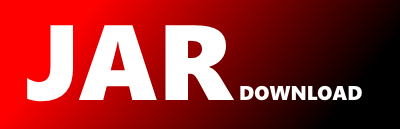
com.unboundid.ldap.sdk.unboundidds.tasks.CollectSupportDataTask Maven / Gradle / Ivy
/*
* Copyright 2020 Ping Identity Corporation
* All Rights Reserved.
*/
/*
* Copyright 2020 Ping Identity Corporation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*
* Copyright (C) 2020 Ping Identity Corporation
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License (GPLv2 only)
* or the terms of the GNU Lesser General Public License (LGPLv2.1 only)
* as published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, see .
*/
package com.unboundid.ldap.sdk.unboundidds.tasks;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import com.unboundid.ldap.sdk.Attribute;
import com.unboundid.ldap.sdk.Entry;
import com.unboundid.util.Debug;
import com.unboundid.util.NotMutable;
import com.unboundid.util.NotNull;
import com.unboundid.util.Nullable;
import com.unboundid.util.StaticUtils;
import com.unboundid.util.ThreadSafety;
import com.unboundid.util.ThreadSafetyLevel;
import com.unboundid.util.args.ArgumentException;
import com.unboundid.util.args.DurationArgument;
import static com.unboundid.ldap.sdk.unboundidds.tasks.TaskMessages.*;
/**
* This class defines a Directory Server task that can be used to invoke the
* collect-support-data tool to capture a variety of information that may help
* monitor the state of the server or diagnose potential problems.
*
*
* NOTE: This class, and other classes within the
* {@code com.unboundid.ldap.sdk.unboundidds} package structure, are only
* supported for use against Ping Identity, UnboundID, and
* Nokia/Alcatel-Lucent 8661 server products. These classes provide support
* for proprietary functionality or for external specifications that are not
* considered stable or mature enough to be guaranteed to work in an
* interoperable way with other types of LDAP servers.
*
*
* The properties that are available for use with this type of task include:
*
* - The path (on the server filesystem) to which the support data archive
* should be written. If this is not provided, then the server will
* determine an appropriate output file to use. If this is provided and
* refers to a file that exists, that file will be overwritten. If this
* is provided and refers to a directory that exists, then a file will
* be created in that directory with a server-generated name. If this
* is provided and refers to a file that does not exist, then its parent
* directory must exist, and a new file will be created with the specified
* path.
* - The path (on the server filesystem) to a file containing the passphrase
* to use to encrypt the contents of the support data archive. If this is
* not provided, then the support data archive will not be encrypted.
* - A flag that indicates whether to include data that may be expensive to
* capture in the support data archive. This information will not be
* included by default.
* - A flag that indicates whether to include a replication state dump
* (which may be several megabytes in size) in the support data
* archive. This information will not be included by default.
* - A flag that indicates whether to include binary files in the support
* data archive. Binary files will not be included by default.
* - A flag that indicates whether to include source code (if available) to
* any third-party extensions installed in the server. Extension source
* code will not be included by default.
* - The data security level to use when redacting data to include in the
* support data archive. If this is not specified, the server will
* select an appropriate security level.
* - A flag that indicates whether to capture items in sequential mode
* (which will use less memory, but at the expense of taking longer to
* complete) rather than in parallel. Support data will be captured in
* parallel by default.
* - The number and duration between intervals for use when collecting
* output of tools (like vmstat, iostat, mpstat, etc.) that use
* sampling over time. If this is not provided, the server will use a
* default count and interval.
* - The number of times to invoke the jstack utility to obtain a stack
* trace of threads running in the JVM. If this is not provided, the
* server will use a default count.
* - The duration (the length of time before the time the task is invoked)
* for log messages to be included in the support data archive. If this
* is not provided, the server will automatically select the amount of
* log content to include.
* - The amount of data in kilobytes to capture from the beginning or end of
* each log file. If this is not provided, the server will automatically
* select the amount of log content to include.
* - An optional comment to include in the support data archive.
*
*/
@NotMutable()
@ThreadSafety(level=ThreadSafetyLevel.COMPLETELY_THREADSAFE)
public final class CollectSupportDataTask
extends Task
{
/**
* The fully-qualified name of the Java class that is used for the collect
* support data task.
*/
@NotNull static final String COLLECT_SUPPORT_DATA_TASK_CLASS =
"com.unboundid.directory.server.tasks.CollectSupportDataTask";
/**
* The prefix that will appear at the beginning of all attribute names used by
* the collect support data task.
*/
@NotNull private static final String ATTR_PREFIX =
"ds-task-collect-support-data-";
/**
* The name of the attribute used to specify a comment to include in the
* support data archive.
*/
@NotNull public static final String ATTR_COMMENT = ATTR_PREFIX + "comment";
/**
* The name of the attribute used to specify the path to a file containing the
* passphrase to use to encrypt the contents of the support data archive.
*/
@NotNull public static final String ATTR_ENCRYPTION_PASSPHRASE_FILE =
ATTR_PREFIX + "encryption-passphrase-file";
/**
* The name of the attribute used to indicate whether the support data archive
* may include binary files that may otherwise have been omitted.
*/
@NotNull public static final String ATTR_INCLUDE_BINARY_FILES =
ATTR_PREFIX + "include-binary-files";
/**
* The name of the attribute used to indicate whether the support data archive
* should include information that may be expensive to capture.
*/
@NotNull public static final String ATTR_INCLUDE_EXPENSIVE_DATA =
ATTR_PREFIX + "include-expensive-data";
/**
* The name of the attribute used to indicate whether the support data archive
* may include the source code (if available) for any third-party extensions
* installed in the server.
*/
@NotNull public static final String ATTR_INCLUDE_EXTENSION_SOURCE =
ATTR_PREFIX + "include-extension-source";
/**
* The name of the attribute used to indicate whether the support data archive
* should include a replication state dump (which may be several megabytes in
* size).
*/
@NotNull public static final String ATTR_INCLUDE_REPLICATION_STATE_DUMP =
ATTR_PREFIX + "include-replication-state-dump";
/**
* The name of the attribute used to specify the number of times to invoke the
* jstack utility to capture server thread stack traces.
*/
@NotNull public static final String ATTR_JSTACK_COUNT =
ATTR_PREFIX + "jstack-count";
/**
* The name of the attribute used to specify the length of time that should be
* covered by the log data included in the support data archive.
*/
@NotNull public static final String ATTR_LOG_DURATION =
ATTR_PREFIX + "log-duration";
/**
* The name of the attribute used to specify the amount of data in kilobytes
* to capture from the beginning of each log file included in the support data
* archive.
*/
@NotNull public static final String ATTR_LOG_FILE_HEAD_COLLECTION_SIZE_KB =
ATTR_PREFIX + "log-file-head-collection-size-kb";
/**
* The name of the attribute used to specify the amount of data in kilobytes
* to capture from the end of each log file included in the support data
* archive.
*/
@NotNull public static final String ATTR_LOG_FILE_TAIL_COLLECTION_SIZE_KB =
ATTR_PREFIX + "log-file-tail-collection-size-kb";
/**
* The name of the attribute used to specify the path to which the support
* data archive should be written.
*/
@NotNull public static final String ATTR_OUTPUT_PATH =
ATTR_PREFIX + "output-path";
/**
* The name of the attribute used to specify the number of intervals to
* capture for tools that capture multiple samples.
*/
@NotNull public static final String ATTR_REPORT_COUNT =
ATTR_PREFIX + "report-count";
/**
* The name of the attribute used to specify the length of time, in seconds,
* between samples collected from tools that capture multiple samples.
*/
@NotNull public static final String ATTR_REPORT_INTERVAL_SECONDS =
ATTR_PREFIX + "report-interval-seconds";
/**
*The name of the attribute used to specify the minimum age of previous
* support data archives that should be retained.
*/
@NotNull public static final String ATTR_RETAIN_PREVIOUS_ARCHIVE_AGE =
ATTR_PREFIX + "retain-previous-support-data-archive-age";
/**
*The name of the attribute used to specify the minimum number of previous
* support data archives that should be retained.
*/
@NotNull public static final String ATTR_RETAIN_PREVIOUS_ARCHIVE_COUNT =
ATTR_PREFIX + "retain-previous-support-data-archive-count";
/**
* The name of the attribute used to specify the security level to use for
* information added to the support data archive.
*/
@NotNull public static final String ATTR_SECURITY_LEVEL =
ATTR_PREFIX + "security-level";
/**
* The name of the attribute used to indicate whether to collect items
* sequentially rather than in parallel.
*/
@NotNull public static final String ATTR_USE_SEQUENTIAL_MODE =
ATTR_PREFIX + "use-sequential-mode";
/**
* The name of the object class used in collect support data task entries.
*/
@NotNull public static final String OC_COLLECT_SUPPORT_DATA_TASK =
"ds-task-collect-support-data";
/**
* The task property that will be used for the comment.
*/
@NotNull static final TaskProperty PROPERTY_COMMENT =
new TaskProperty(ATTR_COMMENT, INFO_CSD_DISPLAY_NAME_COMMENT.get(),
INFO_CSD_DESCRIPTION_COMMENT.get(), String.class, false, false,
false);
/**
* The task property that will be used for the encryption passphrase file.
*/
@NotNull static final TaskProperty PROPERTY_ENCRYPTION_PASSPHRASE_FILE =
new TaskProperty(ATTR_ENCRYPTION_PASSPHRASE_FILE,
INFO_CSD_DISPLAY_NAME_ENC_PW_FILE.get(),
INFO_CSD_DESCRIPTION_ENC_PW_FILE.get(), String.class, false, false,
false);
/**
* The task property that will be used for the include binary files flag.
*/
@NotNull static final TaskProperty PROPERTY_INCLUDE_BINARY_FILES =
new TaskProperty(ATTR_INCLUDE_BINARY_FILES,
INFO_CSD_DISPLAY_NAME_INCLUDE_BINARY_FILES.get(),
INFO_CSD_DESCRIPTION_INCLUDE_BINARY_FILES.get(), Boolean.class, false,
false, false);
/**
* The task property that will be used for the include expensive data flag.
*/
@NotNull static final TaskProperty PROPERTY_INCLUDE_EXPENSIVE_DATA =
new TaskProperty(ATTR_INCLUDE_EXPENSIVE_DATA,
INFO_CSD_DISPLAY_NAME_INCLUDE_EXPENSIVE_DATA.get(),
INFO_CSD_DESCRIPTION_INCLUDE_EXPENSIVE_DATA.get(), Boolean.class,
false, false, false);
/**
* The task property that will be used for the include extension source flag.
*/
@NotNull static final TaskProperty PROPERTY_INCLUDE_EXTENSION_SOURCE =
new TaskProperty(ATTR_INCLUDE_EXTENSION_SOURCE,
INFO_CSD_DISPLAY_NAME_INCLUDE_EXTENSION_SOURCE.get(),
INFO_CSD_DESCRIPTION_INCLUDE_EXTENSION_SOURCE.get(), Boolean.class,
false, false, false);
/**
* The task property that will be used for the include replication state dump
* flag.
*/
@NotNull static final TaskProperty PROPERTY_INCLUDE_REPLICATION_STATE_DUMP =
new TaskProperty(ATTR_INCLUDE_REPLICATION_STATE_DUMP,
INFO_CSD_DISPLAY_NAME_INCLUDE_REPLICATION_STATE_DUMP.get(),
INFO_CSD_DESCRIPTION_INCLUDE_REPLICATION_STATE_DUMP.get(),
Boolean.class, false, false, false);
/**
* The task property that will be used for the jstack count.
*/
@NotNull static final TaskProperty PROPERTY_JSTACK_COUNT =
new TaskProperty(ATTR_JSTACK_COUNT,
INFO_CSD_DISPLAY_NAME_JSTACK_COUNT.get(),
INFO_CSD_DESCRIPTION_JSTACK_COUNT.get(),
Long.class, false, false, false);
/**
* The task property that will be used for the log duration.
*/
@NotNull static final TaskProperty PROPERTY_LOG_DURATION =
new TaskProperty(ATTR_LOG_DURATION,
INFO_CSD_DISPLAY_NAME_LOG_DURATION.get(),
INFO_CSD_DESCRIPTION_LOG_DURATION.get(),
String.class, false, false, false);
/**
* The task property that will be used for the log head size.
*/
@NotNull static final TaskProperty PROPERTY_LOG_FILE_HEAD_COLLECTION_SIZE_KB =
new TaskProperty(ATTR_LOG_FILE_HEAD_COLLECTION_SIZE_KB,
INFO_CSD_DISPLAY_NAME_LOG_HEAD_SIZE_KB.get(),
INFO_CSD_DESCRIPTION_LOG_HEAD_SIZE_KB.get(),
Long.class, false, false, false);
/**
* The task property that will be used for the log tail size.
*/
@NotNull static final TaskProperty PROPERTY_LOG_FILE_TAIL_COLLECTION_SIZE_KB =
new TaskProperty(ATTR_LOG_FILE_TAIL_COLLECTION_SIZE_KB,
INFO_CSD_DISPLAY_NAME_LOG_TAIL_SIZE_KB.get(),
INFO_CSD_DESCRIPTION_LOG_TAIL_SIZE_KB.get(),
Long.class, false, false, false);
/**
* The task property that will be used for the output path.
*/
@NotNull static final TaskProperty PROPERTY_OUTPUT_PATH =
new TaskProperty(ATTR_OUTPUT_PATH,
INFO_CSD_DISPLAY_NAME_OUTPUT_PATH.get(),
INFO_CSD_DESCRIPTION_OUTPUT_PATH.get(),
String.class, false, false, false);
/**
* The task property that will be used for the report count.
*/
@NotNull static final TaskProperty PROPERTY_REPORT_COUNT =
new TaskProperty(ATTR_REPORT_COUNT,
INFO_CSD_DISPLAY_NAME_REPORT_COUNT.get(),
INFO_CSD_DESCRIPTION_REPORT_COUNT.get(),
Long.class, false, false, false);
/**
* The task property that will be used for the report interval.
*/
@NotNull static final TaskProperty PROPERTY_REPORT_INTERVAL_SECONDS =
new TaskProperty(ATTR_REPORT_INTERVAL_SECONDS,
INFO_CSD_DISPLAY_NAME_REPORT_INTERVAL.get(),
INFO_CSD_DESCRIPTION_REPORT_INTERVAL.get(),
Long.class, false, false, false);
/**
* The task property that will be used for the retain previous support data
* archive age.
*/
@NotNull static final TaskProperty PROPERTY_RETAIN_PREVIOUS_ARCHIVE_AGE =
new TaskProperty(ATTR_RETAIN_PREVIOUS_ARCHIVE_AGE,
INFO_CSD_DISPLAY_NAME_RETAIN_PREVIOUS_ARCHIVE_AGE.get(),
INFO_CSD_DESCRIPTION_RETAIN_PREVIOUS_ARCHIVE_AGE.get(),
String.class, false, false, false);
/**
* The task property that will be used for the retain previous support data
* archive count.
*/
@NotNull static final TaskProperty PROPERTY_RETAIN_PREVIOUS_ARCHIVE_COUNT =
new TaskProperty(ATTR_RETAIN_PREVIOUS_ARCHIVE_COUNT,
INFO_CSD_DISPLAY_NAME_RETAIN_PREVIOUS_ARCHIVE_COUNT.get(),
INFO_CSD_DESCRIPTION_RETAIN_PREVIOUS_ARCHIVE_COUNT.get(),
Long.class, false, false, false);
/**
* The task property that will be used for the security level.
*/
@NotNull static final TaskProperty PROPERTY_SECURITY_LEVEL =
new TaskProperty(ATTR_SECURITY_LEVEL,
INFO_CSD_DISPLAY_NAME_SECURITY_LEVEL.get(),
INFO_CSD_DESCRIPTION_SECURITY_LEVEL.get(
CollectSupportDataSecurityLevel.NONE.getName(),
CollectSupportDataSecurityLevel.OBSCURE_SECRETS.getName(),
CollectSupportDataSecurityLevel.MAXIMUM.getName()),
String.class, false, false, false,
new Object[]
{
CollectSupportDataSecurityLevel.NONE.getName(),
CollectSupportDataSecurityLevel.OBSCURE_SECRETS.getName(),
CollectSupportDataSecurityLevel.MAXIMUM.getName()
});
/**
* The task property that will be used for the use sequential mode flag.
*/
@NotNull static final TaskProperty PROPERTY_USE_SEQUENTIAL_MODE =
new TaskProperty(ATTR_USE_SEQUENTIAL_MODE,
INFO_CSD_DISPLAY_NAME_USE_SEQUENTIAL_MODE.get(),
INFO_CSD_DESCRIPTION_USE_SEQUENTIAL_MODE.get(),
Boolean.class, false, false, false);
/**
* The serial version UID for this serializable class.
*/
private static final long serialVersionUID = -3414981969721886291L;
// Indicates whether to include binary files in the support data archive.
@Nullable private final Boolean includeBinaryFiles;
// Indicates whether to include expensive data in the support data archive.
@Nullable private final Boolean includeExpensiveData;
// Indicates whether to include third-party extension source code in the
// support data archive.
@Nullable private final Boolean includeExtensionSource;
// Indicates whether to include a replication state dump in the support data
// archive.
@Nullable private final Boolean includeReplicationStateDump;
// Indicates whether to capture information sequentially rather than in
// parallel.
@Nullable private final Boolean useSequentialMode;
// The security level to use for data included in the support data archive.
@Nullable private final CollectSupportDataSecurityLevel securityLevel;
// The number of jstacks to include in the support data archive.
@Nullable private final Integer jstackCount;
// The amount of data in kilobytes to capture from the beginning of each log
// file.
@Nullable private final Integer logFileHeadCollectionSizeKB;
// The amount of data in kilobytes to capture from the end of each log file.
@Nullable private final Integer logFileTailCollectionSizeKB;
// The report count to use for sampled metrics.
@Nullable private final Integer reportCount;
// The report interval, in seconds, to use for sampled metrics.
@Nullable private final Integer reportIntervalSeconds;
// The minimum number of existing support data archives that should be
// retained.
@Nullable private final Integer retainPreviousSupportDataArchiveCount;
// A comment to include in the support data archive.
@Nullable private final String comment;
// The path to the encryption passphrase file.
@Nullable private final String encryptionPassphraseFile;
// A string representation of the log duration to capture.
@Nullable private final String logDuration;
// The path to which the support data archive should be written.
@Nullable private final String outputPath;
// The minimum age for existing support data archives that should be retained.
@Nullable private final String retainPreviousSupportDataArchiveAge;
/**
* Creates a new collect support data task instance that will use default
* settings for all properties. This instance may be used to invoke the
* task, but it can also be used for obtaining general information about this
* task, including the task name, description, and supported properties.
*/
public CollectSupportDataTask()
{
this(new CollectSupportDataTaskProperties());
}
/**
* Creates a new collect support data task instance using the provided
* properties.
*
* @param properties The properties to use to create the collect support
* data task. It must not be {@code null}.
*/
public CollectSupportDataTask(
@NotNull final CollectSupportDataTaskProperties properties)
{
super(properties.getTaskID(), COLLECT_SUPPORT_DATA_TASK_CLASS,
properties.getScheduledStartTime(), properties.getDependencyIDs(),
properties.getFailedDependencyAction(), properties.getNotifyOnStart(),
properties.getNotifyOnCompletion(), properties.getNotifyOnSuccess(),
properties.getNotifyOnError(), properties.getAlertOnStart(),
properties.getAlertOnSuccess(), properties.getAlertOnError());
includeBinaryFiles = properties.getIncludeBinaryFiles();
includeExpensiveData = properties.getIncludeExpensiveData();
includeExtensionSource = properties.getIncludeExtensionSource();
includeReplicationStateDump = properties.getIncludeReplicationStateDump();
useSequentialMode = properties.getUseSequentialMode();
securityLevel = properties.getSecurityLevel();
jstackCount = properties.getJStackCount();
logFileHeadCollectionSizeKB = properties.getLogFileHeadCollectionSizeKB();
logFileTailCollectionSizeKB = properties.getLogFileTailCollectionSizeKB();
reportCount = properties.getReportCount();
reportIntervalSeconds = properties.getReportIntervalSeconds();
retainPreviousSupportDataArchiveCount =
properties.getRetainPreviousSupportDataArchiveCount();
comment = properties.getComment();
encryptionPassphraseFile = properties.getEncryptionPassphraseFile();
logDuration = properties.getLogDuration();
outputPath = properties.getOutputPath();
retainPreviousSupportDataArchiveAge =
properties.getRetainPreviousSupportDataArchiveAge();
}
/**
* Creates a new collect support data task from the provided entry.
*
* @param entry The entry to use to create this collect support data task.
*
* @throws TaskException If the provided entry cannot be parsed as a collect
* support data task entry.
*/
public CollectSupportDataTask(@NotNull final Entry entry)
throws TaskException
{
super(entry);
includeBinaryFiles =
entry.getAttributeValueAsBoolean(ATTR_INCLUDE_BINARY_FILES);
includeExpensiveData =
entry.getAttributeValueAsBoolean(ATTR_INCLUDE_EXPENSIVE_DATA);
includeExtensionSource =
entry.getAttributeValueAsBoolean(ATTR_INCLUDE_EXTENSION_SOURCE);
includeReplicationStateDump =
entry.getAttributeValueAsBoolean(ATTR_INCLUDE_REPLICATION_STATE_DUMP);
useSequentialMode =
entry.getAttributeValueAsBoolean(ATTR_USE_SEQUENTIAL_MODE);
jstackCount = entry.getAttributeValueAsInteger(ATTR_JSTACK_COUNT);
logFileHeadCollectionSizeKB = entry.getAttributeValueAsInteger(
ATTR_LOG_FILE_HEAD_COLLECTION_SIZE_KB);
logFileTailCollectionSizeKB = entry.getAttributeValueAsInteger(
ATTR_LOG_FILE_TAIL_COLLECTION_SIZE_KB);
reportCount = entry.getAttributeValueAsInteger(ATTR_REPORT_COUNT);
reportIntervalSeconds =
entry.getAttributeValueAsInteger(ATTR_REPORT_INTERVAL_SECONDS);
retainPreviousSupportDataArchiveCount =
entry.getAttributeValueAsInteger(ATTR_RETAIN_PREVIOUS_ARCHIVE_COUNT);
comment = entry.getAttributeValue(ATTR_COMMENT);
encryptionPassphraseFile =
entry.getAttributeValue(ATTR_ENCRYPTION_PASSPHRASE_FILE);
outputPath = entry.getAttributeValue(ATTR_OUTPUT_PATH);
final String securityLevelStr =
entry.getAttributeValue(ATTR_SECURITY_LEVEL);
if (securityLevelStr == null)
{
securityLevel = null;
}
else
{
securityLevel = CollectSupportDataSecurityLevel.forName(securityLevelStr);
if (securityLevel == null)
{
throw new TaskException(ERR_CSD_ENTRY_INVALID_SECURITY_LEVEL.get(
entry.getDN(), securityLevelStr, ATTR_SECURITY_LEVEL,
CollectSupportDataSecurityLevel.NONE.getName(),
CollectSupportDataSecurityLevel.OBSCURE_SECRETS.getName(),
CollectSupportDataSecurityLevel.MAXIMUM.getName()));
}
}
logDuration = entry.getAttributeValue(ATTR_LOG_DURATION);
if (logDuration != null)
{
try
{
DurationArgument.parseDuration(logDuration, TimeUnit.MILLISECONDS);
}
catch (final Exception e)
{
Debug.debugException(e);
throw new TaskException(
ERR_CSD_ENTRY_INVALID_DURATION.get(entry.getDN(), logDuration,
ATTR_LOG_DURATION),
e);
}
}
retainPreviousSupportDataArchiveAge =
entry.getAttributeValue(ATTR_RETAIN_PREVIOUS_ARCHIVE_AGE);
if (retainPreviousSupportDataArchiveAge != null)
{
try
{
DurationArgument.parseDuration(retainPreviousSupportDataArchiveAge,
TimeUnit.MILLISECONDS);
}
catch (final Exception e)
{
Debug.debugException(e);
throw new TaskException(
ERR_CSD_ENTRY_INVALID_DURATION.get(entry.getDN(),
retainPreviousSupportDataArchiveAge,
ATTR_RETAIN_PREVIOUS_ARCHIVE_AGE),
e);
}
}
}
/**
* Creates a new collect support data task from the provided set of task
* properties.
*
* @param properties The set of task properties and their corresponding
* values to use for the task. It must not be
* {@code null}.
*
* @throws TaskException If the provided set of properties cannot be used to
* create a valid collect support data task.
*/
public CollectSupportDataTask(
@NotNull final Map> properties)
throws TaskException
{
super(COLLECT_SUPPORT_DATA_TASK_CLASS, properties);
Boolean includeBinary = null;
Boolean includeExpensive = null;
Boolean includeReplicationState = null;
Boolean includeSource = null;
Boolean sequentialMode = null;
CollectSupportDataSecurityLevel secLevel = null;
Integer logHeadSizeKB = null;
Integer logTailSizeKB = null;
Integer numJStacks = null;
Integer numReports = null;
Integer reportIntervalSecs = null;
Integer retainCount = null;
String commentStr = null;
String encPWFile = null;
String logDurationStr = null;
String outputPathStr = null;
String retainAge = null;
for (final Map.Entry> entry :
properties.entrySet())
{
final TaskProperty p = entry.getKey();
final String attrName = StaticUtils.toLowerCase(p.getAttributeName());
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy