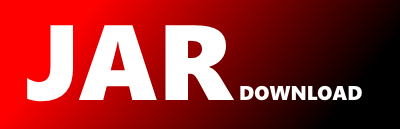
com.undefinedlabs.scope.rules.sql.model.PreparedStatementQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scope-rule-java-sql Show documentation
Show all versions of scope-rule-java-sql Show documentation
Scope is a APM for tests to give engineering teams unprecedented visibility into their CI process to quickly identify, troubleshoot and fix failed builds.
This artifact contains the classes to instrument the Java SQL package.
package com.undefinedlabs.scope.rules.sql.model;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.Map;
public class PreparedStatementQuery {
public static final PreparedStatementQuery EMPTY = new PreparedStatementQuery.Builder().build();
private final String sqlMethod;
private final String sqlPreparedStatement;
private final Map sqlParameterMap;
private final String sqlStatement;
PreparedStatementQuery(final Builder builder){
this.sqlMethod = builder.sqlMethod;
this.sqlPreparedStatement = builder.sqlPreparedStatement;
this.sqlParameterMap = builder.sqlParameterMap;
this.sqlStatement = builder.sqlStatement;
}
public String getSqlMethod() {
return sqlMethod;
}
public String getSqlPreparedStatement() {
return sqlPreparedStatement;
}
public Map getSqlParameterMap() {
return sqlParameterMap;
}
public String getSqlStatement() {
return sqlStatement;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PreparedStatementQuery that = (PreparedStatementQuery) o;
return new EqualsBuilder()
.append(sqlMethod, that.sqlMethod)
.append(sqlStatement, that.sqlStatement)
.append(sqlParameterMap, that.sqlParameterMap)
.append(sqlStatement, that.sqlStatement)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37)
.append(sqlMethod)
.append(sqlPreparedStatement)
.append(sqlParameterMap)
.append(sqlStatement)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("sqlMethod", sqlMethod)
.append("sqlPreparedStatement", sqlPreparedStatement)
.append("sqlParameterMap", sqlParameterMap)
.append("sqlStatement", sqlStatement)
.toString();
}
public static Builder newBuilder() {
return new Builder();
}
public static class Builder {
private String sqlMethod;
private String sqlPreparedStatement;
private Map sqlParameterMap;
private String sqlStatement;
public Builder withSqlStatement(final String sqlStatement){
this.sqlStatement = sqlStatement;
return this;
}
public Builder withSqlMethod(final String sqlMethod) {
this.sqlMethod = sqlMethod;
return this;
}
public Builder withSqlPreparedStatement(final String sqlPreparedStatement){
this.sqlPreparedStatement = sqlPreparedStatement;
return this;
}
public Builder withSqlParameterMap(final Map sqlParameterMap){
this.sqlParameterMap = sqlParameterMap;
return this;
}
public PreparedStatementQuery build() {
return new PreparedStatementQuery(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy