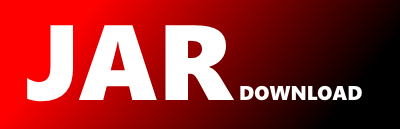
uw.task.TaskData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of uw-task Show documentation
Show all versions of uw-task Show documentation
uw-task包是一个分布式任务框架,通过uw-task可以快速构建基于docker的分布式任务体系,同时支持基于influx的任务运维监控。
The newest version!
package uw.task;
import java.io.Serializable;
import java.util.Date;
/**
* TaskData用于任务执行的传值,以为任务完成后返回结构。 TaskParam和ResultData可通过泛型参数制定具体类型。
* TP,TD应和TaskRunner的泛型参数完全一致,否则会导致运行时出错。
*
* @author axeon
*
*/
public class TaskData implements Serializable {
/**
* serialVersionUID
*/
private static final long serialVersionUID = 1333167065535557828L;
/**
* 任务状态:未设置
*/
public static final int STATUS_UNKNOW = 0;
/**
* 任务状态:成功
*/
public static final int STATUS_SUCCESS = 1;
/**
* 任务状态:程序错误
*/
public static final int STATUS_FAIL_PROGRAM = 2;
/**
* 任务状态:配置错误,如超过流量限制
*/
public static final int STATUS_FAIL_CONFIG = 3;
/**
* 任务状态:第三方接口错误
*/
public static final int STATUS_FAIL_PARTNER = 4;
/**
* 运行模式:本地运行
*/
public static final int RUN_TYPE_LOCAL = 1;
/**
* 运行模式:全局运行
*/
public static final int RUN_TYPE_GLOBAL = 3;
/**
* 运行模式:全局运行RPC返回结果
*/
public static final int RUN_TYPE_GLOBAL_RPC = 5;
/**
* id,此序列值由框架自动生成,无需手工设置。
*/
private long id;
/**
* 关联id,由调用方根据需要设置。
*/
private long refId;
/**
* 需要执行的类名,此数值必须由调用方设置。
*/
private String taskClass = "";
/**
* 执行参数,此数值必须有调用方设置。
*/
private TP taskParam;
/**
* 任务运行类型。由框架设置,无需手工设置。
*/
private int runType;
/**
* 指定运行目标。由框架设置,无需手工设置。
*/
private String runTarget = "";
/**
* 任务运行时主机IP,此信息由框架自动设置。
*/
private String hostIp;
/**
* 任务运行时主机ID(可能为docker的ContainerID),此信息由框架自动设置。
*/
private String hostId;
/**
* 进入队列时间,此信息由框架自动设置。
*/
private Date queueDate;
/**
* 开始消费时间,此信息由框架自动设置。
*/
private Date consumeDate;
/**
* 开始运行时间,此信息由框架自动设置。
*/
private Date runDate;
/**
* 运行结束日期,此信息由框架自动设置。
*/
private Date finishDate;
/**
* 执行信息,用于存储框架自动设置。
*/
private RD resultData;
/**
* 已经执行的次数,此信息由框架自动设置。
*/
private int ranTimes;
/**
* 执行状态,此信息由框架自动设置。
*/
private int status;
/**
* @return the id
*/
public long getId() {
return id;
}
/**
* @param id
* the id to set
*/
public void setId(long id) {
this.id = id;
}
/**
* @return the refId
*/
public long getRefId() {
return refId;
}
/**
* @param refId
* the refId to set
*/
public void setRefId(long refId) {
this.refId = refId;
}
/**
* @return the taskClass
*/
public String getTaskClass() {
return taskClass;
}
/**
* @param taskClass
* the taskClass to set
*/
public void setTaskClass(String taskClass) {
this.taskClass = taskClass;
}
/**
* @return the taskParam
*/
public TP getTaskParam() {
return taskParam;
}
/**
* @param taskParam
* the taskParam to set
*/
public void setTaskParam(TP taskParam) {
this.taskParam = taskParam;
}
/**
* @return the runType
*/
public int getRunType() {
return runType;
}
/**
* @param runType
* the runType to set
*/
public void setRunType(int runType) {
this.runType = runType;
}
/**
* @return the runTarget
*/
public String getRunTarget() {
return runTarget;
}
/**
* @param runTarget
* the runTarget to set
*/
public void setRunTarget(String runTarget) {
this.runTarget = runTarget;
}
/**
* @return the hostIp
*/
public String getHostIp() {
return hostIp;
}
/**
* @param hostIp
* the hostIp to set
*/
public void setHostIp(String hostIp) {
this.hostIp = hostIp;
}
/**
* @return the hostId
*/
public String getHostId() {
return hostId;
}
/**
* @param hostId
* the hostId to set
*/
public void setHostId(String hostId) {
this.hostId = hostId;
}
/**
* @return the queueDate
*/
public Date getQueueDate() {
return queueDate;
}
/**
* @param queueDate
* the queueDate to set
*/
public void setQueueDate(Date queueDate) {
this.queueDate = queueDate;
}
/**
* @return the consumeDate
*/
public Date getConsumeDate() {
return consumeDate;
}
/**
* @param consumeDate
* the consumeDate to set
*/
public void setConsumeDate(Date consumeDate) {
this.consumeDate = consumeDate;
}
/**
* @return the runDate
*/
public Date getRunDate() {
return runDate;
}
/**
* @param runDate
* the runDate to set
*/
public void setRunDate(Date runDate) {
this.runDate = runDate;
}
/**
* @return the finishDate
*/
public Date getFinishDate() {
return finishDate;
}
/**
* @param finishDate
* the finishDate to set
*/
public void setFinishDate(Date finishDate) {
this.finishDate = finishDate;
}
/**
* @return the resultData
*/
public RD getResultData() {
return resultData;
}
/**
* @param resultData
* the resultData to set
*/
public void setResultData(RD resultData) {
this.resultData = resultData;
}
/**
* @return the ranTimes
*/
public int getRanTimes() {
return ranTimes;
}
/**
* @param ranTimes
* the ranTimes to set
*/
public void setRanTimes(int ranTimes) {
this.ranTimes = ranTimes;
}
/**
* @return the status
*/
public int getStatus() {
return status;
}
/**
* @param status
* the status to set
*/
public void setStatus(int status) {
this.status = status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy