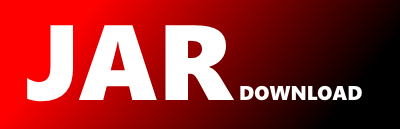
com.undefinedlabs.scope.rules.events.log.Slf4jLogEventFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scope-rule-slf4j Show documentation
Show all versions of scope-rule-slf4j Show documentation
Scope is a APM for tests to give engineering teams unprecedented visibility into their CI process to quickly
identify, troubleshoot and fix failed builds.
This artifact contains the classes to instrument the SLF4J logging framework.
The newest version!
package com.undefinedlabs.scope.rules.events.log;
import com.undefinedlabs.scope.events.log.LogEvent;
import com.undefinedlabs.scope.events.log.LogLevel;
import com.undefinedlabs.scope.utils.JarUtils;
import com.undefinedlabs.scope.utils.sourcecode.SourceCodeFrame;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.slf4j.Logger;
import org.slf4j.Marker;
public enum Slf4jLogEventFactory {
INSTANCE;
public LogEvent newEvent(
final Logger logger,
final Object[] logArgs,
final LogLevel logLevel,
final SourceCodeFrame sourceCodeFrame) {
final LogEvent.Builder builder = LogEvent.newBuilder();
builder.withLoggerName(logger.getName());
builder.withLoggerModule(JarUtils.resolveJarName(logger.getClass()));
builder.withMessage(buildMessage(logArgs));
builder.withLogLevel(logLevel);
builder.withLogMarker(buildMarker(logArgs));
builder.withSource(sourceCodeFrame);
return builder.build();
}
private String buildMarker(final Object[] logArgs) {
if (logArgs == null || logArgs.length == 0) {
return null;
}
return (logArgs[0] instanceof Marker) ? ((Marker) logArgs[0]).getName() : null;
}
private String buildMessage(final Object[] logArgs) {
if (logArgs == null || logArgs.length == 0) {
return null;
}
// Optimization: Most of log interaction will be this one
if (logArgs[0] instanceof String && logArgs.length == 1) {
return (String) logArgs[0];
}
// If Marker exists, it is invoked recursively without marker in logArgs.
if (logArgs[0] instanceof Marker) {
final Object[] logArgsWithoutMarker = new Object[logArgs.length - 1];
System.arraycopy(logArgs, 1, logArgsWithoutMarker, 0, logArgs.length - 1);
return buildMessage(logArgsWithoutMarker);
}
// If log has been invoked using Throwable
if (logArgs[1] instanceof Throwable) {
final String baseMessage = (String) logArgs[0];
final Throwable throwable = (Throwable) logArgs[1];
final StringBuilder sb = new StringBuilder();
sb.append(baseMessage).append("\n").append(ExceptionUtils.getStackTrace(throwable));
return sb.toString();
}
// String format with default CharSequence {} in SLF4J
final String baseMessage = (String) logArgs[0];
if (StringUtils.isEmpty(baseMessage)) {
return null;
}
final Object[] logParams = extractLogParams(logArgs);
return (logParams.length > 0)
? String.format(baseMessage.replace("{}", "%s"), logParams)
: baseMessage;
}
private Object[] extractLogParams(final Object[] logArgs) {
if (logArgs[1] instanceof Object[]) {
return (Object[]) logArgs[1];
}
final Object[] logParams = new Object[logArgs.length - 1];
System.arraycopy(logArgs, 1, logParams, 0, logArgs.length - 1);
return logParams;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy