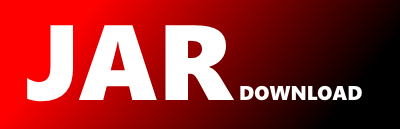
com.undefinedlabs.scope.rules.sql.model.ConnectionInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scope-rule-java-sql Show documentation
Show all versions of scope-rule-java-sql Show documentation
Scope is a APM for tests to give engineering teams unprecedented visibility into their CI process to quickly identify, troubleshoot and fix failed builds.
This artifact contains the classes to instrument the Java SQL package.
package com.undefinedlabs.scope.rules.sql.model;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
public class ConnectionInfo {
public static final ConnectionInfo EMPTY = new ConnectionInfo();
private final String url;
private final String userName;
private final String instance;
private final String productName;
private final String productVersion;
private final String driverName;
private final String driverVersion;
private final String peerService;
ConnectionInfo(){
this.url = "";
this.userName = "";
this.instance = "";
this.productName = "";
this.productVersion = "";
this.driverName = "";
this.driverVersion = "";
this.peerService = "";
}
ConnectionInfo(final Builder builder){
this.url = builder.url;
this.userName = builder.userName;
this.instance = builder.instance;
this.productName = builder.productName;
this.productVersion = builder.productVersion;
this.driverName = builder.driverName;
this.driverVersion = builder.driverVersion;
this.peerService = builder.peerService;
}
public static Builder newBuilder() {
return new Builder();
}
public String getUrl() {
return url;
}
public String getUserName() {
return userName;
}
public String getInstance() {
return instance;
}
public String getProductName() {
return productName;
}
public String getProductVersion() {
return productVersion;
}
public String getDriverName() {
return driverName;
}
public String getDriverVersion() {
return driverVersion;
}
public String getPeerService() { return peerService; }
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ConnectionInfo that = (ConnectionInfo) o;
return new EqualsBuilder()
.append(url, that.url)
.append(userName, that.userName)
.append(instance, that.instance)
.append(productName, that.productName)
.append(productVersion, that.productVersion)
.append(driverName, that.driverName)
.append(driverVersion, that.driverVersion)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37)
.append(url)
.append(userName)
.append(instance)
.append(productName)
.append(productVersion)
.append(driverName)
.append(driverVersion)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("url", url)
.append("userName", userName)
.append("instance", instance)
.append("productName", productName)
.append("productVersion", productVersion)
.append("driverName", driverName)
.append("driverVersion", driverVersion)
.toString();
}
public static class Builder {
private String url;
private String userName;
private String instance;
private String productName;
private String productVersion;
private String driverName;
private String driverVersion;
private String peerService;
public Builder withUrl(final String url) {
this.url = url;
return this;
}
public Builder withUserName(final String userName) {
this.userName = userName;
return this;
}
public Builder withInstance(final String instance) {
this.instance = instance;
return this;
}
public Builder withProductName(final String productName) {
this.productName = productName;
return this;
}
public Builder withProductVersion(final String productVersion) {
this.productVersion = productVersion;
return this;
}
public Builder withDriverName(final String driverName) {
this.driverName = driverName;
return this;
}
public Builder withDriverVersion(final String driverVersion) {
this.driverVersion = driverVersion;
return this;
}
public Builder withPeerService(final String peerService) {
this.peerService = peerService;
return this;
}
public ConnectionInfo build() {
return new ConnectionInfo(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy