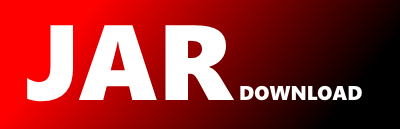
com.undefinedlabs.scope.rules.model.TestNGScopeDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scope-rule-testng Show documentation
Show all versions of scope-rule-testng Show documentation
Scope is a APM for tests to give engineering teams unprecedented visibility into their CI process to quickly identify, troubleshoot and fix failed builds.
This artifact contains the classes to instrument the TestNG tests.
package com.undefinedlabs.scope.rules.model;
import com.undefinedlabs.scope.utils.HashUtils;
import com.undefinedlabs.scope.deps.org.apache.commons.lang3.StringUtils;
import com.undefinedlabs.scope.deps.org.apache.commons.lang3.builder.EqualsBuilder;
import com.undefinedlabs.scope.deps.org.apache.commons.lang3.builder.HashCodeBuilder;
import com.undefinedlabs.scope.deps.org.apache.commons.lang3.builder.ToStringBuilder;
import org.testng.ITestResult;
import java.util.HashMap;
import java.util.Map;
public class TestNGScopeDescription {
private final String className;
private final String methodName;
private final Map parameters;
private final String hashedParameterValues;
public TestNGScopeDescription(final ITestResult testResult) {
this.className = testResult.getInstanceName();
this.methodName = testResult.getMethod().getMethodName();
this.parameters = new HashMap<>();
if (testResult.getParameters() != null) {
for (int i = 0; i < testResult.getParameters().length; i++) {
this.parameters.put(String.valueOf(i), String.valueOf(testResult.getParameters()[i]));
}
}
final StringBuilder sb = new StringBuilder();
for (String paramValue : this.parameters.values()) {
sb.append(paramValue).append(";");
}
final String paramsValues = sb.toString();
this.hashedParameterValues = StringUtils.isNotEmpty(paramsValues) ? HashUtils.sha1Hex(sb.toString(), 8) : "";
}
public String getClassName() {
return className;
}
public String getMethodName() {
return methodName;
}
public Map getParameters() {
return parameters;
}
public String getHashedParameterValues() {
return hashedParameterValues;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TestNGScopeDescription that = (TestNGScopeDescription) o;
return new EqualsBuilder()
.append(className, that.className)
.append(methodName, that.methodName)
.append(parameters, that.parameters)
.append(hashedParameterValues, that.hashedParameterValues)
.isEquals();
}
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37)
.append(className)
.append(methodName)
.append(parameters)
.append(hashedParameterValues)
.toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("className", className)
.append("methodName", methodName)
.append("parameters", parameters)
.append("hashedParameterValues", hashedParameterValues)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy