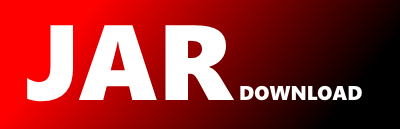
com.unboundid.scim.ldap.Transformation Maven / Gradle / Ivy
/*
* Copyright 2011-2019 Ping Identity Corporation
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License (GPLv2 only)
* or the terms of the GNU Lesser General Public License (LGPLv2.1 only)
* as published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*/
package com.unboundid.scim.ldap;
import com.unboundid.asn1.ASN1OctetString;
import com.unboundid.scim.schema.AttributeDescriptor;
import com.unboundid.scim.sdk.Debug;
import com.unboundid.scim.sdk.SCIMAttributeValue;
import com.unboundid.util.ByteString;
import org.w3c.dom.Element;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Attribute mapping transformations may be used to alter the value of mapped
* attributes. Transformations may be performed on an SCIM attribute value
* when mapping SCIM resources to LDAP entries and vice versa. When no
* transformation class is specified in the resources configuration file,
* the DefaultTransformation implementation is used. It will simply return
* the value as is without any alterations.
*
* Transformations are often useful when the syntax of an attribute is different
* between SCIM and LDAP. The LDAP GeneralizedTime attribute syntax is
* a good example where transformations are necessary when mapping those
* attributes.
*
* To use a custom transformation class, use the transform attribute to
* specify the implementation class in any mapping or
* subMapping configuration elements. For example:
*
*
* <subMapping name="formatted"
* ldapAttribute="postalAddress"
* transform="com.unboundid.scim.ldap.PostalAddressTransformation"
* />
*
*
* This API is volatile and could change in future releases.
*/
public abstract class Transformation
{
/**
* The map of arguments provided to this transformation. This maps the
* element name to its value. See getArguments() for an example.
*/
private final Map arguments = new HashMap();
/**
* Create a transformation from the name of a class that extends the
* {@code Transformation} abstract class.
*
* @param className The name of a class that extends {@code Transformation}.
* @param args The set of arguments that are configured for this
* {@code Transformation}.
*
* @return A new instance of the transformation class.
*/
public static Transformation create(final String className,
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy