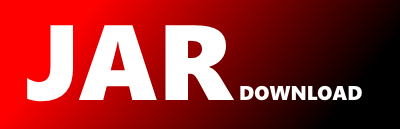
com.ufoscout.vertk.web.K.kt Maven / Gradle / Ivy
package com.ufoscout.vertk.web
import io.vertx.core.Vertx
import io.vertx.core.buffer.Buffer
import io.vertx.core.http.HttpServerResponse
import io.vertx.core.json.Json
import io.vertx.ext.web.Route
import io.vertx.ext.web.Router
import io.vertx.ext.web.RoutingContext
import io.vertx.kotlin.coroutines.awaitEvent
import io.vertx.kotlin.coroutines.dispatcher
import kotlinx.coroutines.experimental.launch
import java.lang.Exception
inline fun HttpServerResponse.endWithJson(obj: Any) {
this.putHeader("Content-Type", "application/json; charset=utf-8").end(Json.encode(obj))
}
inline fun Route.awaitHandler(noinline handler: suspend (rc: RoutingContext) -> Any) {
this.handler {
launch(Vertx.currentContext().dispatcher()) {
try {
handler(it)
} catch (e: Exception) {
it.fail(e)
}
}
}
}
fun Router.awaitRestDelete(path: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.delete(path), handler)
}
fun Router.awaitRestDeleteWithRegex(regex: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.deleteWithRegex(regex), handler)
}
fun Router.awaitRestGet(path: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.get(path), handler)
}
fun Router.awaitRestGetWithRegex(regex: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.getWithRegex(regex), handler)
}
fun Router.awaitRestHeadWithRegex(regex: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.headWithRegex(regex), handler)
}
fun Router.awaitRestOptions(path: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.options(path), handler)
}
fun Router.awaitRestOptionsWithRegex(regex: String, handler: suspend (rc: RoutingContext) -> Any) {
rest(this.optionsWithRegex(regex), handler)
}
inline fun Router.awaitRestPatch(path: String, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
restWithBody(this.patch(path), handler)
}
inline fun Router.awaitRestPatchWithRegex(regex: String, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
restWithBody(this.patchWithRegex(regex), handler)
}
inline fun Router.awaitRestPost(path: String, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
restWithBody(this.post(path), handler)
}
inline fun Router.awaitRestPostWithRegex(regex: String, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
restWithBody(this.postWithRegex(regex), handler)
}
inline fun Router.awaitRestPut(path: String, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
restWithBody(this.put(path), handler)
}
inline fun Router.awaitRestPutWithRegex(regex: String, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
restWithBody(this.putWithRegex(regex), handler)
}
fun rest(route: Route, handler: suspend (rc: RoutingContext) -> Any) {
route
.produces("application/json")
.awaitHandler({
val result = handler(it)
var resultJson = if (result is String) {
result
} else {
Json.encode(result)
}
it.response().putHeader("Content-Type", "application/json; charset=utf-8").end(resultJson)
})
}
inline fun restWithBody(route: Route, noinline handler: suspend (rc: RoutingContext, body: I) -> Any) {
route
.consumes("application/json")
.produces("application/json")
.awaitHandler({ rc ->
val bodyBuffer = awaitEvent {
rc.request().bodyHandler(it)
}
val body = bodyBuffer.toJsonObject().mapTo(I::class.java)
val result = handler(rc, body)
var resultJson = if (result is String) {
result
} else {
Json.encode(result)
}
rc.response().putHeader("Content-Type", "application/json; charset=utf-8").end(resultJson)
})
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy