argumentsBuilder.toString()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy